子查询
什么叫子查询?如何实现子查询?
定义:什么叫子查询,也就是先执行子查询,后执行父查询
❓✅面试题:如何实现替换,执行顺序?
1、使用子查询,因为子查询会先执行子句,在执行父句,效率非常低
sql
select * from student where sid = (select max(sid) from student)
-
查询id最大的一个学生(子查询)
select max(sid) from student ,select * from student where sid = 9
如何将这两行合并为一行sql进行执行呢?sqlselect * from student where sid = (select max(sid) from student)
-
查询每个班级下面id最大的学生?
sqlselect * from student left join class on student.classid = class.classid where sid in( select max(sid) from student group by classid )
-
查询张三老师教了哪些学生?
sqlselect * from student where sid in( select sid from sc where cid = ( select cid from course where tid =( (select tid from teacher where tname = '张三') ) ) )
-
✅面试题目:请查询没有学习过张三老师的课的学生?
- 思想
- 找出上了张三老师课程的学生❗
- 然后使用非❗
- 使用not in
sqlselect * from student where sid not in( select sid from sc where cid = ( select cid from course where tid =( (select tid from teacher where tname = '张三') ) ) )
from子查询
定义: 查询结果作为一张表进行使用
-
查询大于5 人的班级的名称和人数
- 使用group by 进行解决
sqlselect * from class left join student on class.classid = student.classid GROUP BY class.classid having count(*) > 5
- 使用子查询进行
sql-- 使用子查询 -- 班级和学生进行了连接,进行了查询 select classname , 人数 from class left join -- 查询每个班级有多少个人,然后在将班级表的classid和学生表的classid进行连接 (select classid,count(*) 人数 from student group by classid )t1 on class.classid = t1.classid where 人数 > 5
exist子查询
👀:定义:exist 子查询 子句有结果,父句执行,子句没有结果,父句不执行
sql
select * from teacher
where exists (select * from student where classid = 10)
any some or 子查询
- 查出一班成绩比二班最低成绩高的学生
sql
select DISTINCT student.* from sc left join student on sc.Sid = student.Sid
where student.classid = 1 and score > some (select min(score) from sc left join student on sc.Sid = student.Sid
where student.classid = 2 )
- 查询一般比二班最高的成绩还要高的学生,all满足所有的条件是and
sql
select DISTINCT student.* from sc
left join student on sc.Sid = student.Sid
where student.classid = 1 and score > all (
select max(score) from sc
left join student on sc.Sid = student.Sid
where student.classid = 2 )
流程控制函数
1️⃣if
格式:
- if(value1, value2, value3)
- vaue1:条件成立
- value2:条件成立,显示数据
- value3:条件不成立 ,显示数据
- 结果集的控制如果为1,则显示女,否则显示男
sql
select * from teacher
select tid, tname, if(tsex=1,'女','男')tsex, Tbirthday,Taddress from teacher
2️⃣idnull (expres1, expre2)
格式:
- expre1 :字段
- expre2 :当字段值为null时,,默认值
- 如果有生日,则显示原来的生日,如果没有生日,则显示默认值
sql
select * from student
select sid, IFNULL(birthday,'我是石猴子'), sname from student
👍面试⭐️ case when then end
- 当有0 时候显示男,当有1 的时候显示女
sql
select tid, tname,
case tsex
when 0 then '男'
when 1 then '女'
end tsex, tbirthday from teacher
-
如果出现没有指定的性别,直接是一个null
sqlselect tid, tname, case tsex when 0 then '男' when 1 then '女' else '保密' end tsex, tbirthday from teacher
-
第二种写法
sqlselect tid,tname, case when tsex > 1 then '男' when tsex = 1 then '女' when Tsex < 1 then '位置' END , tbirthday from teacher
-
查询学生的所有成绩进行排名输出
sql
select
case
when score >= 90 then 'A'
when score >= 80 then 'B'
when score > 70 then 'C'
when score > 60 then 'D'
when score < 60 then '不及格'
else '无成绩'
end
成绩 from sc
- -- 统计各个分数段的人数 ,分数段 人数
SQL
select
case
when score >= 90 then '100-90'
when score >= 80 then 'B'
when score > 70 then 'C'
when score > 60 then 'D'
when score < 60 then '不及格'
else '无成绩'
end
分数段 from sc
✅面试题目:给你一个页面让你写另一个页面
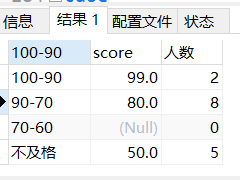
sql
给出的页面
-- 面试题目
select '100-90', score , count(score) 人数 from sc where score > 90 and score <100
union
select '90-70', score , count(score) 人数 from sc where score > 70 and score <90
union
select '70-60', score , count(score) 人数 from sc where score > 60 and score <70
union
select '不及格', score , count(score) 人数 from sc where score <60
如何实现到这个步骤?

sql
select '人数' 分数,
count(case when score <= 100 and score >=90 then score end)'100-90',
count(case when score <= 90 and score >=90 then score end)'90-70',
count(case when score <= 70 and score >=90 then score end)'70-60',
count(case when score <= 60 and score >=90 then score end)'不及格'
from sc