目录
- [1- 思路](#1- 思路)
- [2- 实现](#2- 实现)
-
- [⭐++84. 柱状图中最大的矩形++------题解思路](#⭐84. 柱状图中最大的矩形——题解思路)
- [3- ACM 实现](#3- ACM 实现)
- 原题链接:84. 柱状图中最大的矩形
1- 思路
题目识别
- 识别1 :给定一个数组
heights
,求解柱状图的最大面积
单调栈
- 使用
Stack
来实现,遍历数组,存入下标。 - 本题实现的是一个单调递减栈,目标是找一个以当前为基准,左边第一个比这个小的以及右边第一个比该元素小的元素。
2- 实现
⭐++84. 柱状图中最大的矩形++------题解思路
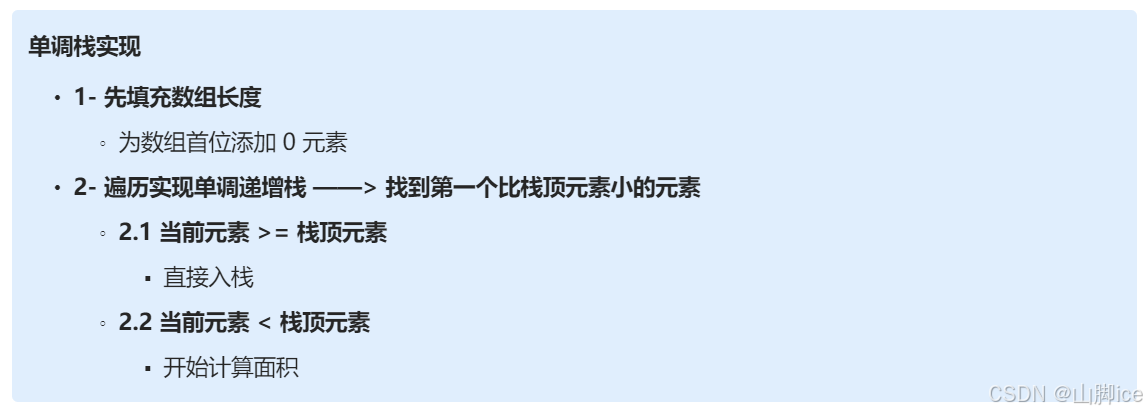
java
class Solution {
public int largestRectangleArea(int[] heights) {
int len = heights.length;
int[] newH = new int[len+2];
int index = 1;
for(int i = 0 ; i < len;i++){
newH[index++] = heights[i];
}
heights = newH;
len = heights.length;
Stack<Integer> st = new Stack<>();
int res = 0;
st.push(0);
// 单调栈
for(int i = 0 ; i < len;i++){
if(heights[i] >= heights[st.peek()]){
st.push(i);
}else{
while(heights[i] < heights[st.peek()]){
int mid = st.peek();
st.pop();
int left = st.peek();
int right = i;
int h = heights[mid];
int width = right-left-1;
res = Math.max(res,h*width);
}
st.push(i);
}
}
return res;
}
}
3- ACM 实现
java
public class maxArea {
public static int maxA(int[] heights){
int len = heights.length;
int[] newH = new int[len+2];
int index = 1;
for(int i = 1 ; i < len;i++){
newH[index++] = heights[i];
}
heights = newH;
len = heights.length;
Stack<Integer> st = new Stack<>();
int res = 0;
st.push(0);
// 单调栈
for(int i = 0 ; i < len;i++){
if(heights[i] >= heights[st.peek()]){
st.push(i);
}else{
while(heights[i] < heights[st.peek()]){
int mid = st.peek();
st.pop();
int left = st.peek();
int right = i;
int h = heights[mid];
int width = right-left-1;
res = Math.max(res,h*width);
}
st.push(i);
}
}
return res;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String input = sc.nextLine();
input = input.replace("[","").replace("]","");
String[] parts = input.split(",");
int[] nums = new int[parts.length];
for(int i = 0 ; i < nums.length;i++){
nums[i] = Integer.parseInt(parts[i]);
}
System.out.println("结果是"+maxA(nums));
}
}