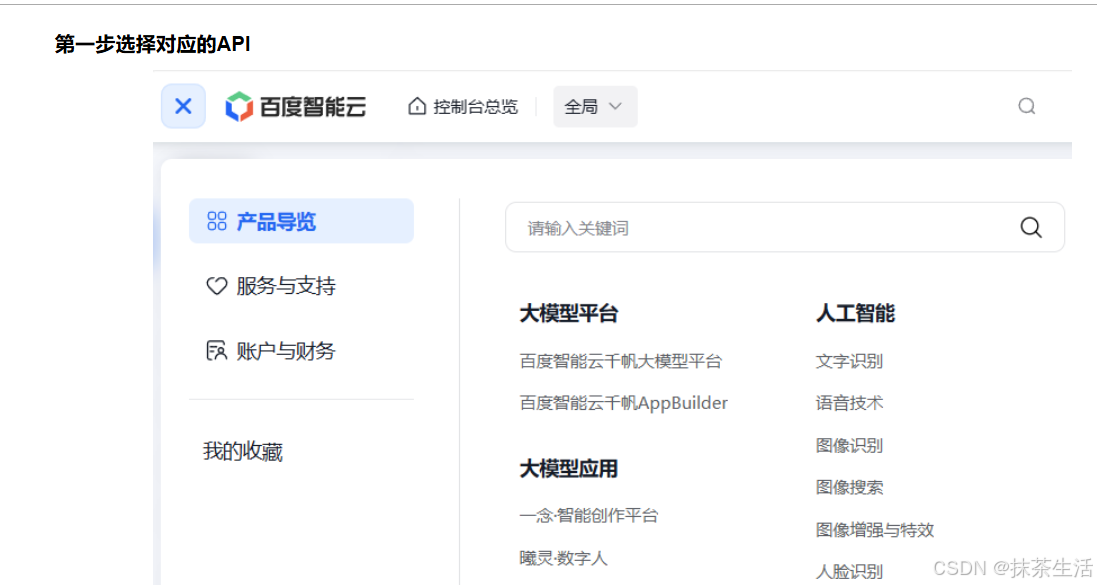
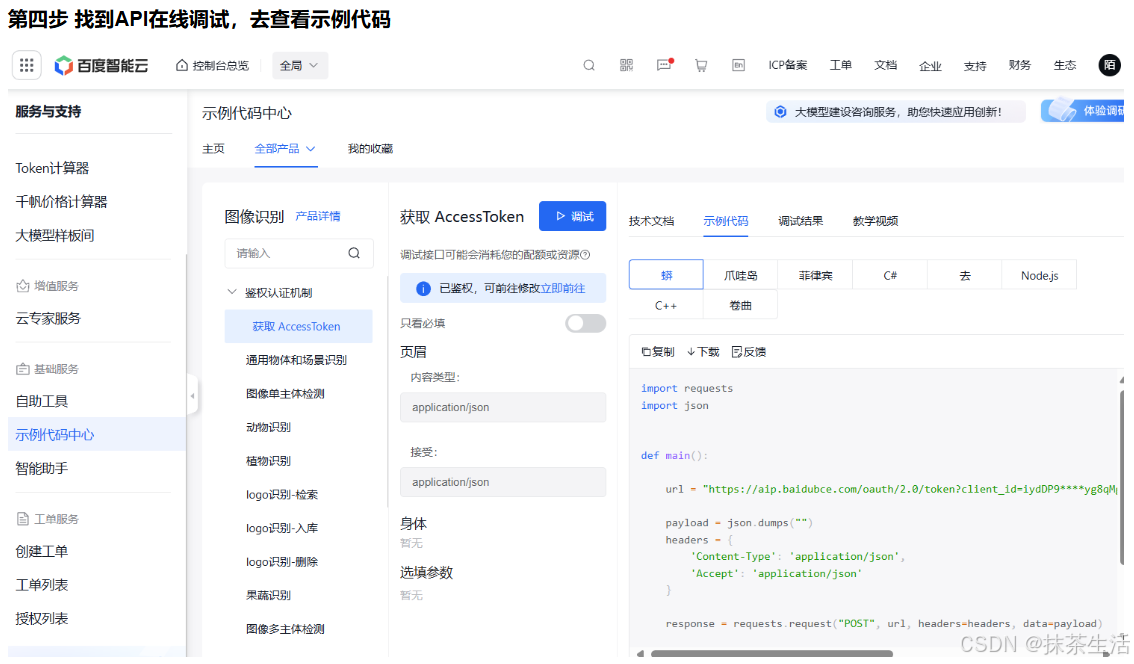
植物识别API
import base64
import urllib
import requests
API_KEY = "你的图像识别API_KEY"
SECRET_KEY = "你的图像识别SECRET_KEY"
def main():
url = "https://aip.baidubce.com/rest/2.0/image-classify/v1/plant?access_token=" + get_access_token()
# image 可以通过 get_file_content_as_base64("C:\fakepath\苹果.png",True) 方法获取
image=r"C:\Users\yly\Desktop\波罗蜜.png"
base_image=get_file_content_as_base64(image)
payload={'image':base_image}
headers = {
'Content-Type': 'application/x-www-form-urlencoded',
'Accept': 'application/json'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
def get_file_content_as_base64(path, urlencoded=False):
"""
获取文件base64编码
:param path: 文件路径
:param urlencoded: 是否对结果进行urlencoded
:return: base64编码信息
"""
with open(path, "rb") as f:
content = base64.b64encode(f.read()).decode("utf8")
if urlencoded:
content = urllib.parse.quote_plus(content)
return content
def get_access_token():
"""
使用 AK,SK 生成鉴权签名(Access Token)
:return: access_token,或是None(如果错误)
"""
url = "https://aip.baidubce.com/oauth/2.0/token"
params = {"grant_type": "client_credentials", "client_id": API_KEY, "client_secret": SECRET_KEY}
return str(requests.post(url, params=params).json().get("access_token"))
main()

人脸识别API
import base64
import urllib
import requests
API_KEY = "你的人脸识别API_KEY"
SECRET_KEY = "你的人脸识别SECRET_KEY"
def main():
url = "https://aip.baidubce.com/rest/2.0/face/v3/detect?access_token=" + get_access_token()
# image 可以通过 get_file_content_as_base64("C:\fakepath\微信截图_20240910210450.png",True) 方法获取
headers = {
'Content-Type': 'application/x-www-form-urlencoded',
'Accept': 'application/json'
}
image=r"C:\Users\yly\Desktop\liu.jpg"
base_image=get_file_content_as_base64(image)
payload = {"image":base_image,"image_type":"BASE64","face_field":'age,gender'}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
def get_file_content_as_base64(path, urlencoded=False):
"""
获取文件base64编码
:param path: 文件路径
:param urlencoded: 是否对结果进行urlencoded
:return: base64编码信息
"""
with open(path, "rb") as f:
content = base64.b64encode(f.read()).decode("utf8")
if urlencoded:
content = urllib.parse.quote_plus(content)
return content
def get_access_token():
"""
使用 AK,SK 生成鉴权签名(Access Token)
:return: access_token,或是None(如果错误)
"""
url = "https://aip.baidubce.com/oauth/2.0/token"
params = {"grant_type": "client_credentials", "client_id": API_KEY, "client_secret": SECRET_KEY}
return str(requests.post(url, params=params).json().get("access_token"))
main()
