- 操作系统:ubuntu22.04
- OpenCV版本:OpenCV4.9
- IDE:Visual Studio Code
- 编程语言:C++11
算法描述
创建一个滑动条并将其附加到指定的窗口。
该函数 createTrackbar
创建一个具有指定名称和范围的滑动条(滑块或范围控制),将变量 value
分配为与滑动条位置同步的位置,并指定回调函数 onChange
在滑动条位置改变时被调用。创建的滑动条将显示在指定的窗口 winname
中。
cv::createTrackbar() 函数用于在 OpenCV 的窗口中创建一个滑动条(trackbar),可以用来调整一些参数,并实时反映在图像处理的效果中。
注释
仅 Qt 后端\] 如果滑动条应附加到控制面板,则 `winname` 可以为空。
点击每个滑动条的标签可以手动编辑滑动条的值。
### 函数原型
```cpp
int cv::createTrackbar
(
const String & trackbarname,
const String & winname,
int * value,
int count,
TrackbarCallback onChange = 0,
void * userdata = 0
)
```
### 参数
* 参数trackbarname 创建的滑动条的名称。
* 参数winname 将作为创建的滑动条父级的窗口的名称。
* 参数value 指向一个整型变量的可选指针,该变量的值反映了滑块的位置。在创建时,滑块的位置由这个变量定义。
* 参数count 滑块的最大位置。最小位置始终为 0。
* 参数onChange 指向每次滑块位置改变时将被调用的函数的指针。此函数应该原型化为 `void Foo(int, void*);`,其中第一个参数是滑块的位置,第二个参数是用户数据(参见下一个参数)。如果回调是指向 `NULL` 的指针,则不调用回调,但只会更新 `value`。
* 参数userdata 作为参数原样传递给回调的用户数据。它可以用来在不使用全局变量的情况下处理滑块事件。
### 返回值
返回一个整数值,表示操作的结果或状态。具体含义取决于 OpenCV 实现。
### 代码示例:
```cpp
#include
#include
// 回调函数
void onTrackbarSlide( int pos, void* userData )
{
cv::Mat img = *( cv::Mat* )userData;
cv::Mat thresholdImg;
cv::threshold( img, thresholdImg, pos, 255, cv::THRESH_BINARY );
cv::imshow( "Threshold Image", thresholdImg );
}
int main()
{
// 加载图像
cv::Mat img = cv::imread( "/media/dingxin/data/study/OpenCV/sources/images/hawk.jpg", cv::IMREAD_GRAYSCALE );
if ( img.empty() )
{
std::cerr << "Error: Image not found!" << std::endl;
return -1;
}
// 创建窗口
cv::namedWindow( "Threshold Image" );
// 创建滑动条
int thresholdValue = 128;
int result = cv::createTrackbar( "Threshold Value", "Threshold Image", &thresholdValue, 255, onTrackbarSlide, &img );
if ( result < 0 )
{
std::cerr << "Failed to create trackbar." << std::endl;
return -1;
}
// 初始显示
cv::Mat thresholdImg;
cv::threshold( img, thresholdImg, thresholdValue, 255, cv::THRESH_BINARY );
cv::imshow( "Threshold Image", thresholdImg );
// 主循环
while ( true )
{
int key = cv::waitKey( 1 );
if ( key == 27 )
{ // ESC 键
break;
}
}
// 释放资源
cv::destroyAllWindows();
return 0;
}
```
### 运行结果
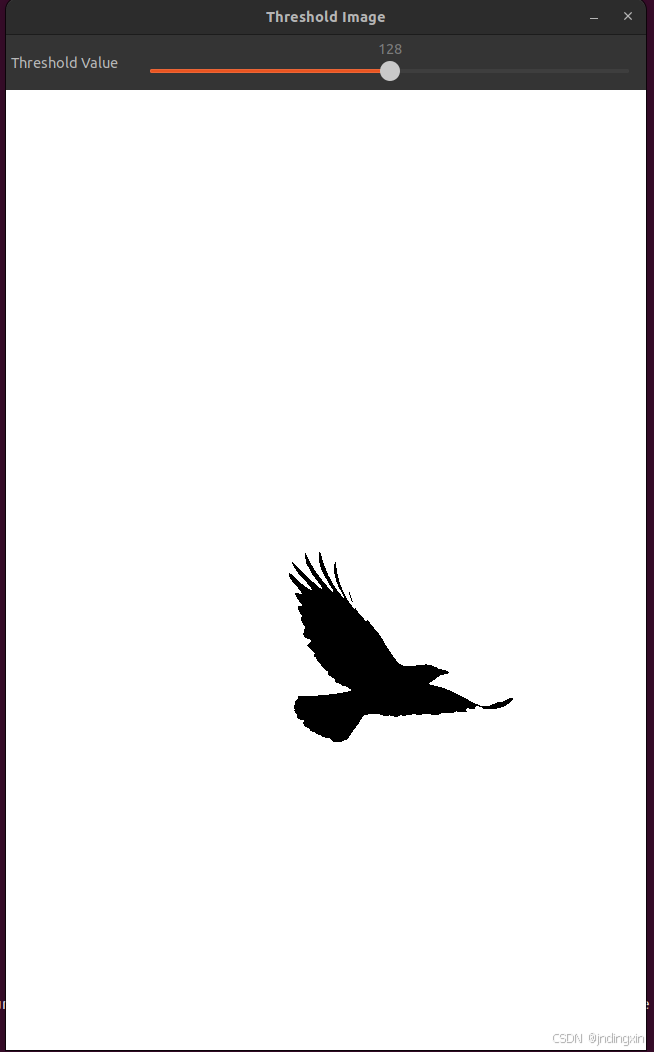