实现AP模式下,http-server功能
在ESP8266_RTOS_SDK\ESP8266_RTOS_SDK\examples\wifi\getting_started\softAP增加webserver部分代码
1. 代码
//softap_example_main.c
c
/* WiFi softAP Example
This example code is in the Public Domain (or CC0 licensed, at your option.)
Unless required by applicable law or agreed to in writing, this
software is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
CONDITIONS OF ANY KIND, either express or implied.
*/
#include <string.h>
#include "freertos/FreeRTOS.h"
#include "freertos/task.h"
#include "esp_system.h"
#include "esp_wifi.h"
#include "esp_event.h"
#include "esp_log.h"
#include "nvs_flash.h"
#include "lwip/err.h"
#include "lwip/sys.h"
#include "esp_http_server.h"
#include "web_server.h"
/* The examples use WiFi configuration that you can set via project configuration menu.
If you'd rather not, just change the below entries to strings with
the config you want - ie #define EXAMPLE_WIFI_SSID "mywifissid"
*/
#define EXAMPLE_ESP_WIFI_SSID CONFIG_ESP_WIFI_SSID
#define EXAMPLE_ESP_WIFI_PASS ""//CONFIG_ESP_WIFI_PASSWORD
#define EXAMPLE_MAX_STA_CONN CONFIG_ESP_MAX_STA_CONN
static const char *TAG = "wifi softAP";
static httpd_handle_t server = NULL;
static void wifi_event_handler(void* arg, esp_event_base_t event_base,
int32_t event_id, void* event_data)
{
if (event_id == WIFI_EVENT_AP_STACONNECTED) {
wifi_event_ap_staconnected_t* event = (wifi_event_ap_staconnected_t*) event_data;
ESP_LOGI(TAG, "station "MACSTR" join, AID=%d",
MAC2STR(event->mac), event->aid);
} else if (event_id == WIFI_EVENT_AP_STADISCONNECTED) {
wifi_event_ap_stadisconnected_t* event = (wifi_event_ap_stadisconnected_t*) event_data;
ESP_LOGI(TAG, "station "MACSTR" leave, AID=%d",
MAC2STR(event->mac), event->aid);
}
}
void wifi_init_softap()
{
tcpip_adapter_init();
ESP_ERROR_CHECK(esp_event_loop_create_default());
wifi_init_config_t cfg = WIFI_INIT_CONFIG_DEFAULT();
ESP_ERROR_CHECK(esp_wifi_init(&cfg));
ESP_ERROR_CHECK(esp_event_handler_register(WIFI_EVENT, ESP_EVENT_ANY_ID, &wifi_event_handler, NULL));
wifi_config_t wifi_config = {
.ap = {
.ssid = EXAMPLE_ESP_WIFI_SSID,
.ssid_len = strlen(EXAMPLE_ESP_WIFI_SSID),
.password = EXAMPLE_ESP_WIFI_PASS,
.max_connection = EXAMPLE_MAX_STA_CONN,
.authmode = WIFI_AUTH_WPA_WPA2_PSK
},
};
if (strlen(EXAMPLE_ESP_WIFI_PASS) == 0) {
wifi_config.ap.authmode = WIFI_AUTH_OPEN;
}
ESP_ERROR_CHECK(esp_wifi_set_mode(WIFI_MODE_AP));
ESP_ERROR_CHECK(esp_wifi_set_config(ESP_IF_WIFI_AP, &wifi_config));
ESP_ERROR_CHECK(esp_wifi_start());
ESP_LOGI(TAG, "wifi_init_softap finished. SSID:%s password:%s",
EXAMPLE_ESP_WIFI_SSID, EXAMPLE_ESP_WIFI_PASS);
}
void app_main()
{
ESP_ERROR_CHECK(nvs_flash_init());
ESP_LOGI(TAG, "ESP_WIFI_MODE_AP");
wifi_init_softap();
server = start_webserver();
}
//web_server.c
c
#include "esp_http_server.h"
#include "esp_log.h"
#include "web_server.h"
static const char *TAG_http = "wifi web_server";
// HTTP服务器配置
esp_err_t http_get_handler(httpd_req_t *req)
{
const char* resp_str = "<html><body><h1>Hello ESP8266!</h1></body></html>";
ESP_LOGI(TAG_http, "Send Index HTML");
httpd_resp_send(req, resp_str, strlen(resp_str));
return ESP_OK;
}
httpd_handle_t start_webserver(void)
{
httpd_handle_t server = NULL;
httpd_config_t config = HTTPD_DEFAULT_CONFIG();
// 设置HTTP请求处理路由
httpd_uri_t uri = {
.uri = "/",
.method = HTTP_GET,
.handler = http_get_handler,
.user_ctx = NULL
};
// 启动HTTP服务器
ESP_LOGI(TAG_http, "Starting server on port: '%d'", config.server_port);
if (httpd_start(&server, &config) == ESP_OK) {
ESP_LOGI(TAG_http, "Server started");
ESP_LOGI(TAG_http, "Adding URI: %s", uri.uri);
if (httpd_register_uri_handler(server, &uri) == ESP_OK) {
ESP_LOGI(TAG_http, "URI added");
}
}
return server;
}
//web_server.h
c
#ifndef EXAMPLES_WIFI_GETTING_STARTED_SOFTAP_MAIN_WEB_SERVER_H_
#define EXAMPLES_WIFI_GETTING_STARTED_SOFTAP_MAIN_WEB_SERVER_H_
httpd_handle_t start_webserver(void);
#endif /* EXAMPLES_WIFI_GETTING_STARTED_SOFTAP_MAIN_WEB_SERVER_H_ */
2. 下载
bash
Writing at 0x00000000... (100 %)
Wrote 11088 bytes (7436 compressed) at 0x00000000 in 0.7 seconds (effective 134.8 kbit/s)...
Hash of data verified.
Compressed 473120 bytes to 302098...
Writing at 0x00010000... (5 %)
Writing at 0x00014000... (10 %)
Writing at 0x00018000... (15 %)
Writing at 0x0001c000... (21 %)
Writing at 0x00020000... (26 %)
Writing at 0x00024000... (31 %)
Writing at 0x00028000... (36 %)
Writing at 0x0002c000... (42 %)
Writing at 0x00030000... (47 %)
Writing at 0x00034000... (52 %)
Writing at 0x00038000... (57 %)
Writing at 0x0003c000... (63 %)
Writing at 0x00040000... (68 %)
Writing at 0x00044000... (73 %)
Writing at 0x00048000... (78 %)
Writing at 0x0004c000... (84 %)
Writing at 0x00050000... (89 %)
Writing at 0x00054000... (94 %)
Writing at 0x00058000... (100 %)
Wrote 473120 bytes (302098 compressed) at 0x00010000 in 26.6 seconds (effective 142.4 kbit/s)...
Hash of data verified.
Compressed 3072 bytes to 83...
Writing at 0x00008000... (100 %)
Wrote 3072 bytes (83 compressed) at 0x00008000 in 0.0 seconds (effective 1890.6 kbit/s)...
Hash of data verified.
3. 效果
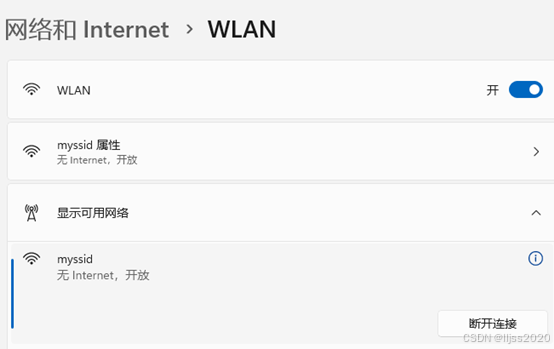
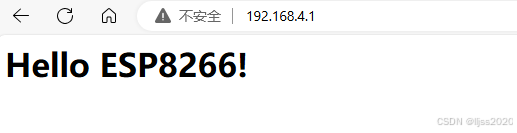