问题: 编写程序完成如下功能:
1> open一个文件
2> 创建一个新线程(记得将描述符传给线程入口函数)
3> 新线程向文件里写入"abc"
4> 主线程向文件里写入"def"
要求确保文件内容为"abcdef"
pthread_create ( )
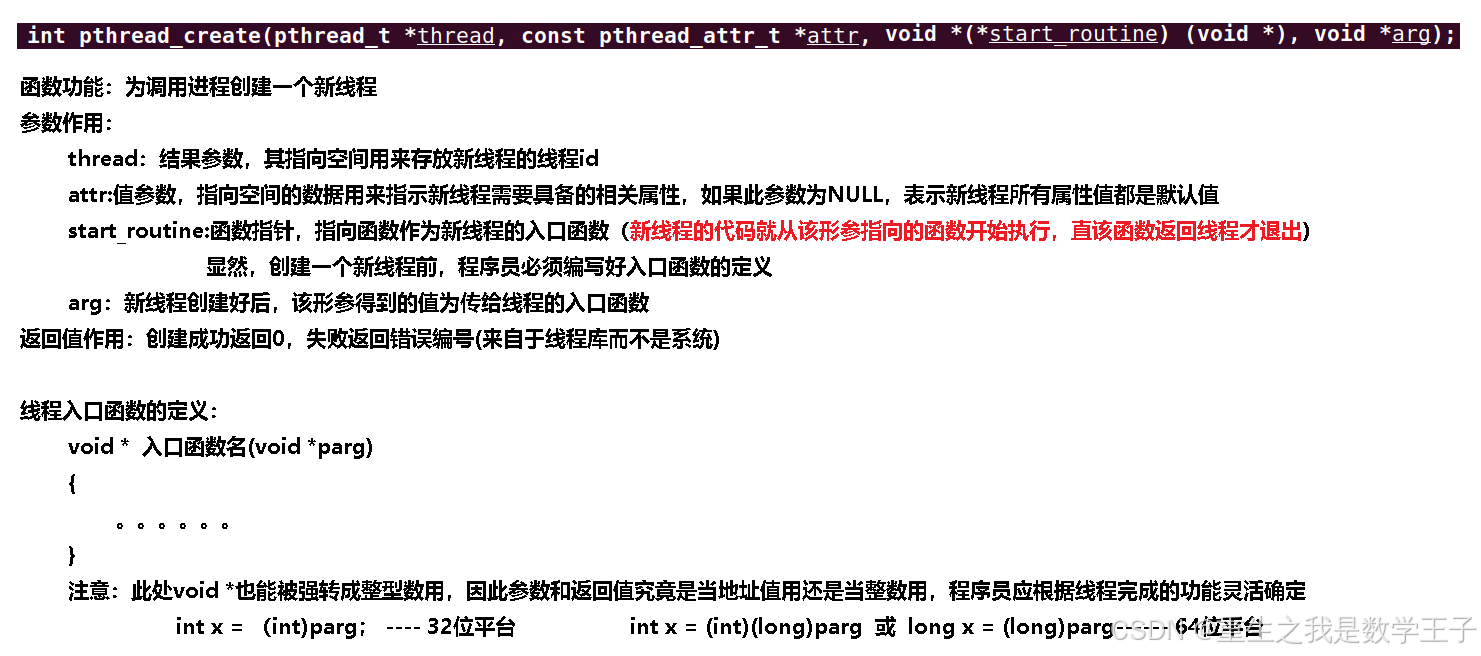
pthread_join( )
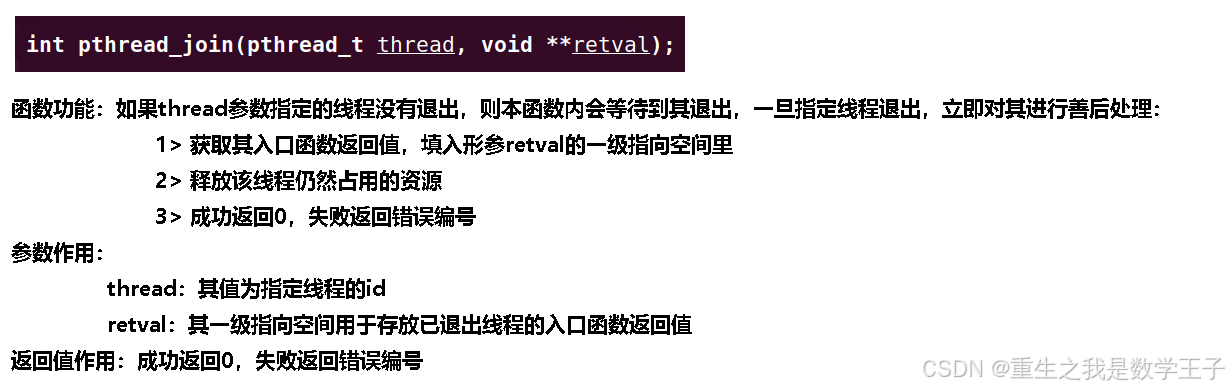
代码:
cpp
#include <stdio.h>
#include <pthread.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <unistd.h>
void *func(void *parg){
int fd = (int)(long)parg;
write(fd,"abc",3);
return (void*)(long)199; // 返回值测试,先强转成long型(8字节),再强转成void*
}
int main(int argc,char *argv[]){
int fd = -1;
pthread_t threadId; // threadId
int ret = 0;
void* s=NULL; // 存储返回值
fd = open("test.txt",O_RDWR | O_CREAT | O_TRUNC,0666); // open file
if(fd < 0){
printf("w-open %s failed\n","test.txt");
return 1;
}
ret = pthread_create(&threadId, NULL, func, (void*)(long)fd); // pthread_create()
if(ret){
close(fd);
fd = -1;
printf("pthread_create failed.\n");
return 2;
}
pthread_join(threadId,&s); // pthread_join()
printf("%ld\n",threadId);
printf("%d\n",(int)(long)s); // test return value
write(fd,"def",3);
close(fd);
fd = -1;
return 0;
}
输出:
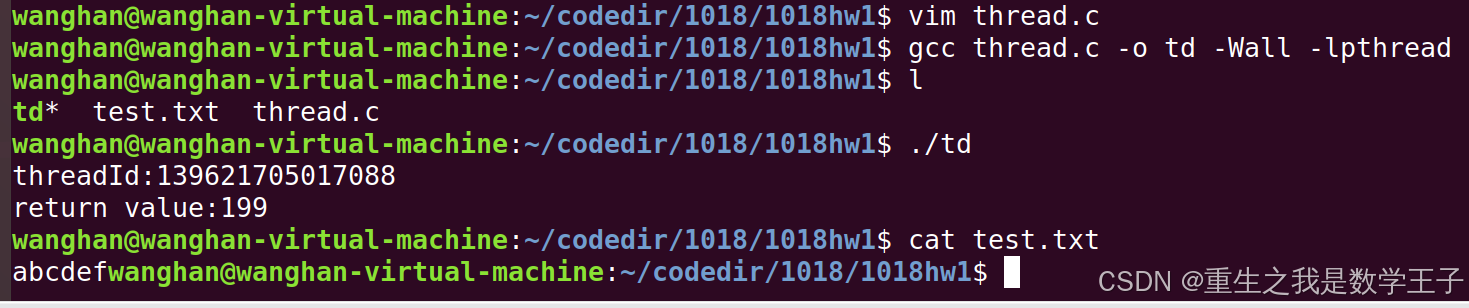