目录
513.找树左下角的值
题目
给定一个二叉树的 根节点 root
,请找出该二叉树的 最底层 最左边 节点的值。
假设二叉树中至少有一个节点。
示例:
输入: [1,2,3,4,null,5,6,null,null,7]
输出: 7
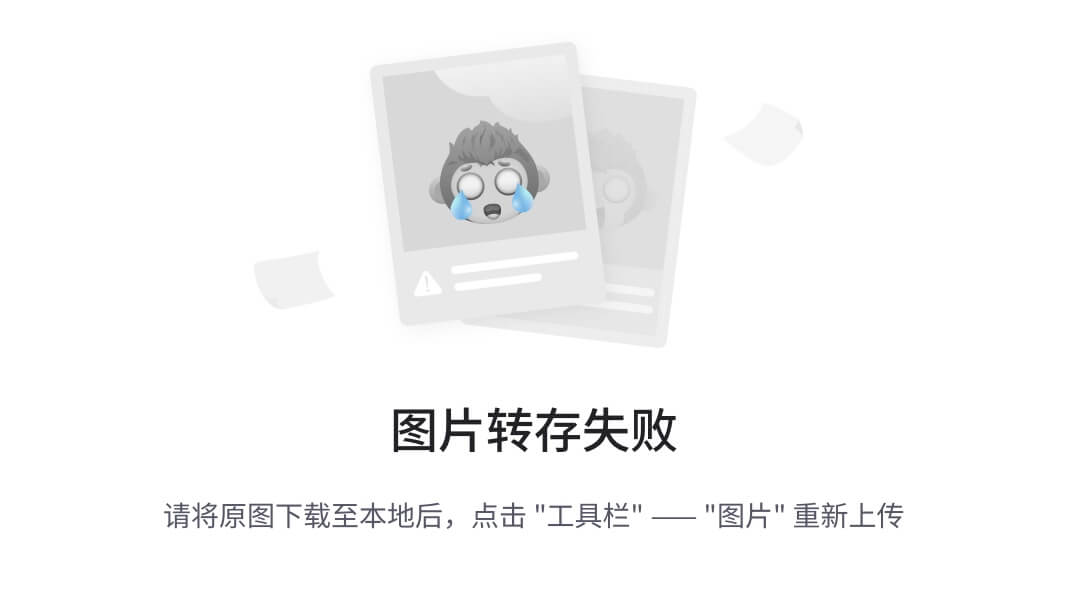
思路
使用层序遍历,每遍历到新的一层,就记录下这一层最左侧的节点。
递归法:定义两个全局变量,一个存储已遍历到的叶子节点的最大深度,一个存储当前结果。
每当遇到叶子节点时,判断当前深度是否大于最大深度,如果大于,则更新最大深度为当前深度,并且将结果更新为该节点的值。
在寻找最大深度的过程中使用回溯,调用递归函数前将depth++
再进行传参,调用完之后再depth--
,确保对左右两个孩子调用递归函数时深度相同(此处可以优化,见代码
题解
层序遍历:
java
class Solution {
public int findBottomLeftValue(TreeNode root) {
Deque<TreeNode> deque = new ArrayDeque<>();
int res = root.val;
deque.offer(root);
while (!deque.isEmpty()) {
res = deque.peek().val;
int size = deque.size();
for (int i = 0; i < size; i++) {
TreeNode node = deque.poll();
if (node.left != null)
deque.offer(node.left);
if (node.right != null)
deque.offer(node.right);
}
}
return res;
}
}
递归法:
java
class Solution {
int MAX_DPETH = -1;
int res = 0;
public int findBottomLeftValue(TreeNode root) {
way(root, 1);
return res;
}
void way(TreeNode root, int dpeth) {
if (root == null)
return;
if (root.left == null && root.right == null) {
if (dpeth > MAX_DPETH) {
MAX_DPETH = dpeth;
res = root.val;
}
}
if (root.left != null) {
dpeth++;
way(root.left, dpeth);
dpeth--;
}
if (root.right != null) {
dpeth++;
way(root.right, dpeth);
dpeth--;
}
// way(root.left,dpeth+1); 隐藏回溯
// way(root.right,dpeth+1);
}
}
112.路径总和
题目
给你二叉树的根节点 root
和一个表示目标和的整数 targetSum
。判断该树中是否存在 根节点到叶子节点 的路径,这条路径上所有节点值相加等于目标和 targetSum
。如果存在,返回 true
;否则,返回 false
。
叶子节点 是指没有子节点的节点。
示例1:
输入:root = [5,4,8,11,null,13,4,7,2,null,null,null,1], targetSum = 22
输出:true
解释:等于目标和的根节点到叶节点路径如上图所示。
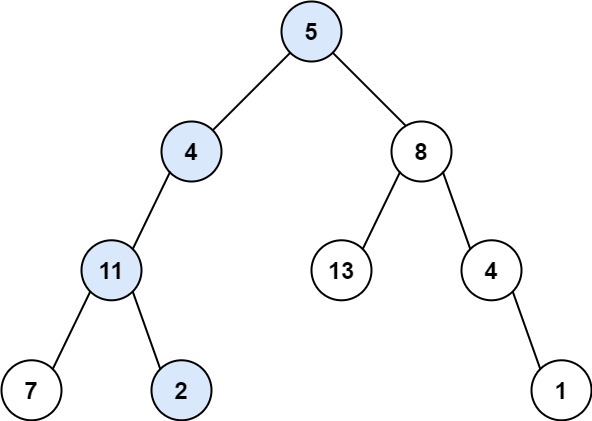
示例2:
输入:root = [], targetSum = 0
输出:false
解释:由于树是空的,所以不存在根节点到叶子节点的路径。
提示:
- 树中节点的数目在范围
[0, 5000]
内 -1000 <= Node.val <= 1000
-1000 <= targetSum <= 1000
思路
递归法思路比较简单。
除了递归法还可以使用层序遍历,使用两个队列分别存储节点和到该节点的值之和。
题解
独立解题:
java
class Solution {
boolean res = false;
public boolean hasPathSum(TreeNode root, int targetSum) {
getPathSum(root, 0, targetSum);
return res;
}
void getPathSum(TreeNode root, int sum, int targetSum) {
if (root == null)
return;
sum += root.val;
if (root.left == null && root.right == null && sum == targetSum)
res = true;
getPathSum(root.left, sum, targetSum);
getPathSum(root.right, sum, targetSum);
}
}
优化:
java
class Solution {
public boolean hasPathSum(TreeNode root, int targetSum) {
return getPathSum(root, 0, targetSum);
}
boolean getPathSum(TreeNode root, int sum, int targetSum) {
if (root == null)
return false;
sum += root.val;
if (root.left == null && root.right == null && sum == targetSum)
return true;
if (getPathSum(root.left, sum, targetSum))
return true;
if (getPathSum(root.right, sum, targetSum))
return true;
return false;
}
}
113.路径总和Ⅱ
题目
给你二叉树的根节点 root
和一个整数目标和 targetSum
,找出所有 从根节点到叶子节点 路径总和等于给定目标和的路径。
叶子节点 是指没有子节点的节点。
示例1:
输入:root = [5,4,8,11,null,13,4,7,2,null,null,5,1], targetSum = 22
输出:[[5,4,11,2],[5,8,4,5]]
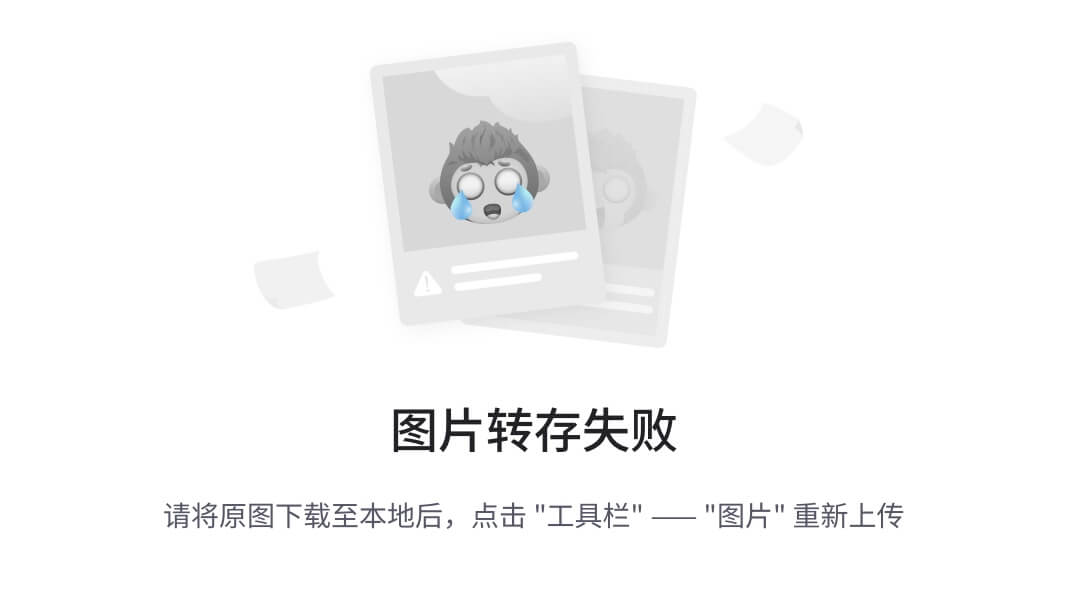
示例2:
输入:root = [1,2], targetSum = 0
输出:[]
提示:
- 树中节点总数在范围
[0, 5000]
内 -1000 <= Node.val <= 1000
-1000 <= targetSum <= 1000
思路
思路比较简单。
题解
独立题解:
java
class Solution {
public List<List<Integer>> pathSum(TreeNode root, int targetSum) {
List<List<Integer>> res = new ArrayList<>();
List<Integer> path = new ArrayList<>();
getpath(root, res, path, targetSum);
return res;
}
void getpath(TreeNode root, List res, List<Integer> path, int targetSum) {
if (root == null)
return;
path.add(root.val);
int sum = 0;
if (root.left == null && root.right == null) {
for (Integer i : path) {
sum += i;
}
if (sum == targetSum) {
res.add(path.toArray());
}
}
if(root.left!=null){
getpath(root.left, res, path, targetSum);
path.remove(path.size()-1);
}
if(root.right!=null){
getpath(root.right, res, path, targetSum);
path.remove(path.size()-1);
}
}
}
106.从中序与后序遍历序列构造二叉树
题目
106. 从中序与后序遍历序列构造二叉树 - 力扣(LeetCode)
给定两个整数数组 inorder
和 postorder
,其中 inorder
是二叉树的中序遍历, postorder
是同一棵树的后序遍历,请你构造并返回这颗 二叉树 。
示例1:
输入:inorder = [9,3,15,20,7], postorder = [9,15,7,20,3]
输出:[3,9,20,null,null,15,7]
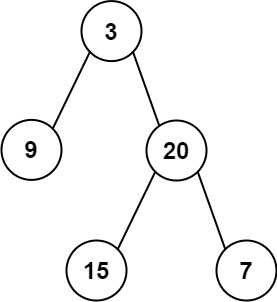
示例2:
输入:inorder = [-1], postorder = [-1]
输出:[-1]
提示:
1 <= inorder.length <= 3000
postorder.length == inorder.length
-3000 <= inorder[i], postorder[i] <= 3000
inorder
和postorder
都由 不同 的值组成postorder
中每一个值都在inorder
中inorder
保证是树的中序遍历postorder
保证是树的后序遍历
思路
视频讲解:LeetCode:106.从中序与后序遍历序列构造二叉树
题解
105.从前序与中序遍历序列构造二叉树
题目
105. 从前序与中序遍历序列构造二叉树 - 力扣(LeetCode)
给定两个整数数组 preorder
和 inorder
,其中 preorder
是二叉树的先序遍历 , inorder
是同一棵树的中序遍历,请构造二叉树并返回其根节点。
示例1:
输入:inorder = [9,3,15,20,7], postorder = [9,15,7,20,3]
输出:[3,9,20,null,null,15,7]
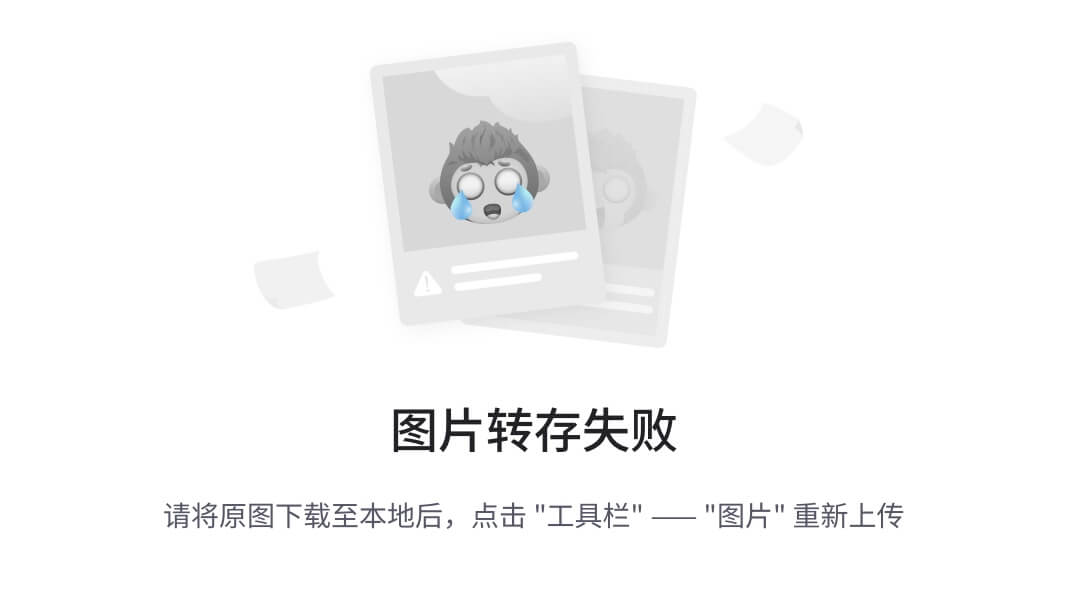
示例2:
输入:inorder = [-1], postorder = [-1]
输出:[-1]
思路
题解
norder` 是同一棵树的中序遍历,请构造二叉树并返回其根节点。
示例1:
输入:inorder = [9,3,15,20,7], postorder = [9,15,7,20,3]
输出:[3,9,20,null,null,15,7]
外链图片转存中...(img-zMkNCbaF-1727407896313)
示例2:
输入:inorder = [-1], postorder = [-1]
输出:[-1]
思路
题解