文章目录
QRadioButton
QRadioButton
是单选按钮,可以让我们在多个选项当中选择一个
作为
QAbstractButton
和QWidget
的子类,它们的属性和语法,对于QRadioButton
同样适用
QAbstractButton
中和QRadioButton
关联较大的属性
属性 | 说明 |
---|---|
checkable | 是否能被使用 |
checked | 是否已经被选择(checkable是check的前提条件) |
autoExclusive | 是否排他 选中一个按钮之后,是否会取消其他按钮的选中 对于QRadioButton 来说,默认排他的(单选) |
cpp
#include "widget.h"
#include "ui_widget.h"
Widget::Widget(QWidget *parent)
: QWidget(parent)
, ui(new Ui::Widget)
{
ui->setupUi(this);
//添加默认选项
ui->radioButton_other->setChecked(true);
ui->label->setText("您的性别为: ?");
}
Widget::~Widget()
{
delete ui;
}
void Widget::on_radioButton_male_clicked()
{
ui->label->setText("您的性别为: 男");
}
void Widget::on_radioButton_female_clicked()
{
ui->label->setText("您的性别为: 女");
}
void Widget::on_radioButton_other_clicked()
{
ui->label->setText("您的性别为: 其他");
}
这里可以看到QRadioButton
默认是排他的,单选
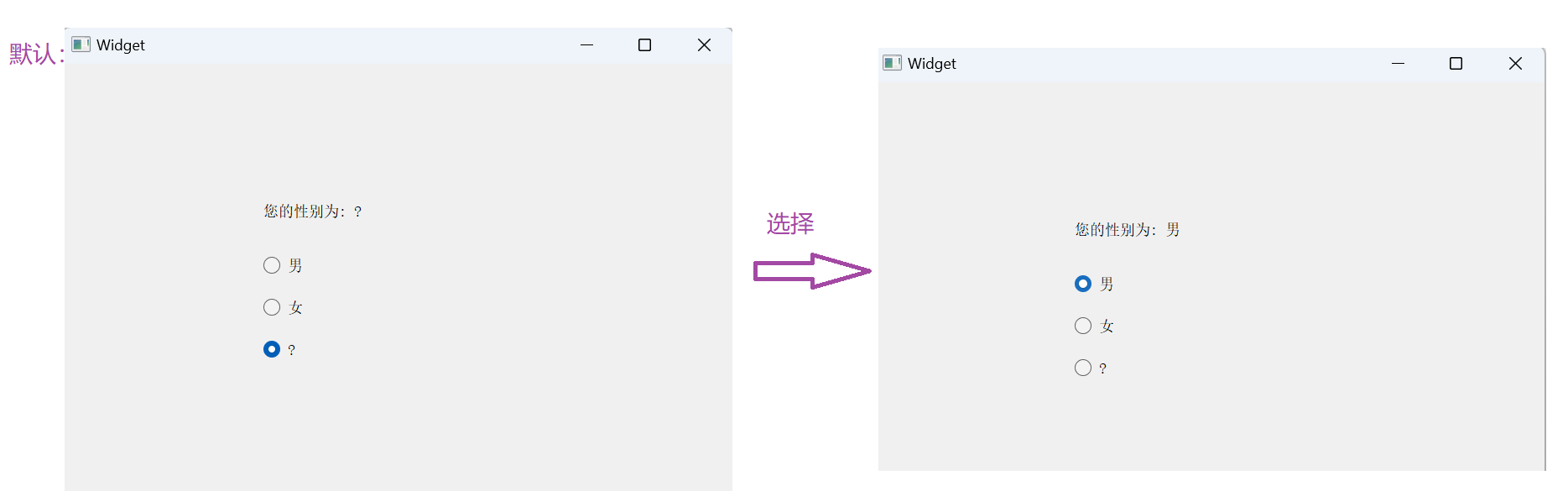
禁用选项:
想要禁用某个按钮,可以用setCheckable(false)
cpp
#include "widget.h"
#include "ui_widget.h"
Widget::Widget(QWidget *parent)
: QWidget(parent)
, ui(new Ui::Widget)
{
ui->setupUi(this);
//添加默认选项
ui->radioButton_other->setChecked(true);
ui->label->setText("您的性别为: ?");
//禁用
ui->radioButton_disable->setCheckable(false);
}
Widget::~Widget()
{
delete ui;
}
void Widget::on_radioButton_male_clicked()
{
ui->label->setText("您的性别为: 男");
}
void Widget::on_radioButton_female_clicked()
{
ui->label->setText("您的性别为: 女");
}
void Widget::on_radioButton_other_clicked()
{
ui->label->setText("您的性别为: 其他");
}
void Widget::on_radioButton_disable_clicked()
{
ui->label->setText("error");
}
这里虽然不可选中,但是还是触发了点击事件。
这是因为checkable
只能够让按钮不被选中,但还是可以响应点击事件
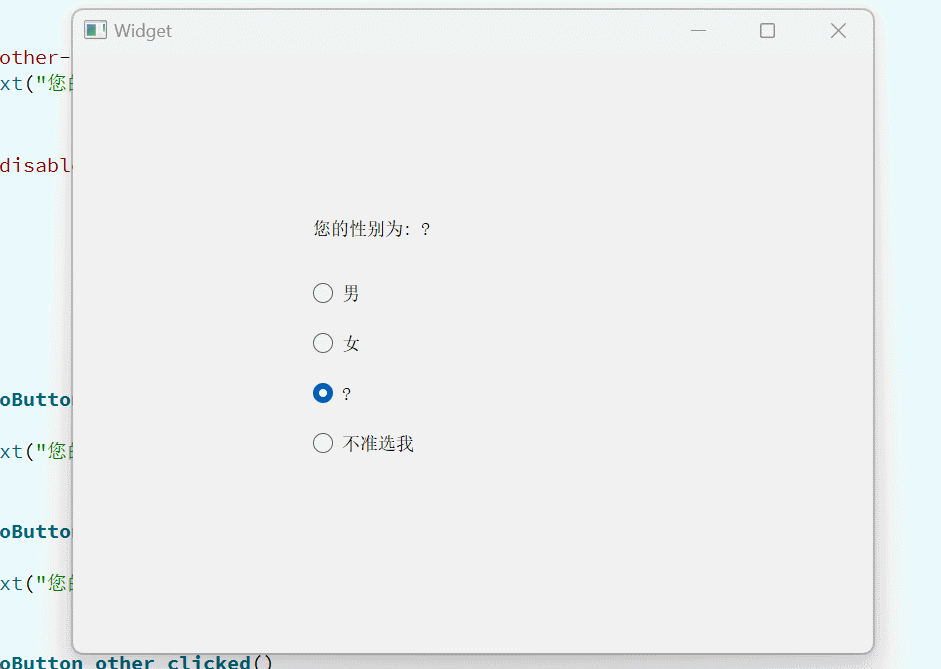
可以采用setEnabled
或者setDisabled
:
cpp
ui->radioButton_disable->setDisabled(true);
//ui->radioButton_disable->setEnabled(false);
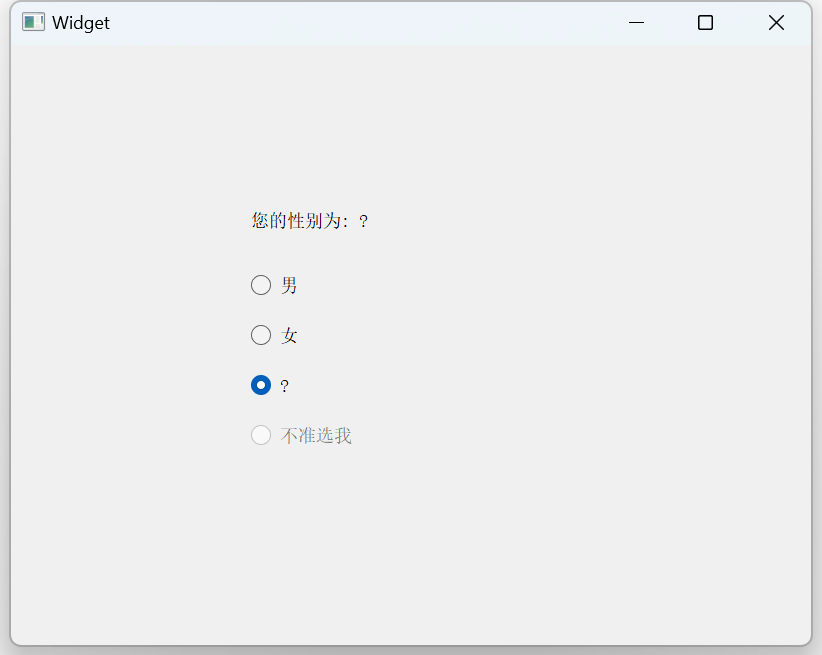
QAbstractButton信号
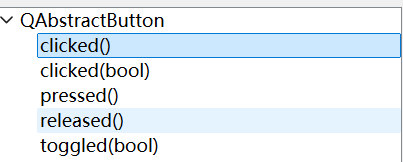
clicked
:之前大多数用的都是cicked()
信号,表示一次点击事件(等于一次pressed + released
)clicked(bool)
:参数的bool
,表示是否被选中pressed()
:鼠标按下released()
:鼠标释放toggled(bool)
:切换的时候会触发
cpp
void Widget::on_radioButton_clicked(bool checked)
{
//checked表示当前radioButton的选中状态
qDebug() << "clicked: " << checked;
}
void Widget::on_radioButton_4_pressed()
{
qDebug() << "pressd";
}
void Widget::on_radioButton_2_released()
{
qDebug() << "released";
}
void Widget::on_radioButton_3_toggled(bool checked)
{
//checked状态发生改变,就会触发这个信号
qDebug() << "toggled: " << checked;
}
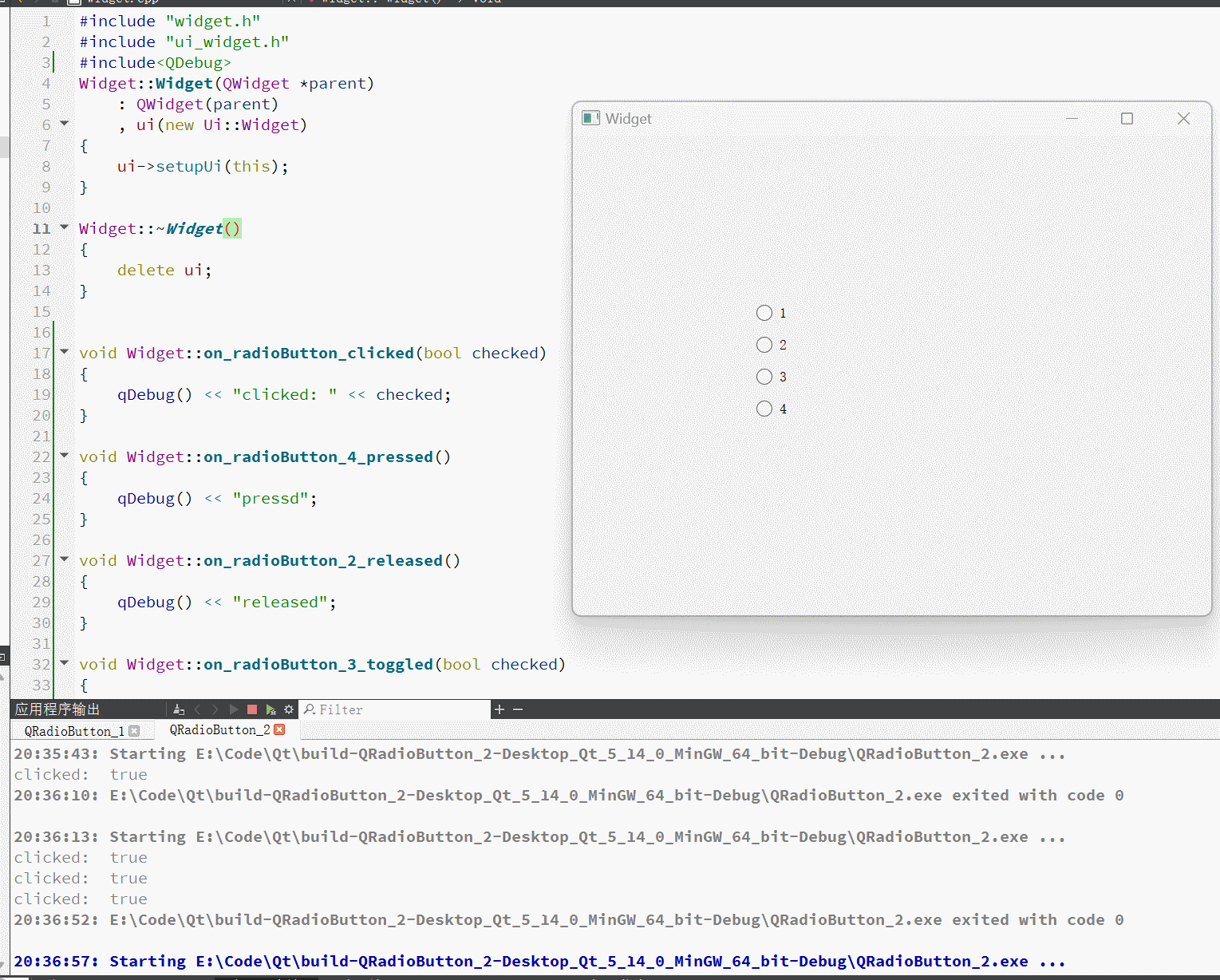
实现简单的点餐页面
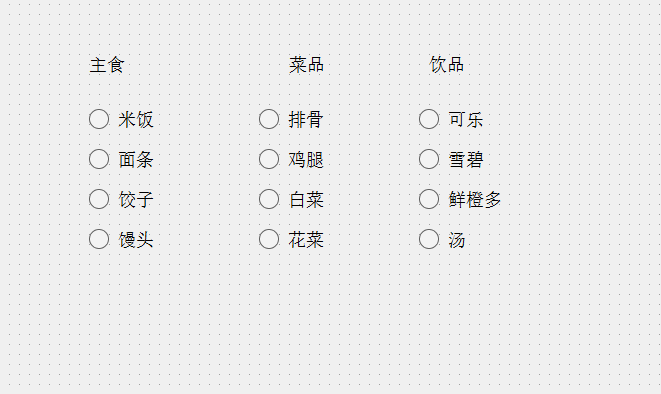
这里分为三组,需要选择三个,但是radioButton
默认是排他的,所有我们可以分组,采用QButtonGroup
针对单选按钮进行分组
cpp
#include "widget.h"
#include "ui_widget.h"
#include<QButtonGroup>
Widget::Widget(QWidget *parent)
: QWidget(parent)
, ui(new Ui::Widget)
{
ui->setupUi(this);
//进行分组
QButtonGroup *group1 = new QButtonGroup(this);
QButtonGroup *group2 = new QButtonGroup(this);
QButtonGroup *group3 = new QButtonGroup(this);
//将按钮放入不同组里
group1->addButton(ui->radioButton);
group1->addButton(ui->radioButton_2);
group1->addButton(ui->radioButton_3);
group1->addButton(ui->radioButton_4);
group2->addButton(ui->radioButton_5);
group2->addButton(ui->radioButton_6);
group2->addButton(ui->radioButton_7);
group2->addButton(ui->radioButton_8);
group3->addButton(ui->radioButton_9);
group3->addButton(ui->radioButton_10);
group3->addButton(ui->radioButton_11);
group3->addButton(ui->radioButton_12);
}
Widget::~Widget()
{
delete ui;
}
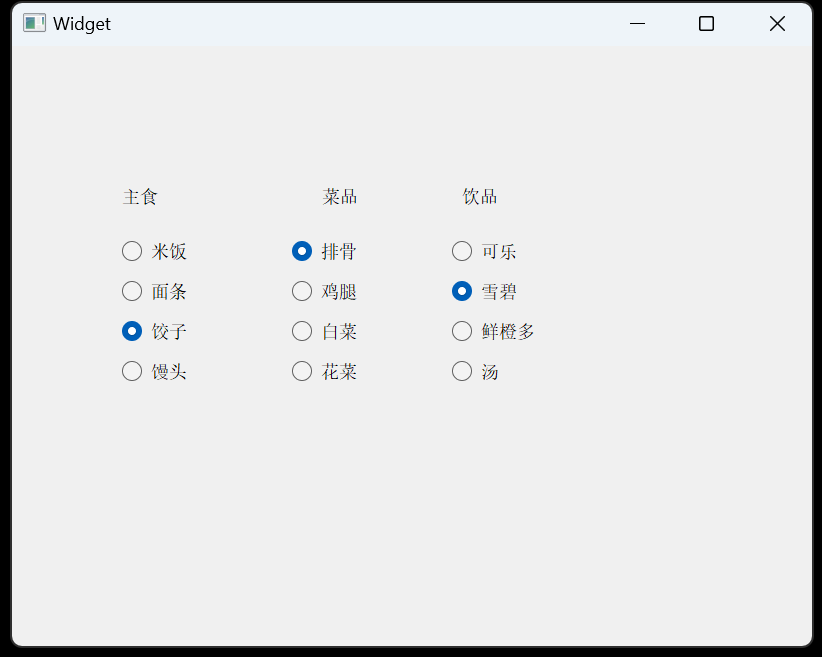
QCheckBox
QRadioButton
只能选一个,而QCheckBox
可以选中多个。
和QCheckBox
最相关的属性也是checkable
和checked
,都是继承自QAbstractButton
。
QCheckBox
独有的属性tristate
,用来实现"三态复选框"
示例:
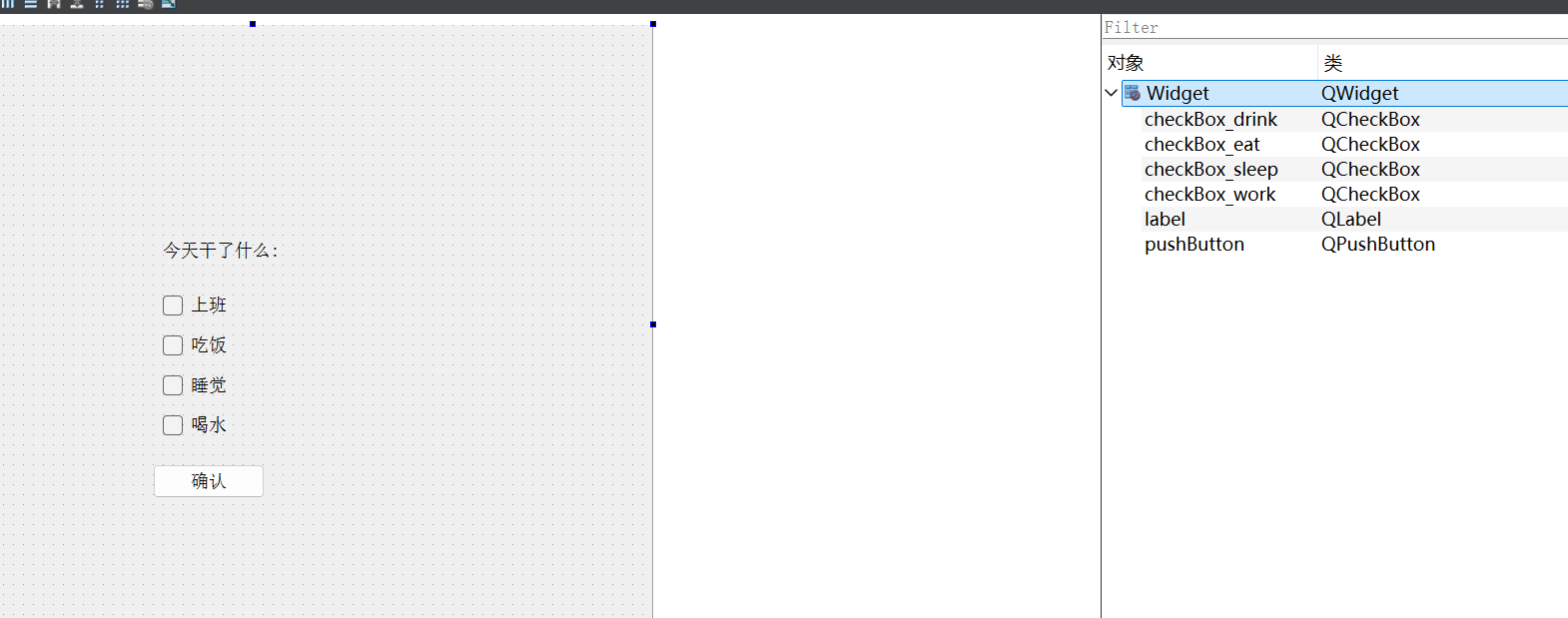
cpp
#include "widget.h"
#include "ui_widget.h"
Widget::Widget(QWidget *parent)
: QWidget(parent)
, ui(new Ui::Widget)
{
ui->setupUi(this);
}
Widget::~Widget()
{
delete ui;
}
void Widget::on_pushButton_clicked()
{
QString ret = "今日:";
if(ui->checkBox_work->isChecked())
{
ret += ui->checkBox_work->text() + " ";
}
if(ui->checkBox_eat->isChecked())
{
ret += ui->checkBox_eat->text() + " ";
}
if(ui->checkBox_sleep->isChecked())
{
ret += ui->checkBox_sleep->text() + " ";
}
if(ui->checkBox_drink->isChecked())
{
ret += ui->checkBox_drink->text() + " ";
}
ui->label->setText(ret);
}
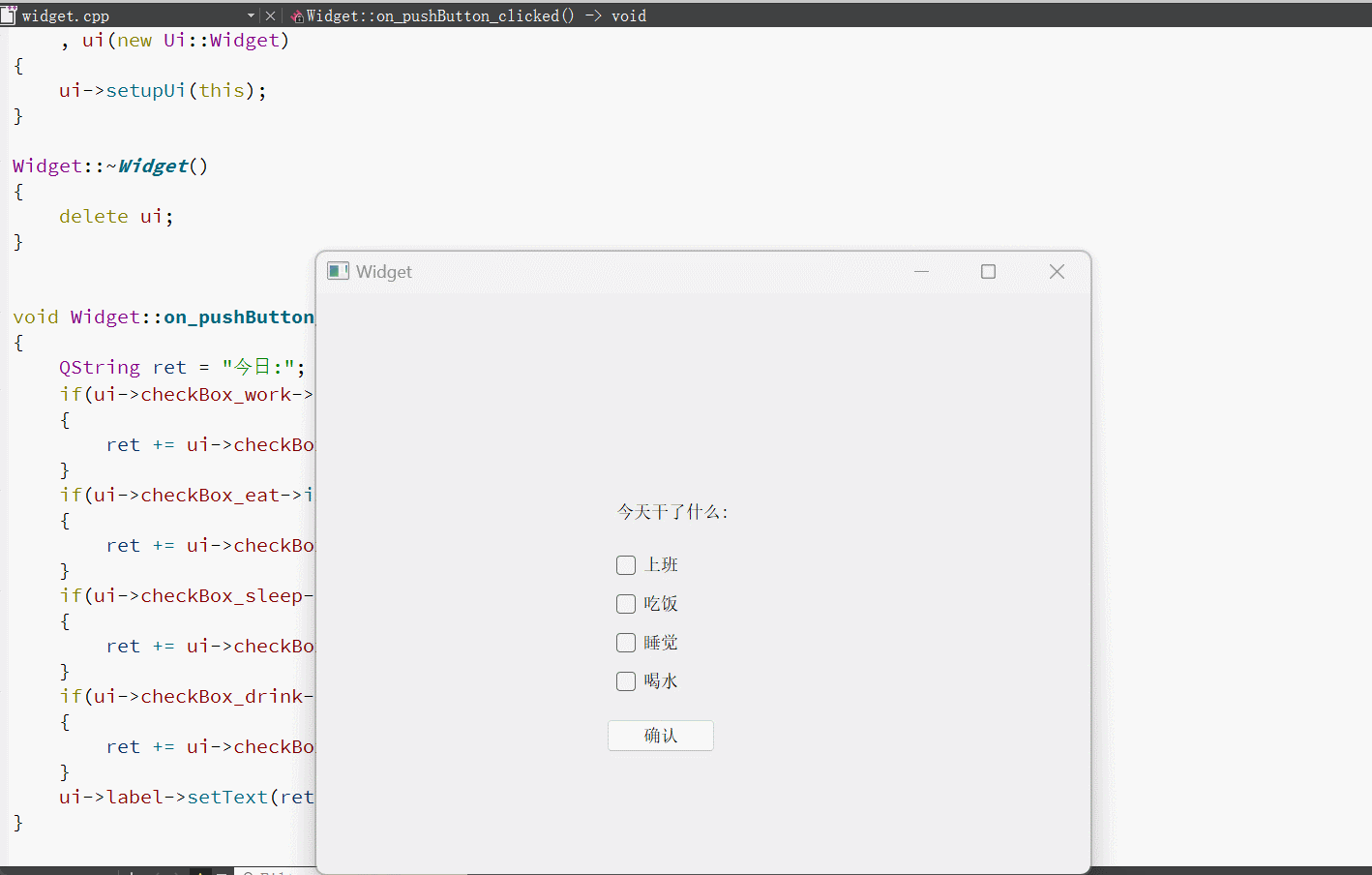