目录
- 四、指针
-
- [4.1 指针基础](#4.1 指针基础)
- [4.2 指针与数组](#4.2 指针与数组)
- [4.3 指针与结构](#4.3 指针与结构)
- [4.4 二重指针](#4.4 二重指针)
- [4.5 字符指针](#4.5 字符指针)
- [4.6 动态内存](#4.6 动态内存)
- 五、函数
-
- [5.1 函数参数](#5.1 函数参数)
- [5.2 函数与数组](#5.2 函数与数组)
- [5.3 函数指针](#5.3 函数指针)
- [5.4 函数与结构](#5.4 函数与结构)
- [5.5 递归函数](#5.5 递归函数)
- [5.6 作用域与存储类型](#5.6 作用域与存储类型)
- [5.7 模块化设计](#5.7 模块化设计)
四、指针
4.1 指针基础
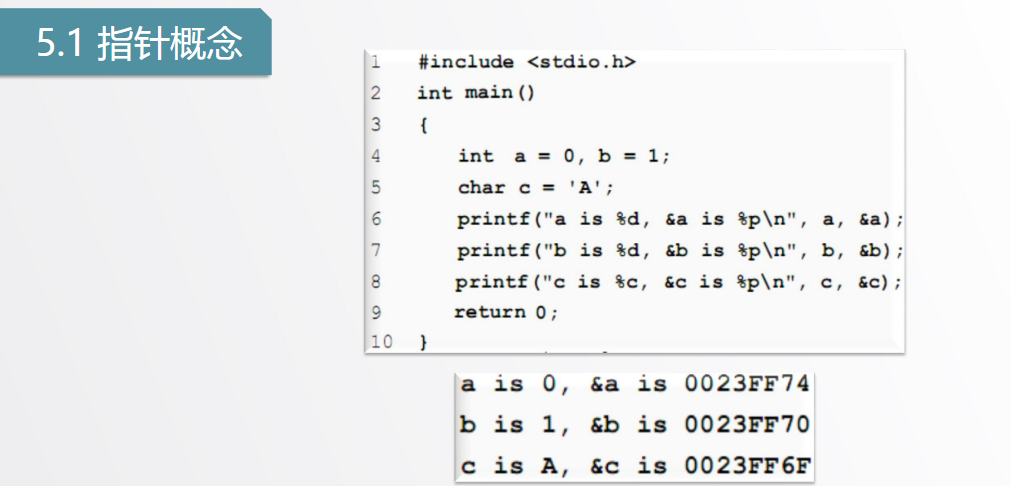
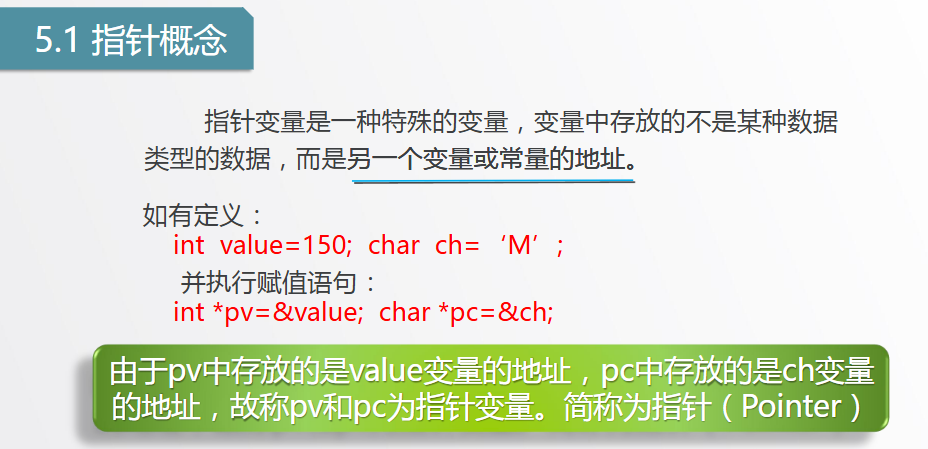
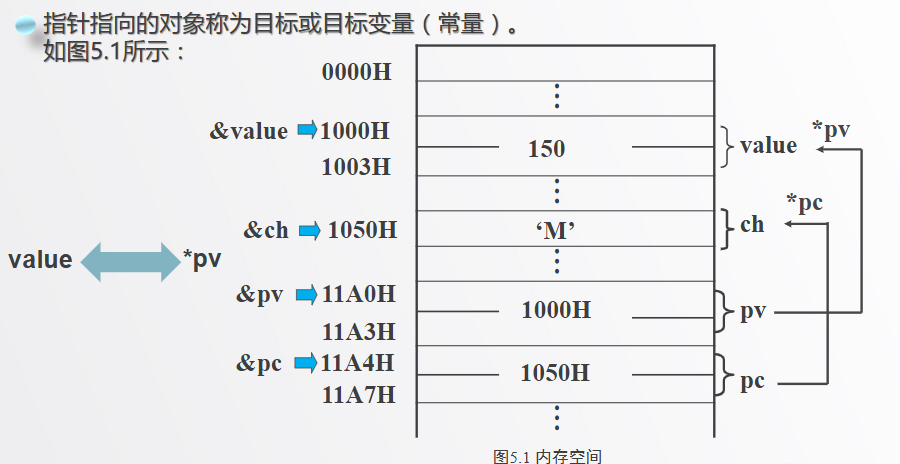
内存中的地址按字节编号,每个字节的存储单元均对应一个地址。
cpp
#include<stdio.h>
#include<stdlib.h>
int main()
{
int a,b;
scanf("%d%d",&a,&b);
int *p_max = &a,*p_min = &b,*p;
if(*p_max < *p_min){
p = p_max;
p_max = p_min;
p_min = p;
}
printf("%d %d\n",*p_min,*p_max);
return 0;
}
4.2 指针与数组
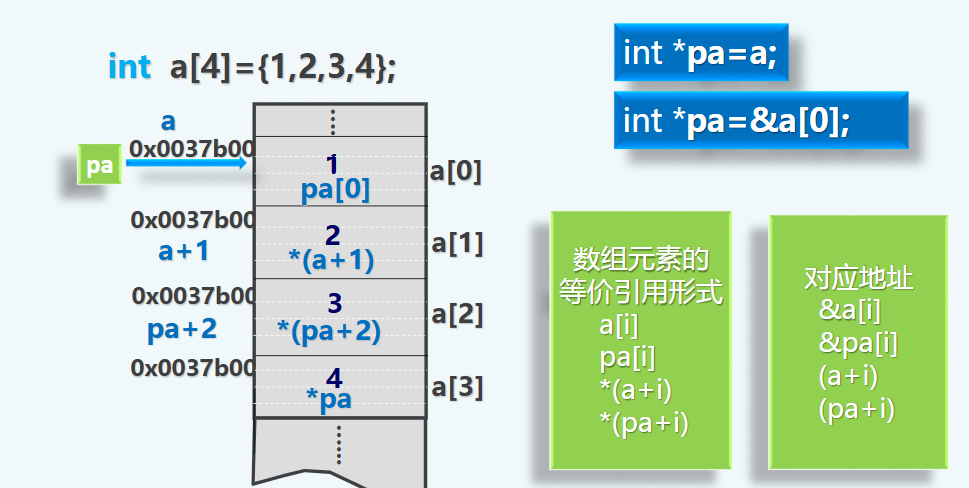
注意:数组名是指针常量,不是指针变量,不能给数组名赋值。
用指针访问数组,计算数组元素之和
cpp
#include<stdio.h>
int main()
{
int array[10] = {0,2,4,6,8,10,12,14,16,18};
int sum = 0;
int *p = array; //指针指向数组
for(int i=0;i<10;i++){
sum += *p;
p++;
}
printf("总和是%d\n",sum);
return 0;
}
注意不能写成:sum+=*array;array++;
数组名不可以执行++操作,因为它是一个常量
p++并不是将指针变量p的值简单加1,而是加上了一个基类型所占有的字节数,即移动了sizeof(int)=4个字节
我们可以通过指针移动快速访问数组,但通过指针也只能逐个处理数据,不能一次性整体处理数组所有元素。
利用数组求和的例子,总结一下有5种访问数组元素的方法:
第一种:
cpp
#include<stdio.h>
int main()
{
int array[] = {1,4,2,7,13,32,21,48,16,30};
int size = sizeof(array)/sizeof(*array),sum = 0;
for(int i=0;i<size;i++){
sum += array[i];
}
printf("%d\n",sum);
return 0;
}
第二种:
cpp
#include<stdio.h>
int main()
{
int array[] = {1,4,2,7,13,32,21,48,16,30};
int size = sizeof(array)/sizeof(*array),sum = 0;
int *p = array;
for(int i=0;i<size;i++){
sum += *p;
p++;
}
printf("%d\n",sum);
return 0;
}
第三种:
cpp
#include<stdio.h>
int main()
{
int array[] = {1,4,2,7,13,32,21,48,16,30};
int size = sizeof(array)/sizeof(*array),sum = 0;
int *p = array;
for(int i=0;i<size;i++){
sum += *(p+i);
}
printf("%d\n",sum);
return 0;
}
第四种:
cpp
#include<stdio.h>
int main()
{
int array[] = {1,4,2,7,13,32,21,48,16,30};
int size = sizeof(array)/sizeof(*array),sum = 0;
int *p = array;
for(int i=0;i<size;i++){
sum += p[i];
}
printf("%d\n",sum);
return 0;
}
第五种:
cpp
#include<stdio.h>
int main()
{
int array[] = {1,4,2,7,13,32,21,48,16,30};
int size = sizeof(array)/sizeof(*array),sum = 0;
for(int i=0;i<size;i++){
sum += *(array+i);
}
printf("%d\n",sum);
return 0;
}
指针与二维数组
二维数组元素的访问
cpp
#include<stdio.h>
int main()
{
int a[3][4] = {1,2,3,4,6,6,6,6,5,7,9,8};
int (*p)[4];
p=a;
for(int i=0;i<3;i++){
for(int j=0;j<4;j++){
printf("%d ",p[i][j]);
}
}
return 0;
}
cpp
#include<stdio.h>
int main()
{
int a[3][4] = {1,2,3,4,6,6,6,6,5,7,9,8};
int (*p)[4];
p=a;
for(int i=0;i<3;i++){
for(int j=0;j<4;j++){
printf("%d ",*(p[i]+j));
}
}
return 0;
}
cpp
#include<stdio.h>
int main()
{
int a[3][4] = {1,2,3,4,6,6,6,6,5,7,9,8};
int (*p)[4];
p=a;
for(int i=0;i<3;i++){
for(int j=0;j<4;j++){
printf("%d ",*(*(p+i)+j));
}
}
return 0;
}
cpp
#include<stdio.h>
int main()
{
int a[3][4] = {1,2,3,4,6,6,6,6,5,7,9,8};
int (*p)[4];
p=a;
for(int i=0;i<3;i++){
for(int j=0;j<4;j++){
printf("%d ",(*(p+i))[j]);
}
}
return 0;
}
用列地址初始化
cpp
#include<stdio.h>
int main()
{
int a[3][4] = {1,2,3,4,6,6,6,6,5,7,9,8};
int *p = *a;
for(int i=0;i<3;i++){
for(int j=0;j<4;j++){
printf("%d ",*(p+i*4+j));
}
}
return 0;
}
cpp
#include<stdio.h>
int main()
{
int a[3][4] = {1,2,3,4,6,6,6,6,5,7,9,8};
int *p = *a;
for(int i=0;i<3;i++){
for(int j=0;j<4;j++){
printf("%d ",p[i*4+j]);
}
}
return 0;
}
4.3 指针与结构
cpp
#include<stdio.h>
#include<string.h>
struct info{
short num;
char name[5];
};
int main()
{
struct info info1,info2;
struct info *p1 = &info1,*p2 = &info2;
p1 -> num = 2;
strcpy((*p1).name,"good");
*p2 = *p1;
printf("%d\n",p2->num);
printf("%c\n",p2->name[3]);
return 0;
}
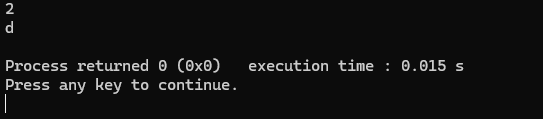
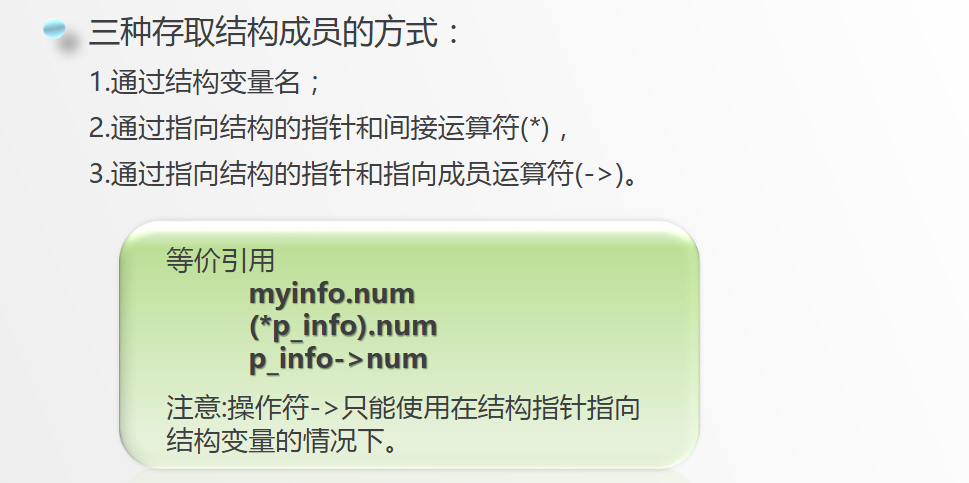
4.4 二重指针
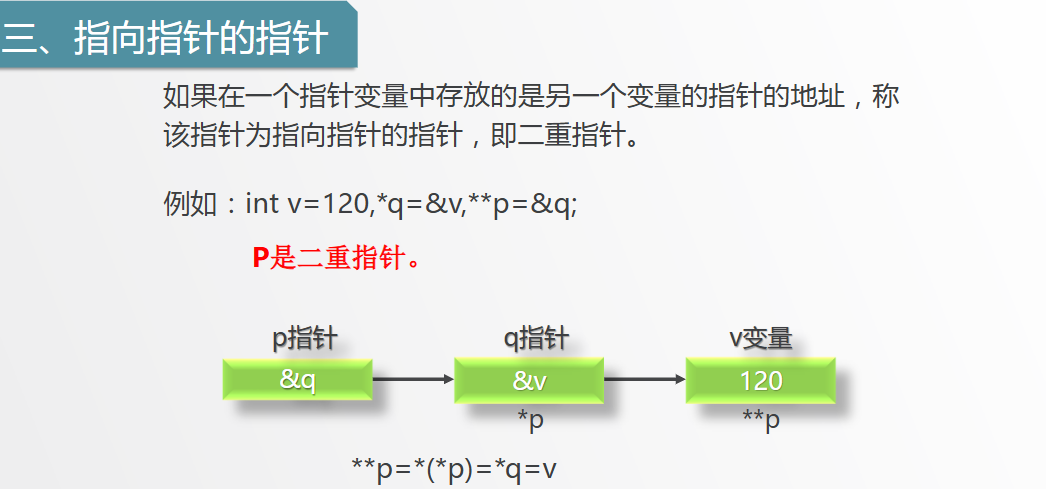
4.5 字符指针
cpp
#include<stdio.h>
#include<string.h>
int main()
{
char buffer[] = {'h','e','l','l','o'};
char *p = buffer;
printf("%s\n",p);
return 0;
}
输出:hello*****(后面是乱字符,直到最后为\0为止)
cpp
#include<stdio.h>
#include<string.h>
int main()
{
char buffer[] = {'h','e','l','l','o'};
char *p = buffer;
printf("%c\n",*p);
return 0;
}
输出:h
cpp
#include<stdio.h>
#include<string.h>
int main()
{
char buffer[] = "hello";
char *p = buffer;
printf("%s\n",p);
return 0;
}
完美输出hello
cpp
#include<stdio.h>
#include<string.h>
int main()
{
char buffer[] = "hello";
char *p = buffer;
printf("%c\n",*p);
return 0;
}
输出:h
4.6 动态内存
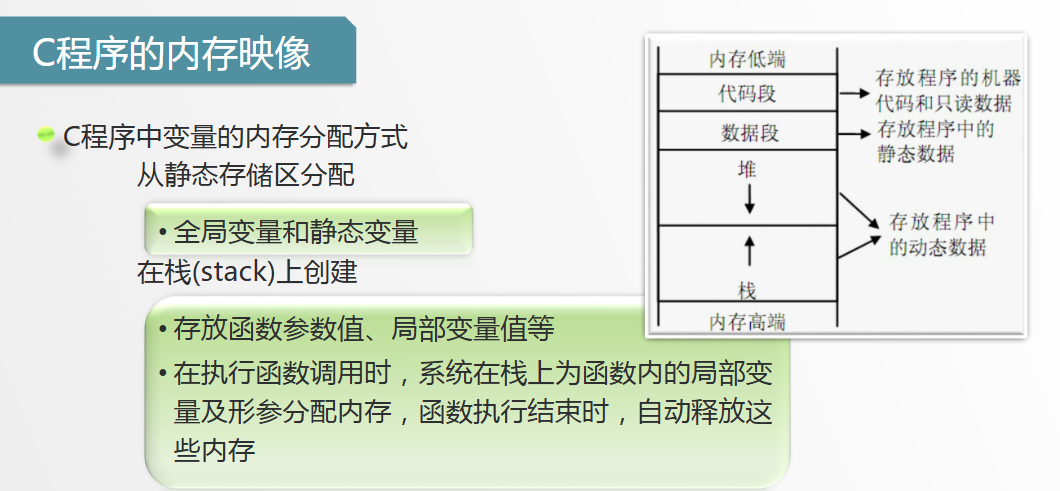
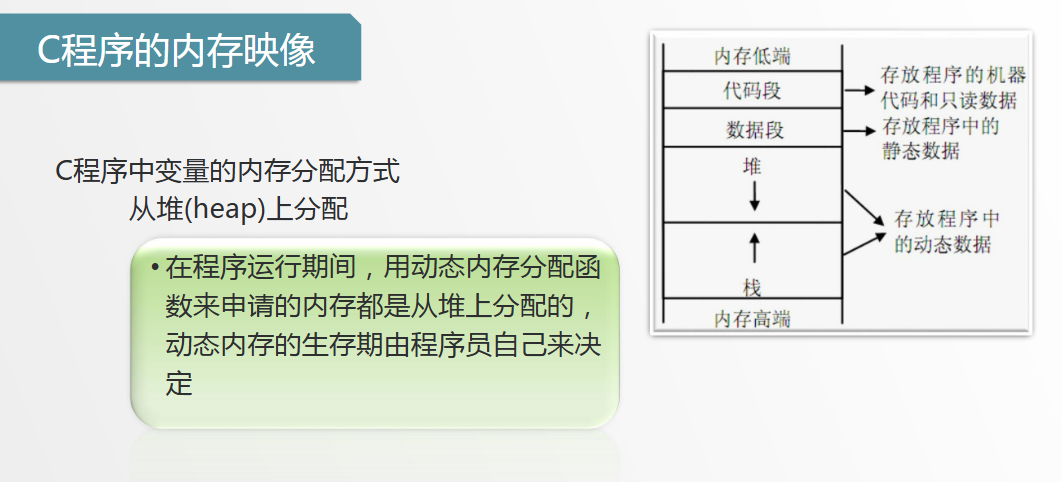
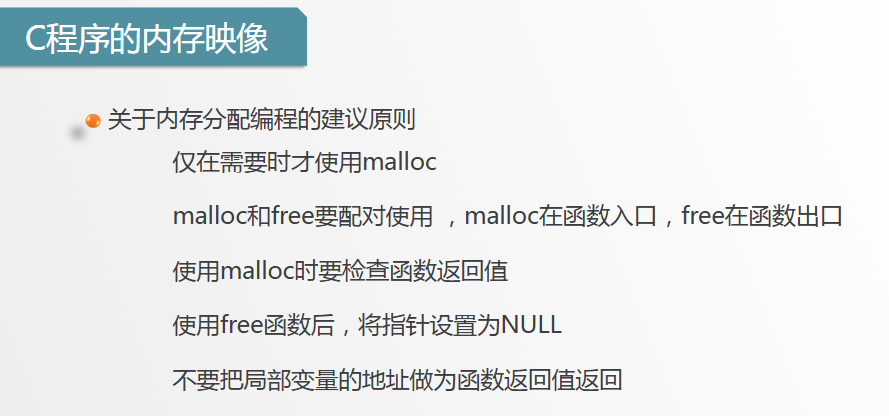
五、函数
1.返回值类型
2.函数名
3.形参表,函数入口
4.函数内部可以定义只能自己使用的变量,称内部变亘
5.返回值作为函数调用表达式的值
5.1 函数参数
5.2 函数与数组
5.3 函数指针
cpp
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
char* match(char c, char *sp);
int main()
{
char s[80],ch,*p;
int pos;
gets(s);
ch = getchar();
p = match(ch,s);
if(p){
pos = strlen(s) - strlen(p) + 1;
printf("%s %d\n",p,pos);
}
else{
printf("no match found");
}
return 0;
}
char* match(char c,char *sp){
int count = 0;
while(c!=sp[count] && sp[count]!='\0'){
count++;
}
if(sp[count]) return (&sp[count]);
return (0);
}

5.4 函数与结构
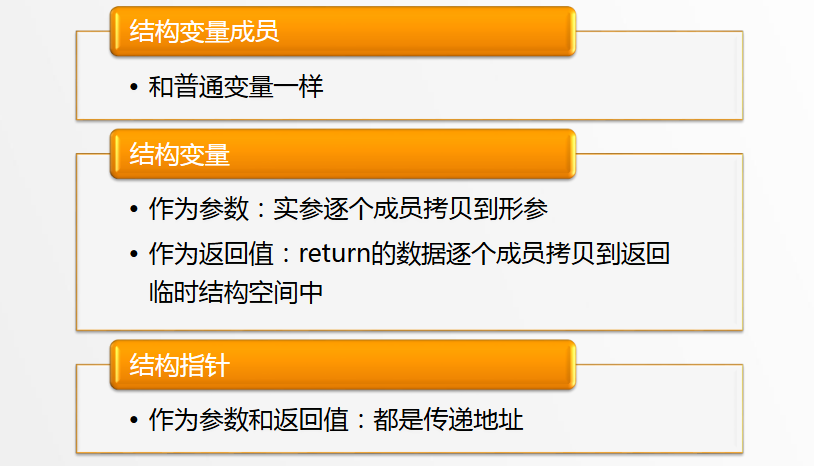
例:计算一组学生的平均成绩和不及格人数
cpp
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
struct stu{
int num;
char *name;
char sex;
float score;
};
int ave(struct stu *ps,int n,float *average);
struct stu boy[5] = {
{101,"Li ping",'M',45},
{102,"zhang ping",'M',62.5},
{103,"he fang",'F',92.5},
{104,"cheng ling",'F',87},
{105,"wang ming",'M',58}
};
int main()
{
float average;
int m;
m = ave(boy,5,&average);
printf("平均分是%.2f,未通过人数%d\n",average,m);
return 0;
}
int ave(struct stu *ps,int n,float *average){
int c = 0,i;
float ave,s = 0;
for(i=0;i<n;i++,ps++){
s+=ps->score;
if(ps->score<60) c+=1;
}
*average = s/n;
return c;
}
5.5 递归函数
5.6 作用域与存储类型
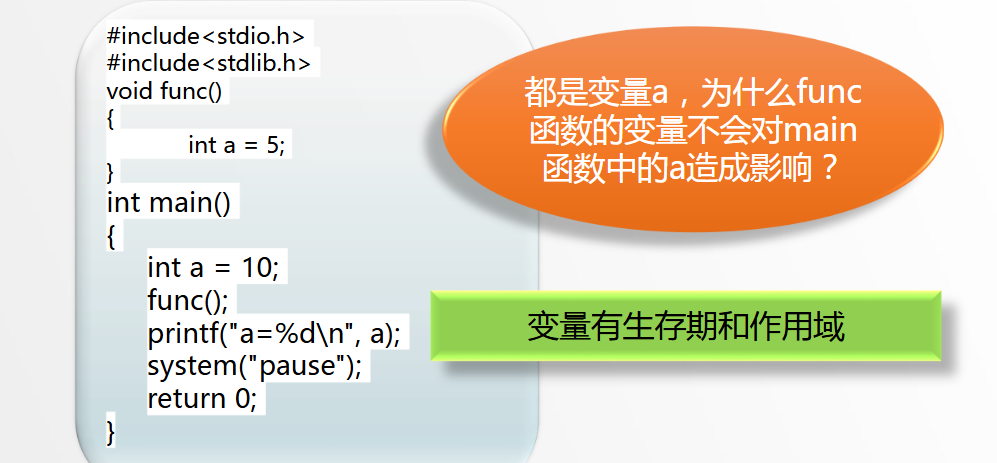
5.7 模块化设计
未完待续,后续更新C++内容敬请关注~
声明:仅供学习参考使用,不另做他用,侵删
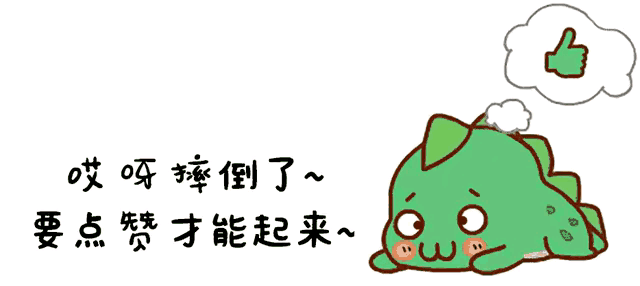