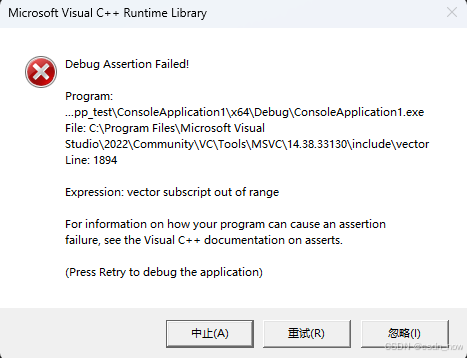
Debug Assertion Failed
Expression: vector subscript out of range
出现上面的弹窗,导致程序崩溃,如何让系统不崩溃呢。答:使用vec.at(i)。
有问题的程序
cpp
i#include <iostream>
#include <vector>
using namespace std;
int main()
{
vector<int> aa(5);
for (int i = 0; i < 6; ++i) // 超出了vector的范围了
{
std::cout << aa[i] << std::endl; // 方式1
std::cout << *(aa.begin() + i) << std::endl; // 方式2
std::cout << aa.at(i) << std::endl; // 方式3
}
printf("==========\n");
return 0;
}
出现的问题
方式一报错
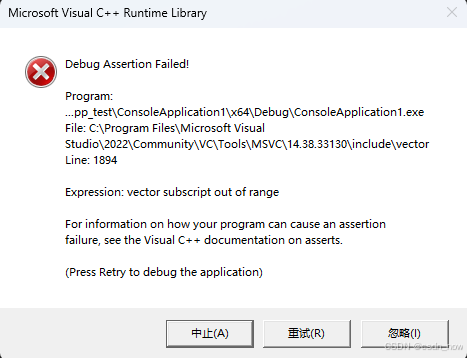
方式二报错
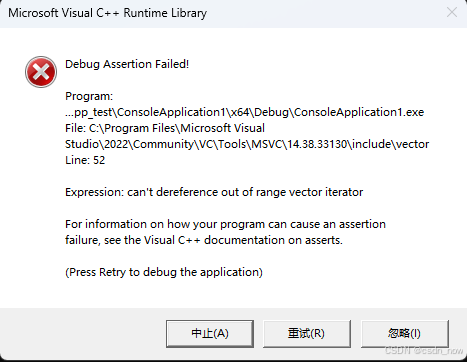
方式三报错。
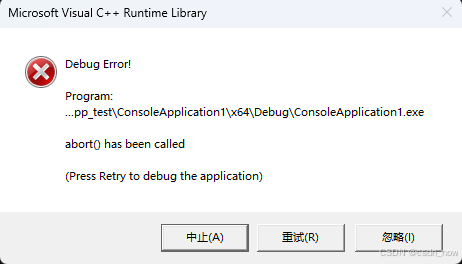
解决方案
修改后的代码,添加try-catch组合,使用第三种方式 vec.at(i)。在win中的三种方式,只有 at(n) 会抛出 std::out_of_range 异常,try-catch才能捕获到。
参考:
关于 std::vector 的下标越界检查_vector下标超出范围-CSDN博客
C++中的vector subscript out of range问题解决 - 极简博客
cpp
#include <iostream>
#include <vector>
using namespace std;
int main()
{
vector<int> aa(5);
try
{
for (int i = 0; i < 6; ++i) // 超出了vector的范围了
{
//std::cout << aa[i] << std::endl; // 方式1
//std::cout << *(aa.begin() + i) << std::endl; // 方式2
std::cout << aa.at(i) << std::endl; // 方式3
}
}
catch (std::out_of_range)
{
printf("check the range\n");
}
printf("==========\n");
return 0;
}
cpp
try {
std::cout << vec.at(20) << std::endl;
} catch (const std::out_of_range& e) {
std::cout << "Invalid index" << std::endl;
}
不过,最好还是在程序中,vector用vector.size() 来判断是否越界。
补充知识:
异常处理 try-catch
在C++编程中,异常处理 try-catch 是一种重要的错误管理机制,它允许程序在遇到不可预见的问题时能够优雅地恢复或报告错误,而不是直接崩溃。
基本的try-catch结构
cpp
try {
// 可能抛出异常的代码
throw std::runtime_error("发生错误");
} catch (const std::exception& e) {
// 处理异常
std::cerr << "捕获到异常: " << e.what() << '\n';
}
当有多个catch挨着,运行了第一个catch后,后面的catch就不运行了。
cpp
#include <iostream>
#include <vector>
using namespace std;
int main()
{
vector<int> aa(5);
try
{
aa.at(5) = 10;
}
// 当有多个catch挨着,运行了第一个catch后,后面的catch就不运行了。
catch (const std::exception& e)
{
std::cerr << "捕获到异常: " << e.what() << '\n'; // 处理异常
}
catch (std::out_of_range)
{
printf("check the range\n");
}
return 0;
}
cpp
#include <iostream>
#include <vector>
using namespace std;
int main()
{
vector<int> aa(5);
try
{
try { aa.at(5) = 0; }
catch (std::out_of_range) // 这个catch后,后面的就不再catch了。
{
printf("check the range 2\n");
}
}
// 当有多个catch挨着,运行了第一个catch后,后面的catch就不运行了。
catch (const std::exception& e)
{
std::cerr << "捕获到异常: " << e.what() << '\n'; // 处理异常
}
catch (std::out_of_range)
{
printf("check the range 1\n");
}
return 0;
}