1、整型和浮点型
a = 10
b = 1.1
print(type(a))
print(type(b))
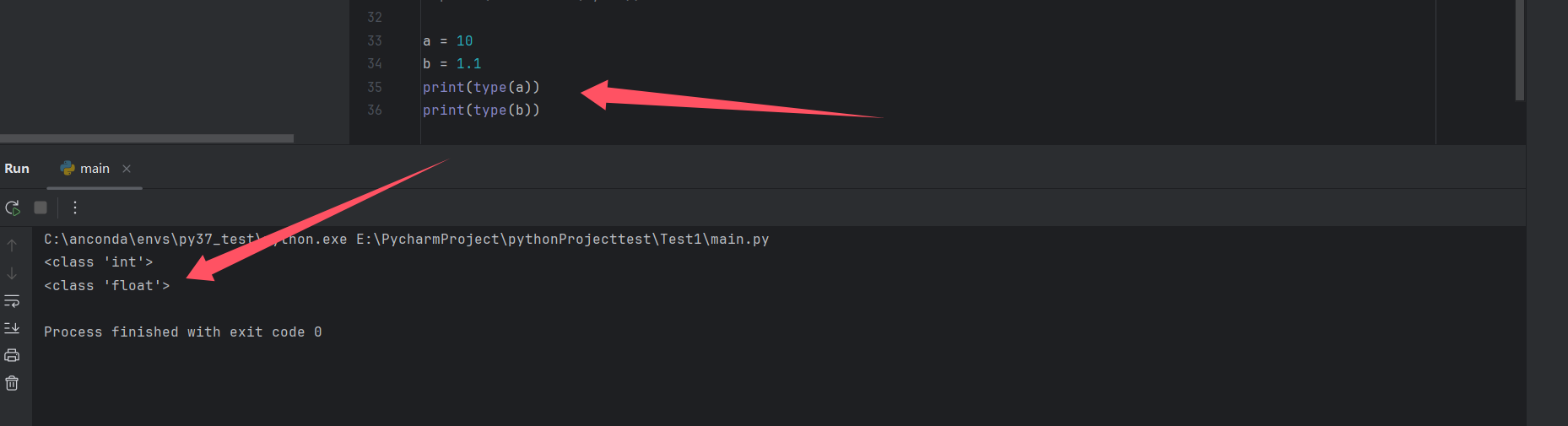
2、字符串
Python中字符串可以是单引号引用,也可以是双引号引用。
str1 = 'This is an example'
str2 = "This is an example"
print(str1,"\n",str2)
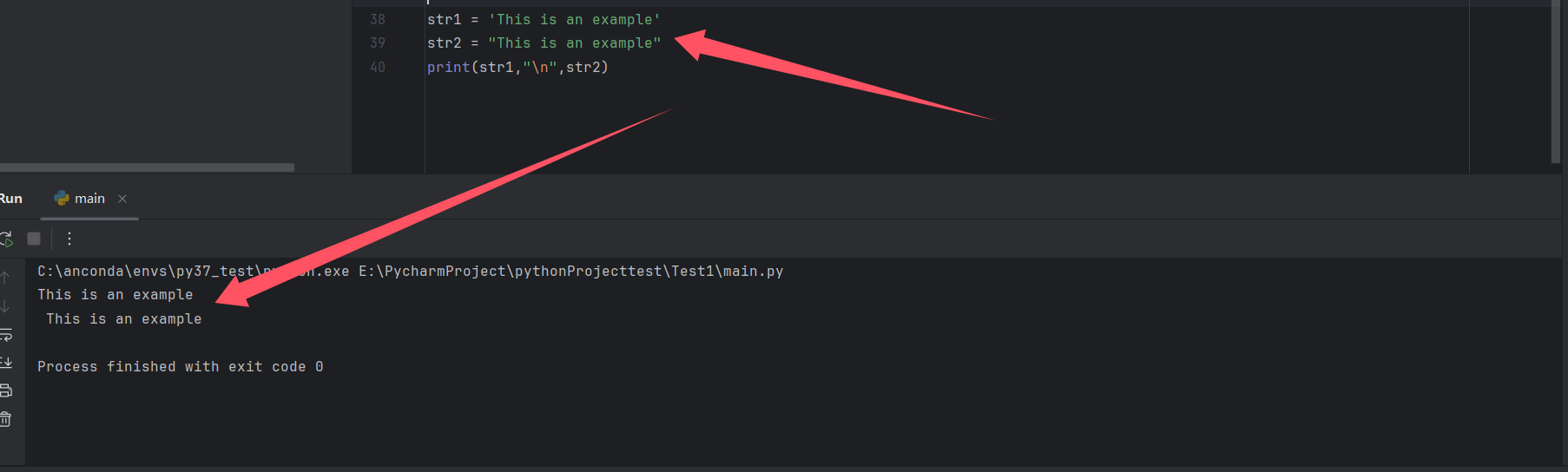
如果想要输入长字符串,其长度超过一行了怎么办呢?
可以使用三引号。
str1 = '''
This is an example
This is an example
'''
print(str1)
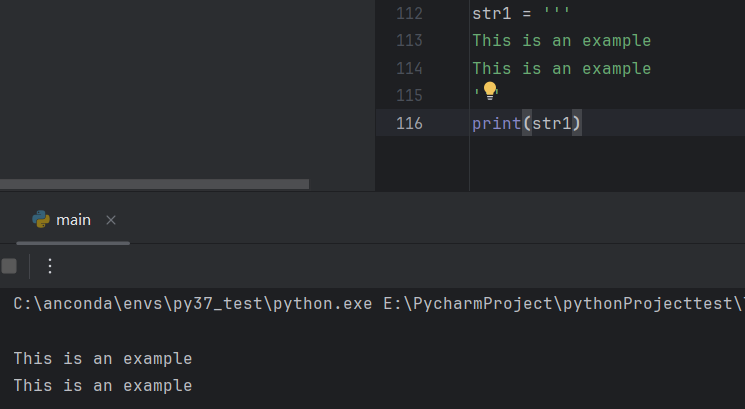
注意:如果字符串中有单引号,外层需要使用双引号。相似,如果字符串中有双引号,外层需要使用单引号。
str1 = "This's is an example"
str2 = 'This"s is an example'
print(str1)
print(str2)
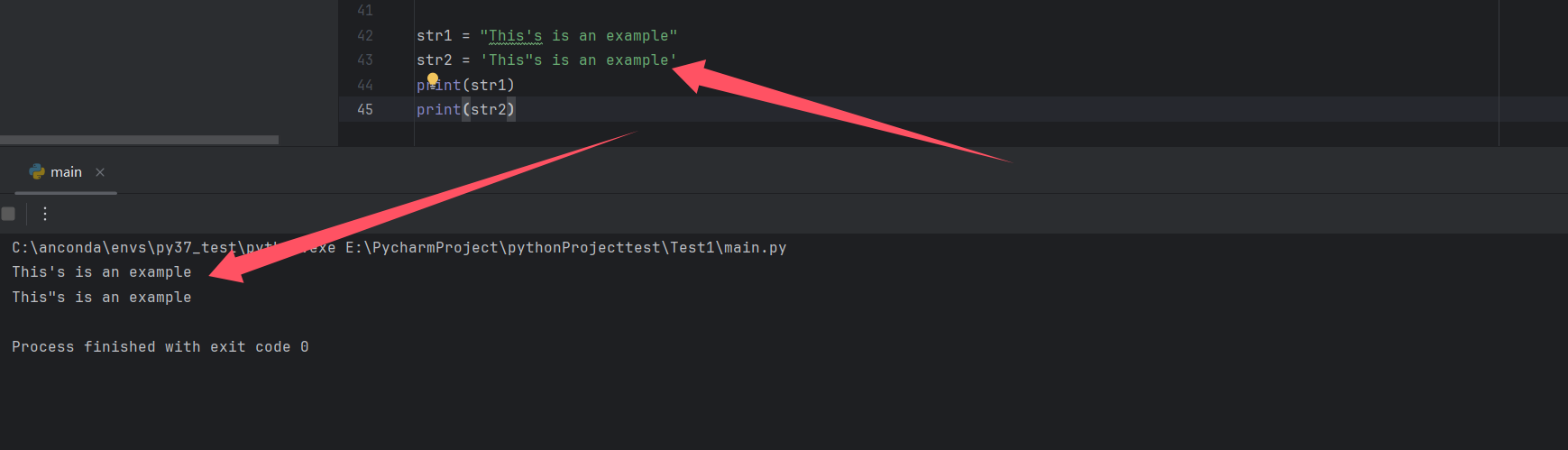
还有另一种方法:使用转义字符反斜杠\。
str1 = 'This\'s is an example'
str2 = "This\"s is an example"
print(str1)
print(str2)
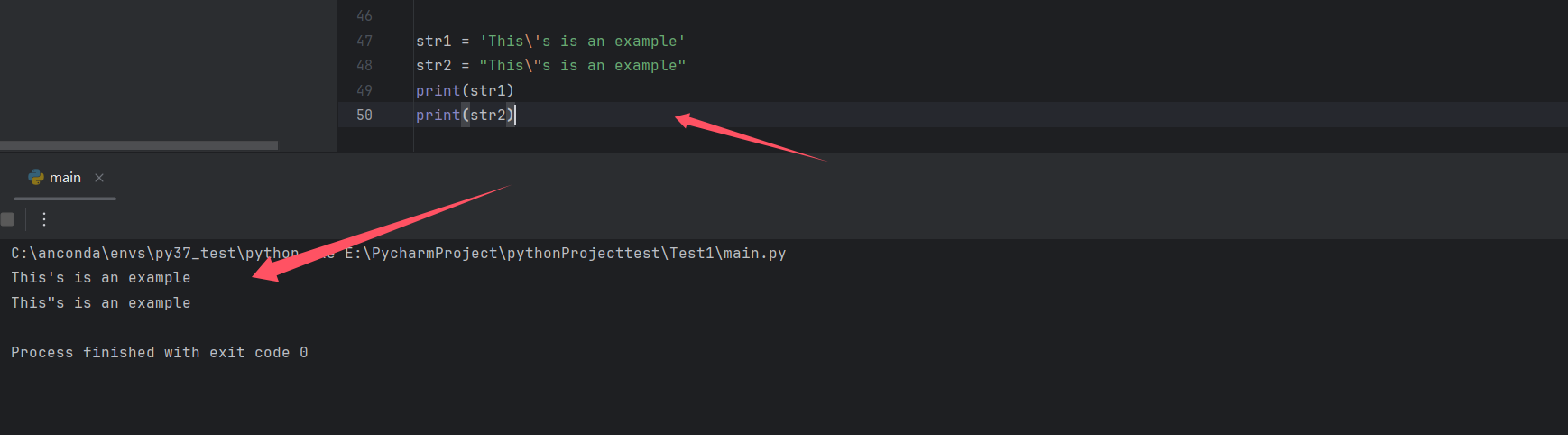
3、布尔型
布尔型通常用在判断上,用来判断正确与否。
bool1 = True
bool2 = False
bool3 = bool1 == bool2
print(bool1)
print(bool2)
print(bool3)
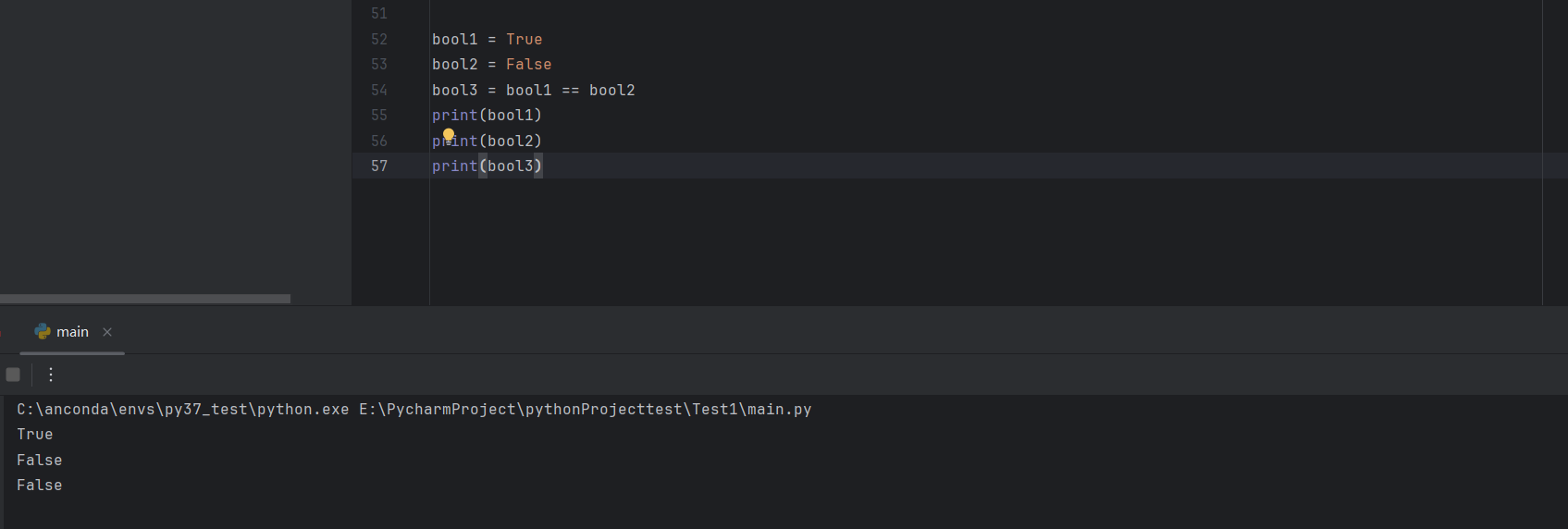
4、列表
存储不同元素的有序容器,它的作用就是该存数据的时候存数据,该处理数据的时候处理数据,最后该拿数据的时候拿数据。
list1 = [1,2,3,4,5,6,7,8]
list2 = [1,"1",True,1.1]
print(list1)
print(list2)
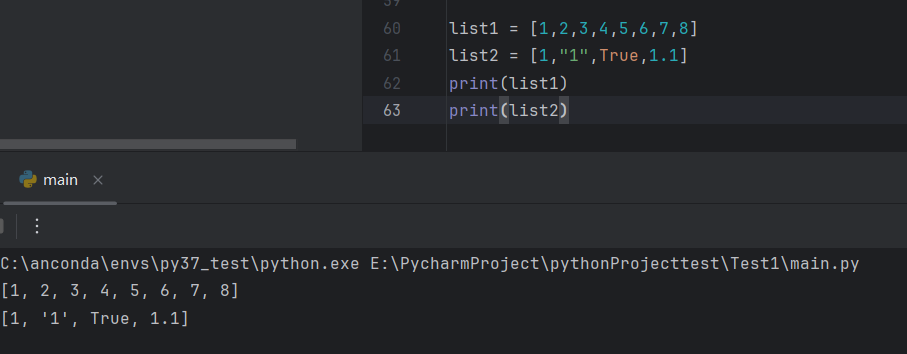
5、元组
与列表相同,也是存储元素的容器。但有一点不一样,即:它不能修改存储的元素。
tuple1 = (1,2,3,4)
tuple2 = (1,"1",True,1.1,5,6,7,8,9,0)
print(tuple1)
print(tuple2)
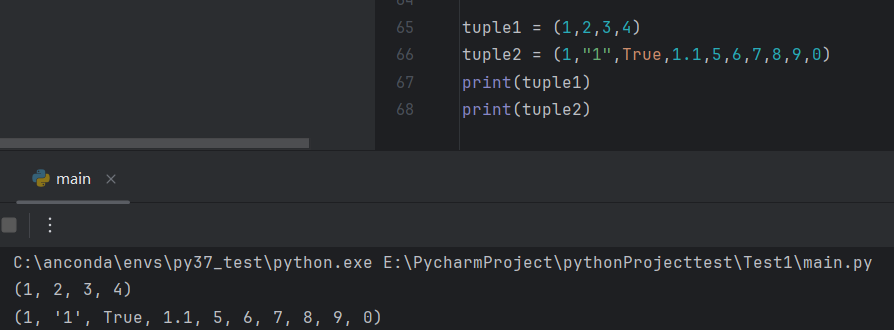
6、集合
与前二者类似,但也有区别。第一:它是无序的容器。第二:它存储的元素不能重复。
set1 = {1,2,3,4,5}
set2 = {True,False}
set3 = {1,"1",True,0,False,0,0}
print(set1)
print(set2)
print(set3)
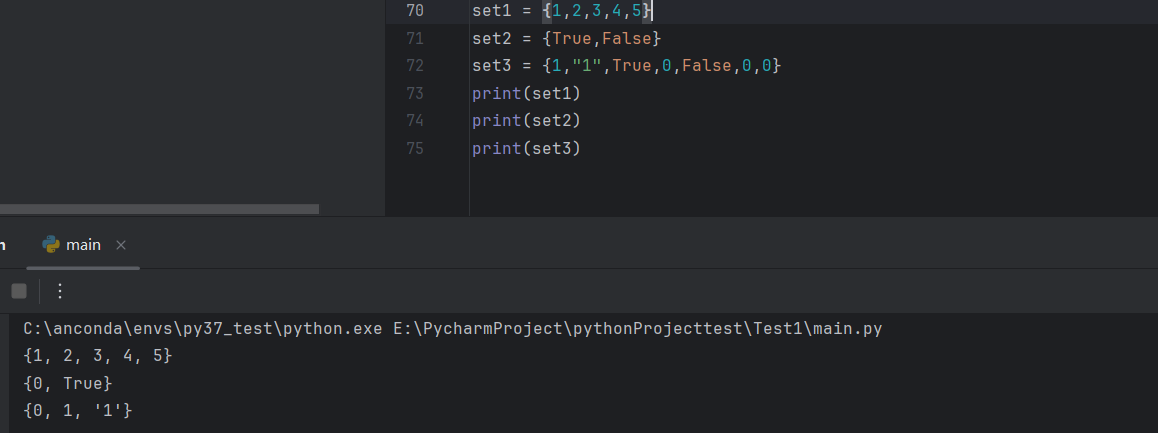
7、字典
这是Python中较为特殊的容器,首先,它是无序的。其次,它是键值对组成的。
dict1 = {0:1,1:0}
print(dict1)
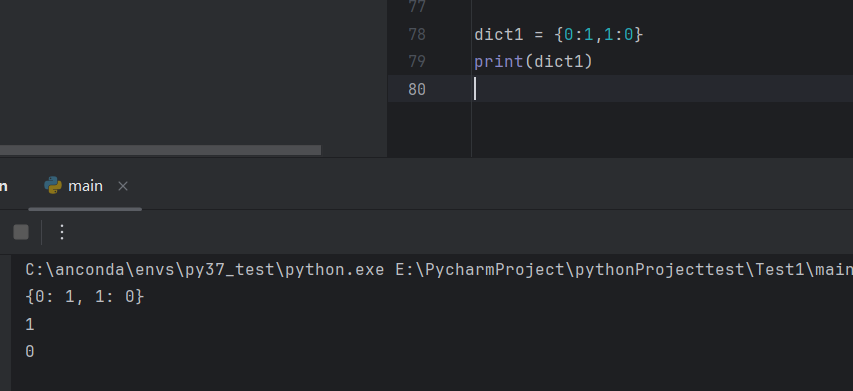
8、数据类型转换
Python不同数据类型之间是可以相互转换的。
-
转为整型
a = 1.1
b = int(a)
print(a)
print(b)
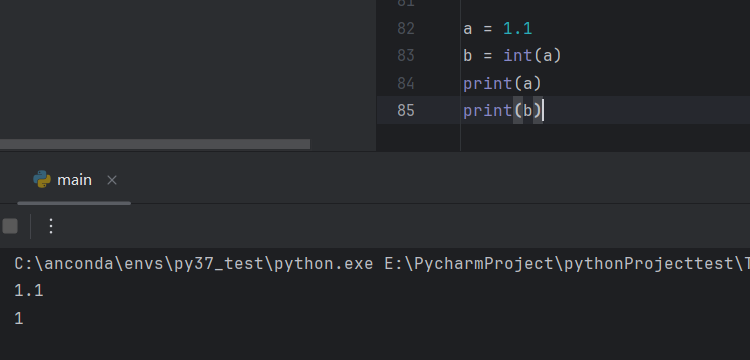
-
转为浮点型
a = 1
b = float(a)
print(a)
print(b)
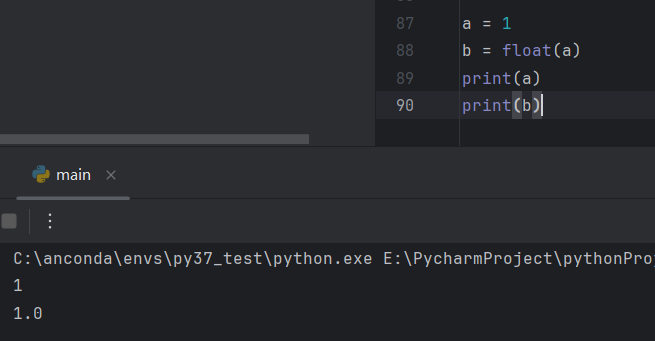
-
转为字符串
a = 12414
b = str(a)
print(a,type(a))
print(b,type(b))
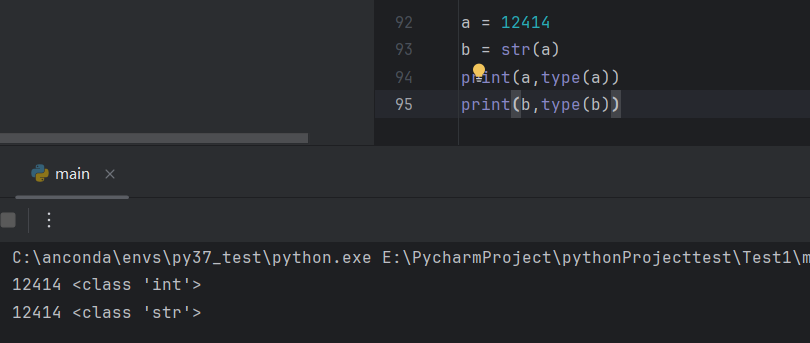
-
转为列表
tuple1 = (1,2,3)
list1 = list(tuple1)
print(list1)
print(tuple1)
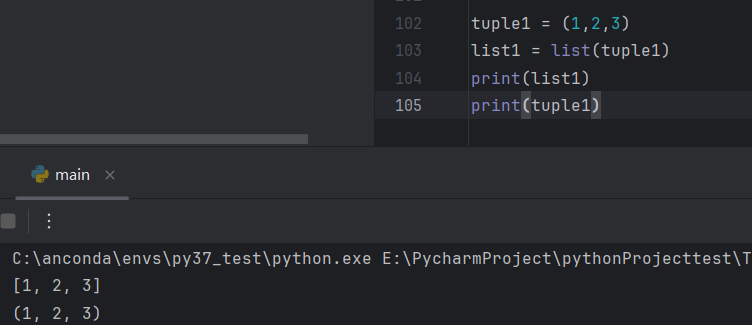
-
转为元组
list1 = [1,2,3]
tuple1 = tuple(list1)
print(list1)
print(tuple1)
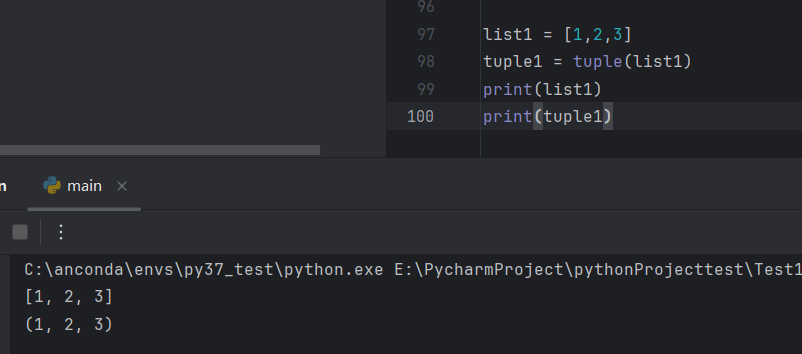