GoogleDrive使用ServiceAccount的授权方式:(科学上网)
1.在Google Cloud中查看自己的项目: Dashboard -- My First Project -- Google Cloud console, 没有的话新建项目。默认名称:My First Project
2. 创建ServiceAccount服务账号:
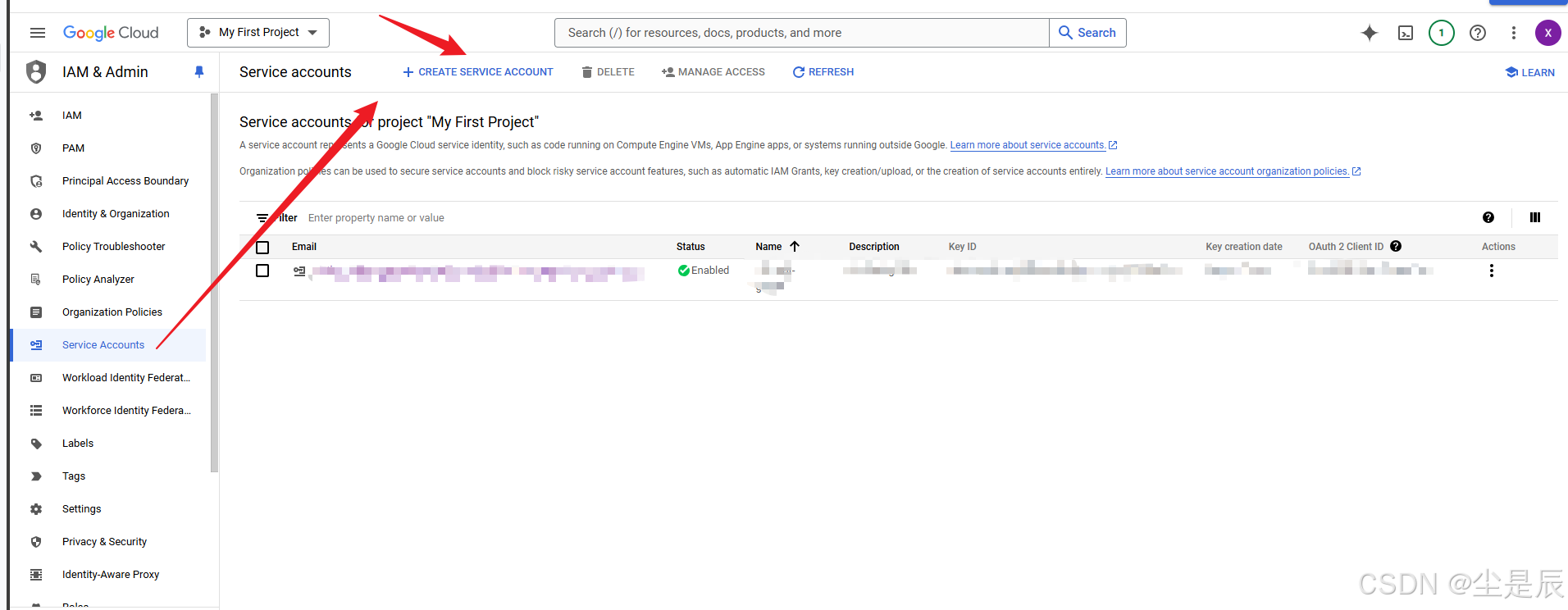
3. 添加私钥,可选用json格式,方便读取,可下载下来
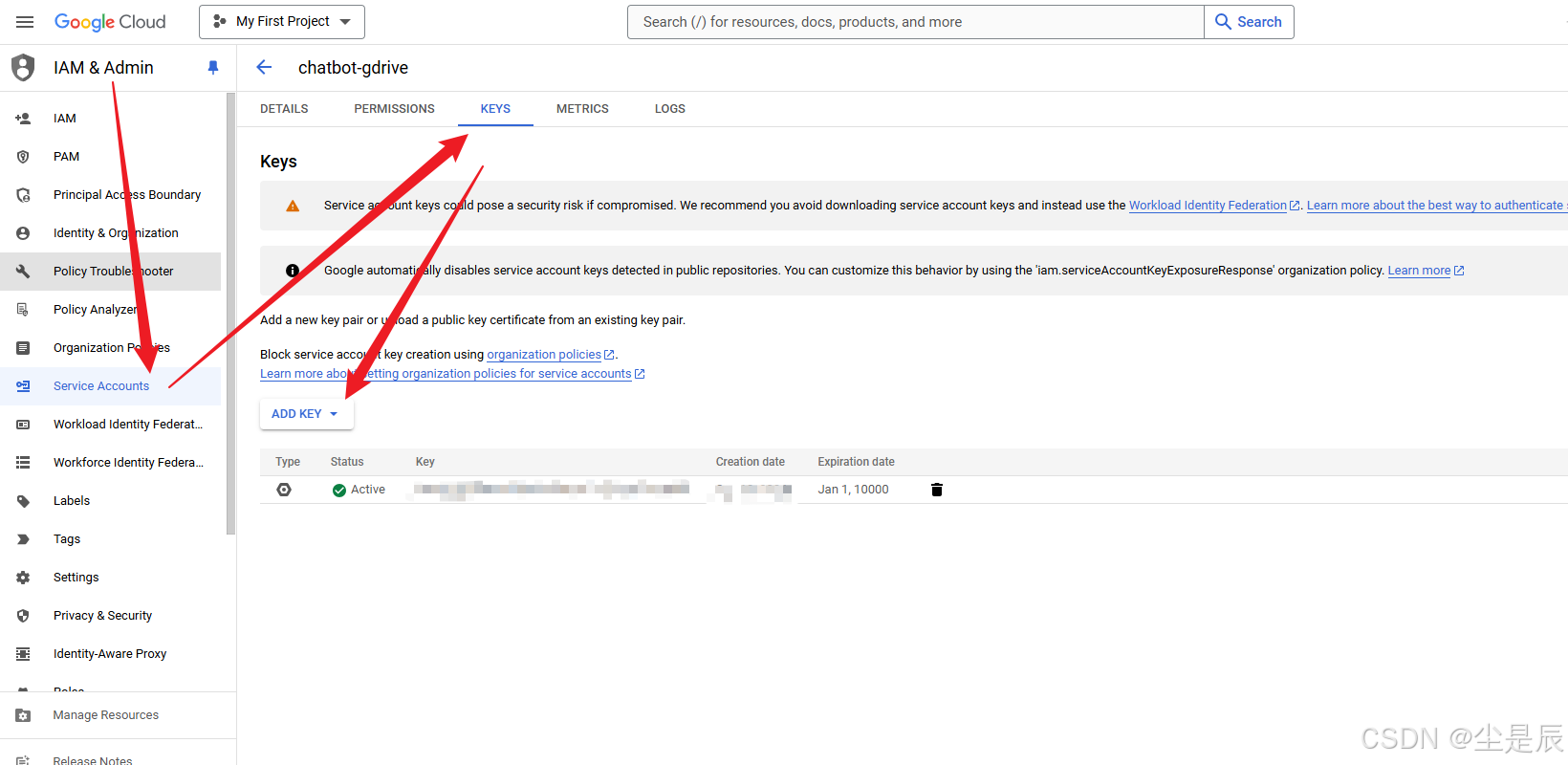
4. 开启Google Drive Api,直接在搜索栏里搜索即可:点击ENABLE后显示API Enabled
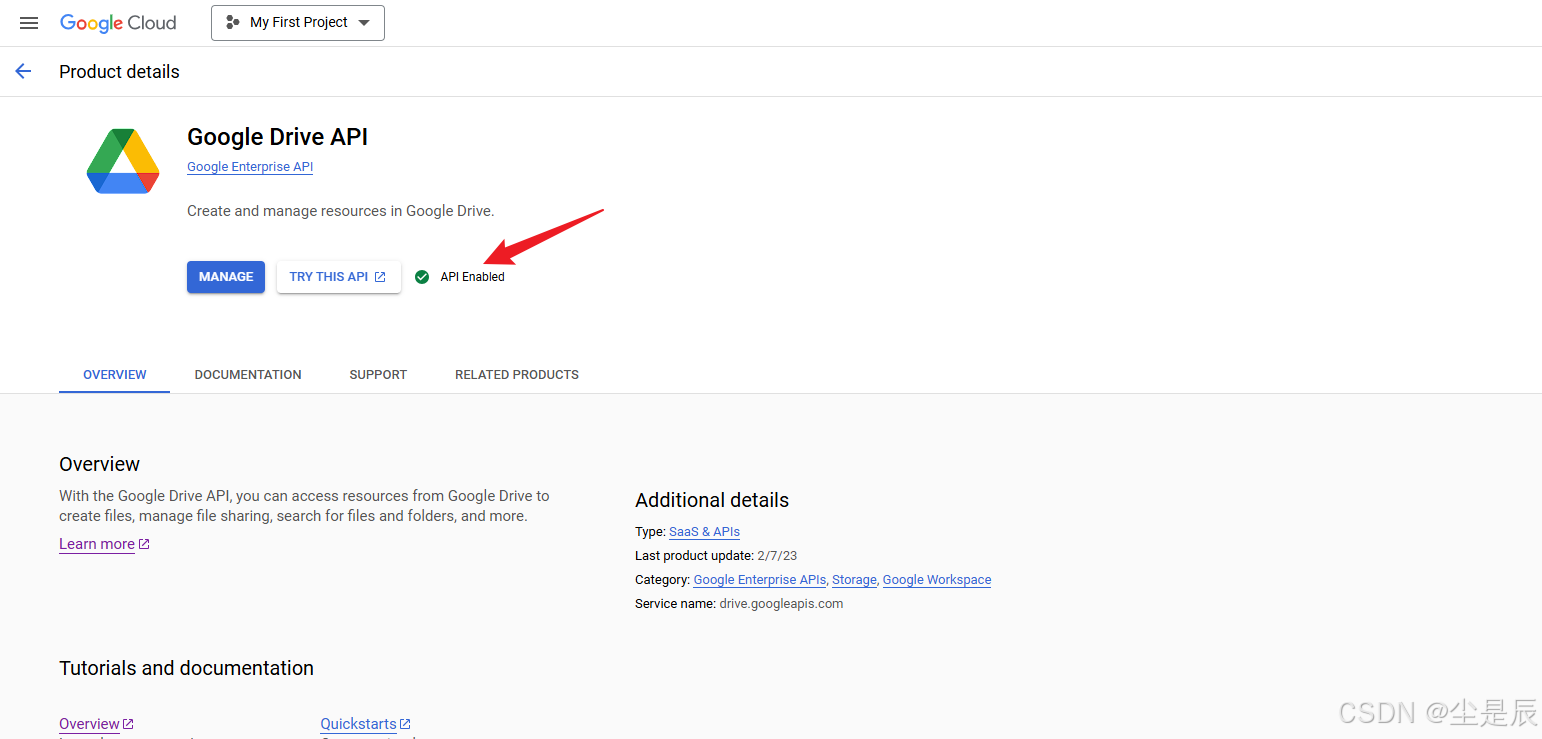
5. 在云端硬盘中操作: Home - Google Drive, 创建目录
1)其中的link是:
https://drive.google.com/drive/folders/17hvNdQUwd10cnD9Ur2JlxrybMuYBCpw0?usp=drive_link,
那么这里的17hvNdQUwd10cnD9Ur2JlxrybMuYBCpw0就是fileId,后面代码中也有体现:
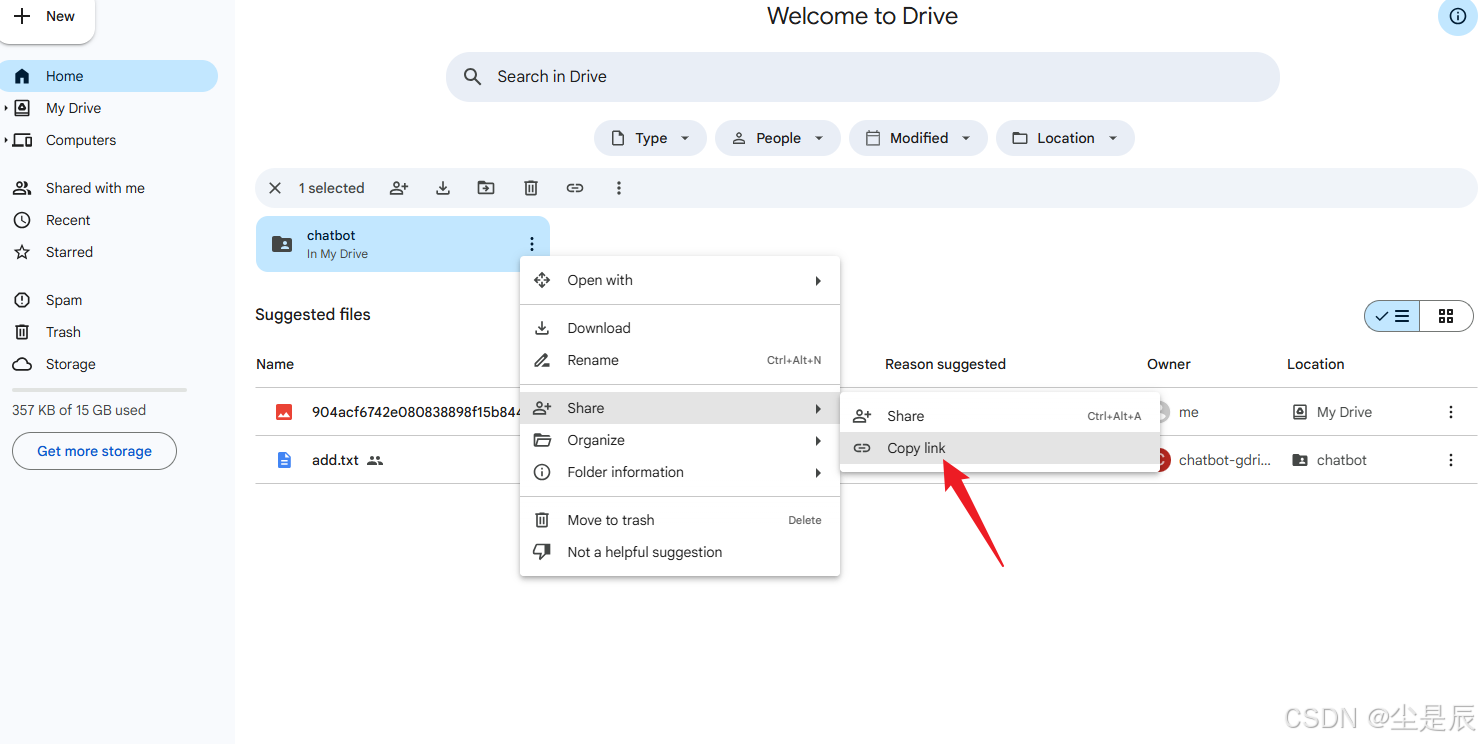
2)而权限也是至关重要:点击这个文件夹的share,或者是点击右侧的Manage access:
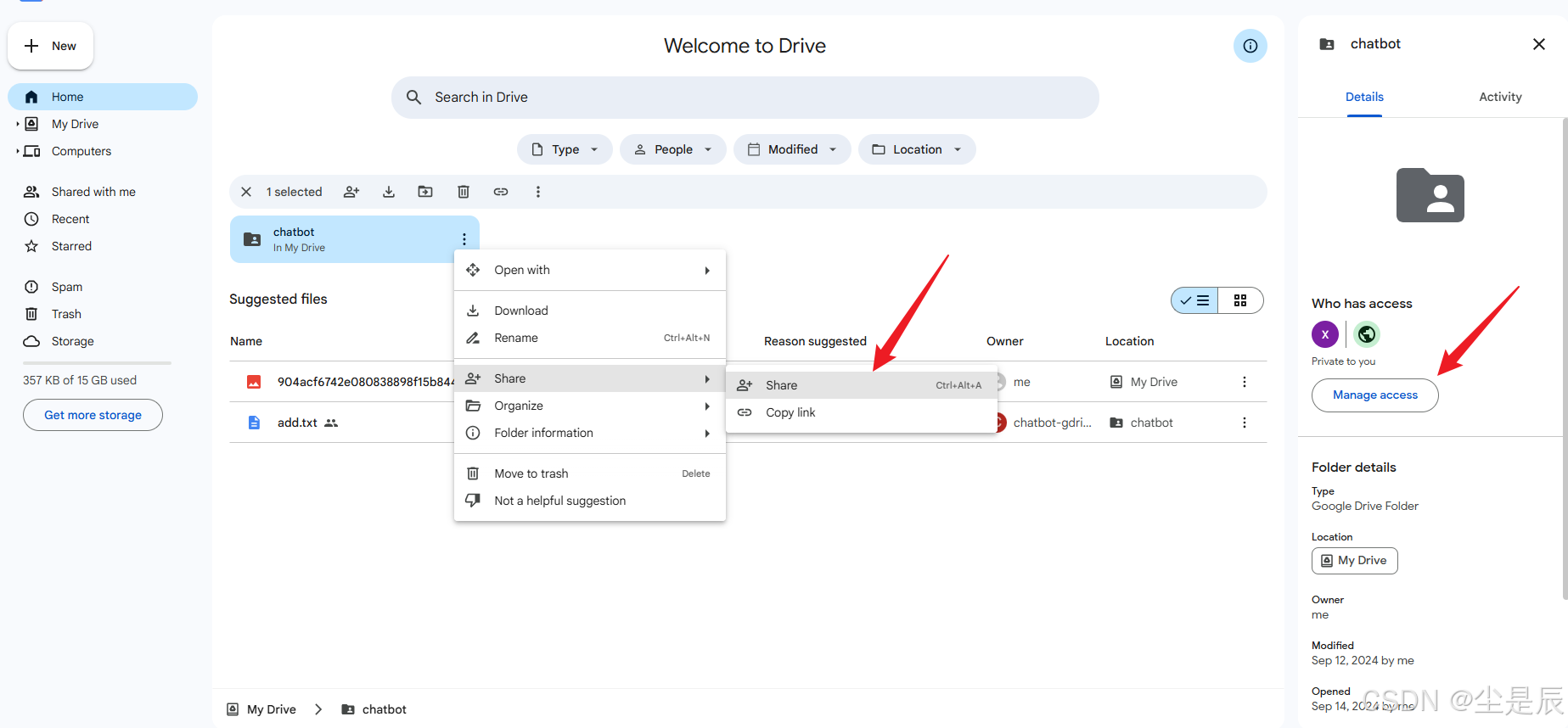
3)其中share中我们要选择editor才可以上传文件:
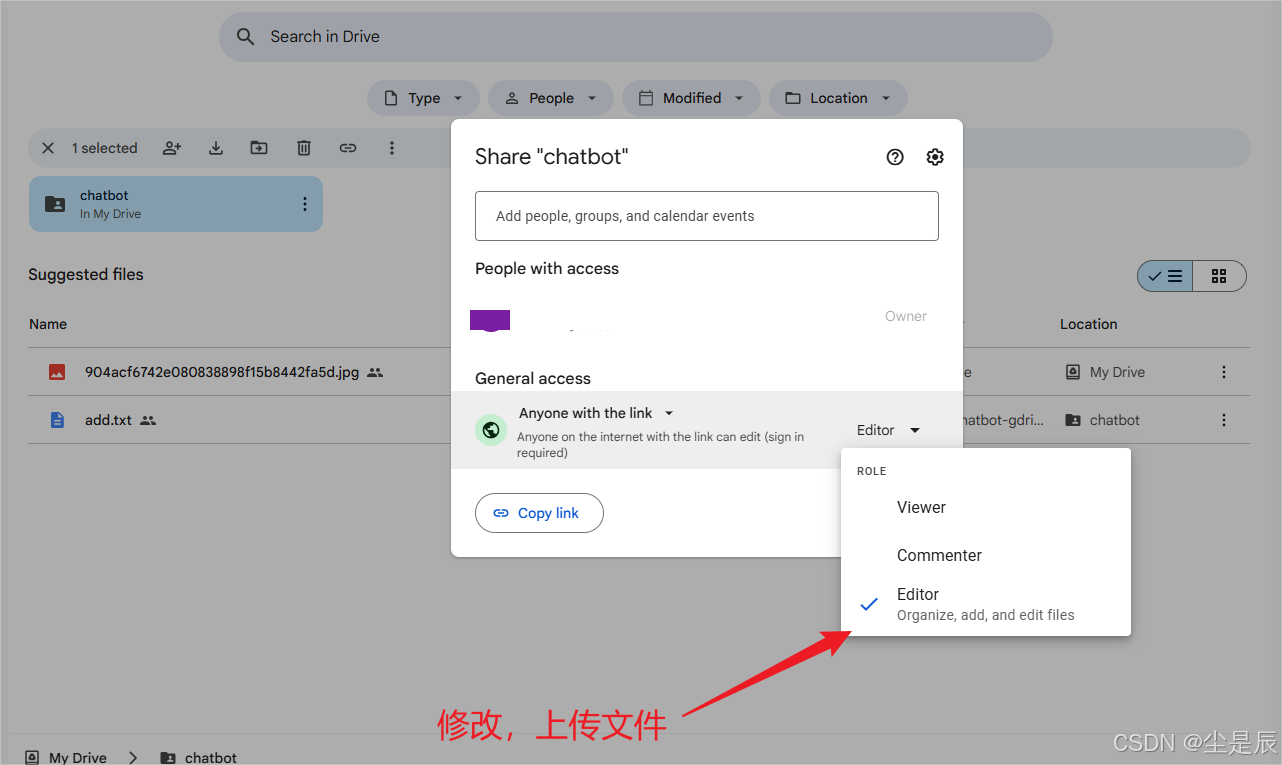
至此,账号及权限问题我们都解决了。现在直接上源码:
1. maven管理依赖:
<!-- GoogleDrive 相关依赖包 -->
<!-- https://mvnrepository.com/artifact/com.google.apis/google-api-services-drive -->
<dependency>
<groupId>com.google.apis</groupId>
<artifactId>google-api-services-drive</artifactId>
<version>v3-rev197-1.25.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.google.api-client/google-api-client -->
<dependency>
<groupId>com.google.api-client</groupId>
<artifactId>google-api-client</artifactId>
<version>1.34.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.google.oauth-client/google-oauth-client-jetty -->
<dependency>
<groupId>com.google.auth</groupId>
<artifactId>google-auth-library-oauth2-http</artifactId>
<version>1.23.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.google.oauth-client/google-oauth-client-jetty -->
<dependency>
<groupId>com.google.oauth-client</groupId>
<artifactId>google-oauth-client-jetty</artifactId>
<version>1.35.0</version>
</dependency>
2. 代码如下:
// 一些 scope 权限
private static final List<String> SCOPES = Arrays.asList(DriveScopes.DRIVE_APPDATA,DriveScopes.DRIVE,DriveScopes.DRIVE_METADATA,DriveScopes.DRIVE_READONLY,DriveScopes.DRIVE_METADATA_READONLY,DriveScopes.DRIVE_SCRIPTS);
/**
* 校验认证权限
* @param configJsonFile
* @return
* @throws IOException
*/
private static HttpRequestInitializer fromServiceAccount(InputStream configJsonFile) throws IOException {
GoogleCredentials credentials = ServiceAccountCredentials.fromStream (configJsonFile).createScoped(SCOPES );
return new HttpCredentialsAdapter(credentials);
}
/**
* 根据后缀名获取文件类型
* @param suffix
* @return
*/
public static String findMineTypeBySuffix(String suffix) {
for (MineTypeOnGoogleDriveEnum type : MineTypeOnGoogleDriveEnum.values ()) {
if (type.getSuffix().equalsIgnoreCase(suffix)) {
return type.getFileType();
}
}
return "Unknown mine type";
}
/**
* GoogleDrive 的后缀名 === 》 mineType ,枚举类
*/
@Getter
@AllArgsConstructor
public enum MineTypeOnGoogleDriveEnum {
TXT ("txt", "text/plain"),
JPG ("jpg", "image/jpeg"),
JPEG ("jpeg", "image/jpeg"),
PNG ("png", "image/png"),
PDF ("pdf", "application/pdf"),
DOC ("doc", "application/msword"),
DOCX ("docx", "application/vnd.openxmlformats-officedocument.wordprocessingml.document"),
XLS ("xls", "application/vnd.ms-excel"),
XLSX ("xlsx", "application/vnd.openxmlformats-officedocument.spreadsheetml.sheet"),
PPT ("ppt", "application/vnd.ms-powerpoint"),
PPTX ("pptx", "application/vnd.openxmlformats-officedocument.presentationml.presentation"),
ZIP ("zip", "application/zip");
private final String suffix;
private final String fileType;
}
Psvm:
public static void main(String[] args) {
try {
// 0. 构建 http 传输
NetHttpTransport httpTransport = GoogleNetHttpTransport.newTrustedTransport ();
// 1. 根据 json 配置文件,构建输入流
InputStream configFileStream = GoogleDriveServiceImpl.class.getResourceAsStream("/google-drive-config.json");
// 2. 创建 Drive 实例
Drive drive = new Drive.Builder(httpTransport, new GsonFactory(), fromServiceAccount (configFileStream))
.setApplicationName("Application-Name")
.build();
// 3. 指定上传文件的路径
java.io.File filePath = new java.io.File("/22.txt");
String suffix = FileUtil.getSuffix (filePath);
// 4. 构建文件元数据:文件名,父目录,文件的 mineType
File fileMetadata = new File();
fileMetadata.setName("add."+ suffix);
fileMetadata.setParents(Collections.singletonList ("17hvNdQUwd10cnD9Ur2JlxrybMuYBCpw0"));
String mineType = findMineTypeBySuffix (suffix);
FileContent mediaContent = new FileContent(mineType, filePath);
// 5. 上传文件
File uploadedFile = drive.files().create(fileMetadata, mediaContent)
.setFields("id,name")
.execute();
System.out .println("FileId: " + uploadedFile.getId());
System.out .println("FileName: " + uploadedFile.getName());
} catch (IOException | GeneralSecurityException e) {
throw new RuntimeException(e);
}
}
最后,FileId就是文件id,那么文件的链接就是https://drive.google.com/file/d/s%/view中的s%替换即可。
3. 测试:
尤为重要的是看是否可以ping通:googleapis.com。如果可以那么就能上传成功。若不可以也能上传成功,可以私信我,欢迎交流一下。