图形验证码是网站安全防护的重要组成部分,能有效防止自动化脚本进行恶意操作,如何实现一个简单的运算图形验证码?本文封装了一个简单的js类,可以用于生成简单但安全的图形验证码。它支持自定义验证码样式,包括字体大小、颜色以及干扰线的数量和样式。如下图。
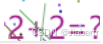
核心js文件如下:
javascript
// mcaptcha.js
export class Mcaptcha {
constructor(options) {
this.options = options;
this.fontSize = 24;
this.init();
this.refresh();
}
init() {
this.ctx = wx.createCanvasContext(this.options.el);
this.ctx.setTextBaseline("middle");
this.ctx.setFillStyle(this.randomColor(180, 240));
}
refresh() {
var code = '';
var num1 = this.randomNum(0, 9)
var num2 = this.randomNum(0, 9)
this.options.createCodeImg = code;
code = num1 + '+' + num2 + '=?'
this.options.num1 = num1;
this.options.num2 = num2;
let arr = [code]
if (arr.length === 0) {
arr = ['e', 'r', 'r', 'o', 'r'];
};
let offsetLeft = this.options.width * 0.6 / (arr.length - 1);
let marginLeft = this.options.width * 0.2;
arr.forEach((item, index) => {
this.ctx.setFillStyle(this.randomColor(0, 180));
let size = this.randomNum(24, this.fontSize);
this.ctx.setFontSize(size);
let dis = offsetLeft * index + marginLeft - size * 0.3;
let deg = this.randomNum(-1, 1);
this.ctx.translate(dis, this.options.height * 0.45);
this.ctx.rotate(deg * Math.PI / 180);
this.ctx.fillText(item, 2, this.randomNum(0,10));
this.ctx.rotate(-deg * Math.PI / 180);
this.ctx.translate(-20, -this.options.height * 0.6);
})
for (var i = 0; i < 4; i++) {
this.ctx.strokeStyle = this.randomColor(40, 180);
this.ctx.beginPath();
this.ctx.moveTo(this.randomNum(0, this.options.width), this.randomNum(0, this.options.height));
this.ctx.lineTo(this.randomNum(0, this.options.width), this.randomNum(0, this.options.height));
this.ctx.stroke();
}
for (var i = 0; i < this.options.width / 4; i++) {
this.ctx.fillStyle = this.randomColor(0, 255);
this.ctx.beginPath();
this.ctx.arc(this.randomNum(0, this.options.width), this.randomNum(0, this.options.height), 1, 0, 2 * Math.PI);
this.ctx.fill();
}
this.ctx.draw();
}
validate(code) {
var code = Number(code)
var v_code = this.options.num1 + this.options.num2
if (code == v_code) {
return true
} else {
this.refresh()
return false
}
}
randomNum(min, max) {
return Math.floor(Math.random() * (max - min) + min);
}
randomColor(min, max) {
let r = this.randomNum(min, max);
let g = this.randomNum(min, max);
let b = this.randomNum(min, max);
return "rgb(" + r + "," + g + "," + b + ")";
}
}
使用方法
以微信小程序为例,其他环境适当修改。
- 引入Mcaptcha.js
javascript
import Mcaptcha from './mcaptcha.js';
2.初始化Mcaptcha实例
在你的页面的JS文件中,创建一个Mcaptcha实例,并传入相应的配置选项。
javascript
Page({
onLoad: function() {
new Mcaptcha({
el: 'captchaCanvas', // canvas的ID
width: 300, // canvas的宽度
height: 150 // canvas的高度
});
}
});
3.在WXML中添加Canvas元素
在页面的WXML文件中,添加一个Canvas元素,用于展示验证码。
html
<canvas canvas-id="captchaCanvas" style="width: 300px; height: 150px;"></canvas>
4.使用Mcaptcha实例生成验证码
调用Mcaptcha实例的refresh
方法来生成验证码。
javascript
Page({
// ...
onShow: function() {
// 假设mcaptcha是之前创建的Mcaptcha实例
mcaptcha.refresh();
}
});
5.验证用户输入
当用户输入验证码并提交时,调用Mcaptcha实例的validate
方法进行验证
javascript
Page({
// ...
checkCaptcha: function(inputCode) {
// 假设mcaptcha是之前创建的Mcaptcha实例
if (mcaptcha.validate(inputCode)) {
wx.showToast({
title: '验证成功!',
icon: 'success'
});
} else {
wx.showToast({
title: '验证失败,请重试。',
icon: 'none'
});
}
}
});
Mcaptcha.js的核心方法
refresh()
: 生成新的验证码,包括随机数字、运算符和干扰元素。validate(code)
: 验证用户输入的验证码是否正确,返回布尔值。randomNum(min, max)
: 生成指定范围内的随机数。randomColor(min, max)
: 生成指定范围内的随机颜色。