全局异常处理
1、减少try...catch
2、统一处理注解的message
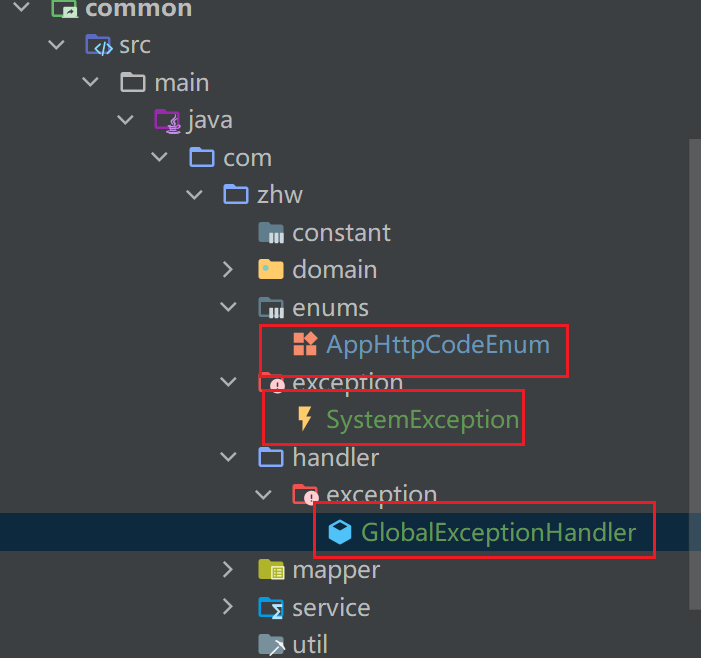
1、响应结果枚举
java
package com.zhw.enums;
@SuppressWarnings("all")
public enum AppHttpCodeEnum {
// 成功
SUCCESS(200,"操作成功"),
// 登录
PARAM_ERROR(400,"接口参数异常"),
NEED_LOGIN(401,"需要登录后操作"),
NO_OPERATOR_AUTH(403,"无权限操作"),
SYSTEM_ERROR(500,"全局捕获的系统错误"),
USERNAME_EXIST(501,"用户名已存在"),
PASSWORD_ERROR(504,"密码错误"),
USER_NAME_ERROR(505,"用户名错误");
int code;
String msg;
AppHttpCodeEnum(int code, String errorMessage){
this.code = code;
this.msg = errorMessage;
}
public int getCode() {
return code;
}
public String getMsg() {
return msg;
}
}
2、新建异常类
java
package com.zhw.exception;
import com.zhw.enums.AppHttpCodeEnum;
@SuppressWarnings("all")
public class SystemException extends RuntimeException {
private int code;
private String msg;
public int getCode() {
return code;
}
public String getMsg() {
return msg;
}
public SystemException(AppHttpCodeEnum httpCodeEnum) {
super(httpCodeEnum.getMsg());
this.code = httpCodeEnum.getCode();
this.msg = httpCodeEnum.getMsg();
}
}
3、新建全局异常处理handler
java
package com.zhw.handler.exception;
import com.zhw.domain.ResponseResult;
import com.zhw.enums.AppHttpCodeEnum;
import com.zhw.exception.SystemException;
import lombok.extern.slf4j.Slf4j;
import org.springframework.validation.ObjectError;
import org.springframework.web.bind.MethodArgumentNotValidException;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.RestControllerAdvice;
@RestControllerAdvice // 1、全局异常处理,代替局部try catch 2、统一对message信息返回
@Slf4j
@SuppressWarnings("all")
public class GlobalExceptionHandler {
// RequestBody 参数校验不通过处理处理
@ExceptionHandler(MethodArgumentNotValidException.class)
public ResponseResult handleValidException(MethodArgumentNotValidException e) {
// 打印异常信息
log.error("接口参数! {}", e);
ObjectError objectError = e.getBindingResult().getAllErrors().get(0);
// 从异常对象中获取提示信息封装返回
return ResponseResult.errorResult(AppHttpCodeEnum.PARAM_ERROR.getCode(), objectError.getDefaultMessage());
}
@ExceptionHandler(SystemException.class) // 指定需要处理的异常类型
public ResponseResult systemExceptionHandler(SystemException e) {
// 打印异常信息
log.error("出现了异常! {}", e);
// 从异常对象中获取提示信息封装返回
return ResponseResult.errorResult(e.getCode(), e.getMsg());
}
@ExceptionHandler(Exception.class)
public ResponseResult exceptionHandler(Exception e) {
// 打印异常信息
log.error("出现了异常! {}", e);
// 从异常对象中获取提示信息封装返回
return ResponseResult.errorResult(AppHttpCodeEnum.SYSTEM_ERROR.getCode(), e.getMessage());
}
}