一 List的介绍
1.什么是List?
List是一个接口,继承于Collection。
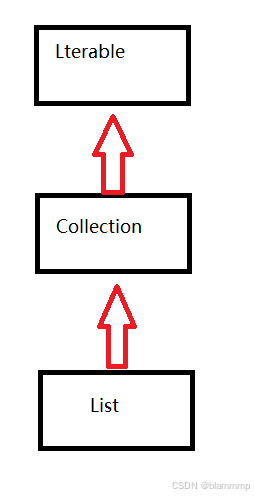
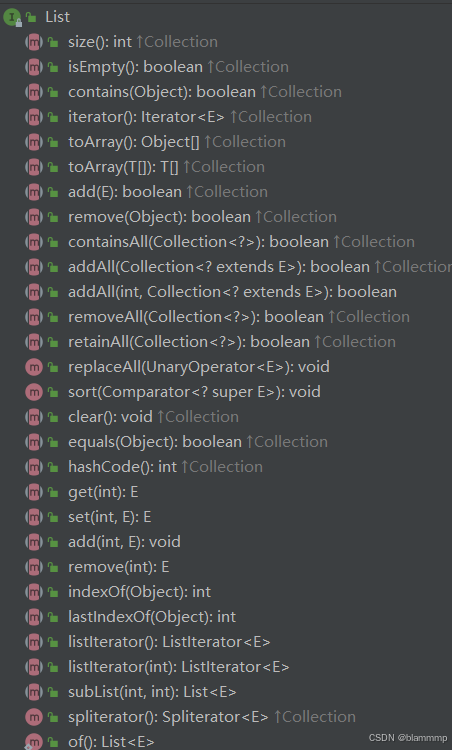
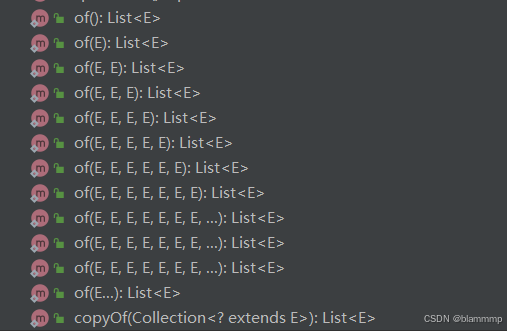
Collection也是一个接口
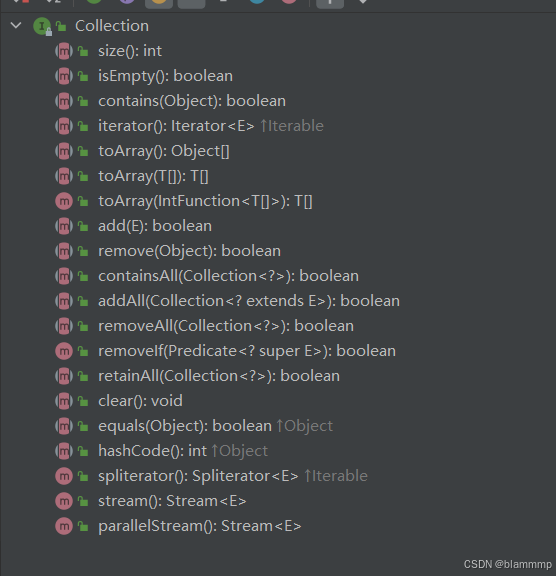
Lterable也是一个接口,表示实现该接口的类是可以逐个元素遍历的类。
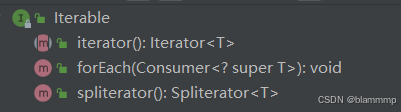
2.List的使用
List是一个接口,不能被实例化,ArrayList和LinkedList都实现了List接口。
二 ArrayList和顺序表
在集合框架中ArrayList是一个普通的类,实现了List接口。
- 动态大小 :
ArrayList
的大小可以根据需要动态调整,不需要手动管理数组的大小。 - 易于使用 :提供了许多方便的方法,如
add()
,remove()
,contains()
,get()
等,使得操作更为简单。 - 自动扩展 :当元素超过当前容量时,
ArrayList
会自动扩展其内部数组的大小。
我们可以实现一些关于ArrayList类中的方法
注:
java
public static final int DEFAULT_CAPACITY = 10;
public MyArrayList() {
this.array = new int[DEFAULT_CAPACITY];
}
这些代码的作用:在一个自定义数组实现中,使用这样的常量可以在初始化时指定数组的默认容量。
1.新增元素,默认在数组最后新增
在实现add()方法时,我们需要检验这个数组的大小,如果数组容量满了,我们需要对数组进行扩容。
java
public class MyArrayList implements IList{
public int size;
public int[] array;
public static final int DEFAULT_CAPACITY = 10;
public MyArrayList() {
this.array = new int[DEFAULT_CAPACITY];
}
public boolean arrayIsFull(){
return this.size == array.length;
}
private void expand(){
this.array= Arrays.copyOf(this.array,2*array.length);
}
public void add(int data) {
if(arrayIsFull()){
expand();
}
this.array[this.size]=data;
this.size++;
}
}
2.在 pos 位置新增元素
首先我们新建一个类,手动写一个异常,如果定位不合法时,抛出这个异常。然后在指定位置增加元素时,我们需要判断指定的位置是否合法,是否满足条件,如果不满足,则会抛出这个定位不合法异常,再进行判断,判断这个数组是否满了,如果容量满了,则进行扩容,最后用catch对异常进行处理。
java
public class PosIllegal extends RuntimeException {
public PosIllegal(){
};
public PosIllegal(String mas){
super(mas);
};
}
public class MyArrayList implements IList{
public int size;
public int[] array;
public static final int DEFAULT_CAPACITY = 10;
public MyArrayList() {
this.array = new int[DEFAULT_CAPACITY];
}
public boolean arrayIsFull(){
return this.size == array.length;
}
private void expand(){
this.array= Arrays.copyOf(this.array,2*array.length);
}
public void checkPos(int pos) throws PosIllegal{
if(pos<0 || pos>size){
throw new PosIllegal("pos位置不合法");
}
}
public void add(int pos, int data) {
try {
checkPos(pos);
if (arrayIsFull()){
expand();
}
for (int i = size-1; i >=pos; i--) {
this.array[i+1]=this.array[i];
}
array[pos]=data;
}catch (PosIllegal e){
System.out.println("pos位置不合法");
e.printStackTrace();
}
}
3.判定是否包含某个元素
java
public class MyArrayList implements IList{
public int size;
public int[] array;
public static final int DEFAULT_CAPACITY = 10;
public MyArrayList() {
this.array = new int[DEFAULT_CAPACITY];
}
public boolean contains(int toFind) {
for (int i = 0; i < array.length; i++) {
if(array[i]==toFind) {
return true;
}
}
return false;
}
}
4.查找某个元素对应的位置
java
public class MyArrayList implements IList{
public int size;
public int[] array;
public static final int DEFAULT_CAPACITY = 10;
public MyArrayList() {
this.array = new int[DEFAULT_CAPACITY];
}
public int indexOf(int toFind) {
for (int i = 0; i < array.length; i++) {
if(array[i]==array[toFind]) {
return array[i];
}
}
return 0;
}
}
5. 获取 pos 位置的元素
首先我们手写两个异常,一个是判断是否数组为空,另一个判断pos的位置是否合法,然后处理异常,最后将pos位置的元素返回。
java
public class PosIllegal extends RuntimeException {
public PosIllegal(){
};
public PosIllegal(String mas){
super(mas);
};
}
public class NullException extends RuntimeException{
public NullException() {
}
public NullException(String mas) {
super(mas);
}
}
public class MyArrayList implements IList{
public int size;
public int[] array;
public static final int DEFAULT_CAPACITY = 10;
public MyArrayList() {
this.array = new int[DEFAULT_CAPACITY];
}
public void checkPos(int pos) throws PosIllegal{
if(pos<0 || pos>size){
throw new PosIllegal("pos位置不合法");
}
}
private void checkNull()throws NullException{
throw new NullException("顺序表为空");
}
public void checkEmpty(){
if (size==0){
checkNull();
}
}
public int get(int pos) {
try {
checkEmpty();
checkPos(pos);
return array[pos];
}catch (PosIllegal e){
e.printStackTrace();
}catch (NullException e){
e.printStackTrace();
}
return -1;
}
}
6.给 pos 位置的元素设为 value
首先我们手写两个异常,一个是数组为空时抛出,另一个判断pos的位置是否合法,然后处理异常,最后将pos位置的元素设置为value。
java
public class PosIllegal extends RuntimeException {
public PosIllegal(){
};
public PosIllegal(String mas){
super(mas);
};
}
public class NullException extends RuntimeException{
public NullException() {
}
public NullException(String mas) {
super(mas);
}
}
public class MyArrayList implements IList{
public int size;
public int[] array;
public static final int DEFAULT_CAPACITY = 10;
public MyArrayList() {
this.array = new int[DEFAULT_CAPACITY];
}
public void checkPos(int pos) throws PosIllegal{
if(pos<0 || pos>size){
throw new PosIllegal("pos位置不合法");
}
}
private void checkNull()throws NullException{
throw new NullException("顺序表为空");
}
public void checkEmpty(){
if (size==0){
checkNull();
}
}
public void set(int pos, int value) {
try {
checkEmpty();
checkPos(pos);
array[pos]=value;
}catch (PosIllegal e){
e.printStackTrace();
}catch (NullException e){
e.printStackTrace();
}
}
7.删除第一次出现的关键字key
首先手写一个异常,数组为空时抛出,然后将index的返回值赋给pos,如果pos不为-1时,将pos+1位置的值赋给pos位置,然后将size的大小-1。
indexOf(toRemove)
是一个方法调用,用于查找 toRemove
在数组中的索引。它会遍历数组,如果找到 toRemove
,就返回其索引。如果没有找到,则返回 -1
。在你的 remove
方法中,它用于确定要删除的元素的位置。
java
public class NullException extends RuntimeException{
public NullException() {
}
public NullException(String mas) {
super(mas);
}
}
public class MyArrayList implements IList{
public int size;
public int[] array;
public static final int DEFAULT_CAPACITY = 10;
public MyArrayList() {
this.array = new int[DEFAULT_CAPACITY];
}
private void checkNull()throws NullException{
throw new NullException("顺序表为空");
}
public void checkEmpty(){
if (size==0){
checkNull();
}
}
public void remove(int toRemove) {
try {
checkEmpty();
int pos=indexOf(toRemove);
if(pos==-1){
return;
}
for (int i =pos; i <size-1 ; i++) {
array[i]=array[i+1];
}
array[size-1]=0;
size--;
}catch (NullException e){
e.printStackTrace();
}
}
8.清空顺序表
java
public class MyArrayList implements IList{
public int size;
public int[] array;
public static final int DEFAULT_CAPACITY = 10;
public MyArrayList() {
this.array = new int[DEFAULT_CAPACITY];
}
public void clear() {
size=0;
//如果将数组元素设置为默认值
/*for (int i = 0; i < size - 1; i++) {
array[i]=0;
}*/
}
}
希望能对大家有所帮助!!!!!
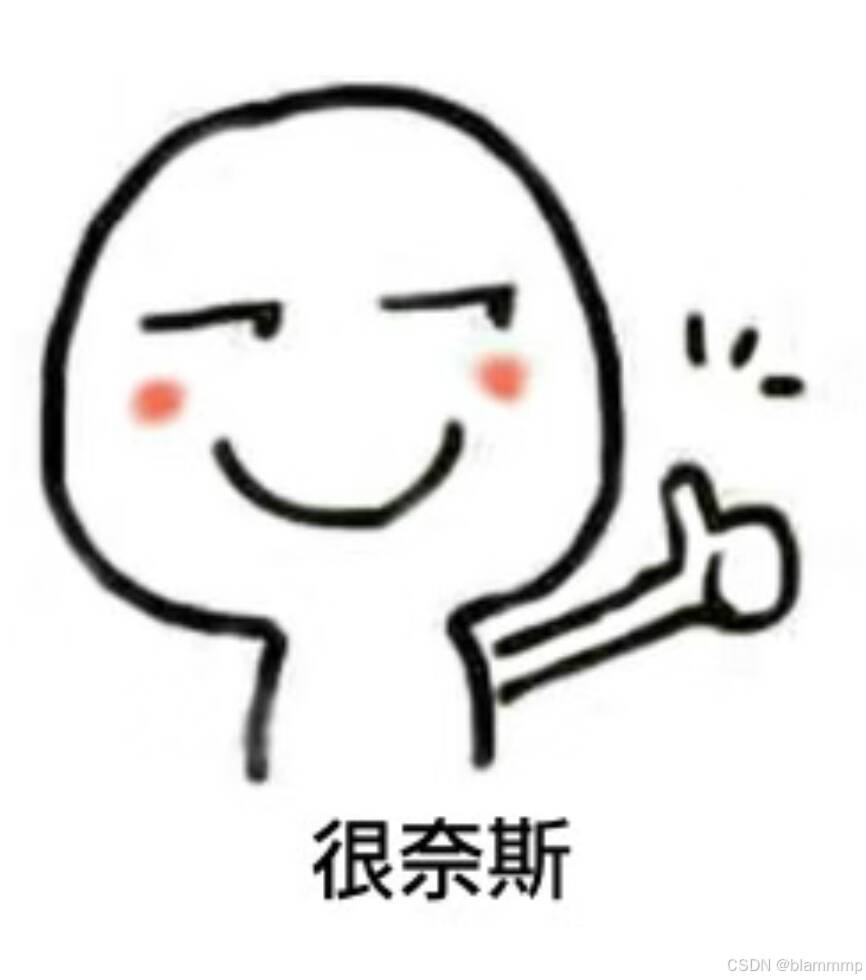