1.My_string
头文件
#ifndef MY_STRINGHEAD_H
#define MY_STRINGHEAD_H
#include <iostream>
#include <cstring>
using namespace std;
class My_string
{
private:
char *ptr;//指向字符数组的指针
int size;//字符数组的最大容量
int len ;//当前字符串的长度
public:
My_string()
{
this->size=15;
this->len=0;
this->ptr[len]='\0';
}
My_string(const char *src);//有参构造
My_string(const My_string & other);//拷贝构造
My_string & operator=(const My_string &other);
~My_string();//析构函数
bool My_empty();//判空
void push_back(char value);//尾插
void pop_back();//尾删
char * data ();
char &at(int index);
int get_len();
int get_size();
void Double_String();//双倍扩容
void show();
};
#endif // MY_STRINGHEAD_H
功能函数
#include "my_stringhead.h"
My_string::My_string(const char *src)//有参构造
{
this->size = strlen(src);
this->len=this->size;
this->ptr = new char[this->size+1];
strcpy(ptr,src);
}
My_string::My_string(const My_string & other):size(other.size),len(other.len)//拷贝构造
{
this->ptr = new char[this->size+1];
strcpy(this->ptr,other.ptr);
}
My_string & My_string::operator=(const My_string &other)//拷贝赋值
{
if(this!=&other)
{
this->size = other.size;
this->len = other.len;
strcpy(this->ptr,other.ptr);
}
return *this;
}
My_string::~My_string()//析构函数
{
delete this->ptr;
}
bool My_string:: My_empty()//判空
{
return len==0;
}
void My_string:: push_back(char value)//尾插
{
this->size++;
char *Newptr=new char[this->size];
strcpy(Newptr,ptr);
delete this->ptr;
this->ptr=Newptr;
this->ptr[this->len]=value;
this->len++;
this->ptr[this->len]='\0';
}
void My_string::pop_back()//尾删
{
this->size--;
this->len--;
this->ptr[this->len]='\0';
}
char & My_string::at(int index)
{
if(index>=0&&index<this->len)
{
return this->ptr[index];
}
else
{
cout<<"输入不合法"<<endl;
exit(-1);
}
}
char *My_string::data ()//转换为c风格字符串
{
return this->ptr;
}
int My_string::get_len()
{
return this->len;
}
int My_string:: get_size()
{
return this->len;
}
void My_string::Double_String()//双倍扩容
{
if(this->len+1>=this->size)
{
this->size*=2;
char *Newptr=new char[this->size];
strcpy(Newptr,ptr);
delete this->ptr;
this->ptr=Newptr;
}
}
void My_string:: show()
{
cout<<this->ptr<<endl;
}
主函数
#include "my_stringhead.h"
int main()
{
My_string str("hello world");
str.show();
My_string str1;
str1.operator = (str);
str1.show();
return 0;
}
2.思维导图
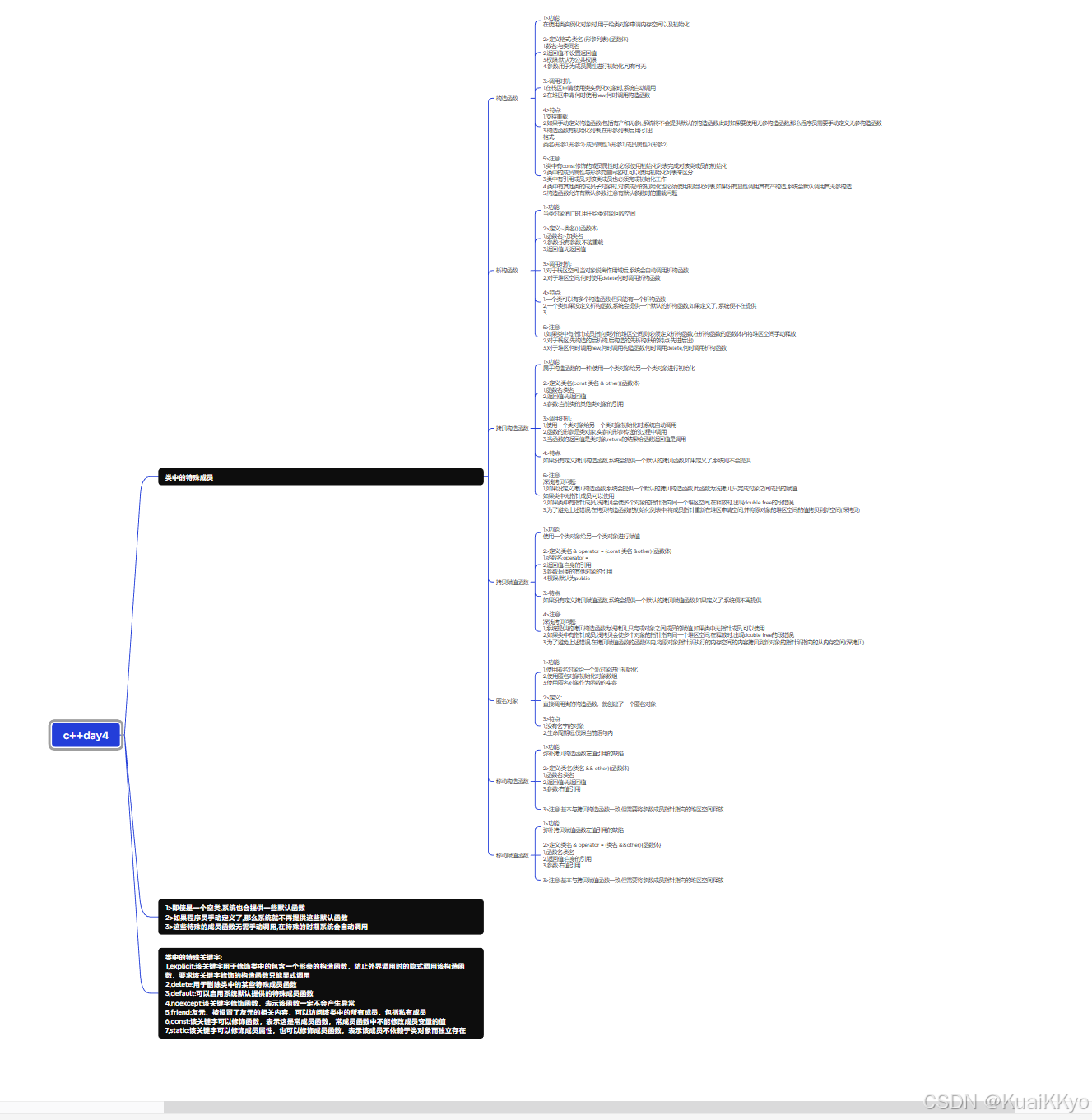