myString.h
#ifndef MYSTERAM_H
#define MYSTERAM_H
#include <iostream>
#include<cstring>
using namespace std;
class myString
{
private:
char *str; //字符串
int size; //字符串容量
char error[20] = "error";
public:
//无参构造
myString():size(10)
{
size = 10;
str = new char[size]();
}
//有参构造
myString(const char *s)
{
size = strlen(s); //计算传入的字符串长度
str = new char[size];//创建空间为strlen(s)的空间
strcpy(str,s); //拷贝形参给实参
}
//析构函数
~myString()
{
delete []str;
}
//为字符串赋值
myString operator=(char *s);
//访问指定字符,有边界检查
char &at(int index);
//访问指定字符
char &operator[](int index)const;
//返回指向字符串首字符的指针
char *data()const;
//返回字符串不可修改的C字符数组版本
char c_str()const;
//检查字符串是否为空
bool empty();
//返回字符数
int mysize();
//返回字符数
int length();
//返回当前对象分配的存储空间能保存的字符数量
int capacity();
//清空内容
void clear();
//后附字符到结尾
bool push_back(char s);
//移除末尾字符
bool pop_back();
//后附字符到结尾
bool append(const char s);
//后附字符到结尾
myString &operator+=(const char s);
//连接两个字符串或者一个字符串和一个字符
myString &operator+(const char *s);
//判断两个字符串是否相等
bool operator==(const char *s);
//判断两个字符串!=
bool operator!=(const char *s);
//判断两个字符串<
bool operator<(const char *s);
//判断两个字符串>
bool operator>(char *s);
//判断两个字符串<=
bool operator<=(const char *s);
//判断两个字符串>=
bool operator>=(const char *s);
//展示
void show();
friend ostream & operator<<(ostream &L,const myString &s);
friend istream & operator>>(istream &in, myString &s);
};
//执行字符串的流输入
ostream & operator<<(ostream &L,const myString &s);
//执行字符串的流输出
istream & operator>>(istream &in, myString &s);
#endif // MYSTERAM_H
myString.cpp
#ifndef MYSTERAM_H
#define MYSTERAM_H
#include <iostream>
#include<cstring>
using namespace std;
class myString
{
private:
char *str; //字符串
int size; //字符串容量
char error[20] = "error";
public:
//无参构造
myString():size(10)
{
size = 10;
str = new char[size]();
}
//有参构造
myString(const char *s)
{
size = strlen(s); //计算传入的字符串长度
str = new char[size];//创建空间为strlen(s)的空间
strcpy(str,s); //拷贝形参给实参
}
//析构函数
~myString()
{
delete []str;
}
//为字符串赋值
myString operator=(char *s);
//访问指定字符,有边界检查
char &at(int index);
//访问指定字符
char &operator[](int index)const;
//返回指向字符串首字符的指针
char *data()const;
//返回字符串不可修改的C字符数组版本
char c_str()const;
//检查字符串是否为空
bool empty();
//返回字符数
int mysize();
//返回字符数
int length();
//返回当前对象分配的存储空间能保存的字符数量
int capacity();
//清空内容
void clear();
//后附字符到结尾
bool push_back(char s);
//移除末尾字符
bool pop_back();
//后附字符到结尾
bool append(const char s);
//后附字符到结尾
myString &operator+=(const char s);
//连接两个字符串或者一个字符串和一个字符
myString &operator+(const char *s);
//判断两个字符串是否相等
bool operator==(const char *s);
//判断两个字符串!=
bool operator!=(const char *s);
//判断两个字符串<
bool operator<(const char *s);
//判断两个字符串>
bool operator>(char *s);
//判断两个字符串<=
bool operator<=(const char *s);
//判断两个字符串>=
bool operator>=(const char *s);
//展示
void show();
friend ostream & operator<<(ostream &L,const myString &s);
friend istream & operator>>(istream &in, myString &s);
};
//执行字符串的流输入
ostream & operator<<(ostream &L,const myString &s);
//执行字符串的流输出
istream & operator>>(istream &in, myString &s);
#endif // MYSTERAM_H
main.cpp
#include <mySteram.h>
int main()
{
myString s;//无参构造
cout<<"字符串长度"<<s.length()<<endl;
cout<<"字符串长度"<<s.mysize()<<endl;
cout<<"开辟空间大小"<<s.capacity()<<endl;
cout<<"*****************************************"<<endl;
myString ss("zhenxinye");//有参构造
ss.show();
cout<<ss.length()<<endl;
cout<<ss.mysize()<<endl;
cout<<"开辟空间大小"<<ss.capacity()<<endl;
cout<<"*****************************************"<<endl;
cout<<"结尾加上字符 a ";
ss.append('a');
ss.show();
cout<<"*****************************************"<<endl;
cout<<"结尾加上字符 a ";
ss.append('a');
ss.show();
cout<<"*****************************************"<<endl;
cout<<"结尾加上字符 b ";
ss+='b';
ss.show();
cout<<"*****************************************"<<endl;
cout<<"删除最后一个字符:";
ss.pop_back();
ss.show();
cout<<"*****************************************"<<endl;
cout<<"拼接字符串 hello world:";
ss = ss+" hello world";
ss.show();
cout<<"*****************************************"<<endl;
cout<<"ss == zhenxinyeaa hello world?"<<endl;
ss == "zhenxinyeaa hello world";
cout<<"*****************************************"<<endl;
cout<<"cout<<ss "<<ss;
cout<<"*****************************************"<<endl;
cout<<"cin>>ss "<<endl;
cin>>ss;
cout<<"--------"<<endl;
ss.show();
return 0;
}
效果展示
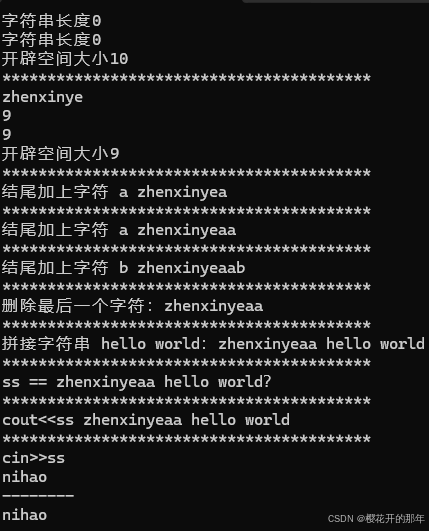