目录
[1. Instant 类](#1. Instant 类)
[1)plus(Duration duration)](#1)plus(Duration duration))
[2)minus(Duration duration)](#2)minus(Duration duration))
[4)ofEpochMilli(long epochMilli)](#4)ofEpochMilli(long epochMilli))
[2. LocalDate 类](#2. LocalDate 类)
[2)LocalDate.of(int year, int month, int dayOfMonth)](#2)LocalDate.of(int year, int month, int dayOfMonth))
[1)plusDays(long daysToAdd)](#1)plusDays(long daysToAdd))
[2)minusDays(long daysToSubtract)](#2)minusDays(long daysToSubtract))
[3)getYear(), getMonthValue(), getDayOfMonth()](#3)getYear(), getMonthValue(), getDayOfMonth())
[4)with(TemporalAdjuster adjuster)](#4)with(TemporalAdjuster adjuster))
[3. LocalTime 类](#3. LocalTime 类)
[2)LocalTime.of(int hour, int minute, int second, int nanoOfSecond)](#2)LocalTime.of(int hour, int minute, int second, int nanoOfSecond))
[1)plusHours(long hoursToAdd)](#1)plusHours(long hoursToAdd))
[2)minusMinutes(long minutesToSubtract)](#2)minusMinutes(long minutesToSubtract))
[3)getHour(), getMinute(), getSecond(), getNano()](#3)getHour(), getMinute(), getSecond(), getNano())
[4. LocalDateTime 类](#4. LocalDateTime 类)
[2)LocalDateTime.of(LocalDate date, LocalTime time)](#2)LocalDateTime.of(LocalDate date, LocalTime time))
[1)plusDays(long daysToAdd)](#1)plusDays(long daysToAdd))
[2)minusHours(long hoursToSubtract)](#2)minusHours(long hoursToSubtract))
[5. Period 类](#5. Period 类)
[1)Period.between(LocalDate startDate, LocalDate endDate)](#1)Period.between(LocalDate startDate, LocalDate endDate))
[2)getYears(), getMonths(), getDays()](#2)getYears(), getMonths(), getDays())
[3)plusYears(long yearsToAdd)](#3)plusYears(long yearsToAdd))
[4)minusMonths(long monthsToSubtract)](#4)minusMonths(long monthsToSubtract))
[6. Duration 类](#6. Duration 类)
[1)Duration.between(LocalTime startTime, LocalTime endTime)](#1)Duration.between(LocalTime startTime, LocalTime endTime))
[2)Duration.ofSeconds(long seconds)](#2)Duration.ofSeconds(long seconds))
[1)getSeconds(), getNano()](#1)getSeconds(), getNano())
[2)plusSeconds(long secondsToAdd)](#2)plusSeconds(long secondsToAdd))
[3)minusNanos(long nanosToSubtract)](#3)minusNanos(long nanosToSubtract))
1. Instant 类
Instant
表示时间线上的一个瞬间点,通常用于记录应用程序中的时间戳。
构造方法
1)Instant.now()
:获取当前时间戳。
java
Instant now = Instant.now();
常用方法
1)plus(Duration duration)
-
返回一个新的
Instant
对象,表示在当前时间基础上加上指定的持续时间。javaInstant now = Instant.now(); Instant later = now.plus(Duration.ofHours(1));
2)minus(Duration duration)
-
返回一个新的
Instant
对象,表示在当前时间基础上减去指定的持续时间。javaInstant now = Instant.now(); Instant earlier = now.minus(Duration.ofHours(1));
3)toEpochMilli()
-
将
Instant
转换为自 1970-01-01T00:00:00Z(UTC)以来的毫秒数。javaInstant now = Instant.now(); long epochMillis = now.toEpochMilli();
4)ofEpochMilli(long epochMilli)
-
从给定的自 1970-01-01T00:00:00Z 以来的毫秒数创建
Instant
。javaInstant now = Instant.now(); Instant instantFromMillis = Instant.ofEpochMilli(epochMillis);
5)案例:Instant类的简单使用
1.代码
javapackage org.xiji.mydate; import java.time.Duration; import java.time.Instant; public class MyInstant { public static void main(String[] args) { //Instant类的api简单使用 Instant instant = Instant.now(); System.out.println("Instant获取当前时间:"+instant); //表示在当前时间基础上加上指定的持续时间。 System.out.println("Instant获取当前时间戳后面一个小时:"+instant.plus(Duration.ofHours(1))); System.out.println("Instant获取当前时间戳之前一个小时:"+instant.minus(Duration.ofHours(1))); System.out.println("Instant获取当前时间戳的毫秒数:"+instant.toEpochMilli()); } }
2.效果
2. LocalDate 类
LocalDate
表示不带时区的日期(年、月、日)。
构造方法
1)LocalDate.now()
-
获取当前日期。
javaLocalDate today = LocalDate.now();
2)LocalDate.of(int year, int month, int dayOfMonth)
-
从给定的年、月、日创建
LocalDate
。javaLocalDate today = LocalDate.now(); LocalDate specificDate = LocalDate.of(2023, 10, 9);
常用方法
1)plusDays(long daysToAdd)
-
返回一个新的
LocalDate
对象,表示在当前日期基础上加上指定的天数。javaLocalDate today = LocalDate.now(); LocalDate tomorrow = today.plusDays(1);
2)minusDays(long daysToSubtract)
-
返回一个新的
LocalDate
对象,表示在当前日期基础上减去指定的天数。javaLocalDate today = LocalDate.now(); LocalDate yesterday = today.minusDays(1);
3)getYear()
, getMonthValue()
, getDayOfMonth()
-
分别获取年、月、日。
javaLocalDate today = LocalDate.now(); int year = today.getYear(); int month = today.getMonthValue(); int day = today.getDayOfMonth();
4)with(TemporalAdjuster adjuster)
-
使用
TemporalAdjuster
调整日期。javaLocalDate today = LocalDate.now(); LocalDate firstDayOfNextMonth = today.with(TemporalAdjusters.firstDayOfNextMonth());
案例:LocalDate的简单使用
1.代码
javapackage org.xiji.mydate; import java.time.LocalDate; public class MyLocalDate { public static void main(String[] args) { System.out.println("LocalDate的api简单使用"); LocalDate localDate = LocalDate.now(); System.out.println("LocalDate获取当前时间:"+localDate); System.out.println("LocalDate获取当前年份:"+localDate.getYear()); System.out.println("LocalDate获取当前月份:"+localDate.getMonth()); System.out.println("LocalDate获取星期几:"+localDate.getDayOfWeek()); } }
2.效果
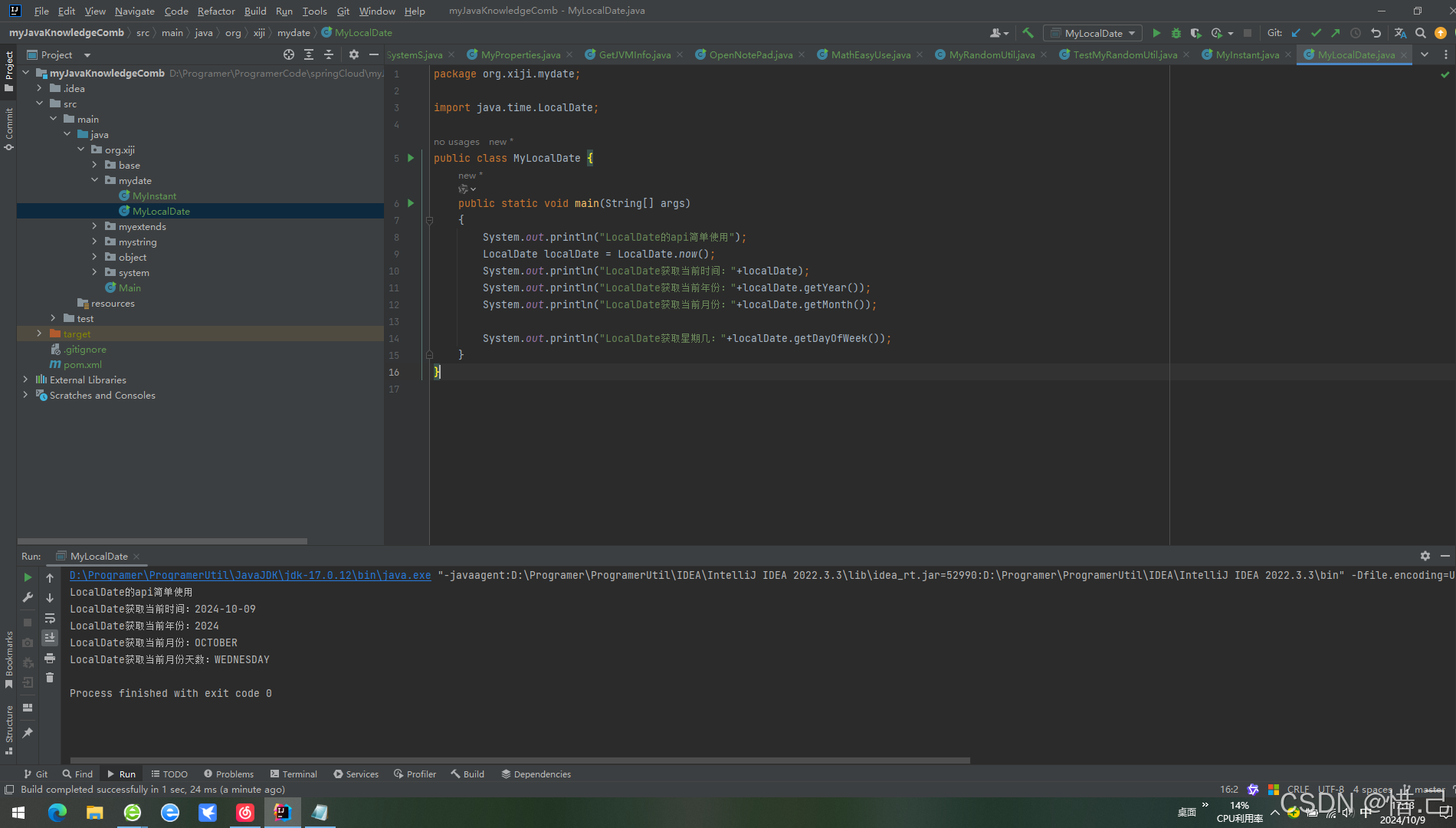
3. LocalTime 类
LocalTime
表示不带时区的时间(小时、分钟、秒、纳秒)。
构造方法
1)LocalTime.now()
-
获取当前时间。
javaLocalTime now = LocalTime.now();
2)LocalTime.of(int hour, int minute, int second, int nanoOfSecond)
-
从给定的小时、分钟、秒、纳秒创建
LocalTime
。javaLocalTime now = LocalTime.now(); LocalTime specificTime = LocalTime.of(14, 30, 0, 0);
常用方法
1)plusHours(long hoursToAdd)
-
返回一个新的
LocalTime
对象,表示在当前时间基础上加上指定的小时数。javaLocalTime now = LocalTime.now(); LocalTime later = now.plusHours(1);
2)minusMinutes(long minutesToSubtract)
-
返回一个新的
LocalTime
对象,表示在当前时间基础上减去指定的分钟数。javaLocalTime now = LocalTime.now(); LocalTime earlier = now.minusMinutes(30);
3)getHour()
, getMinute()
, getSecond()
, getNano()
-
分别获取小时、分钟、秒、纳秒。
javaLocalTime now = LocalTime.now(); int hour = now.getHour(); int minute = now.getMinute(); int second = now.getSecond(); int nano = now.getNano();
案例:LocalTime的简单使用
1.代码
javapackage org.xiji.mydate; import java.time.LocalTime; import java.time.format.DateTimeFormatter; public class MyLocalTime { public static void main(String[] args) { LocalTime localTime = LocalTime.now(); System.out.println("LocalTime获取当前时间:"+localTime); System.out.println("LocalTime获取当前小时:"+localTime.getHour()); System.out.println("LocalTime获取当前分钟:"+localTime.getMinute()); System.out.println("LocalTime获取当前秒:"+localTime.getSecond()); System.out.println("LocalTime获取当前毫秒:"+localTime.getNano()); System.out.println("LocalTime获取当前时间:"+localTime.toString()); //日期格式化 System.out.println("LocalTime日期格式化:"+localTime.format(DateTimeFormatter.ofPattern("HH:mm:ss"))); } }
2.效果
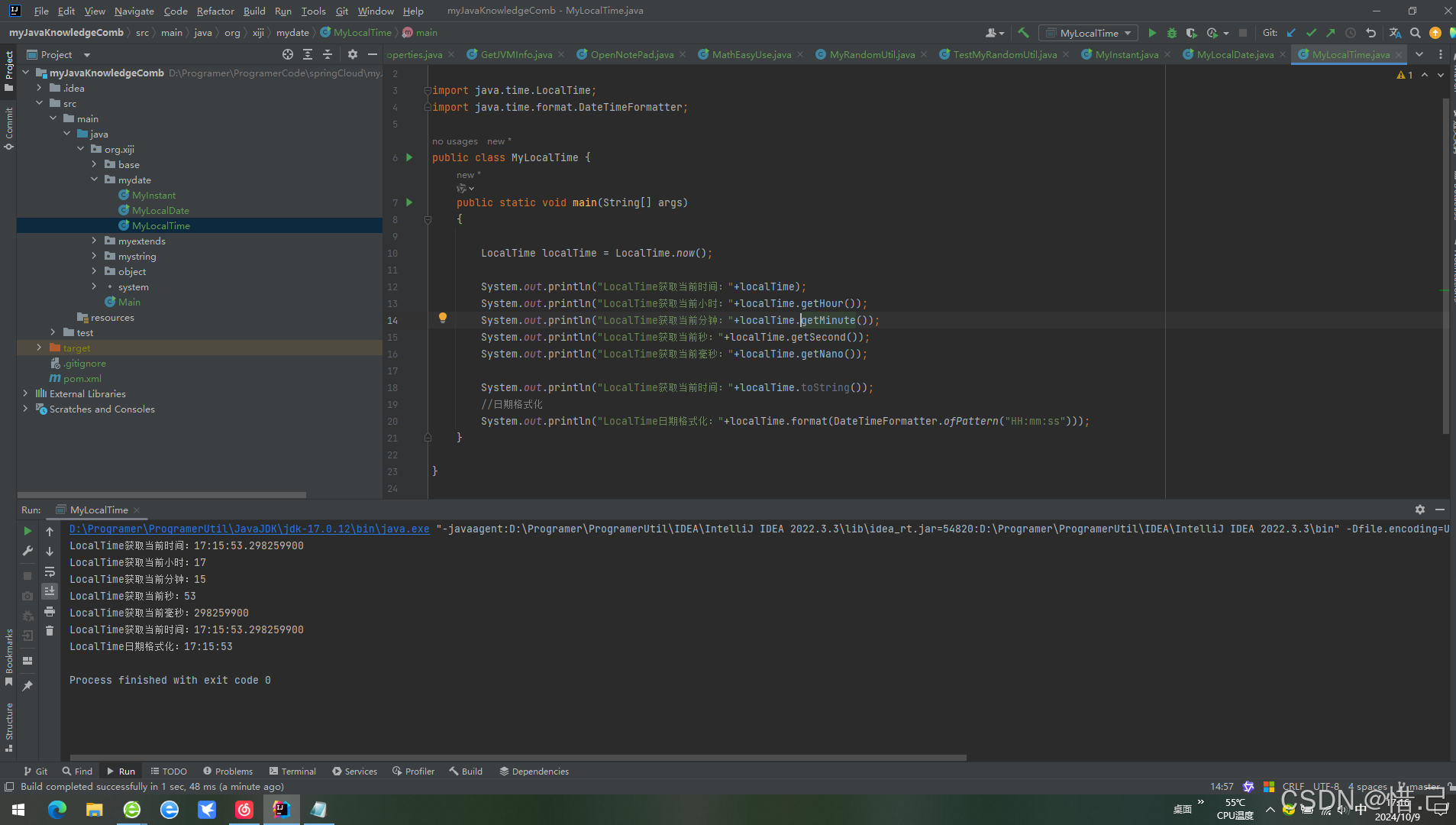
4. LocalDateTime 类
LocalDateTime
表示不带时区的日期和时间(年、月、日、小时、分钟、秒、纳秒)。
构造方法
1)LocalDateTime.now()
-
获取当前日期和时间。
javaLocalDateTime now = LocalDateTime.now();
2)LocalDateTime.of(LocalDate date, LocalTime time)
-
从给定的
LocalDate
和LocalTime
创建LocalDateTime
。javaLocalDateTime now = LocalDateTime.now(); LocalDateTime specificDateTime = LocalDateTime.of(specificDate, specificTime);
常用方法
1)plusDays(long daysToAdd)
-
返回一个新的
LocalDateTime
对象,表示在当前日期和时间基础上加上指定的天数。javaLocalDateTime now = LocalDateTime.now(); LocalDateTime tomorrow = now.plusDays(1);
2)minusHours(long hoursToSubtract)
-
返回一个新的
LocalDateTime
对象,表示在当前日期和时间基础上减去指定的小时数。javaLocalDateTime now = LocalDateTime.now(); LocalDateTime earlier = now.minusHours(1);
3)toLocalDate()
-
获取
LocalDate
部分。javaLocalDateTime now = LocalDateTime.now(); LocalDate datePart = now.toLocalDate();
4)toLocalTime()
-
获取
LocalTime
部分。javaLocalDateTime now = LocalDateTime.now(); LocalTime timePart = now.toLocalTime();
案例:LocalDateTime的简单使用
1.代码
javapackage org.xiji.mydate; import java.time.LocalDateTime; public class MyLocalDateTime { public static void main(String[] args) { System.out.println("LocalDateTime的api简单使用"); System.out.println("LocalDateTime获取当前时间:"+ LocalDateTime.now()); System.out.println("LocalDateTime获取当前年份:"+LocalDateTime.now().getYear()); System.out.println("LocalDateTime获取当前月份:"+LocalDateTime.now().getMonth()); System.out.println("LocalDateTime获取当前月份:"+LocalDateTime.now().getMonthValue()); System.out.println("LocalDateTime获取当前星期几:"+LocalDateTime.now().getDayOfWeek()); System.out.println("LocalDateTime获取当前星期几的值:"+LocalDateTime.now().getDayOfWeek().getValue()); System.out.println("LocalDateTime获取当前星期几的名字:"+LocalDateTime.now().getDayOfWeek().name()); } }
2.效果
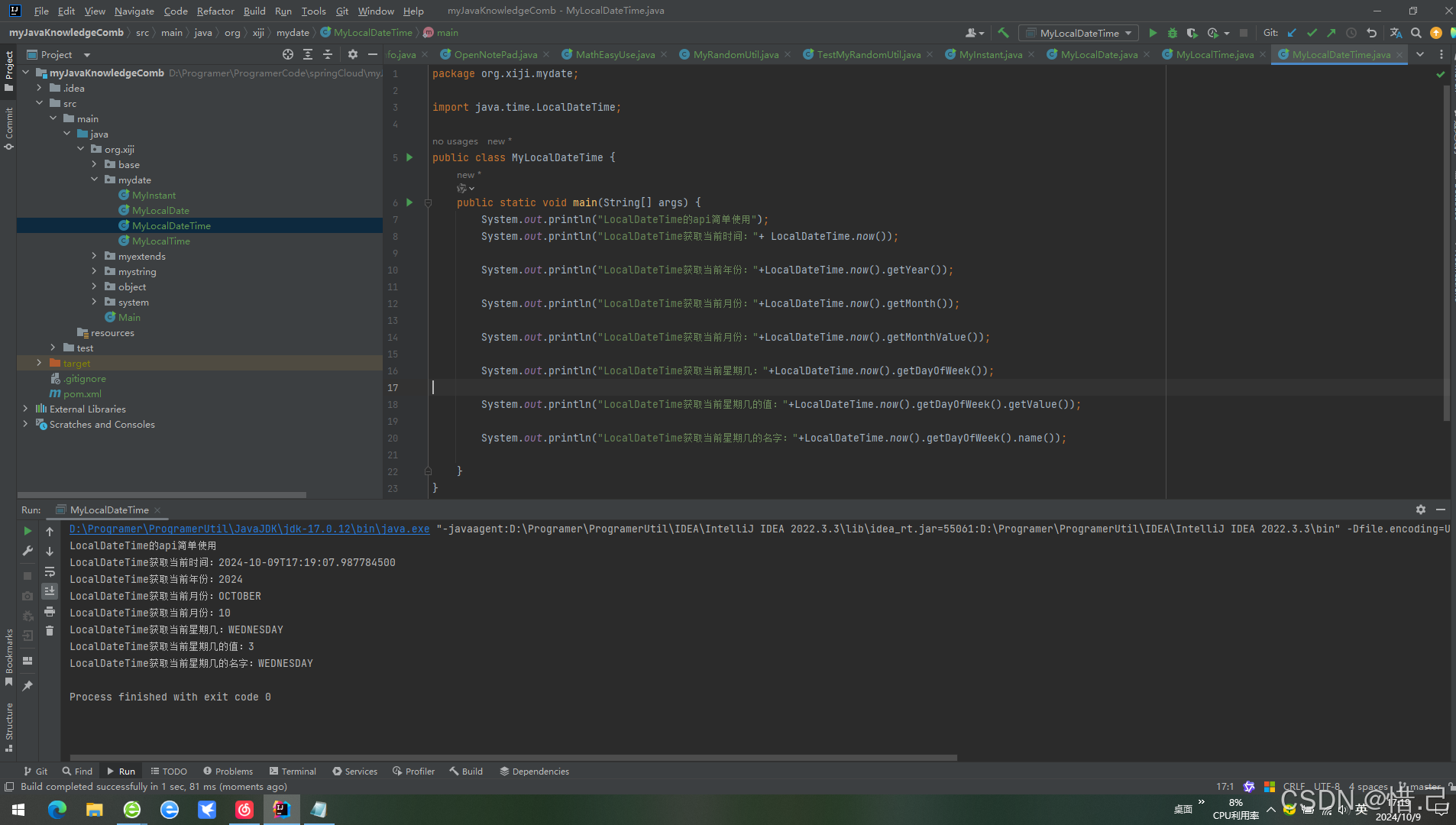
5. Period 类
Period
表示一段时间,通常是日期之间的差值,以年、月、日为单位。
构造方法
1)Period.between(LocalDate startDate, LocalDate endDate)
-
计算两个
LocalDate
之间的差值。javaPeriod period = Period.between(startDate, endDate);
常用方法
2)getYears()
, getMonths()
, getDays()
-
分别获取年、月、日。
javaPeriod period = Period.between(startDate, endDate); int years = period.getYears(); int months = period.getMonths(); int days = period.getDays();
3)plusYears(long yearsToAdd)
-
返回一个新的
Period
对象,表示在当前时间段基础上加上指定的年数。javaPeriod period = Period.between(startDate, endDate); Period longerPeriod = period.plusYears(1);
4)minusMonths(long monthsToSubtract)
-
返回一个新的
Period
对象,表示在当前时间段基础上减去指定的月数。javaPeriod period = Period.between(startDate, endDate); Period shorterPeriod = period.minusMonths(1);
案例:Period的简单使用
1.代码
javapackage org.xiji.mydate; import java.time.LocalDate; public class MyPeriod { public static void main(String[] args) { LocalDate date1 = LocalDate.of(2018, 1, 1); LocalDate date2 = LocalDate.of(2018, 12, 31); System.out.println("LocalDate的api简单使用"); System.out.println("LocalDate获取date1当前时间:"+date1); System.out.println("LocalDate获取date2当前时间:"+date2); System.out.println("LocalDate。date2是否在date1之后:"+(date2.isAfter(date1))); System.out.println("LocalDate。date2是否在date1之前:"+(date2.isBefore(date1))); System.out.println("LocalDate。date2和date1是否相等:"+(date2.isEqual(date1))); System.out.println("LocalDate。date2是否是闰年:"+(date2.isLeapYear())); System.out.println("LocalDate。date2这个月的天数:"+(date2.lengthOfMonth())); System.out.println("LocalDate。date2这一年的天数:"+(date2.lengthOfYear())); System.out.println("LocalDate。date2之前一天:"+(date2.minusDays(1))); System.out.println("LocalDate。date2之前一个月:"+(date2.minusMonths(1))); System.out.println("LocalDate。date2之前一年:"+(date2.minusYears(1))); System.out.println("LocalDate。date2之后一天:"+(date2.plusDays(1))); System.out.println("LocalDate。date2之后一个月:"+(date2.plusMonths(1))); System.out.println("LocalDate。date2之后一年:"+(date2.plusYears(1))); } }
2.效果
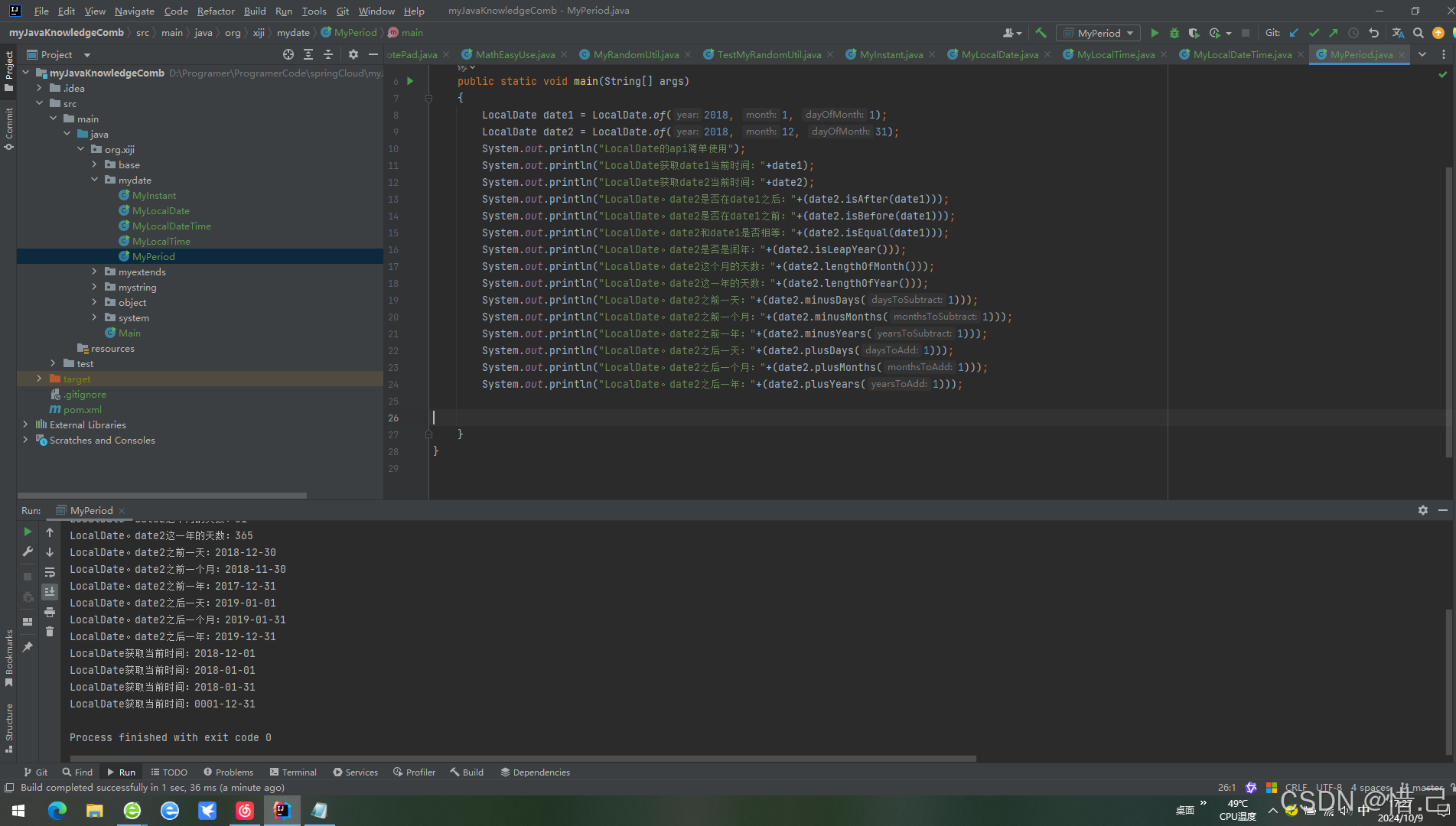
6. Duration 类
Duration
表示一段时间,通常是时间之间的差值,以秒和纳秒为单位。
构造方法
1)Duration.between(LocalTime startTime, LocalTime endTime)
-
计算两个
LocalTime
之间的差值。javaDuration duration = Duration.between(startTime, endTime);
2)Duration.ofSeconds(long seconds)
-
从给定的秒数创建
Duration
。javaDuration oneMinute = Duration.ofSeconds(60);
常用方法
1)getSeconds()
, getNano()
-
分别获取秒数和纳秒数。
javaDuration duration = Duration.between(startTime, endTime); long seconds = duration.getSeconds(); int nano = duration.getNano();
2)plusSeconds(long secondsToAdd)
-
返回一个新的
Duration
对象,表示在当前时间段基础上加上指定的秒数。javaDuration duration = Duration.between(startTime, endTime); Duration longerDuration = duration.plusSeconds(60);
3)minusNanos(long nanosToSubtract)
-
返回一个新的
Duration
对象,表示在当前时间段基础上减去指定的纳秒数。javaDuration duration = Duration.between(startTime, endTime); Duration shorterDuration = duration.minusNanos(1000000);
案例:Duration类的简单使用
1.代码
javapackage org.xiji.mydate; import java.time.Duration; public class MyDuration { public static void main(String[] args) { System.out.println("Duration的api简单使用"); Duration duration = Duration.ofDays(1); System.out.println("Duration获取天数:"+duration.toDays()); System.out.println("Duration获取小时:"+duration.toHours()); System.out.println("Duration获取分钟:"+duration.toMinutes()); System.out.println("Duration获取秒:"+duration.getSeconds()); System.out.println("Duration获取纳秒:"+duration.toNanos()); System.out.println("Duration获取毫秒:"+duration.toMillis()); } }
2.效果
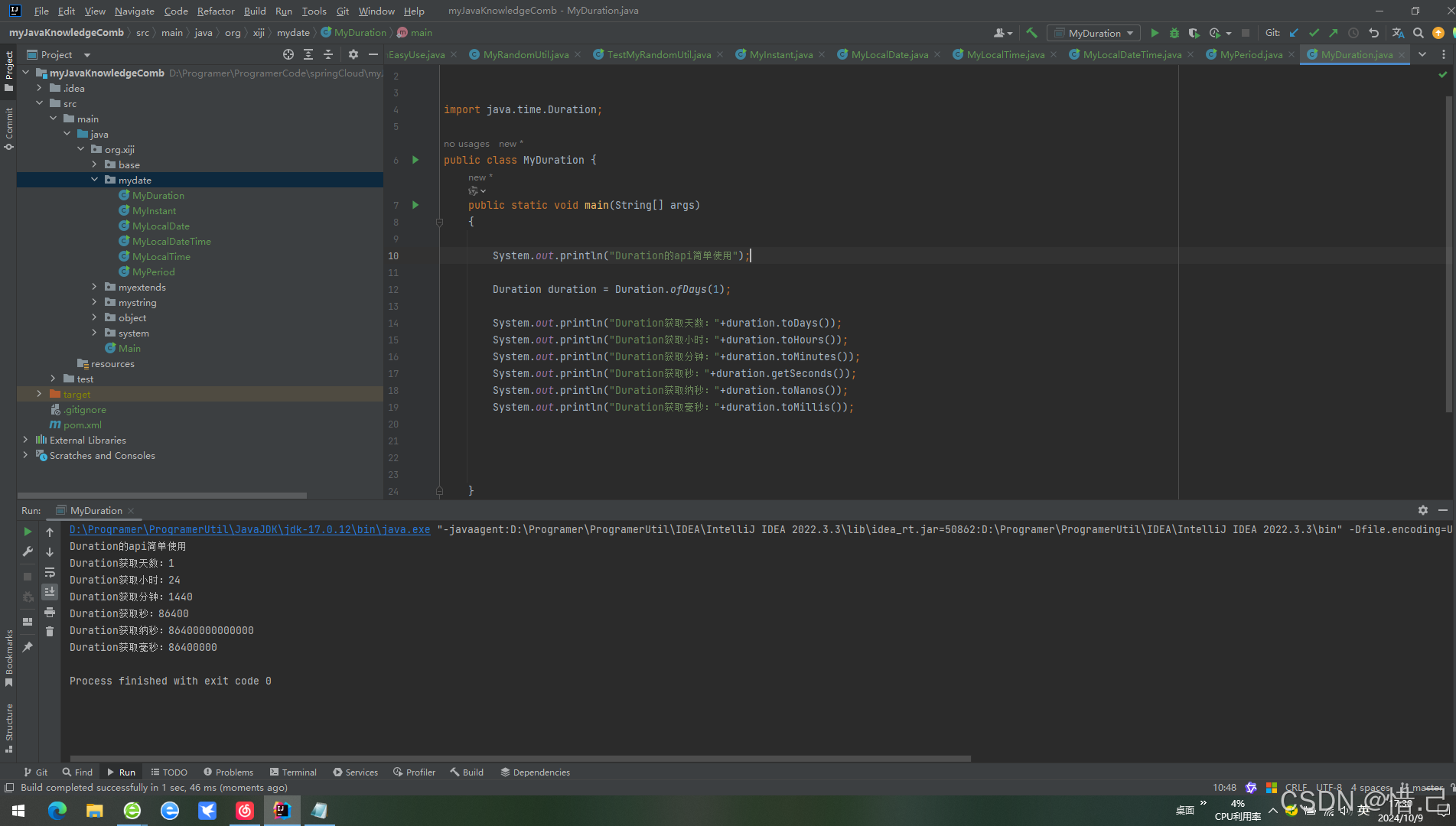