OCR识别行驶证(阿里云和百度云)
一、使用场景
1、通过识别行驶证,获取相关汽车信息,替代手输
二、效果图
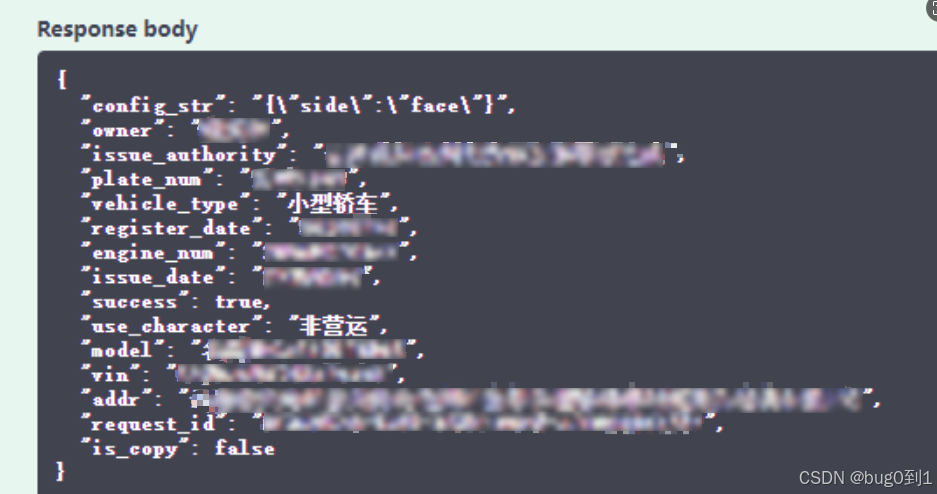
三、代码部分:
1、阿里云OCR
1.1、控制层
@PostMapping("/ocrCard")
public JSONObject ocrCard(@RequestPart("file") MultipartFile file, String type){
//正面为face,反面为back
JSONObject jsonObject = OcrUtils.ocrCard(file,type);
return jsonObject;
}
1.2、OcrUtils工具类
public class OcrUtils {
public static final String host = "https://driving.market.alicloudapi.com";
public static final String path = "/rest/160601/ocr/ocr_vehicle.json";
public static final String appcode = "替换成自己申请的";
/**
* type是前端传值,face为正面,face为反面
*/
public static JSONObject ocrCard(MultipartFile file,String type){
String imgBase64 = encodeImageToBase64(file);
JSONObject jsonObject = new JSONObject();
//如果文档的输入中含有inputs字段,设置为True, 否则设置为False
Boolean is_old_format = false;
//请根据线上文档修改configure字段
JSONObject configObj = new JSONObject();
configObj.put("side", type);
String config_str = configObj.toString();
String method = "POST";
Map<String, String> headers = new HashMap<String, String>();
//header中的格式
headers.put("Authorization", "APPCODE " + appcode);
Map<String, String> querys = new HashMap<String, String>();
// 拼装请求body的json字符串
JSONObject requestObj = new JSONObject();
try {
if(is_old_format) {
JSONObject obj = new JSONObject();
obj.put("image", getParam(50, imgBase64));
if(config_str.length() > 0) {
obj.put("configure", getParam(50, config_str));
}
JSONArray inputArray = new JSONArray();
inputArray.add(obj);
requestObj.put("inputs", inputArray);
}else{
requestObj.put("image", imgBase64);
if(config_str.length() > 0) {
requestObj.put("configure", config_str);
}
}
} catch (JSONException e) {
e.printStackTrace();
}
String bodys = requestObj.toString();
try {
HttpResponse response = HttpUtils.doPost(host, path, method, headers, querys, bodys);
int stat = response.getStatusLine().getStatusCode();
if(stat != 200){
System.out.println("Http code: " + stat);
System.out.println("http header error msg: "+ response.getFirstHeader("X-Ca-Error-Message"));
System.out.println("Http body error msg:" + EntityUtils.toString(response.getEntity()));
return jsonObject;
}
String res = EntityUtils.toString(response.getEntity());
JSONObject res_obj = JSON.parseObject(res);
if(is_old_format) {
JSONArray outputArray = res_obj.getJSONArray("outputs");
String output = outputArray.getJSONObject(0).getJSONObject("outputValue").getString("dataValue");
JSONObject out = JSON.parseObject(output);
return out;
}else{
return res_obj;
}
} catch (Exception e) {
e.printStackTrace();
}
return jsonObject;
}
/*
* 获取参数的json对象
*/
public static JSONObject getParam(int type, String dataValue) {
JSONObject obj = new JSONObject();
try {
obj.put("dataType", type);
obj.put("dataValue", dataValue);
} catch (JSONException e) {
e.printStackTrace();
}
return obj;
}
private static String encodeImageToBase64(MultipartFile file) {
try {
byte[] content = file.getBytes();
return new String(encodeBase64(content));
} catch (IOException e) {
e.printStackTrace();
return "";
}
}
}
2、百度云OCR
2.1、控制层
@PostMapping("/ocrCardBd")
public JSONObject ocrCardBd(@RequestPart("file") MultipartFile file,String type){
JSONObject jsonObject = OcrBdUtils.ocrCard(file,type);
return jsonObject;
}
2.2、OcrBdUtils工具类
public class OcrBdUtils {
public static final String apiKey = "替换成自己的";
public static final String secretKey = "替换成自己的";
public static final String tokenUrl = "https://aip.baidubce.com/oauth/2.0/token";
public static final String apiUrl = "https://aip.baidubce.com/rest/2.0/ocr/v1/vehicle_license";
/**
* type是前端传值,front为正面(默认),back为反面
*/
public static JSONObject ocrCard(MultipartFile file, String type) {
try {
String accessToken = getAccessToken();
String apiEndpoint = apiUrl + "?access_token=" + accessToken;
// 获取文件字节数组并进行Base64编码
byte[] fileBytes = file.getBytes();
String imgStr = Base64Util.encode(fileBytes);
String imgParam = URLEncoder.encode(imgStr, "UTF-8");
// 构造请求的URL和参数
URL url = new URL(apiEndpoint);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("POST");
connection.setDoOutput(true);
connection.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
// 发送请求参数
String postData = "image=" + imgParam + "&vehicle_license_side=" + type;
connection.getOutputStream().write(postData.getBytes());
// 获取响应
InputStream inputStream = connection.getInputStream();
String response = readStream(inputStream);
return JSONObject.parseObject(response);
} catch (IOException e) {
e.printStackTrace();
return new JSONObject();
}
}
// 获取令牌
public static String getAccessToken() throws IOException {
URL url = new URL(tokenUrl + "?grant_type=client_credentials&client_id=" + apiKey + "&client_secret=" + secretKey);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
InputStream responseStream = connection.getInputStream();
String response = StreamUtils.copyToString(responseStream, java.nio.charset.StandardCharsets.UTF_8);
JSONObject jsonObject = JSONObject.parseObject(response);
return jsonObject.getString("access_token");
}
// 读取输入流的内容
private static String readStream(InputStream inputStream) throws IOException {
try (ByteArrayOutputStream outputStream = new ByteArrayOutputStream()) {
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
return outputStream.toString();
}
}
}
一个在学习中的开发者,勿喷,欢迎交流