演示地址 https://www.bilibili.com/video/BV1cW421d79L/?vd_source=b8515e53f6d4c564b541d98dcc9df990
语音控制板的配置
web展示页面
esp32 程序
c
#include <ESP8266WiFi.h>
#include <ESP8266WebServer.h>
#include <LittleFS.h>
#include <WebSocketsServer.h>
ESP8266WebServer server(80);
WebSocketsServer webSocket = WebSocketsServer(81); // WebSocket服务器在端口81上运行
void setup() {
Serial.begin(115200);
WiFi.softAP("ESP8266-AP", "12345678");
// 初始化LittleFS
if (!LittleFS.begin()) {
Serial.println("An Error has occurred while mounting LittleFS");
return;
}
server.on("/", HTTP_GET, handleRoot);
server.on("/paper-full.min.js", HTTP_GET, handleJS);
server.on("/chilun.svg", HTTP_GET, handleSVG); // 添加路由处理SVG请求
server.begin();
Serial.println("HTTP server started");
webSocket.begin(); // 启动WebSocket服务器
webSocket.onEvent(webSocketEvent); // 注册WebSocket事件处理函数
}
void handleRoot() {
File file = LittleFS.open("/index.html", "r");
if (!file) {
Serial.println("File not found");
return;
}
server.streamFile(file, "text/html");
file.close();
}
void handleJS() {
File file = LittleFS.open("/paper-full.min.js", "r"); // 打开JavaScript文件
if (!file) {
Serial.println("File not found");
return;
}
server.streamFile(file, "application/javascript"); // 确保MIME类型是正确的
file.close();
}
// 处理SVG文件的请求
void handleSVG() {
File file = LittleFS.open("/chilun.svg", "r");
if (!file) {
Serial.println("File not found");
return;
}
server.streamFile(file, "image/svg+xml"); // 确保MIME类型设置为SVG
file.close();
}
// WebSocket事件处理函数
void webSocketEvent(uint8_t num, WStype_t type, uint8_t * payload, size_t length) {
switch(type) {
case WStype_DISCONNECTED:
Serial.printf("[%u] Disconnected!\n", num);
break;
case WStype_CONNECTED:
{
IPAddress ip = webSocket.remoteIP(num);
Serial.printf("[%u] Connected from %d.%d.%d.%d\n", num, ip[0], ip[1], ip[2], ip[3]);
webSocket.sendTXT(num, "Hello from ESP8266"); // 向连接的客户端发送消息
}
break;
case WStype_TEXT:
{
Serial.printf("[%u] Received Text: %s\n", num, payload);
// 将接收到的消息广播给所有连接的客户端
String message = String((char *)payload); // 将payload转换为String对象,以便处理
webSocket.broadcastTXT(message); // 使用broadcastTXT直接广播消息
}
}
}
void loop() {
server.handleClient();
webSocket.loop(); // 确保处理WebSocket事件
// 检查串口是否有数据读取
if (Serial.available() > 0) {
String data = Serial.readString(); // 读取串口数据
Serial.print("Received from Serial: "); // 打印接收到的消息
Serial.println(data); // 显示串口接收到的数据
webSocket.broadcastTXT(data); // 将读取到的数据发送给所有WebSocket客户端
}
}
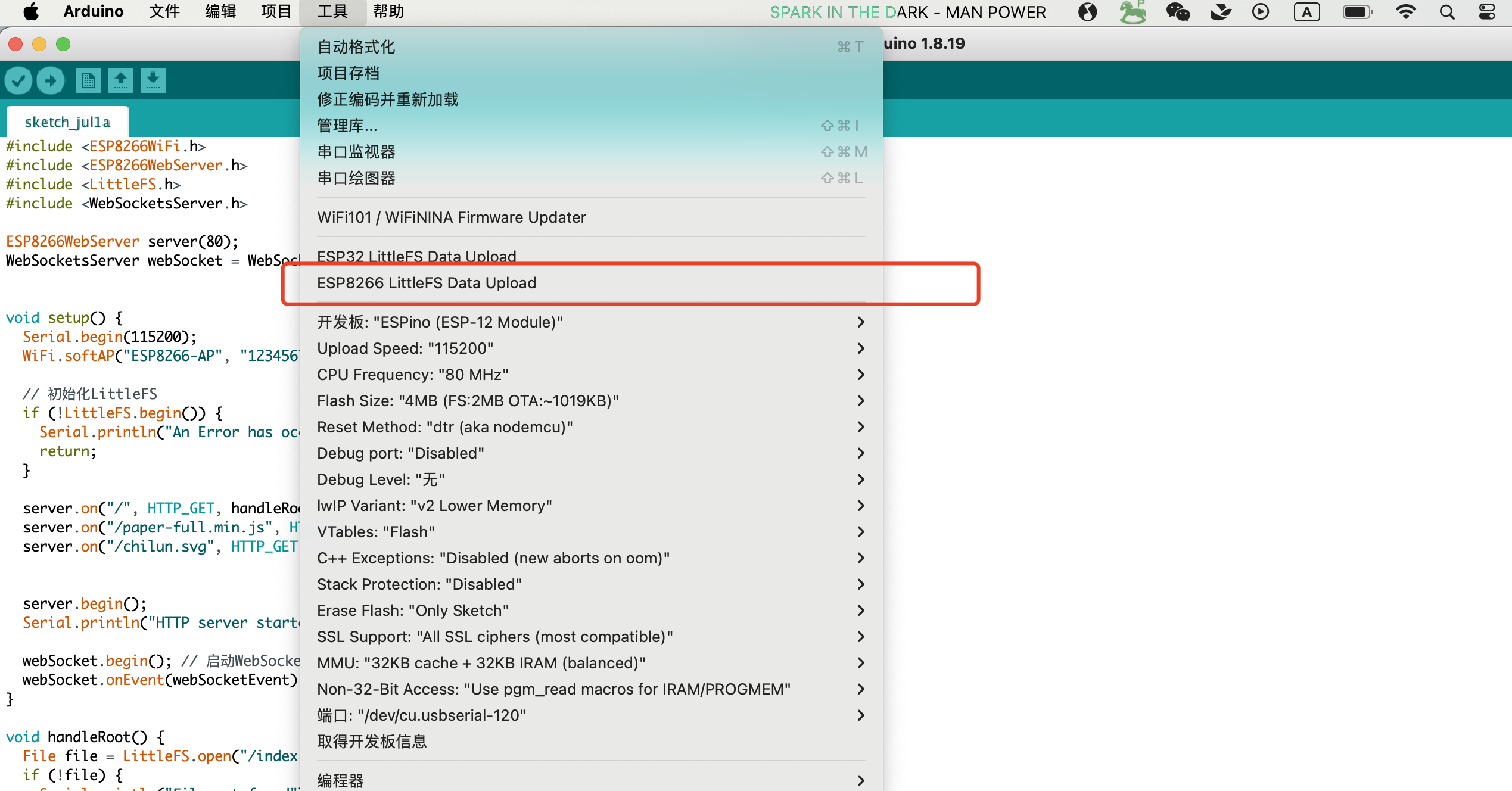
参考资料
https://github.com/earlephilhower/arduino-littlefs-upload
https://github.com/earlephilhower/arduino-esp8266littlefs-plugin