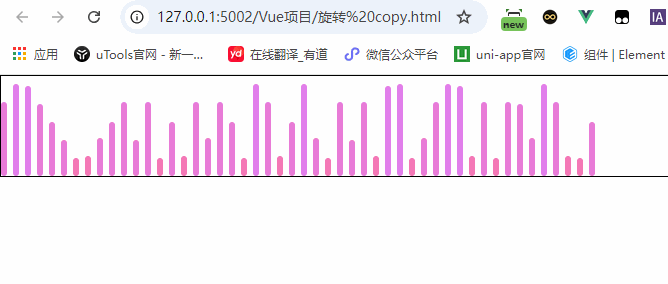
html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<!-- 移动端适配 -->
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!-- 引入VUE CDN -->
<script src="https://cdn.jsdelivr.net/npm/vue@2"></script>
<!-- 引入el样式 -->
<link rel="stylesheet" href="https://unpkg.com/element-ui/lib/theme-chalk/index.css">
<!-- 引入el组件库 -->
<script src="https://unpkg.com/element-ui/lib/index.js"></script>
<title>音波效果</title>
</head>
<style>
/*实现原理就是通过动画控制高度,距离顶部高度,背景色变换*/
@keyframes soundWave {
0%, 100% {
height: 10%;
margin-top: 25%;
background: #f677b0;
}
50% {
height: 100%;
margin-top: 0%;
background: #df7ff2;
}
}
.music {
width: 175px;
height: 100px;
display: flex;
align-items: flex-end;
}
.music span {
width: 6px;
border-radius: 18px;
margin-right: 6px;
background: #f677b0;
animation: soundWave 2s infinite linear;
}
.music span:nth-child(2) {
animation-delay: 0.2s;
background: #df7ff2;
}
.music span:nth-child(3) {
animation-delay: 0.4s;
background: #8c7ff2;
}
.music span:nth-child(4) {
animation-delay: 0.6s;
background: #7fd0f2;
}
.music span:nth-child(5) {
animation-delay: 0.8s;
background: #7ff2d3;
}
.music span:nth-child(6) {
animation-delay: 1.0s;
background: #7ff2a0;
}
.music span:nth-child(7) {
animation-delay: 1.2s;
background: #adf27f;
}
.music span:nth-child(8) {
animation-delay: 1.4s;
background: #e7f27f;
}
.music span:nth-child(9) {
animation-delay: 1.6s;
background: #ecaa64;
}
</style>
<body>
<div id="app">
<div class="music">
<span></span>
<span></span>
<span></span>
<span></span>
<span></span>
<span></span>
<span></span>
<span></span>
<span></span>
</div>
</div>
<script>
/* 实例化vue */
var app = new Vue({
el: '#app',
data: {
},
mounted() {
},
methods: {
}
})
</script>
</body>
</html>