<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>贪吃蛇游戏</title>
<style>
canvas {
display: block;
margin: 20px auto;
border: 1px solid #000;
}
</style>
</head>
<body>
<canvas id="gameCanvas" width="400" height="400"></canvas>
<script>
const canvas = document.getElementById('gameCanvas');
const ctx = canvas.getContext('2d');
const boxSize = 20;
let snake = [{x: 10, y: 10}];
let food = {x: 15, y: 15};
let dx = 0, dy = 0;
function draw() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
drawSnake();
drawFood();
}
function drawSnake() {
snake.forEach(segment => {
ctx.fillStyle = 'green';
ctx.fillRect(segment.x * boxSize, segment.y * boxSize, boxSize, boxSize);
});
}
function drawFood() {
ctx.fillStyle = 'red';
ctx.fillRect(food.x * boxSize, food.y * boxSize, boxSize, boxSize);
}
function update() {
const head = {x: snake[0].x + dx, y: snake[0].y + dy};
snake.unshift(head);
if (head.x === food.x && head.y === food.y) {
food = {x: Math.floor(Math.random() * (canvas.width / boxSize)), y: Math.floor(Math.random() * (canvas.height / boxSize))};
} else {
snake.pop();
}
}
function checkCollision() {
const head = snake[0];
if (head.x < 0 || head.x >= canvas.width / boxSize || head.y < 0 || head.y >= canvas.height / boxSize) {
return true;
}
for (let i = 1; i < snake.length; i++) {
if (head.x === snake[i].x && head.y === snake[i].y) {
return true;
}
}
return false;
}
function gameLoop() {
if (checkCollision()) {
alert('游戏结束');
document.location.reload();
return;
}
update();
draw();
setTimeout(gameLoop, 100);
}
document.addEventListener('keydown', (e) => {
switch (e.key) {
case 'ArrowUp':
if (dy === 0) { dx = 0; dy = -1; }
break;
case 'ArrowDown':
if (dy === 0) { dx = 0; dy = 1; }
break;
case 'ArrowLeft':
if (dx === 0) { dx = -1; dy = 0; }
break;
case 'ArrowRight':
if (dx === 0) { dx = 1; dy = 0; }
break;
}
});
gameLoop();
</script>
</body>
</html>
上述代码实现了一个基本的贪吃蛇游戏,包括蛇的移动、食物的生成、碰撞检测和游戏循环。你可以通过方向键来控制蛇的移动。游戏会在蛇撞到墙壁或自身时结束,并提示"游戏结束"。
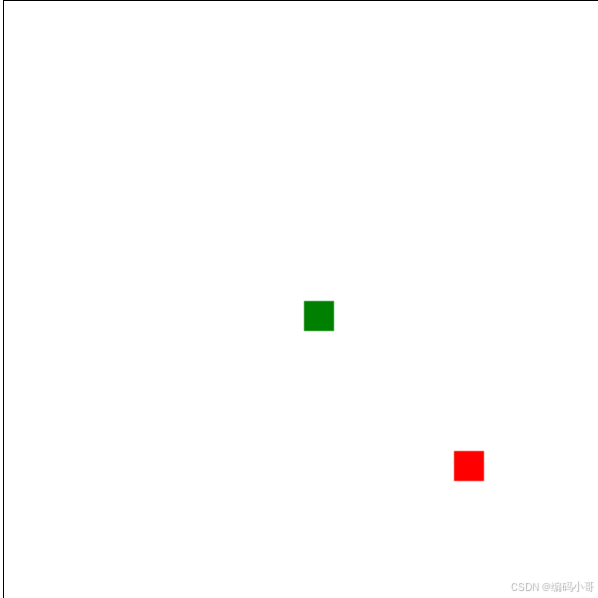