题目:
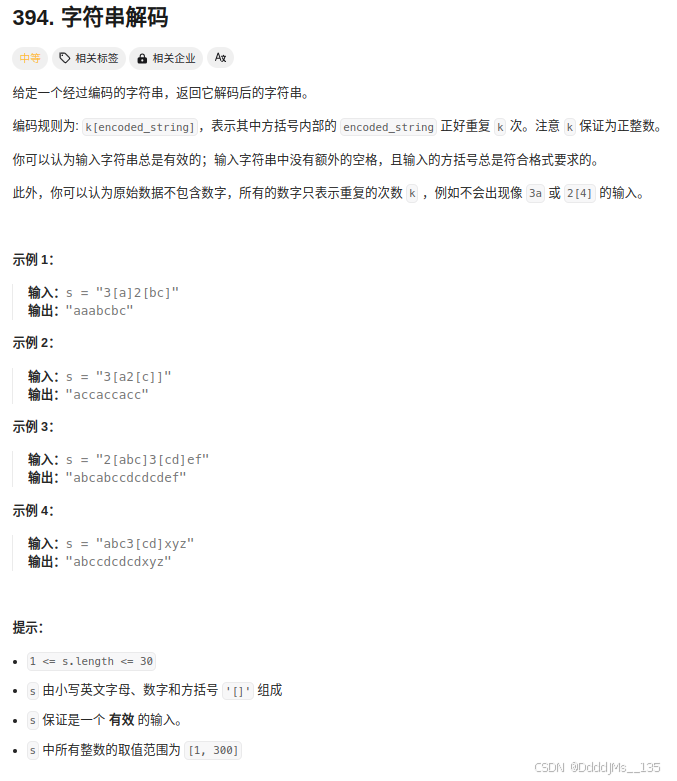
题解:
cpp
#define N 2000
typedef struct {
int data[30];;
int top;
} Stack;
void push(Stack *s, int e) { s->data[(s->top)++] = e; }
int pop(Stack *s) { return s->data[--(s->top)]; }
//多位数字串转换成int
int strToInt(char *s)
{
char val[] = {'\0', '\0', '\0', '\0'};
int result = 0;
for(int i = 0; isdigit(s[i]); ++i)
val[i] = s[i];
for(int i = strlen(val) - 1, temp = 1; i >= 0; --i, temp *= 10)
result += ((val[i] - '0') * temp);
return result;
}
char* decodeString(char *s)
{
Stack magnification; magnification.top = 0;
Stack position; position.top = 0;
char *result = (char*)malloc(sizeof(char) * N);
char *rear = result;
for(int i = 0; s[i] != '\0'; ) {
if(isdigit(s[i])) {
push(&magnification, strToInt(&s[i]));
while(isdigit(s[i]))
++i;
}
else if(s[i] == '[') {
push(&position, rear - result);
++i;
}
else if(s[i] == ']') {
char *p = result + pop(&position);
int count = (rear - p) * (pop(&magnification) - 1);
for(; count > 0; --count)
*(rear++) = *(p++);
++i;
}
else
*(rear++) = s[i++];
}
*rear = '\0';
return result;
}