一、基础接口
java
public interface BaseMapper<T> {
/**
* 插入一条记录
* @param entity 实体对象
*/
int insert(T entity);
/**
* 根据 ID 删除
* @param id 主键ID
*/
int deleteById(Serializable id);
/**
* 根据 columnMap 条件,删除记录
* @param columnMap 表字段 map 对象
*/
int deleteByMap(@Param(Constants.COLUMN_MAP) Map<String, Object> columnMap);
/**
* 根据 entity 条件,删除记录
* @param queryWrapper 实体对象封装操作类(可以为 null)
*/
int delete(@Param(Constants.WRAPPER) Wrapper<T> queryWrapper);
/**
* 删除(根据ID 批量删除)
* @param idList 主键ID列表(不能为 null 以及 empty)
*/
int deleteBatchIds(@Param(Constants.COLLECTION) Collection<? extends Serializable> idList);
/**
* 根据 ID 修改
* @param entity 实体对象
*/
int updateById(@Param(Constants.ENTITY) T entity);
/**
* 根据 whereEntity 条件,更新记录
* @param entity 实体对象 (set 条件值,不能为 null)
* @param updateWrapper 实体对象封装操作类(可以为 null,里面的 entity 用于生成 where 语句)
*/
int update(@Param(Constants.ENTITY) T entity, @Param(Constants.WRAPPER) Wrapper<T> updateWrapper);
/**
* 根据 ID 查询
* @param id 主键ID
*/
T selectById(Serializable id);
/**
* 查询(根据ID 批量查询)
* @param idList 主键ID列表(不能为 null 以及 empty)
*/
List<T> selectBatchIds(@Param(Constants.COLLECTION) Collection<? extends Serializable> idList);
/**
* 查询(根据 columnMap 条件)
* @param columnMap 表字段 map 对象
*/
List<T> selectByMap(@Param(Constants.COLUMN_MAP) Map<String, Object> columnMap);
/**
* 根据 entity 条件,查询一条记录
* @param queryWrapper 实体对象
*/
T selectOne(@Param(Constants.WRAPPER) Wrapper<T> queryWrapper);
/**
* 根据 Wrapper 条件,查询总记录数
* @param queryWrapper 实体对象
*/
Integer selectCount(@Param(Constants.WRAPPER) Wrapper<T> queryWrapper);
/**
* 根据 entity 条件,查询全部记录
* @param queryWrapper 实体对象封装操作类(可以为 null)
*/
List<T> selectList(@Param(Constants.WRAPPER) Wrapper<T> queryWrapper);
/**
* 根据 Wrapper 条件,查询全部记录
* @param queryWrapper 实体对象封装操作类(可以为 null)
*/
List<Map<String, Object>> selectMaps(@Param(Constants.WRAPPER) Wrapper<T> queryWrapper);
/**
* 根据 Wrapper 条件,查询全部记录
* 注意: 只返回第一个字段的值
* @param queryWrapper 实体对象封装操作类(可以为 null)
*/
List<Object> selectObjs(@Param(Constants.WRAPPER) Wrapper<T> queryWrapper);
/**
* 根据 entity 条件,查询全部记录(并翻页)
* @param page 分页查询条件(可以为 RowBounds.DEFAULT)
* @param queryWrapper 实体对象封装操作类(可以为 null)
*/
IPage<T> selectPage(IPage<T> page, @Param(Constants.WRAPPER) Wrapper<T> queryWrapper);
/**
* 根据 Wrapper 条件,查询全部记录(并翻页)
* @param page 分页查询条件
* @param queryWrapper 实体对象封装操作类
*/
IPage<Map<String, Object>> selectMapsPage(IPage<T> page, @Param(Constants.WRAPPER) Wrapper<T> queryWrapper);
}
二、条件构造器Wrapper及语法
Wrapper: 条件构造抽象类,最顶端父类
AbstractWrapper :用于查询条件封装,生成 sql 的 where 条件
QueryWrapper :Entity 对象封装操作类,不是用 lambda 语法
UpdateWrapper: Update 条件封装,用于 Entity 对象更新操作
AbstractLambdaWrapper: Lambda 语法使用 Wrapper 统一处理解析 lambda 获取 column
LambdaQueryWrapper: 用于 Lambda 语法使用的查询 Wrapper
**LambdaUpdateWrapper:**Lambda 更新封装 Wrapper
三、插件的使用
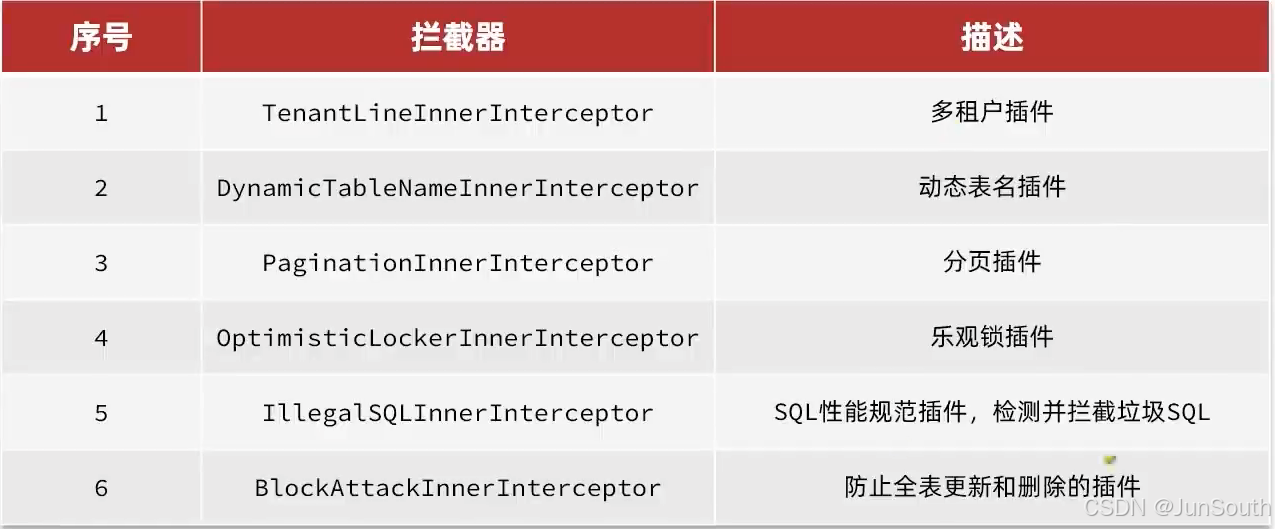
四、Dao层的组装及规范
1.Entity
java
import com.baomidou.mybatisplus.annotation.IdType;
import com.baomidou.mybatisplus.annotation.TableField;
import com.baomidou.mybatisplus.annotation.TableId;
import com.baomidou.mybatisplus.annotation.TableName;
import lombok.Builder;
import lombok.AllArgsConstructor;
import lombok.NoArgsConstructor;
import lombok.Data;
import java.io.Serializable;
import java.util.Date;
import java.math.BigDecimal;
@Builder
@Data
@AllArgsConstructor
@NoArgsConstructor
@TableName("customs")
public class Customs implements Serializable {
private static final long serialVersionUID=1L;
/**
* id
*/
@TableId(value = "id", type = IdType.AUTO)
private String id;
/**
* 贸易方式
*/
@TableField(value = "trade_way")
private String tradeWay;
/**
* 报关发票号
*/
@TableField(value = "report_invoice_no")
private String reportInvoiceNo;
/**
* 唛头
*/
@TableField(value = "mark_head")
private String markHead;
/**
* 发票金额
*/
@TableField(value = "invoice_amount")
private BigDecimal invoiceAmount;
/**
* 状态(10草稿、20审批中、21审批通过、22驳回、30中止、40废弃)
*/
@TableField(value = "status")
private String status;
/**
* 是否删除 0 否 1 是
*/
@TableField("del_flag")
private Integer delFlag;
}
2.Dto
java
import com.fasterxml.jackson.annotation.JsonFormat;
import io.swagger.annotations.ApiModelProperty;
import lombok.Builder;
import lombok.AllArgsConstructor;
import lombok.NoArgsConstructor;
import lombok.Data;
import javax.validation.constraints.NotBlank;
import javax.validation.constraints.NotNull;
import java.util.Date;
import java.math.BigDecimal;
import java.util.List;
@Builder
@Data
@AllArgsConstructor
@NoArgsConstructor
public class CustomsDto {
/**
* id
*/
@ApiModelProperty(value = "ID",example = "")
private String id;
/**
* 清关单号
*/
@ApiModelProperty(value = "清关单号",example = "")
private String clearCustomsNo;
/**
* 报关单ID
*/
@ApiModelProperty(value = "报关单ID",example = "")
private String reportCustomsId;
/**
* 报关流水号
*/
@ApiModelProperty(value = "报关流水号",example = "")
@NotBlank(message = "报关流水号 不能为空", groups = { AddGroup.class, EditGroup.class })
private String reportCustomsPipeNo;
/**
* 贸易方式
*/
@ApiModelProperty(value = "贸易方式",example = "")
private String tradeWay;
/**
* 币种
*/
@ApiModelProperty(value = "币种",example = "")
@NotBlank(message = "币种不能为空", groups = { AddGroup.class, EditGroup.class })
private String currency;
/**
* 状态(10草稿、20审批中、21审批通过、22驳回、30中止、40废弃)
*/
@ApiModelProperty(value = "状态(10草稿、20审批中、21审批通过、22驳回、30中止、40废弃)",example = "")
private String status;
}
3. Mapper
java
import com.dao.entity.customs.clear.Customs;
import com.dto.customs.clear.CustomsDto;
import com.adp.commons.utils.mapper.BaseMapperPlus;
public interface CustomsMapper extends BaseMapperPlus<CustomsMapper, Customs, CustomsDto> {
}
4.Dao
java
import com.commons.group.beans.AuxResponse;
import com.commons.utils.AuxBeanUtil;
import com.commons.utils.AuxStringUtils;
import com.commons.utils.page.QueryBody;
import com.constants.CommonConst;
import com.constants.cumtoms.CumtomsConst;
import com.dao.entity.customs.clear.Customs;
import com.dao.mapper.customs.clear.CustomsMapper;
import com.dto.customs.clear.CustomsDto;
import com.enums.customs.CustomsNumberEnum;
import com.enums.customs.CustomsStatusEnum;
import com.query.customs.clear.CustomsParams;
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper;
import com.baomidou.mybatisplus.core.toolkit.Wrappers;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
@Slf4j
@Component
public class CustomsDao extends ServiceImpl<CustomsMapper,Customs> {
@Autowired
private CustomsMapper customsMapper;
public String produceSerialNumber(){
LambdaQueryWrapper<Customs> lqw = Wrappers.lambdaQuery();
lqw.eq(Customs::getDelFlag, 1);
lqw.orderByAsc(Customs::getCreateTime);
CustomsDto clearCustomsDto =customsMapper.selectVoOne(lqw);
if(clearCustomsDto==null){
Customs clearCustoms = new Customs();
clearCustoms.setDelFlag(1);
customsMapper.insert(clearCustoms);
return reportCustomsNo;
}
return sequenceService.generate(CustomsNumberEnum.REPORT_CUSTOMS_CLEAR_NO.code).getData();
}
/**
* 通过ID 获取详情
*/
public CustomsDto getInfoById(String id){
CustomsDto dto = customsMapper.selectVoById(id);
if(dto==null){
return null;
}
ShippingCustomsClearAnnexsDto annexsDto = new ShippingCustomsClearAnnexsDto();
annexsDto.setBusinessType(CommonConst.NUMBER_STR.TEN);
annexsDto.setBusinessId(id);
return dto;
}
/**
* 分页查询
*/
public Page<CustomsDto> queryPageList(QueryBody<CustomsParams> queryBody){
LambdaQueryWrapper<Customs> lqw = buildQueryWrapper(queryBody.getParams());
lqw.orderByDesc(Customs::getCreateTime);
return customsMapper.selectVoPage(queryBody.getPageQuery().build(), lqw);
}
/**
* 列表查询
*/
public List<CustomsDto> queryList(CustomsParams params){
LambdaQueryWrapper<Customs> lqw = buildQueryWrapper(params);
lqw.orderByDesc(Customs::getCreateTime);
List<CustomsDto> queryRet = customsMapper.selectVoList(lqw);
Map<String,String> map = new HashMap<>();
List<CustomsDto> ret = new ArrayList<>();
for(CustomsDto clearCustomsDto : queryRet){
String clearInvoiceNo = clearCustomsDto.getClearInvoiceNo();
if(!map.containsKey(clearInvoiceNo)){
ret.add(clearCustomsDto);
}
map.put(clearInvoiceNo,clearInvoiceNo);
}
return ret;
}
/**
* 新增数据
*/
public AuxResponse<String> add(CustomsDto dto){
Customs add = AuxBeanUtil.copy(dto, Customs.class);
String clearCustomsNo = add.getClearCustomsNo();
if(clearCustomsNo==null||clearCustomsNo.isEmpty()){
clearCustomsNo = sequenceService.generate(CustomsNumberEnum.REPORT_CUSTOMS_CLEAR_NO.code).getData();
}
// 判断是否已保存
LambdaQueryWrapper<Customs> lqw = Wrappers.lambdaQuery();
lqw.eq(Customs::getClearCustomsNo, clearCustomsNo);
lqw.last(CommonConst.SQL_LIMIT);
Customs getOneEntity = customsMapper.selectOne(lqw);
if(getOneEntity!=null){
return AuxResponse.FAILED(CumtomsConst.THIS_NO_HAVE_DATA);
}
add.setClearCustomsNo(clearCustomsNo);
boolean flag = customsMapper.insert(add) > 0;
String retId = add.getId();
if (flag) {
return AuxResponse.SUCCESS(retId);
}
return AuxResponse.FAILED(CommonConst.ERROR);
}
/**
* 修改数据
*/
public AuxResponse<String> update(CustomsDto dto){
String id = dto.getId();
Customs ret = customsMapper.selectById(id);
if(ret==null){
return AuxResponse.FAILED(CumtomsConst.NOT_THIS_DATA);
}
String status = ret.getStatus();
if(CustomsStatusEnum.DRAFT.code.equals(status)||CustomsStatusEnum.REJECTED.code.equals(status)||CustomsStatusEnum.APPROVAL_ING.code.equals(status)){
try {
if(CustomsStatusEnum.APPROVAL_ING.code.equals(status)){
Customs update = new Customs();
update.setId(dto.getId());
update.setAmountIsEqual(dto.getAmountIsEqual());
customsMapper.updateById(update);
}else {
Customs update = AuxBeanUtil.copy(dto, Customs.class);
customsMapper.updateById(update);
}
}catch (Exception e){
log.error("ShippingClearCustomsDao---update{}",e.getMessage());
return AuxResponse.FAILED(e.getMessage());
}
return AuxResponse.SUCCESS(id);
}
return AuxResponse.FAILED(CumtomsConst.ONLY_OPERA_DRAFT_DATA);
}
/**
* 取消
*/
public String cancel(String id){
try {
Customs ret = customsMapper.selectById(id);
if(ret==null){
return CumtomsConst.NOT_THIS_DATA;
}
String status = ret.getStatus();
if(CustomsStatusEnum.DRAFT.code.equals(status)||CustomsStatusEnum.REJECTED.code.equals(status)){
ret = new Customs();
ret.setId(id);
ret.setStatus(CustomsStatusEnum.DISUSE.code);
customsMapper.updateById(ret);
return CommonConst.SUCCESS;
}
return CumtomsConst.ONLY_CANEL_DATA;
}catch (Exception e){
log.error("ShippingClearCustomsDao---cancel{}",e.getMessage());
}
return CommonConst.ERROR;
}
private LambdaQueryWrapper<Customs> buildQueryWrapper(CustomsParams params) {
LambdaQueryWrapper<Customs> lqw = Wrappers.lambdaQuery();
lqw.like(AuxStringUtils.isNotBlank(params.getClearCustomsNo()), Customs::getClearCustomsNo, params.getClearCustomsNo());
lqw.eq(AuxStringUtils.isNotBlank(params.getReportCustomsPipeNo()), Customs::getReportCustomsPipeNo, params.getReportCustomsPipeNo());
lqw.eq(AuxStringUtils.isNotBlank(params.getReportInvoiceNo()), Customs::getReportInvoiceNo, params.getReportInvoiceNo());
lqw.like(AuxStringUtils.isNotBlank(params.getClearInvoiceNo()), Customs::getClearInvoiceNo, params.getClearInvoiceNo());
lqw.like(AuxStringUtils.isNotBlank(params.getUnitName()), Customs::getUnitName, params.getUnitName());
lqw.like(AuxStringUtils.isNotBlank(params.getCustomName()), Customs::getCustomName, params.getCustomName());
lqw.eq(AuxStringUtils.isNotBlank(params.getStatus()), Customs::getStatus, params.getStatus());
lqw.ne(AuxStringUtils.isNotBlank(params.getFromStatus()), Customs::getStatus, params.getFromStatus());
return lqw;
}
}
五、常用注解
XML
<!--mybatis-plus-->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.0.5</version>
</dependency>