说明
Electron + Vite + Vue3 + Element Plus
Electron
中写提示有两种方案:
- 系统级:electron带的
dialog
相关API - UI级:UI框架内部的提示,如
ElMessage
、ElMessageBox
、ElNotification
等
今天来封装一下UI级别的提示
代码
效果图
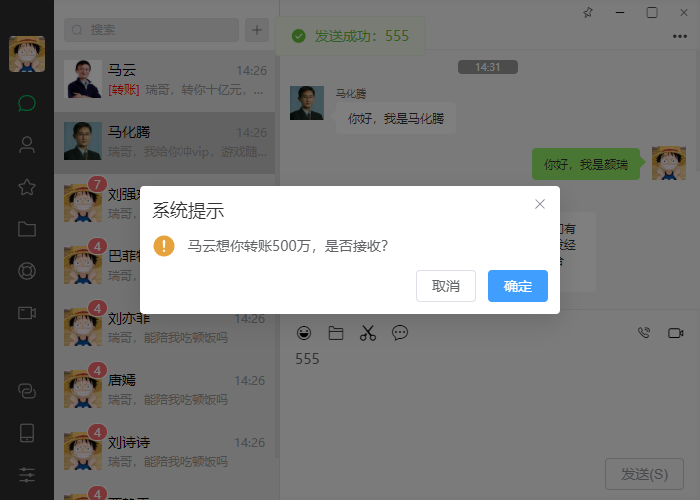
源码
代码封装在hooks中,借鉴了若依:
javascript
// src/hooks/useMessage.js
import { ElMessage, ElMessageBox, ElNotification, ElLoading } from 'element-plus'
let loadingInstance;
export const useMessage = () => {
return {
// 消息提示
info(content) {
ElMessage.info(content)
},
// 错误消息
error(content) {
ElMessage.error(content)
},
// 成功消息
success(content) {
ElMessage.success(content)
},
// 警告消息
warning(content) {
ElMessage.warning(content)
},
// 弹出提示
alert(content) {
ElMessageBox.alert(content, '系统提示')
},
// 错误提示
alertError(content) {
ElMessageBox.alert(content, '系统提示', { type: 'error' })
},
// 成功提示
alertSuccess(content) {
ElMessageBox.alert(content, '系统提示', { type: 'success' })
},
// 警告提示
alertWarning(content) {
ElMessageBox.alert(content, '系统提示', { type: 'warning' })
},
// 通知提示
notify(content) {
ElNotification.info(content)
},
// 错误通知
notifyError(content) {
ElNotification.error(content)
},
// 成功通知
notifySuccess(content) {
ElNotification.success(content)
},
// 警告通知
notifyWarning(content) {
ElNotification.warning(content)
},
// 确认窗体
confirm(content, tip) {
return ElMessageBox.confirm(content, tip ? tip : '系统提示', {
confirmButtonText: '确定',
cancelButtonText: '取消',
type: 'warning'
})
},
// 删除窗体
delConfirm(content, tip) {
return ElMessageBox.confirm(
content ? content : '是否删除所选中数据?',
tip ? tip : '系统提示',
{
confirmButtonText: '确定',
cancelButtonText: '取消',
type: 'warning'
}
)
},
// 提交内容
prompt(content, tip) {
return ElMessageBox.prompt(content, tip, {
confirmButtonText: '确定',
cancelButtonText: '取消',
type: 'warning'
})
},
// 打开遮罩层
loading(content) {
loadingInstance = ElLoading.service({
lock: true,
text: content,
background: "rgba(0, 0, 0, 0.7)",
})
},
// 关闭遮罩层
closeLoading() {
loadingInstance.close();
}
}
}
用法
vue组件中直接引用:
javascript
import { useMessage } from "@/hooks/useMessage";
const message = useMessage()
message.confirm('马云想你转账500万,是否接收?')