1. defineExpose 的概念
defineExpose 是 Vue 3 的 Composition API 中一个新的实用函数,用于在 <script setup> 语法下显式暴露组件的公共属性和方法
这在处理子组件时特别有用,允许父组件访问子组件的特定属性或方法
在 Vue 3 中,当我们使用 <script setup> 语法糖时,组件默认不会自动暴露内部的任何状态或方法给外部使用,为了显式暴露某些属性或方法,可以使用 defineExpose
2. defineExpose如何使用
2.1 实现
实现代码:
2.1.1《demo-child》页面
<template>
<view class="out">
子组件
</view>
</template>
<script setup>
import {
ref
} from "vue"
const count = ref(100)
defineExpose({
count,
str:"vue3"
})
</script>
<style lang="scss">
</style>
当父组件通过模板引用的方式获取到当前组件的实例
获取到的实例会像这样 { count,b: "vue3" }
(ref 会和在普通实例中一样被自动解包)
2.1.2《demo5》页面
<template>
<view>
<demo-child ref="child"></demo-child>
</view>
</template>
<script setup>
import {
onMounted,
ref
} from "vue"
const child = ref(null);
onMounted(()=>{
console.log(child.value);
})
</script>
<style lang="scss" scoped>
</style>
这边直接使用onMounted来进行接收,最后输出到控制台来看看结果
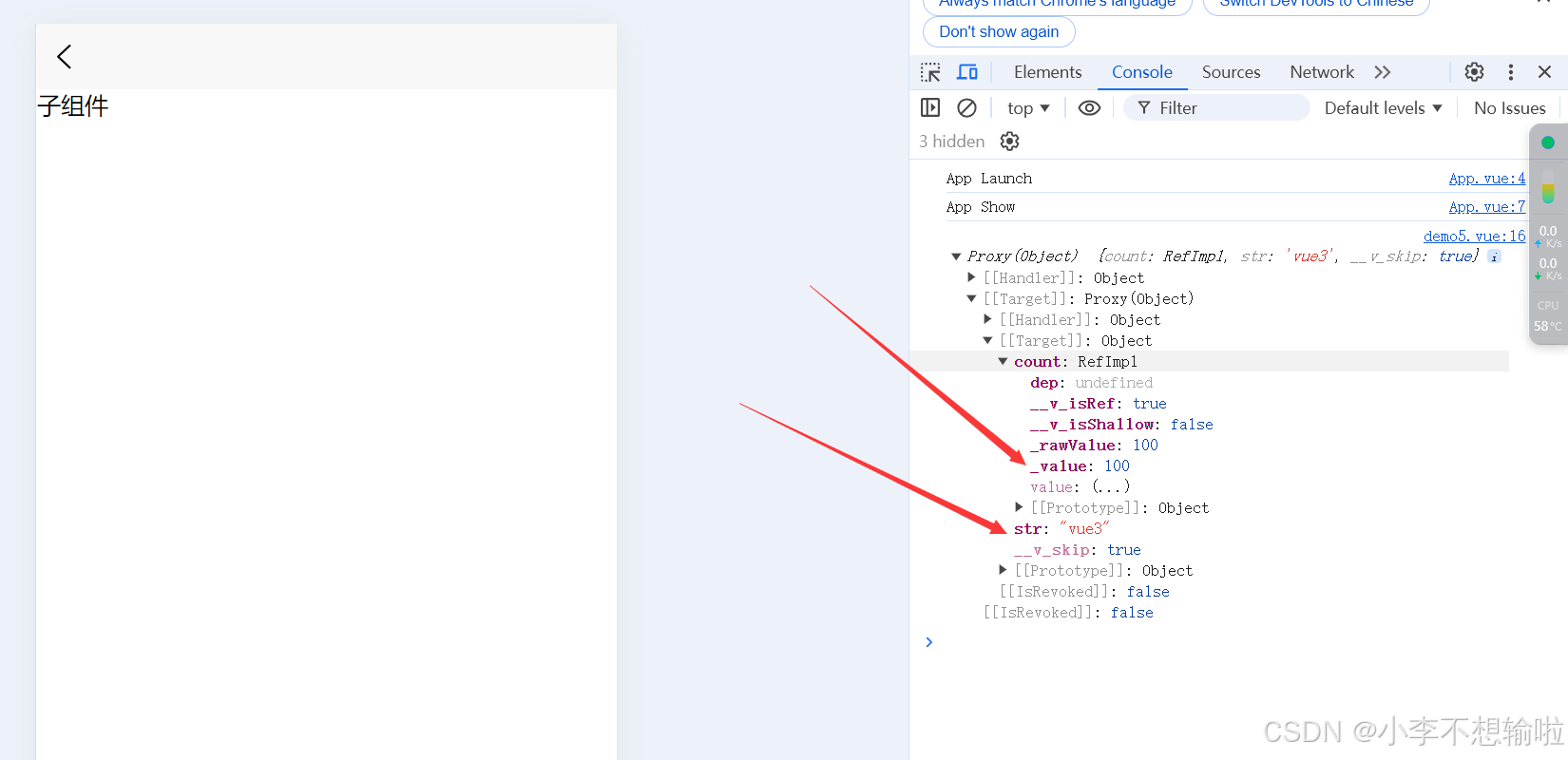
在这个地方就可以找到刚刚传递的count的value值和str的值了
2.2 调用方法实现改变值
2.2.1 《demo-child》页面
<template>
<view class="out">
子组件count值:{{count}}
</view>
</template>
<script setup>
import {
ref
} from "vue"
const count = ref(100);
const updateCount=function(){
count.value++;
}
defineExpose({
count,
str:"vue3",
updateCount
})
</script>
<style lang="scss">
</style>
在这里只需要添加一个方法updateCount,并且将它传递过去,在页面中输出一下就可以了
2.2.2《demo5》页面
<template>
<view class="out">
<demo-child ref="child"></demo-child>
<view>----</view>
<button @click="update">点击修改子值</button>
</view>
</template>
<script setup>
import {
onMounted,
ref
} from "vue"
const child = ref(null);
const update=function(){
child.value.updateCount()
}
onMounted(()=>{
console.log(child.value);
})
</script>
<style lang="scss" scoped>
.out{
padding: 20px;
background-color: #eee;
}
</style>
在这里加上一个按钮,每次点击的时候调用child的方法updateCount方法
这样就可以实现每次点击时数字加一的效果
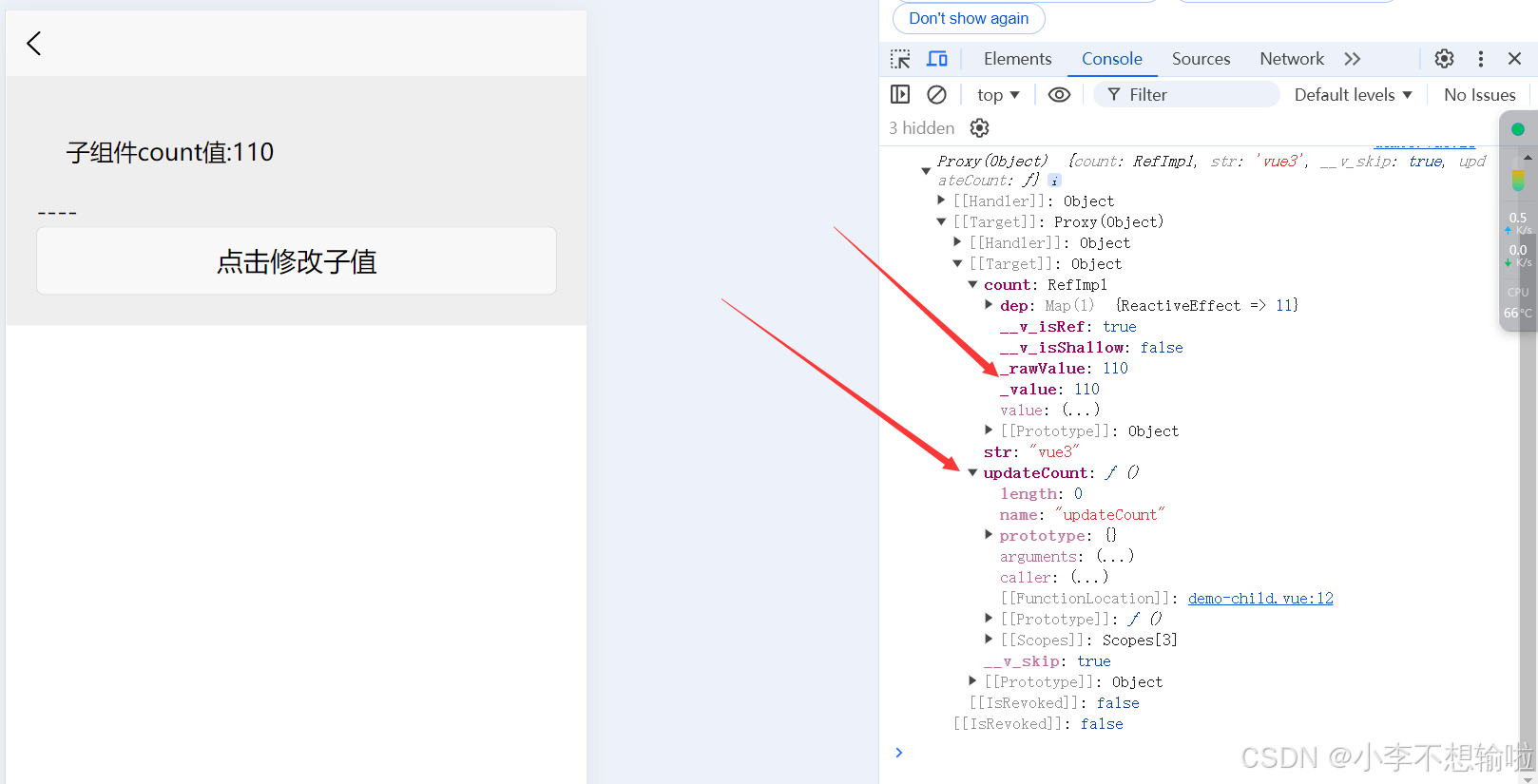
因为在控制台中输出了对象, 所以现在可以看一下结果
count的value值变成了110
而updateCount这个方法也显示在上面
3.总结
使用 <script setup>
的组件是默认关闭的------即通过模板引用或者 $parent
链获取到的组件的公开实例,不会暴露任何在 <script setup>
中声明的绑定。