example1
bash
import numpy as np
from skimage import data
from skimage.transform import rescale
import matplotlib.pyplot as plt
# Load a sample image
image = data.chelsea()
# Notice that you shouldn't use the multichannel parameter
# Rescale the image to 50% of its original size
image_rescaled = rescale(image, 0.5, anti_aliasing=True, channel_axis=2)
# Display the original and rescaled images
fig, (ax1, ax2) = plt.subplots(ncols=2, figsize=(10, 5))
ax1.imshow(image)
ax1.set_title('Original image')
ax2.imshow(image_rescaled)
ax2.set_title('Rescaled image (50%)')
plt.tight_layout()
plt.show()
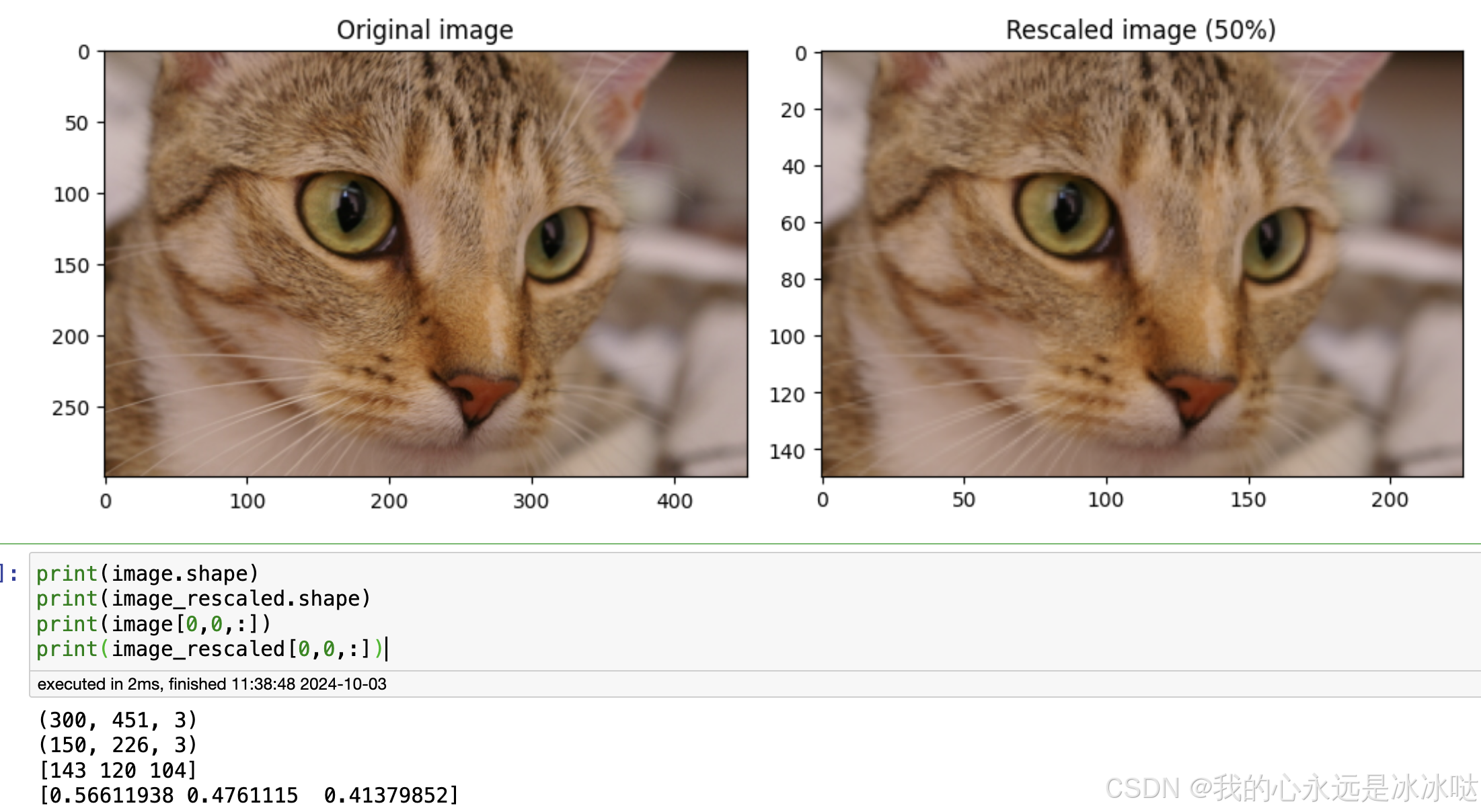
example2
bash
import numpy as np
from skimage import data, img_as_ubyte
from skimage.transform import rescale
import matplotlib.pyplot as plt
# Load a sample image
image = data.chelsea()
# Rescale the image to 50% of its original size
image_rescaled_float = rescale(image, 0.5, anti_aliasing=True, channel_axis=2)
# Convert the rescaled image to 8-bit unsigned integer
image_rescaled = img_as_ubyte(image_rescaled_float)
# Display the original and rescaled images
fig, (ax1, ax2) = plt.subplots(ncols=2, figsize=(10, 5))
ax1.imshow(image)
ax1.set_title('Original image')
ax2.imshow(image_rescaled)
ax2.set_title('Rescaled image (50%)')
plt.tight_layout()
plt.show()
# Print the data types to verify
print("Original image dtype:", image.dtype)
print("Rescaled image dtype:", image_rescaled.dtype)
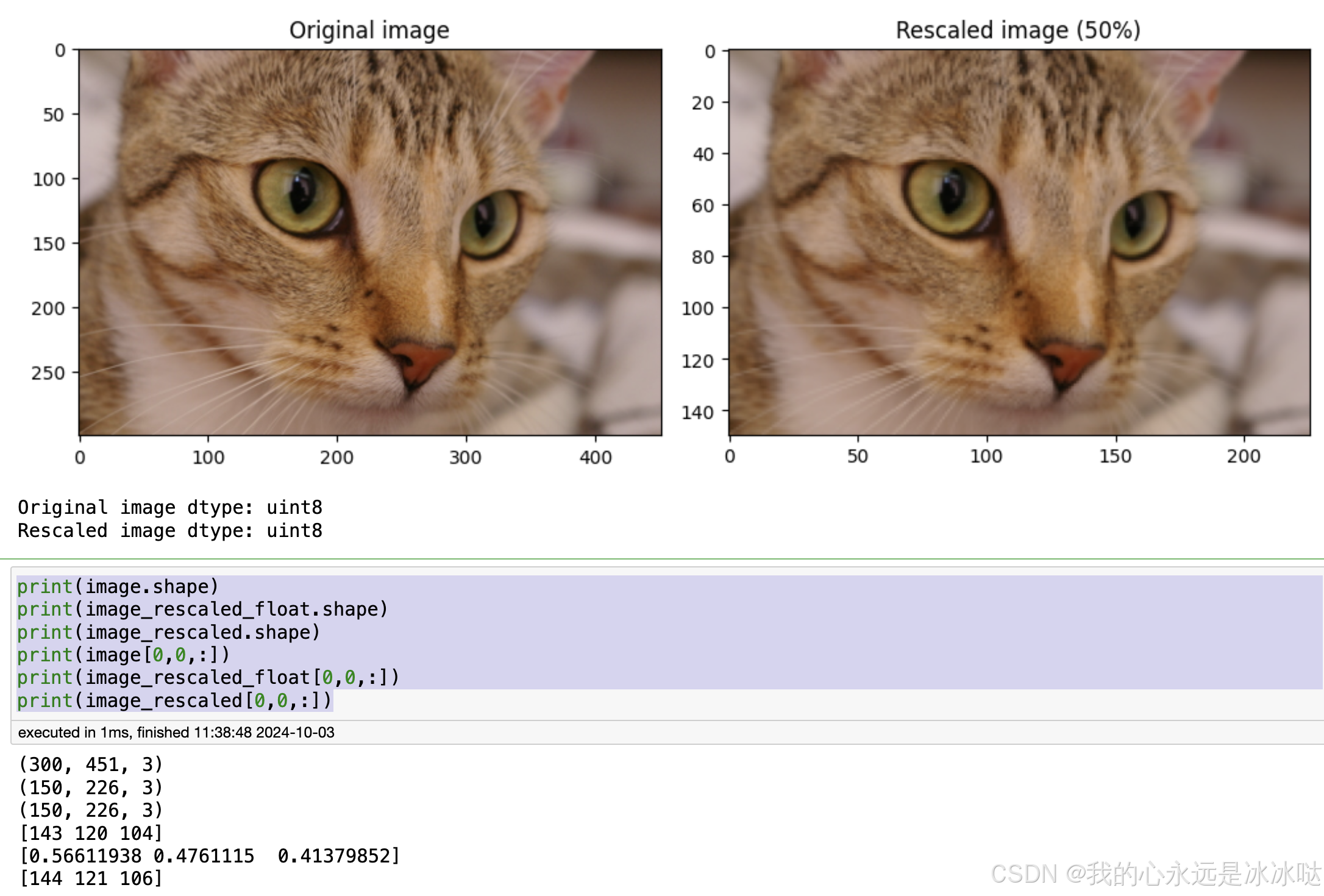