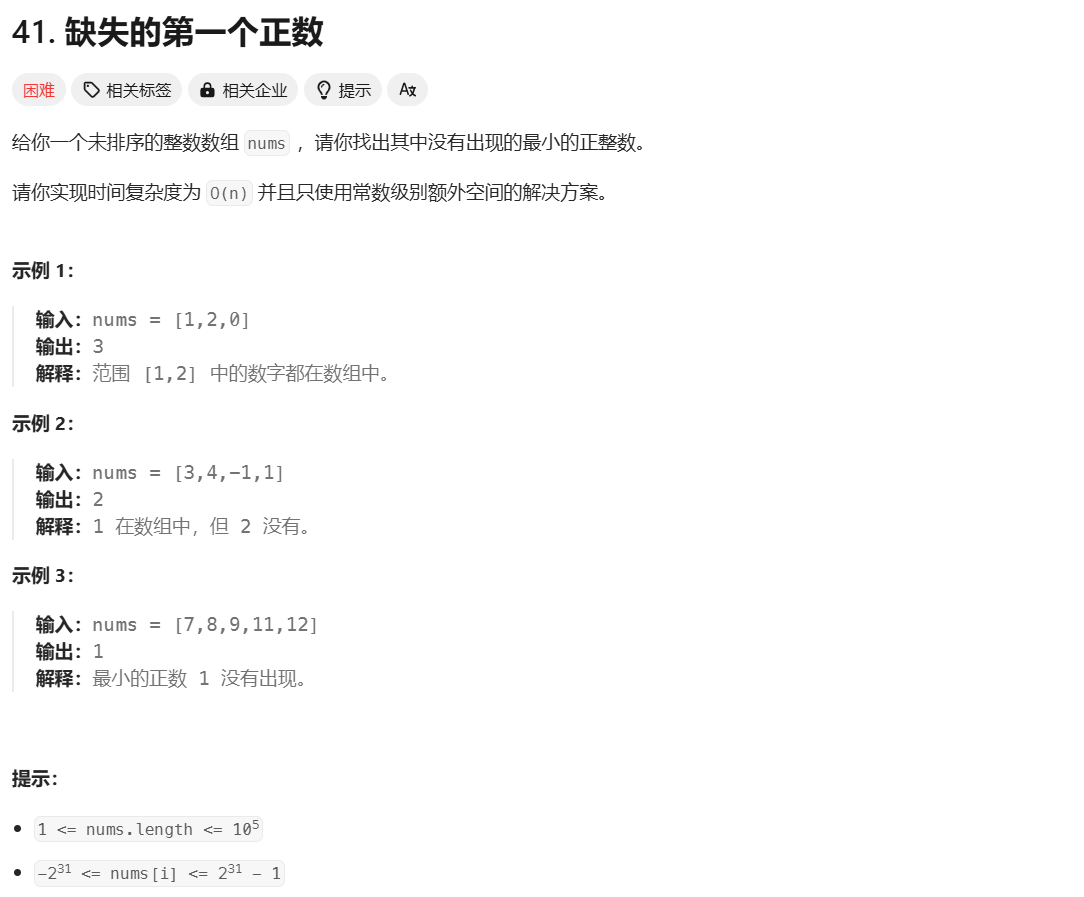
题目意思是找出第一个没出现的最小正整数。
Explanation:
-
Move Numbers to Correct Positions:
- The idea is to place each number in its corresponding index. For example,
1
should be at index0
,2
should be at index1
, and so on. This is done using awhile
loop to swap the elements until all valid numbers (those within the range1
ton
) are in their respective positions.
- The idea is to place each number in its corresponding index. For example,
-
Find the First Missing Positive:
- After the first step, iterate through the array. The first position where the element is not equal to
i + 1
is the smallest missing positive integer.
- After the first step, iterate through the array. The first position where the element is not equal to
-
Return
n + 1
if all numbers are in correct places:- If all numbers are in their correct places, the smallest missing positive integer must be
n + 1
.
- If all numbers are in their correct places, the smallest missing positive integer must be
Time Complexity:
- The time complexity is O ( n ) O(n) O(n) because each element is swapped at most once.
Space Complexity:
- The space complexity is O ( 1 ) O(1) O(1) since we are using constant extra space.
cpp
class Solution {
public:
int firstMissingPositive(vector<int>& nums) {
int n = nums.size(); //由于数组下标是从0开始,所以值为 n 的元素的下标按顺序是 n - 1
//例如1的下标是0,2的下标是1...
for(int i = 0; i < n; i++) {
//仅当当前元素值的范围介于[1,n]之间,并且它所该移动到的位置上的元素不相等时我们移动它到这个位置
//值为 nums[i] 的元素按顺序排列的下标是 nums[i] - 1
while(nums[i] > 0 && nums[i] <=n && nums[i] != nums[nums[i] - 1]) {
swap(nums[i], nums[nums[i] - 1]);
}
}
//然后,我们遍历数组,当下标 i 对应的值不等于 i + 1 时,返回 i + 1
for(int i = 0; i < n; i++) {
if(nums[i] != i + 1) {
return i + 1;
}
}
//如果在上面的循环中并没有return,说明数组所以元素值被排列成了 1,2,...,n
return n + 1;
}
};