文章目录
布局
主轴和交叉轴的概念:
- 对于Column布局而言,主轴是垂直方向,交叉轴是水平方向
- 对于Row布局而言,主轴是水平方向,交叉轴是垂直方向
Column:从上往下的布局
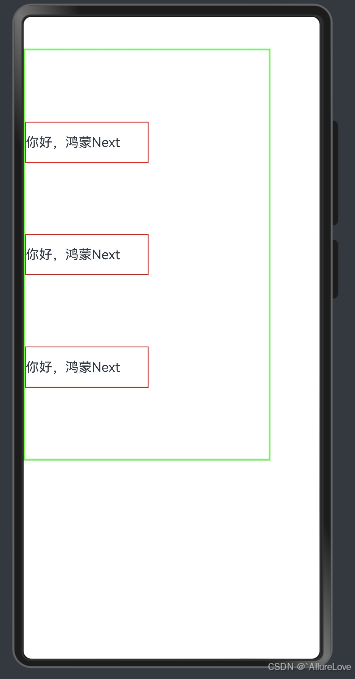
javascript
@Entry
@Component
struct Layouts {
@State message: string = 'Hello World';
build() {
/*
column:从上往下的布局
{space:5}对应每个组件有.margin({top:5})
* 对齐问题:
* 主轴:从上往下的方向
* 交叉轴:横向的为交叉轴
*/
Column({space: 5}) {
Text("你好,鸿蒙Next")
// 组件的基础属性,宽度、高度、边框设置
.width(150)
.height(50)
.border({
width:1,
color: 'red'
})
Text("你好,鸿蒙Next")
.width(150)
.height(50)
.border({
width:1,
color: 'red'
})
Text("你好,鸿蒙Next")
.width(150)
.height(50)
.border({
width:1,
color: 'red'
})
}
.width(300)
.height(500)
.border({color: 'green', width:2})
// 设置交叉轴方向的对齐:Start、Center、End
.alignItems(HorizontalAlign.Start)
// 设置主轴方向的对齐:Start、End、Center、SpaceBetween、SpaceAround、SpaceEvenly
.justifyContent(FlexAlign.SpaceEvenly)
}
}
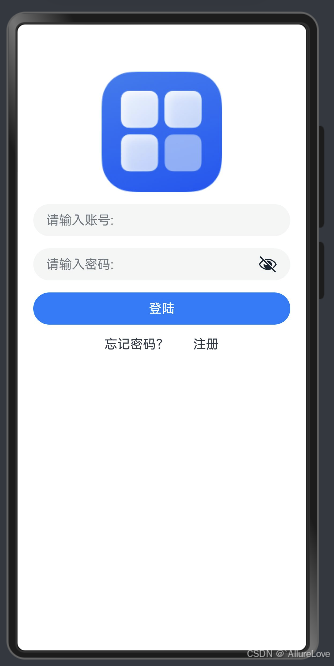
javascript
@Entry
@Component
struct Layouts {
@State message: string = 'Hello World';
build() {
/*
用column实现登陆界面
*/
Column({space:15}) {
Image($r('app.media.startIcon'))
.width(150)
TextInput({placeholder: '请输入账号: '})
TextInput({placeholder: '请输入密码: '})
.type(InputType.Password)
Button('登陆')
// 写函数去实现交互
.onClick(()=>{
// 创建一个对象,可以用字面量来写,需要该进成接口或类
let obj: User = {
name: "",
pwd: ''
}
let user: UserInfo | null = null
AlertDialog.show({ message: JSON.stringify(user) })
})
.width('100%')
Row() {
Text('忘记密码?')
Blank(30)
Text('注册')
}
}
.height('100%')
.width('100%')
.padding(20)
}
}
interface User {
name: string
pwd: string
}
class UserInfo {
name: string = ''
pwd: string = ''
// constructor(name: string, pwd: string) {
// this.name = name;
// this.pwd = pwd;
// }
}
Row:从左往右的布局
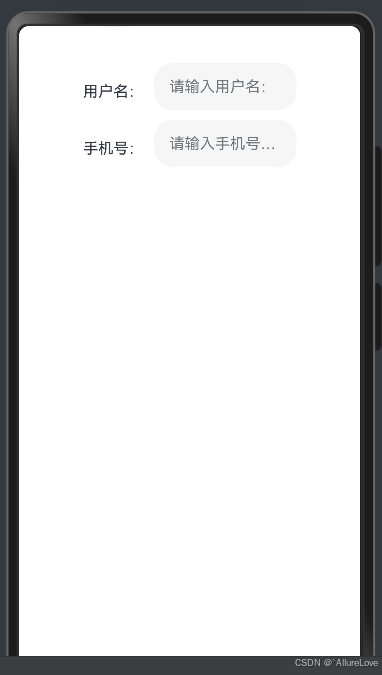
javascript
@Entry
@Component
struct Layouts {
@State message: string = 'Hello World';
build() {
Column() {
/*
Row():行布局,从左往右的布局
* 主轴:水平方向(justifyContent)
* 交叉轴:垂直方向(alignItems)
*/
Row({space:5}) {
Text('用户名:')
.width(70)
.height(60)
TextInput({
placeholder: '请输入用户名:'
})
.width(150)
.height(50)
}
.width('100%')
.justifyContent(FlexAlign.Center)
.alignItems(VerticalAlign.Top)
Row({space:5}) {
Text('手机号:')
.width(70)
.height(60)
TextInput({
placeholder: '请输入手机号码:'
})
.width(150)
.height(50)
}
.width('100%')
// 主轴对齐:Start、Center、End、SpaceBetween、SpaceAround、SpaceEvenly
.justifyContent(FlexAlign.Center)
// 交叉轴对齐:Top、Center、Bottom
.alignItems(VerticalAlign.Top)
}
.height('100%')
.width('100%')
}
}
- 融合示例
javascript
@Entry
@Component
struct Layouts {
@State message: string = 'Hello World';
build() {
Row() {
Column({space: 5}) {
Text('GucciT恤')
Text('价格美丽,上身快乐')
}
.justifyContent(FlexAlign.Start)
.alignItems(HorizontalAlign.Start)
.padding(20)
Row() {
Image($r('app.media.startIcon'))
.width(50)
Image($r('app.media.top_diversion_entrance'))
.width(50)
}
}
.height(70)
.width('100%')
.border({color: '#cccccc', width: 2})
.justifyContent(FlexAlign.SpaceBetween)
.backgroundColor('#eeeeee')
}
}
Stack:堆叠布局
层叠布局的居中设置
javascript
@Entry
@Component
struct Layouts {
@State message: string = '层叠布局';
build() {
/*
Stack():层叠布局
* 可以重叠放置多个
*/
Stack({alignContent: Alignment.Center}) {
Text('1')
.width(400)
.height(400)
.backgroundColor(Color.Green)
// 层级关系设置的属性
.zIndex(3)
Text('2')
.width(300)
.height(300)
.backgroundColor(Color.Blue)
.zIndex(10)
Text('3')
.width(200)
.height(200)
.backgroundColor(Color.Pink)
.zIndex(199)
}
.height('100%')
.width('100%')
}
}
- 页面跳转
javascript
import { router } from '@kit.ArkUI';
@Entry
@Component
struct Layouts {
@State message: string = '层叠布局';
// 定时器执行总时间:@State 变量状态会同步更新
@State time: number = 5
// 定时器句柄
timer: number = -1
// 页面显示之前执行
aboutToAppear(): void {
this.timer = setInterval(()=>{
if (this.time <= 0) {
clearInterval(this.timer)
// 路由功能:倒计时结束后跳转到另一个界面
router.replaceUrl({
url: 'pages/SignPage'
})
}
this.time--
}, 1000)
}
aboutToDisappear(): void {
//清除定时器
clearInterval(this.timer)
}
build() {
/*
Stack():层叠布局
* 可以重叠放置多个
*/
Stack({alignContent: Alignment.TopEnd}) {
Image($r('app.media.startIcon'))
Button('跳过'+ this.time)
.backgroundColor('#ccc')
.fontColor('#fff')
}
.height('100%')
.width('100%')
}
}
Flex:自动换行或列
- 弹性布局的基础设置
javascript
import { router } from '@kit.ArkUI';
@Entry
@Component
struct Layouts {
build() {
/*
Flex():弹性布局
* 也存在主轴和交叉轴的概念,根据direction来决定,默认是Row()行布局
*/
Flex({
direction: FlexDirection.Row,
justifyContent: FlexAlign.SpaceBetween,
alignItems: ItemAlign.Start
}) {
Text('1').width(60).backgroundColor(Color.Yellow)
Text('2').width(60).backgroundColor(Color.Pink)
Text('3').width(60).backgroundColor(Color.Green)
}
.height('100%')
.width('100%')
}
}
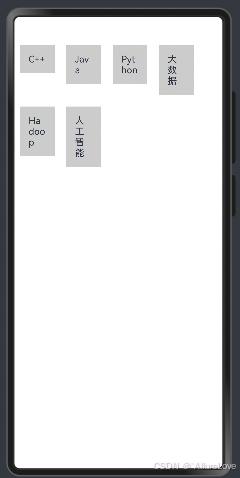
javascript
import { router } from '@kit.ArkUI';
@Entry
@Component
struct Layouts {
build() {
/*
Flex():弹性布局
* 也存在主轴和交叉轴的概念,根据direction来决定,默认是Row()行布局
* 优势:可通过wrap去自动换行
*/
Flex({
direction: FlexDirection.Row,
wrap: FlexWrap.Wrap
}) {
Text('C++').width(60).backgroundColor('#ccc').margin(10).padding(15)
Text('Java').width(60).backgroundColor('#ccc').margin(10).padding(15)
Text('Python').width(60).backgroundColor('#ccc').margin(10).padding(15)
Text('大数据').width(60).backgroundColor('#ccc').margin(10).padding(15)
Text('Hadoop').width(60).backgroundColor('#ccc').margin(10).padding(15)
Text('人工智能').width(60).backgroundColor('#ccc').margin(10).padding(15)
}
.height('100%')
.width('100%')
}
}
组件
Swiper
javascript
@Entry
@Component
struct Layouts {
swipCtl: SwiperController = new SwiperController()
build() {
Column() {
Swiper(this.swipCtl) {
Image($r('app.media.layered_image'))
Image($r('app.media.layered_image'))
Image($r('app.media.layered_image'))
Image($r('app.media.layered_image'))
}
.loop(true)
.autoPlay(true)
.interval(300)
Row() {
Button('<').onClick(()=>{
this.swipCtl.showPrevious()
})
Button('>').onClick(()=>{
this.swipCtl.showNext()
})
}
}
}
}
各种选择组件
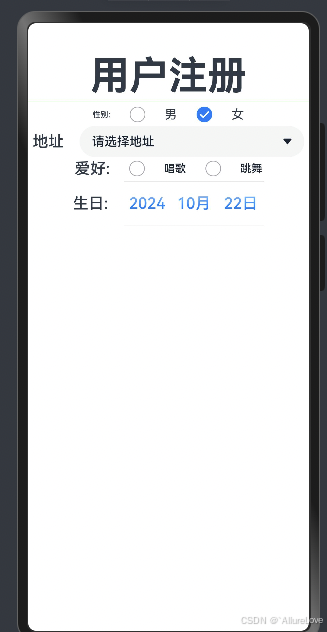
javascript
import { router } from '@kit.ArkUI';
@Entry
@Component
struct Layouts {
@State message: string = '用户注册'
addList: SelectOption[] = [{value:'长沙'}, {value:'广州'}, {value:'上海'}, {value:'北京'}]
build() {
Column() {
Text(this.message)
.id('RegUserPageHelloWorld')
.fontSize(50)
.fontWeight(FontWeight.Bold)
Divider().width('100%').vertical(false).height(5).color(Color.Green)
// 单选组件
Row({space:20}) {
Text('性别:').fontSize(10).fontWeight(FontWeight.Medium)
Radio({value: 'male', group: 'sexGroup'})
.checked(true)
.width(20)
.height(20)
Text('男')
Radio({value: 'female', group: 'sexGroup'})
.checked(true)
.width(20)
.height(20)
Text('女')
}
// 下拉选择框
Row({space: 20}) {
Text('地址').fontSize(20).fontWeight(FontWeight.Medium)
Select(this.addList)
.width('80%')
.selected(2)
.value('请选择地址')
.fontColor('#182431')
.onSelect((index, value)=>{
console.log('ken', index, value)
})
}
// 复选框
Row({space: 20}) {
Text('爱好:').fontSize(20).fontWeight(FontWeight.Medium)
Checkbox({name: 'chkSing', group: "loveGroup"})
Text('唱歌').fontSize(14).fontColor('#182431').fontWeight(500)
Checkbox({name: 'chkDance', group: "loveGroup"}).onChange((value)=>{
//
})
Text('跳舞').fontSize(14).fontColor('#182431').fontWeight(500)
}
// 日期选择框
Row({space: 20}) {
Text('生日:').fontSize(20).fontWeight(FontWeight.Medium)
DatePicker({start: new Date()})
.height(60)
.width('50%')
.onDateChange((value) => {
//
})
}
}
.width('100%')
.height('100%')
}
}
- 更多组件和布局参考官方文档:UI开发(ArkUI)