功能需求:实现选中一个或多个执行批量删除操作
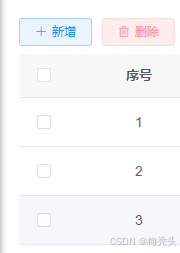
在elementUI官网选择一个表格样式模板,Element - The world's most popular Vue UI framework
这里采用的是
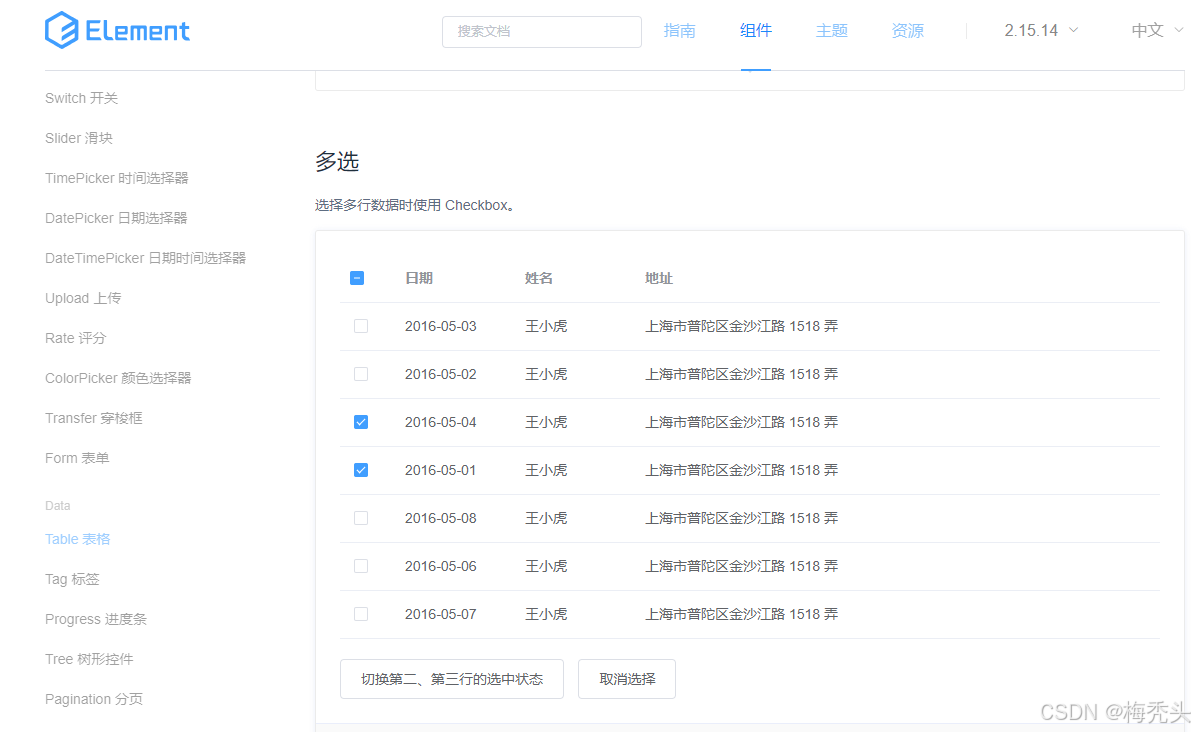
将代码复制到前端,这里是index.vue
html
<template>
<el-button type="danger" plain icon="el-icon-delete" size="mini" @click="handleDeleteByBatch">删除</el-button>
<el-table ref="multipleTable" :data="tableData" tooltip-effect="dark" style="width: 100%"
@selection-change="handleSelectionChange">
<el-table-column type="selection" width="55">
</el-table-column>
<el-table-column label="日期" width="120">
<template slot-scope="scope">{{ scope.row.date }}</template>
</el-table-column>
<el-table-column prop="name" label="姓名" width="120">
</el-table-column>
<el-table-column prop="address" label="地址" show-overflow-tooltip>
</el-table-column>
</el-table>
</template>
<script>
export default {
data() {
return {
//表格数据
tableData: [],
//多选ids
multipleSelection: []
}
},
methods: {
handleSelectionChange(row) {
this.multipleSelection = row;
}
}
}
</script>
添加删除方法
1.script处导入后端执行删除方法的文件a.js(位于api/student文件夹下)
javascript
<script>
import { deleteByBatch } from '@/api/student/a'
</script>
2.a.js
javascript
import request from '@/utils/request'
// 批量删除
export function deleteByBatch(ids) {
return request({
url: '/student/deleteByBatch/'+ids,
method: 'delete'
})
}
3.添加删除方法,修改handleSelectionChange方法
将选中的数组对象中的id传给multipleSelection:this.multipleSelection = row.map(item => item.id);
javascript
// 多选
handleSelectionChange(row) {
console.log("选中row", row);
this.multipleSelection = row.map(item => item.id);
console.log("选中id", this.multipleSelection);
},
// 批量删除
handleDeleteByBatch() {
this.$confirm('确定删除选中的数据吗?', {
confirmButtonText: "确定",
cancelButtonText: "取消",
type: "warning",
}).then(() => {
deleteByBatch(this.multipleSelection).then(res => {
if (res.code === 200) {
this.$message.success("删除成功");
this.getCollectorList();
} else {
this.$message.error("删除失败");
}
})
})
},
后端代码
controller,AjaxResult是返回数据类型类,有需要的文末复制,更全面的代码可以gitee上下载ruoyi框架
java
@RestController
@RequestMapping("/student")
public class AController {
@Autowired
private AService aService;
@DeleteMapping("/deleteByBatch/{ids}")
@ApiOperation("批量删除")
public AjaxResult deleteByBatch( @PathVariable List<Long> ids){
return AjaxResult.success(aService.deleteByBatch(ids));
}
}
xml(此处做的逻辑删除)
XML
<update id="deleteByBatch" parameterType="com.system.domain.A">
update a set del_flag = 2 where id in
<foreach collection="ids" item="id" open="(" separator="," close=")">
#{id}
</foreach>
</update>
AjaxResult
java
public class AjaxResult extends HashMap<String, Object>
{
private static final long serialVersionUID = 1L;
/** 状态码 */
public static final String CODE_TAG = "code";
/** 返回内容 */
public static final String MSG_TAG = "msg";
/** 数据对象 */
public static final String DATA_TAG = "data";
/**
* 初始化一个新创建的 AjaxResult 对象,使其表示一个空消息。
*/
public AjaxResult()
{
}
/**
* 初始化一个新创建的 AjaxResult 对象
*
* @param code 状态码
* @param msg 返回内容
*/
public AjaxResult(int code, String msg)
{
super.put(CODE_TAG, code);
super.put(MSG_TAG, msg);
}
/**
* 初始化一个新创建的 AjaxResult 对象
*
* @param code 状态码
* @param msg 返回内容
* @param data 数据对象
*/
public AjaxResult(int code, String msg, Object data)
{
super.put(CODE_TAG, code);
super.put(MSG_TAG, msg);
if (StringUtils.isNotNull(data))
{
super.put(DATA_TAG, data);
}
}
/**
* 返回成功消息
*
* @return 成功消息
*/
public static AjaxResult success()
{
return AjaxResult.success("操作成功");
}
/**
* 返回成功数据
*
* @return 成功消息
*/
public static AjaxResult success(Object data)
{
return AjaxResult.success("操作成功", data);
}
/**
* 返回成功消息
*
* @param msg 返回内容
* @return 成功消息
*/
public static AjaxResult success(String msg)
{
return AjaxResult.success(msg, null);
}
/**
* 返回成功消息
*
* @param msg 返回内容
* @param data 数据对象
* @return 成功消息
*/
public static AjaxResult success(String msg, Object data)
{
return new AjaxResult(HttpStatus.SUCCESS, msg, data);
}
/**
* 返回警告消息
*
* @param msg 返回内容
* @return 警告消息
*/
public static AjaxResult warn(String msg)
{
return AjaxResult.warn(msg, null);
}
/**
* 返回警告消息
*
* @param msg 返回内容
* @param data 数据对象
* @return 警告消息
*/
public static AjaxResult warn(String msg, Object data)
{
return new AjaxResult(HttpStatus.WARN, msg, data);
}
/**
* 返回错误消息
*
* @return 错误消息
*/
public static AjaxResult error()
{
return AjaxResult.error("操作失败");
}
/**
* 返回错误消息
*
* @param msg 返回内容
* @return 错误消息
*/
public static AjaxResult error(String msg)
{
return AjaxResult.error(msg, null);
}
/**
* 返回错误消息
*
* @param msg 返回内容
* @param data 数据对象
* @return 错误消息
*/
public static AjaxResult error(String msg, Object data)
{
return new AjaxResult(HttpStatus.ERROR, msg, data);
}
/**
* 返回错误消息
*
* @param code 状态码
* @param msg 返回内容
* @return 错误消息
*/
public static AjaxResult error(int code, String msg)
{
return new AjaxResult(code, msg, null);
}
/**
* 是否为成功消息
*
* @return 结果
*/
public boolean isSuccess()
{
return Objects.equals(HttpStatus.SUCCESS, this.get(CODE_TAG));
}
/**
* 是否为警告消息
*
* @return 结果
*/
public boolean isWarn()
{
return Objects.equals(HttpStatus.WARN, this.get(CODE_TAG));
}
/**
* 是否为错误消息
*
* @return 结果
*/
public boolean isError()
{
return Objects.equals(HttpStatus.ERROR, this.get(CODE_TAG));
}
/**
* 方便链式调用
*
* @param key 键
* @param value 值
* @return 数据对象
*/
@Override
public AjaxResult put(String key, Object value)
{
super.put(key, value);
return this;
}
}