1.测试这样没意义,要向后加
wait等待进程结束
1.
2.测试
发送异常结束的信号,通过kill
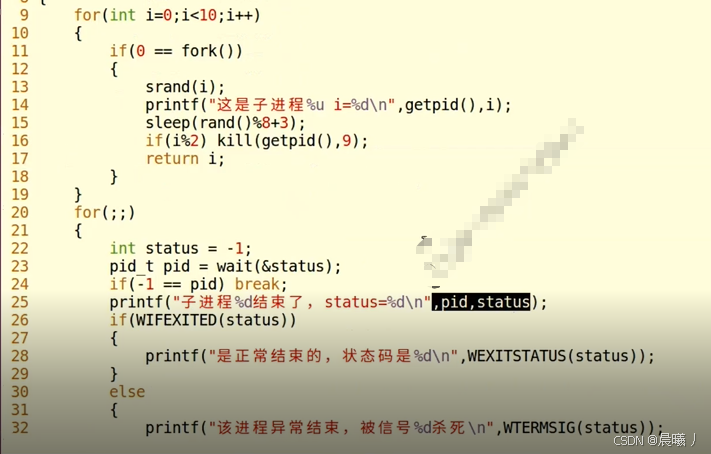
二、子进程的回收
对于子进程的结束而言,都希望父进程能够知道并作出一定的反应,通过 wait、waitpid 函数可以知道子进程是如何结束的以及它的结束状态码
pid_t wait(int *status);
功能:等待子进程结束,并获取结束状态码
status:输出型参数,接收结束状态码
返回值:结束的子进程的ID
1、如果所有子进程都还在运行,则阻塞
2、如果有一个子进程结束,立即返回该进程的结束状态码和ID
3、如果没有子进程返回-1
WIFEXITED(status)
判断进程是否是正常结束,如果是返回真
WEXITSTATUS(status)
如果进程是正常结束的,可以获取到正确的结束状态码
WIFSIGNALED(status)
判断进程是否异常结束,如果是返回真
WTERMSIG(status)
如果进程是异常结束的,可以获取到杀死进程的信号
pid_t waitpid(pid_t pid, int *status, int options);
功能:等待回收指定的某个或某些进程
pid:
>0 等待该进程结束
0 等待同组的任意进程结束
-1 等待任意进程结束,功能与wait等价
<-1 等待abs(pid) 进程组中的任意进程结束
status:输出型参数,接收结束状态码
options:
WNOHANG 非阻塞模式,如果当前没有子进程结束,则立即返回0
WUNTRACED 如果有子进程处于暂停态,返回该进程的状态
WCONTINUED 如果有子进程从暂停态转为继续运行,返回该子进程的状态
WIFSTOPPED(status)
判断子进程是否转为暂停态,是返回真
WSTOPSIG(status)
获取导致子进程进入暂停态的信号
WIFCONTINUED(status)
判断子进程是否由暂停转为继续,是返回真
int system(const char *command);
功能:通过创建子进程去执行一个可执行文件
返回值:子进程结束后才返回
注意:该函数底层调用了fork、vfork、exec、waitpid函数
cpp
#include <stdio.h>
#include<unistd.h>
#include<sys/wait.h>
#include<stdlib.h>
#include<sys/types.h>
#include<signal.h>
//父进程收到信号会进来
void sigchld(int num)
{
//子进程结束的时候父进程会接收到SIGCHLD信号,就会进入注册的处理函数
int status=0;
pid_t pid =wait(&status);
printf("进程%d结束",pid);
//if(pid==-1) exit(0); //无效
if( WIFEXITED(status))
{
printf("是正常结束的,状态码是%d\n", WEXITSTATUS(status));
}
else{
printf("该进程异常结束,被%d信号杀死", WTERMSIG(status));
}
}
int main(int argc,const char* argv[])
{
signal(SIGCHLD,sigchld);
for(int i=0;i<10;i++)
{
if(0==fork())
{
srand(i);
printf("这是子进程%u i=%d\n",getpid(),i);
sleep(rand()%8+3);//0-10
if(i%2) kill(getpid(),9);//发信号当前信号,9 向当前进程发出异异常结束
return i;
}
sleep(1);//防止信号还没来的及处理
}
for(;;)
{
//父进程自己在干事情
printf("*");//在缓冲区中????
fflush(stdout);//刷新缓冲区
sleep(1);
/*
int status=-1;
pid_t pid=wait(&status);
if(-1==pid) break;
printf("子进程%d结束了,status=%d\n",pid,status);
if( WIFEXITED(status))
{
printf("是正常结束的,状态码是%d\n", WEXITSTATUS(status));
}
else{
printf("该进程异常结束,被%d信号杀死", WTERMSIG(status));
}*/
}
printf("没有了子进程\n");
return 0;
}
cpp
#include <stdio.h>
#include<unistd.h>
#include<sys/wait.h>
#include<stdlib.h>
#include<sys/types.h>
#include<signal.h>
/*
//父进程收到信号会进来
void sigchld(int num)
{
//子进程结束的时候父进程会接收到SIGCHLD信号,就会进入注册的处理函数
int status=0;
pid_t pid =wait(&status);
printf("进程%d结束",pid);
//if(pid==-1) exit(0); //无效
if( WIFEXITED(status))
{
printf("是正常结束的,状态码是%d\n", WEXITSTATUS(status));
}
else{
printf("该进程异常结束,被%d信号杀死", WTERMSIG(status));
}
}*/
int main(int argc,const char* argv[])
{
// signal(SIGCHLD,sigchld);
for(int i=0;i<10;i++)
{
if(0==fork())
{
srand(i);
printf("这是子进程%u 父进程%u i=%d\n",getpid(),getppid(),i);
sleep(rand()%8+3);//0-10
if(i%2)
{
exit(i);//发信号当前信号,9 向当前进程发出异异常结束
}
else
{
kill(getpid(),20);
}
// return i;
}
sleep(1);//防止信号还没来的及处理
}
for(;;)
{
//父进程自己在干事情
printf("*");//在缓冲区中????
fflush(stdout);//刷新缓冲区
int status=-1;
pid_t pid=waitpid(0,&status,WNOHANG |WUNTRACED |WCONTINUED );
if(pid>0)
{
if( WIFSTOPPED(status))
{
printf("子进程%d进暂停,导致的信号%d\n",pid, WSTOPSIG(status));
}
else if( WIFCONTINUED(status))
{
printf("子进%d程有暂停转为继续运行", pid);
}
else
{
printf("子进程%d结束,status=%d\n",pid, WEXITSTATUS(status));
}
}
sleep(1);
}return 0;
}