在本文中,我们将介绍如何编译AWS SDK C++库,以便在您的项目中使用。AWS SDK C++库提供了与Amazon Web Services交互的接口,允许您在C++应用程序中使用AWS服务。
一、准备工作
在开始编译AWS SDK C++库之前,请确保您的系统已经安装了以下依赖项:
CMake(版本3.13或更高版本)
支持C++11的编译器(我是使用的是MSVC 2017 64位)
二、下载AWS SDK C++源代码
首先,您需要从GitHub上的AWS SDK C++存储库下载源代码。您可以通过以下命令克隆存储库:
bash
git clone --recurse-submodules https://github.com/aws/aws-sdk-cpp
cd aws-sdk-cpp
三、配置编译环境
接下来,您需要使用CMake来配置编译环境。这将生成适用于您的系统的构建文件。您可以通过以下命令来配置编译环境:
bash
mkdir <BUILD_DIR>
cd <BUILD_DIR>
# 编译Release版本
cmake ..\aws-sdk-cpp -G "Visual Studio 15 2017 Win64" -DCMAKE_BUILD_TYPE=Release -DBUILD_ONLY="s3"
# 开始编译
cmake --build . --config=Release
# 安装到指定目录,需要管理员权限
cmake --install . --config=Release
cmake --install
会把库文件安装到 C:\Program Files\aws-cpp-sdk-all
目录
四、编译测试代码验证
4.1 创建hello_s3.cpp文件
cpp
#include <aws/core/Aws.h>
#include <aws/s3/S3Client.h>
#include <iostream>
#include <aws/core/auth/AWSCredentialsProviderChain.h>
using namespace Aws;
using namespace Aws::Auth;
/*
* A "Hello S3" starter application which initializes an Amazon Simple Storage Service (Amazon S3) client
* and lists the Amazon S3 buckets in the selected region.
*
* main function
*
* Usage: 'hello_s3'
*
*/
int main(int argc, char **argv) {
Aws::SDKOptions options;
// Optionally change the log level for debugging.
// options.loggingOptions.logLevel = Utils::Logging::LogLevel::Debug;
Aws::InitAPI(options); // Should only be called once.
int result = 0;
{
Aws::Client::ClientConfiguration clientConfig;
// Optional: Set to the AWS Region (overrides config file).
// clientConfig.region = "us-east-1";
// You don't normally have to test that you are authenticated. But the S3 service permits anonymous requests, thus the s3Client will return "success" and 0 buckets even if you are unauthenticated, which can be confusing to a new user.
auto provider = Aws::MakeShared<DefaultAWSCredentialsProviderChain>("alloc-tag");
auto creds = provider->GetAWSCredentials();
if (creds.IsEmpty()) {
std::cerr << "Failed authentication" << std::endl;
}
Aws::S3::S3Client s3Client(clientConfig);
auto outcome = s3Client.ListBuckets();
if (!outcome.IsSuccess()) {
std::cerr << "Failed with error: " << outcome.GetError() << std::endl;
result = 1;
} else {
std::cout << "Found " << outcome.GetResult().GetBuckets().size()
<< " buckets\n";
for (auto &bucket: outcome.GetResult().GetBuckets()) {
std::cout << bucket.GetName() << std::endl;
}
}
}
Aws::ShutdownAPI(options); // Should only be called once.
return result;
}
4.2 创建CmakeLists.txt
shell
# Set the minimum required version of CMake for this project.
cmake_minimum_required(VERSION 3.13)
# Set the AWS service components used by this project.
set(SERVICE_COMPONENTS s3)
# Set this project's name.
project("hello_s3")
# Set the C++ standard to use to build this target.
# At least C++ 11 is required for the AWS SDK for C++.
set(CMAKE_CXX_STANDARD 11)
# Use the MSVC variable to determine if this is a Windows build.
set(WINDOWS_BUILD ${MSVC})
if (WINDOWS_BUILD) # Set the location where CMake can find the installed libraries for the AWS SDK.
string(REPLACE ";" "/aws-cpp-sdk-all;" SYSTEM_MODULE_PATH "${CMAKE_SYSTEM_PREFIX_PATH}/aws-cpp-sdk-all")
list(APPEND CMAKE_PREFIX_PATH ${SYSTEM_MODULE_PATH})
endif ()
# Find the AWS SDK for C++ package.
find_package(AWSSDK REQUIRED COMPONENTS ${SERVICE_COMPONENTS})
if (WINDOWS_BUILD AND AWSSDK_INSTALL_AS_SHARED_LIBS)
# Copy relevant AWS SDK for C++ libraries into the current binary directory for running and debugging.
# set(BIN_SUB_DIR "/Debug") # if you are building from the command line you may need to uncomment this
# and set the proper subdirectory to the executables' location.
AWSSDK_CPY_DYN_LIBS(SERVICE_COMPONENTS "" ${CMAKE_CURRENT_BINARY_DIR}${BIN_SUB_DIR})
endif ()
add_executable(${PROJECT_NAME}
hello_s3.cpp)
target_link_libraries(${PROJECT_NAME}
${AWSSDK_LINK_LIBRARIES})
4.3 使用cmake构建
shell
mkdir my_project_build
cd my_project_build
cmake ../
# 然后运行hello_s3
./hello_s3
hello_s3会打印出所有bucket名字,前提是已经配置了AWS的凭证。
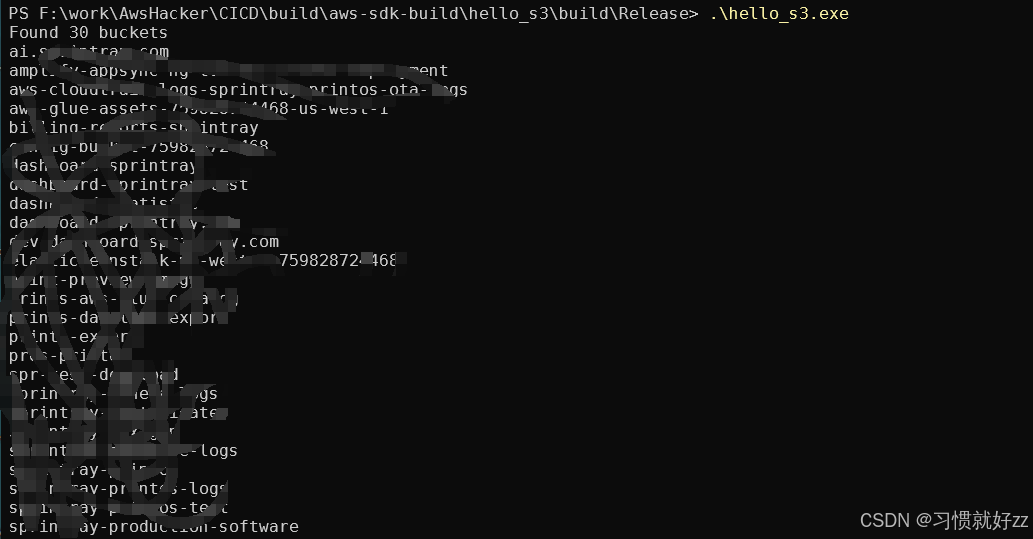