一、背景:可视化大屏通常需要用到自动滚动的效果,本文主要采用的是vue-seamless-scroll 组件来实现(可参考官方文档)
二、实现效果:
自动滚动
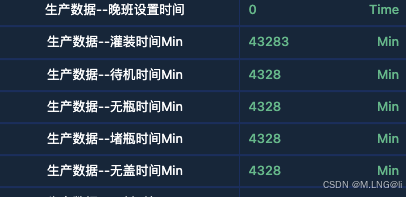
三、代码实现:
解题思路:
1.先安装依赖包
npm install vue-seamless-scroll --save
或
yarn add vue-seamless-scroll
或
<script src="vue-seamless-scroll.min.js"></script>
2.完整源码实现如下:
<template>
<div class="mb-1" v-if="props.title">
<span class="eq-blue-button">{{ props.title }}</span>
</div>
<vue3-seamless-scroll
class="scroll"
:class="heightClass"
:list="list"
:hover="scrollOption.hover"
:step="scrollOption.setp"
:direction="scrollOption.direction"
:limitScrollNum="scrollOption.limitScrollNum"
>
<!-- <slot name="slotScroll" :list="list"></slot> 注:如果想要封装成组件可以使用插槽方式 -->
<!-- 下面代码为具体业务的页面显示代码-->
<div class="eq-list" v-for="(item, index) in list" :key="index">
<div class="eq-tr">{{ item.name }}</div>
<div
class="eq-tr2 flex" :class="[isShowUnit? 'justify-between': 'justify-center']"
:style="{
color: textColor,
'font-Weight': props.fontWeight,
'font-size': props.fontSize,
}"
>
<span>{{ item.value }}</span>
<span>{{ item.unit }}</span>
</div>
</div>
</vue3-seamless-scroll>
</template>
<script lang="ts" setup>
import { ref,computed } from 'vue';
import { Vue3SeamlessScroll } from 'vue3-seamless-scroll';
const props = defineProps({
list: { //数据源 自动滚动 必传
type: Array,
default: () => [],
},
scrollOptions: { // 滚动的配置 比如 滚动方向、滚动达到什么数量开始滚动等
type: Object,
default: () => ({}),
},
heightClass: { // 滚动的高度 可以自己写 比如换成 .名称{height: '对应的高度'}
type: String,
default: "heightMidel"
},
title: {
type: String,
default: '',
},
textColor: {
type: String,
default: '#3cc094',
},
fontSize: {
type: String,
default: '',
},
fontWeight: {
type: String,
default: '500',
},
key: {
type: String,
default: '0',
},
isShowUnit: {
type: Boolean,
default: false,
}
});
const scrollOption = ref({
hover: true, // 鼠标经过触发的事件 默认是true
setp: 0.3, // 数值越大速度滚动越快
limitScrollNum: 8, // 开始无缝滚动的数据量 默认5
hoverStop: true, // 是否开启鼠标悬停stop
direction: 'up', // down向下 up向上 left 向左 right向右
openWatch: true, // 开启数据实时监控刷新dom
singleHeight: 10, //单步运动停止的高度(默认值0是无缝不停止的滚动)
...props.scrollOptions
})
</script>
<style lang="less" scoped>
.scroll {
overflow: hidden;
}
.heightMidel {
height: 36vh;
}
.heightLarge {
height: 51vh;
}
.eq-box {
color: #fff;
margin: 0.6rem 0.3rem;
}
.eq-border-box {
border: 1px solid #135daf;
padding-right: 4px;
}
.eq-center {
margin: 0px 5px;
border: 1px solid #135daf;
}
.eq-blue-button {
background: #2047b8;
padding: 4px 16px;
font-size: 0.8rem;
font-weight: 500;
}
.eq-list {
display: flex;
border: 1px solid #1857f133;
width: 100%;
}
.eq-tr {
width: 60%;
padding: 5px 8px;
border-right: 1px solid #1857f133;
font-size: 0.8rem;
text-align: center;
}
.eq-tr2 {
width: 40%;
padding: 5px 8px;
font-size: 0.8rem;
}
.text-green {
color: #3de5ad;
}
.border-left {
border-left: 1px solid #1857f133;
}
</style>