swift开发从入门到放弃
环境
1.在App Store下载Xcode
2.新建项目(可以先使用这个,简单入手快)
3.运行代码(快捷键 command + r)
swift入门
变量与常量
var
声明变量
let
声明常量
swift
var name = "東三城"
name = "西三城"
print(name) // 西三城
let pi = 3.14
var a = 1, b = 2
var 姓名 = "東三城" // 支持中文
var 😺 = "cat" // 支持符号
print(姓名, 😺) // 東三城 cat
类型安全和类型推断
swift
var age = 18
age = "刚满十八岁"

swift
// 同一种数据类型才能赋值
var age = 18.0
age = 18
print(age) // 18.0
swift
var a = "東"
var b = 18
var c = 18.0
var d = true
print(type(of: a)) // String
print(type(of: b)) // Int
print(type(of: c)) // Double
print(type(of: d)) // Bool
print函数
swift
// 分割
print(18, "a", 18.0, false, separator: "---") // 18---a---18.0---false
// 终止符
print(18, "a", 18.0, false, separator: "---", terminator: "\t")
print("bbb") // 18---a---18.0---false bbb
字符串
swift
// 声明空串
var emptyText = ""
var emptyText1 = String()
// 重复
var text = String(repeating: "東", count: 3)
print(text) // 東東東
// 多行文本
var text1 = """
東
三
城
"""
// 多行文本输出单行
var text1 = """
東\
三\
城
"""
// 输出双引号
var text1 = "\"東三城\""
print(text1) // "東三城"
var text2 = #""123東'三城""#
print(text2) // "123東'三城"
// 拼接
var name = "東三城"
var work = "写代码"
print(name + work) // 東三城写代码
print(name.appending(work)) // 東三城写代码
name += work
print(name) // 東三城写代码
print("\(name)工作是\(work)") // 東三城工作是写代码
// 判空
var name = "東三城"
print(name.isEmpty) // false
// 字符串长度
var name = "東三城"
print(name.count) // 3
// 取值
var name = "東三城"
print(name.description) // 東三城
print(name.debugDescription) // "東三城" 便于调试
print(name.hashValue) // 8034415610457256684
// 大写
var str = "This is a Str"
print(str.uppercased()) // THIS IS A STR
// 小写
print(str.lowercased()) // this is a str
// 相等 不等
print("ab" == "ab") // true
print("ab" != "ab") // false
// 前缀/后缀
print("productName".hasPrefix("product")) // true
print("productName".hasSuffix("Name")) // true
// 遍历
var str = "123abc東三城🦄"
for item in str{
print(item)
}
for index in str.indices{
print(index)
}
for index in str.indices{
print(str[index])
}
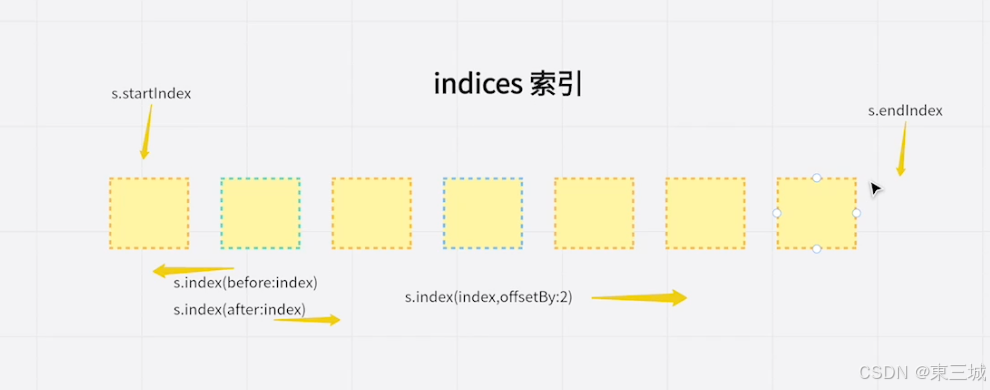
swift
// 索引
var str = "123abc東三城🦄"
print(str[str.startIndex]) // 1
print(str[str.index(before: str.endIndex)]) // 🦄
print(str[str.index(str.endIndex,offsetBy: -2)]) // 城
整数
swift
var a = 1
var b = 2.0
print(type(of: a),type(of: b)) // Int Double
var c:Float = 3.3
print(type(of: c)) // Float
var a = 0b10 // 2进制 2
var b = 0o7 // 8进制 7
var c = 0xf // 16进制 15
var d = 89_000_000 // 89000000
print(a,b,c,d)
// 判断
var num = Int("東") ?? 0
print(num) // 0
// 随机数
print(Int.random(in: 1...100))
print(Int.random(in: 1..<100))
// + - * / == != > <
// 相反数
var num = 18
num.negate()
print(num) // -18
// 绝对值
var num = -18
print(num.magnitude) // 18
print(abs(-18)) // 18
// 商 余数
var x = 100
let (q, r) = x.quotientAndRemainder(dividingBy: 9)
print(q,r) // 11 1
// 符号
var x = 100
print(x.signum()) // 1。 正数1 0为0 负数-1
// 常量
print(Int.zero) // 0
print(Int.max) // 9223372036854775807
print(Int.min) // -9223372036854775808
双精度
swift
var x = 18.0
var y:Double = 18.0
var z = Double(18)
// 随机数
print(Double.random(in: 10.0...100.0)) // 20.736881407455332
// 平方根
print(25.squareRoot()) // 5
// 四舍五入
print(25.1.rounded(.down)) // 25
// 常量
print(Double.pi) // 3.141592653589793
布尔
swift
var x = true
var y:Bool = false
if x { }
if x == true { }
// 取反
x.toggle()
// 随机
print(Bool.random())
运算符
swift
print( -a ) // 一元运算符
print( 1 + 3 ) // 两元运算符
print( 3 > 2 ? 10 : 20) // 三元运算符
// 赋值运算符 =
// 算数运算符 + - * / % += -= *= /= %=
// 比较运算符 > >= < <= != ==
// 空合运算符
var str:String? = nil
print(str ?? "")
// 区间运算
1...10
1..<9
// 逻辑运算符 && || !
数组
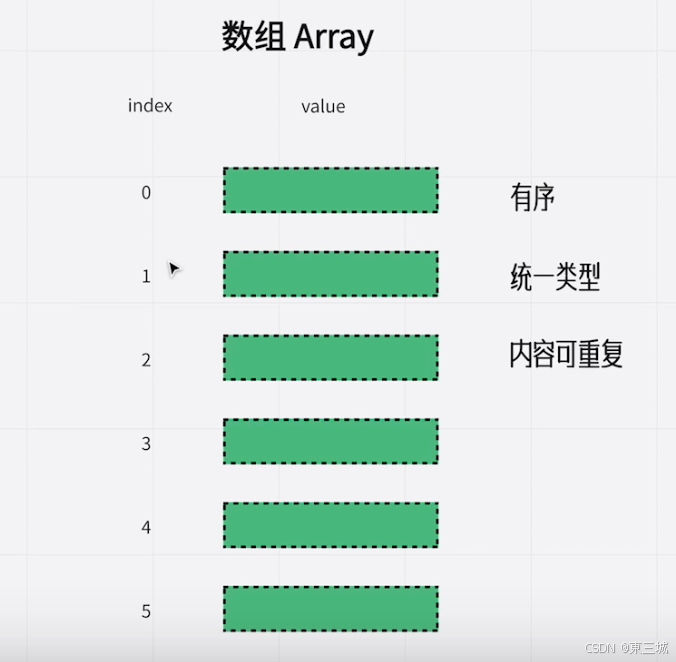
swift
var arr = [1, 2, 3]
var arr1:Array<Int> = [1, 2, 3]
print(arr1) // [1, 2, 3]
var arr2:[String] = ["a", "b", "c"]
var b1 = Array<Int>() // []
var b2 = Array<Int>([1, 2]) // [1, 2]
var b3 = Array(1...4) // [1, 2, 3, 4]
var c1 = Array(repeating: "*", count: 3) // ***
// 数组长度
c1.count
// 是否为空
c1.isEmpty
// 访问数组元素
print(b3[0]) // 1
print(b3[0...2]) // [1, 2, 3]
// 遍历
for item in b3 {
print(item)
}
// 带下标
for (index, value) in b3.enumerated(){
print(index, value)
}
var arr:Array<String> = ["张三", "李四", "王五"]
print(arr.first ?? "") // 张三
print(arr.last ?? "") // 王五
// 随机元素
print(arr.randomElement() ?? "")
// 添加
arr.append("赵六")
arr.append(contentsOf: ["mike", "anna"])
arr += ["🐶"]
// 插入
arr.insert("叶子", at: 0)
arr.insert(contentsOf: ["花", "太阳", "雨" ], at: 1)
print(arr) //["叶子", "花", "太阳", "雨", "张三", "李四", "王五", "赵六", "mike", "anna"]
// 替换
arr.replaceSubrange(0...1, with: ["a", "b", "c"])
print(arr) // ["a", "b", "c", "太阳", "雨", "张三", "李四", "王五", "赵六", "mike", "anna"]
// 删除
arr.remove(at: 3)
arr.removeFirst()
arr.removeLast()
arr.removeAll()
// 查找
var isA = arr.contains("a")
var isB = arr.first(where: {$0 == "b"})
// 遍历
t.forEach({ item in
print(item)
})
// 排序
var arr = [1, 2, 3]
arr.sort(by: >)
arr.sort() // 默认小于
// 乱序
var t = ["叶子", "花", "太阳", "雨", "张三", "李四", "王五", "赵六", "mike", "anna"]
t.shuffle()
print(t) // ["赵六", "张三", "花", "李四", "雨", "太阳", "anna", "叶子", "王五", "mike"]
// 反转
var t = ["叶子", "花", "太阳", "雨", "张三", "李四", "王五", "赵六", "mike", "anna"]
t.reverse()
print(t) // ["anna", "mike", "赵六", "王五", "李四", "张三", "雨", "太阳", "花", "叶子"]
// 分割
var line = "apple orange banana"
print(line.split(separator: " ")) // ["apple", "orange", "banana"]
var line1 = "apple orange+banana"
var l1 = line1.split(whereSeparator: {
$0 == "+" || $0 == " "
})
print(l1) // ["apple", "orange", "banana"]
// 连接
var line = ["apple", "orange", "banana"]
print(line.joined()) // appleorangebanana
print(line.joined(separator: "-")) // apple-orange-banana
集合set
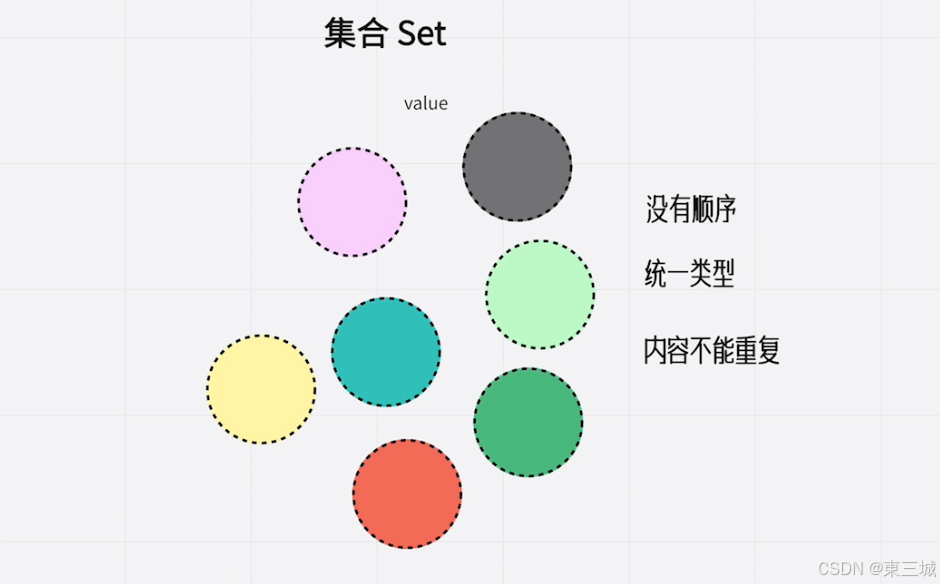
swift
var s:Set<Int> = [1, 2, 3, 4, 4]
var s1 = Set([1, 2, 3, 4])
print(s, s1) // [2, 3, 1, 4] [4, 1, 3, 2]
// 长度
print(s.count) // 4
// 是否空
print(s.isEmpty) // false
// 插入
var (c, d) = s.insert(6)
print(c, d) // true 6
s.update(with: 7)
print(s) // [2, 6, 1, 3, 7, 4]
// 删除
s.remove(7)
print(s) // [2, 3, 1, 4, 6]
s.removeAll()
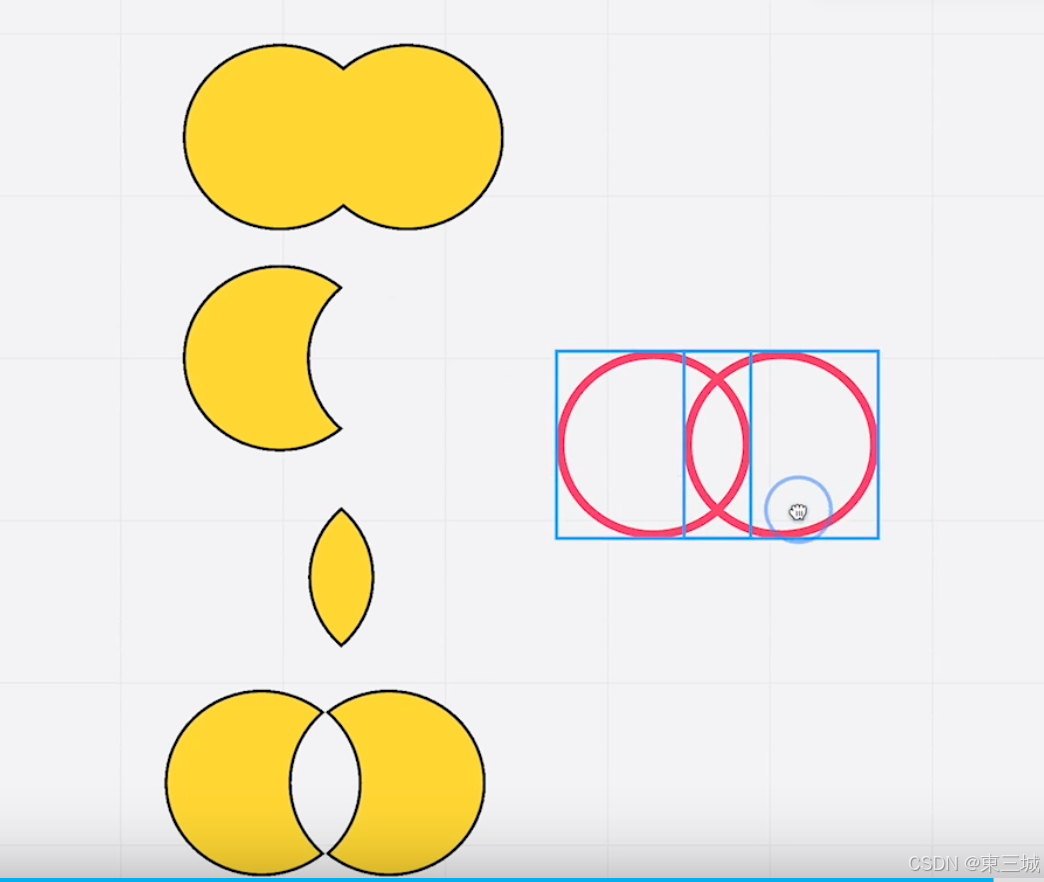
swift
var a = Set(["苹果","香蕉", "桔子", "柚子", "橙子"])
var b = Set(["桃子","香蕉", "桔子", "甜瓜", "西瓜"])
var c = Set(["桃子"])
// 相加
print(a.union(b)) // ["桔子", "柚子", "甜瓜", "香蕉", "桃子", "苹果", "橙子", "西瓜"]
// 相减
print(a.subtracting(b)) // ["柚子", "苹果", "橙子"]
// 相交
print(a.intersection(b)) // ["桔子", "香蕉"]
// 异或
print(a.symmetricDifference(b)) // ["桃子", "苹果", "柚子", "橙子", "甜瓜", "西瓜"]
// 是否子集
print(c.isSubset(of: a)) // false
// 是否超集
print(a.isSuperset(of: c)) // false
// 随机
print(a.randomElement() ?? "")
// a.map()
// a.sorted()
// a.shuffled()
// 遍历
for item in a {
print(item)
}
for (index,item) in a.enumerated(){
print(index,item)
}
a.forEach({
print($0)
})
字典
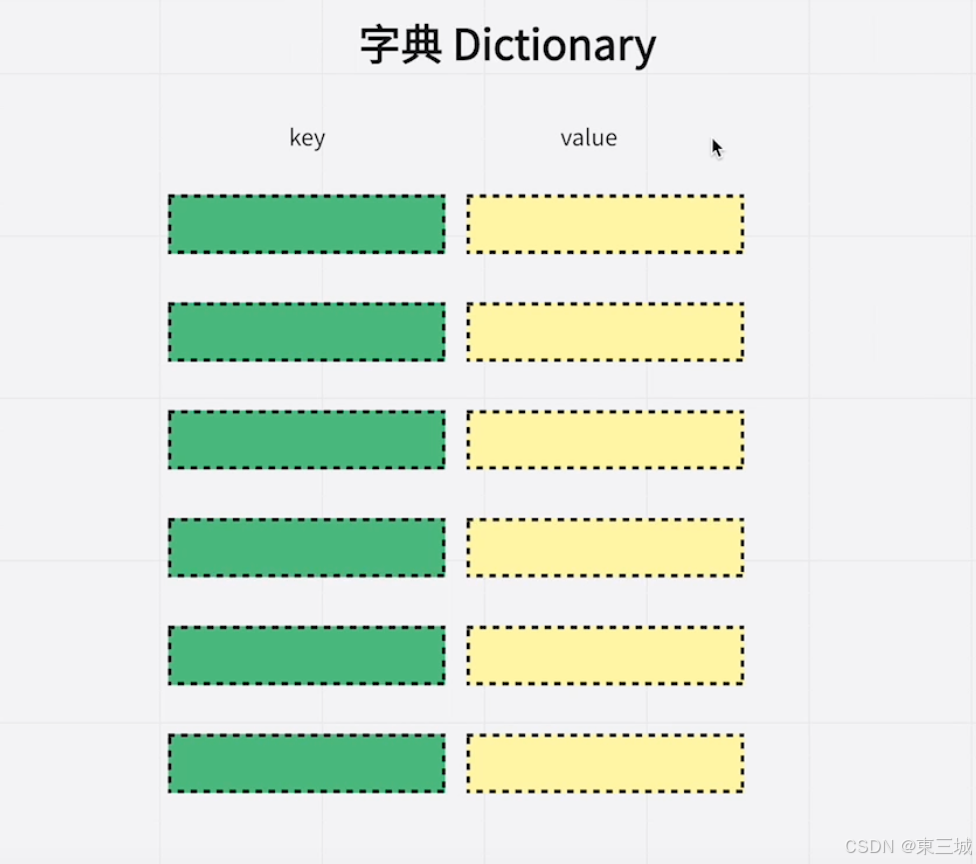
swift
var a:Dictionary<Int, String> = [:] // 空字典
var b:Dictionary<Int, String> = [1: "apple", 2: "orange"]
print(b) // [1: "apple", 2: "orange"]
// 查找
print(b[3,default: "无"]) // 无
// 长度
print(b.count) // 2
// 是否为空
print(b.isEmpty) // false
// 遍历
for (k,v) in b {
print(k, v)
}
for k in b.keys {
print(k)
}
b.forEach({
print($0, $1)
})
// 随机
print(b.randomElement() ?? "")
区间
swift
var a = 1...3 // ClosedRange<Int> 闭区间
var b = 1..<3 // Range<Int> 区间
var c = ...3 // PartialRangeThrough<Int> 半开区间
var d = ..<3 // PartialRangeUpTo<Int>
var e = 1... // PartialRangeFrom<Int>
var f = "a"..."z" // ClosedRange<String>
var g = 0.0...10.0 // ClosedRange<Double>
var h = stride(from: 10, to: 100, by: 2) // StrideTo<Int>
var r1:ClosedRange<String> = "a"..."z"
for i in a {
print(i)
}
// 包含
print(a.contains(11)) // false
// 是否为空
print(a.isEmpty)
// 上限
print(a.upperBound)
// 下限
print(a.lowerBound)
元祖
swift
var p = (10, 20)
var p1:(Int, Int) = (20, 30)
var position = (x:30, y:40)
print(p.0) // 10
print(position.x) // 30
// 解构
let (x, y) = position
print(x, y)
可选类型
swift
var i = Int("1")
print(i) // Optional(1)
print(i!) // 1
// (真正类型,nil)
var a:String? = "a" // 声明加?
print(type(of: a)) // Optional<String>
var b:Optional<Int> = 10
print(type(of: b)) // Optional<Int>
var c:Int? = 11
if let d = c{
if d > 10 {
print("a大于10")
}
}
var s:String? = "ProductA"
print(s?.hasPrefix("Product")) // Optional(true)
if let b = s?.hasPrefix("Product"){
print("有这个前缀", b, type(of: b)) // 有这个前缀 true Bool
}
print(s ?? "空") // ProductA
let number = Int("34")
print(number) // Optional(34)
print(number!) // 解包 34
循环语句
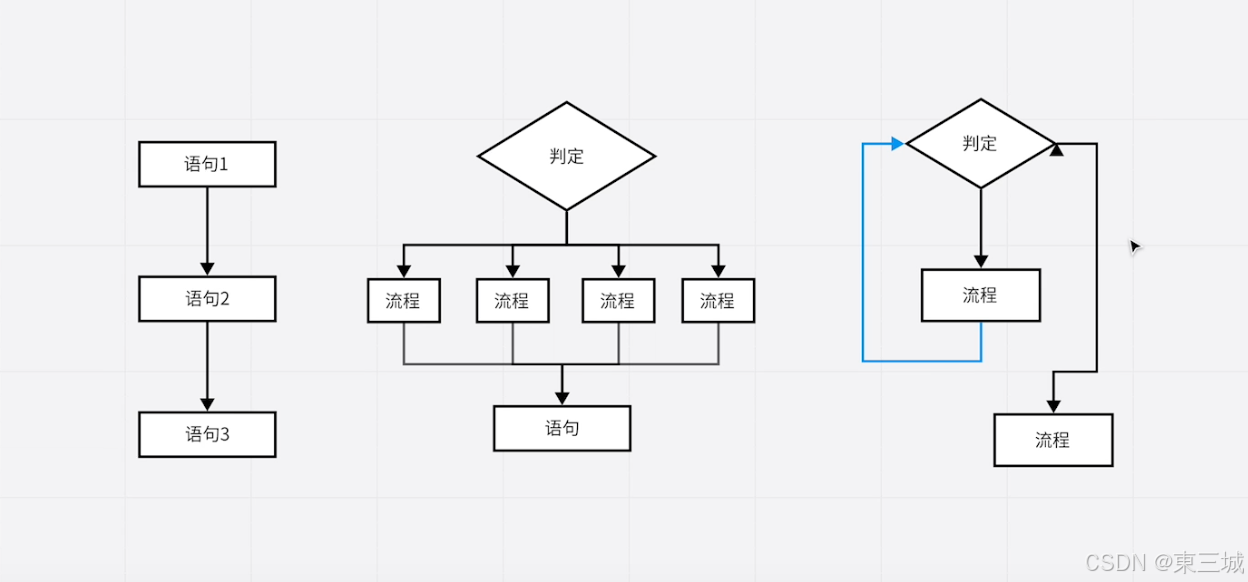
swift
for i in 1...5 {
print(i)
}
var index = 0
while index <= 10{
print(index)
index += 1
}
var index1 = 0
repeat {
print(index1)
index1 += 1
} while index1 <= 10
var i = 1
while i < 10 {
if i == 5 {
break // 跳出循环
}
i += 1
}
print(i) // 5
条件语句
swift
var score = 99
var check = score > 60 ? "合格" : "不合格"
print(check)
if(score > 60){
print("合格")
} else {
print("不合格")
}
if score > 90 {
print("优")
} else if score > 80 {
print("良")
} else if score > 70 {
print("中")
} else {
print("差")
}
print("请输入成绩:", terminator: "")
var input = readLine()!
if let score1 = Int(input) {
if score1 > 90 {
print("优")
} else if score1 > 80 {
print("良")
} else if score1 > 70 {
print("中")
} else {
print("差")
}
}
switch语句
swift
print("请输入成绩:", terminator: "")
var input = readLine()!
if let score1 = Int(input) {
switch score1 {
case 100:
print("牛")
break
case 90...99:
print("优")
case 81, 82, 83, 84, 85, 86, 87, 88, 89:
print("良")
case 60...80:
print("合格")
fallthrough
default:
print("不及格")
}
}
var score = 88
switch score {
case (let s) where s > 90:
print("牛")
case (let x) where x > 80:
print("行")
default:
print("不行")
}
函数
swift
// 定义
func hello(){
print("hello")
}
// 调用
hello()
// 参数
func hello1(name:String){
print("\(name),你好")
}
hello1(name: "東三城")
// 返回值
func hello2(name:String) -> String{
return "\(name),你好"
}
var hello2msg = hello2(name: "東三城")
print(hello2msg)
// 双参数双返回值
func max(a:Int,b:Int) -> (maxNum:Int, minNum:Int){
return a > b ? (a, b) : (b, a)
}
let (maxNum, minNum) = max(a: 3, b: 4)
print(maxNum, minNum) // 4 3
// 对外显示参数别名
func num1(oneNum a:Int, twoNum b:Int) -> Int {
return a + b
}
let x = num1(oneNum: 1, twoNum: 2)
print(x) // 3
// 不对外显示参数名
func num2(_ a:Int, _ b:Int) -> Int {
return a + b
}
print(num2(1, 2)) // 3
// 不确定参数个数
func num3 (_ num:Int... ) -> Int {
var s = 0
for i in num{
s += i
}
return s
}
print(num3(1, 56, 78, 2, 59)) // 196
// 参数默认值
func sum4(a:Int = 10) -> Int {
return a + 1
}
print(sum4()) // 11
枚举类型
swift
enum WeekDay {
case Mon
case The
case Wed
case Thu
case Fri
case Sat
case Sun
}
let a = WeekDay.Mon
print(a) // Mon
var b:WeekDay = WeekDay.The
enum Direction:CaseIterable { // 可遍历的协议
case west, east, south, north
}
var c = Direction.east
c = .north // 可省略
print(c)
// 遍历
Direction.allCases.forEach({
print($0, terminator: "\t")
})
// 匹配枚举
switch c{
case .east:
print("东")
case .north:
print("北")
case .south:
print("南")
case .west:
print("西")
}
enum PlayerState{
case idle
case walk(speed:Int)
case run(speed:Int)
case fly(speed:Int, height:Int)
case die
}
let a1 = PlayerState.fly(speed: 800, height: 1000) // 关联值
switch a1{
case .idle:
print("玩家正在待机")
case .walk(let speed):
print("玩家正在以速度\(speed)行走")
case .run(let speed):
print("玩家正在以速度\(speed)奔跑")
case .fly(let speed, let height):
print("玩家在\(height)高空以速度\(speed)飞行")
case .die:
print("玩家已死 ")
}
// 原始值
enum Gender:Int {
case man = 10
case women
case unknow
}
print(Gender.man.rawValue) // 0 +10
print(Gender.women.rawValue) // 1 + 10
print(Gender.unknow.rawValue) // 2 + 10
// 描述(description是每一种数据类型都有的,重新定义了一下)
enum Weather {
case sunny,cloudy,rainy,sonwy,windy
var description:String {
switch self {
case .sunny:
return "晴天"
case .cloudy:
return "多云"
case .rainy:
return "雨天"
case .sonwy:
return "下雪"
case .windy:
return "大风"
}
}
}
print(Weather.cloudy.description)
enum Shape {
case retangle(width: Double, height: Double)
case circle(redius: Double)
case triangle(side1: Double, side2: Double, side3: Double)
var description: Double {
switch self {
case .circle(let radius):
return Double.pi * radius * radius
case .retangle(let width,let height):
return width * height
case .triangle(let side1,let side2,let side3):
return (side1 * side2 * side3) / (2 * sqrt(side1 * side2 * side3))
}
}
}
print(Shape.circle(redius: 10).description)
闭包
swift
// 函数 ()->Void
func hello(){
print("hello")
}
hello()
// 匿名函数
let hi = {
print("hi")
}
hi()
let hi1 = { (name: String) in
print("你好\(name)")
}
hi1("東三城")
func sum(a:Int, b:Int) -> Int {
a + b
}
print(sum(a: 10, b: 8))
let s = {(a:Int, b:Int) -> Int in
a+b
}
print(s(8, 10))
func sayHi(action:() -> Void ){
action()
print("東三城")
}
let hi2 = {
print("你好")
}
sayHi(action: hi2)
// 尾随闭包
sayHi {
print("hello")
}
// 函数的参数是另一个函数
func travel(action:(String) -> Void) {
print("我现在出发")
action("洛阳")
print("我已经到了")
}
travel{(place: String) in
print("我坐火车去\(place)")
}
// 有参数有返回值
func travel1(action:(String, Int) -> String) {
print("我现在出发")
print(action("洛阳",120))
print("我已经到了")
}
travel1{(place: String,speed: Int) -> String in
return "我坐火车已\(speed)km/h去\(place)"
}
travel1{
"我坐火车已\($1)km/h去\($0)"
}
func travel2() -> (String) -> Void{
return {(place: String) in
print("我想去\(place)")
}
}
let r = travel2()
r("郑州")
数组方法
swift
let numbers = [1, 2, 3, 4, 5, 6, 7, 8 ,9, 10]
// map 产生新的数组,每一个元素要经过map加工
let n1 = numbers.map{
$0 * $0
}
// filter 产生新的数组,每一个元素要经过filter的判断过滤,true返回,false抛弃
let n2 = numbers.filter{
$0 > 5
}
let n3 = numbers.reduce(0, {
$0 + $1
})
print(n1, n2, n3)
结构体
swift
struct Student {
static let tableName = "学生表"
var name = ""
var age:Int? = nil
var chinese:Int? = nil
var math:Int? = nil
var total:Int? = nil
mutating func calc(){
self.total = self.chinese! + self.math!
}
}
var dong = Student(name: "東三城", age: 18, chinese: 99, math: 99) // 实例化
dong.calc()
print(dong)
如有不足,请多指教,
未完待续,持续更新!
大家一起进步!