目录
最近在做项目的过程中发现,props父子通信忘的差不多了。下面写个笔记复习一下。
1.父传子
父组件(FatherComponent.vue):
html
<script setup>
import ChildComponent from "@/components/ChildComponent.vue"
import { ref } from "vue"
const fatherMoney = ref(1000)
</script>
<template>
<div class="bg-blue h-75 w-100 ma-auto">
<h1 class="text-center">我是父组件</h1>
<ChildComponent :money="fatherMoney"></ChildComponent>
</div>
</template>
我们可以在子组件标签上写:money="fatherMoney"
。意思就是把父亲的响应式变量fatherMoney
给子组件,子组件在组件内部要用money
来接受这个变量。
子组件(ChildComponent.vue):
html
<script setup>
const props = defineProps(['money','updateMoney'])
</script>
<template>
<div class="bg-purple h-50 w-75 ma-auto">
<h1 class="text-center">我是子组件</h1>
<h3>父亲给我的钱:{{money}}元</h3>
</div>
</template>
子组件<h3>父亲给我的钱:{``{money}}元</h3>
这一块儿,我们可以用props.money
来渲染这个数据,也可以省略props,直接写money
。
注意,用props来接受的数据是只读的,子组件不能再组件内部更改它。
比如,不能下面这样写,否则控制台会报错:
html
<script setup>
const props = defineProps(['money'])
const updateMoney = () => {
props.money = 100
}
</script>
<template>
<div class="bg-purple h-50 w-75 ma-auto">
<h1 class="text-center">我是子组件</h1>
<h3>父亲给我的钱:{{money}}元</h3>
<v-btn @click="updateMoney" class="text-white bg-blue">修改父亲给我的钱</v-btn>
</div>
</template>
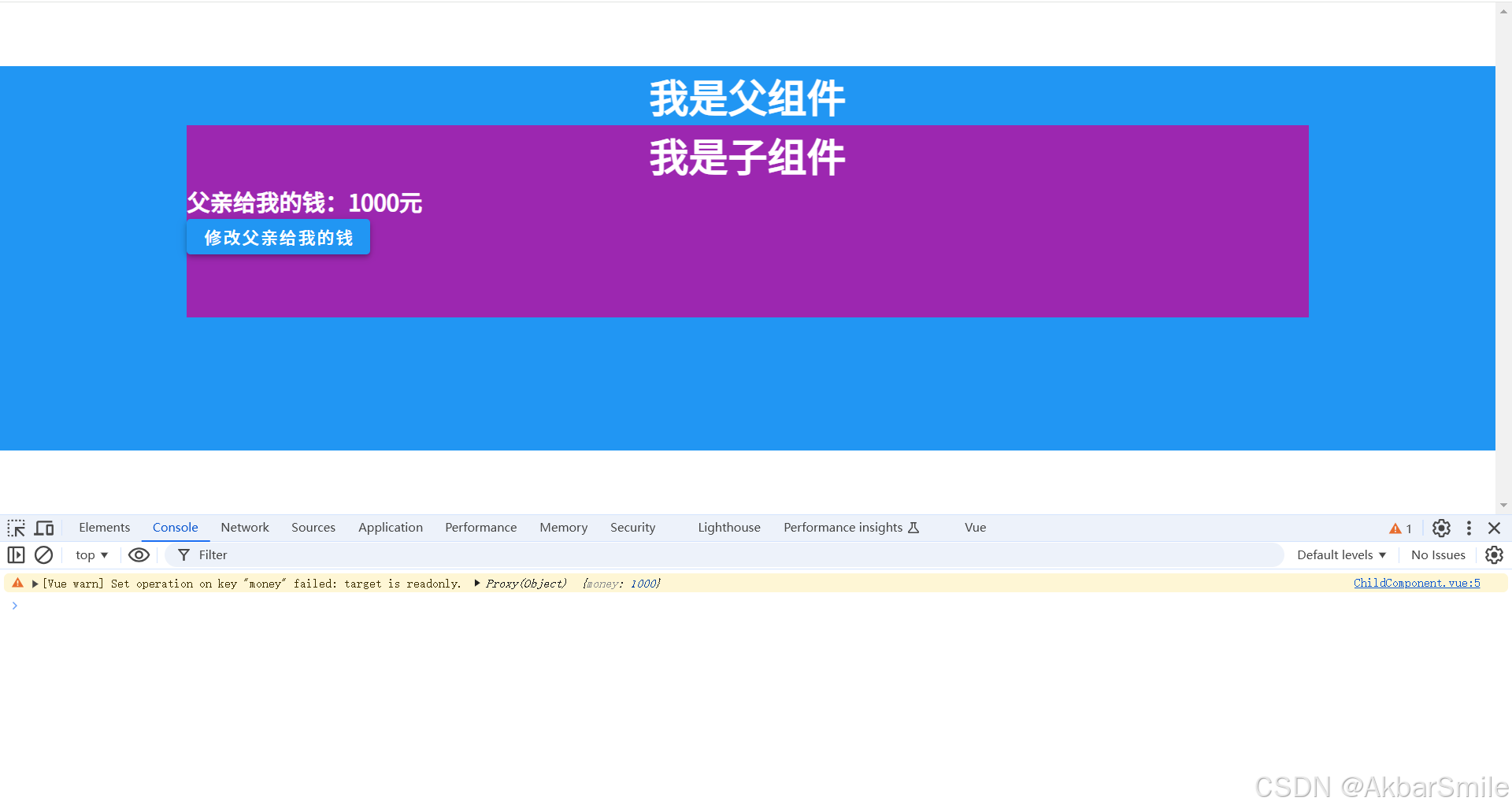
2.子传父
子组件向父组件发送数据,父组件需要定义一个方法,用来接受子组件发送的数据:
父组件(FatherComponent.vue):
html
<script setup>
import ChildComponent from "@/components/ChildComponent.vue"
import { ref } from "vue"
const fatherMoney = ref(1000)
const childToy = ref('')
const getToy = (value)=>{
childToy.value = value
}
</script>
<template>
<div class="bg-blue h-75 w-100 ma-auto">
<h1 class="text-center">我是父组件</h1>
<h3>儿子给我的玩具:{{childToy}}</h3>
<ChildComponent :money="fatherMoney" :sendToy="getToy"></ChildComponent>
</div>
</template>
:sendToy="getToy"
意思就是,父组件给子组件传递了一个方法getToy
,子组件要用方法sendToy
,给父亲发送数据。
子组件(ChildComponent.vue):
html
<script setup>
import {ref} from "vue"
const props = defineProps(['money','sendToy'])
const toy = ref('奥特曼')
</script>
<template>
<div class="bg-purple h-50 w-75 ma-auto">
<h1 class="text-center">我是子组件</h1>
<h3>父亲给我的钱:{{money}}元</h3>
<v-btn @click="sendToy(toy)" class="text-white bg-blue">把玩具给父亲</v-btn>
<h3>儿子的玩具:{{toy}}</h3>
</div>
</template>
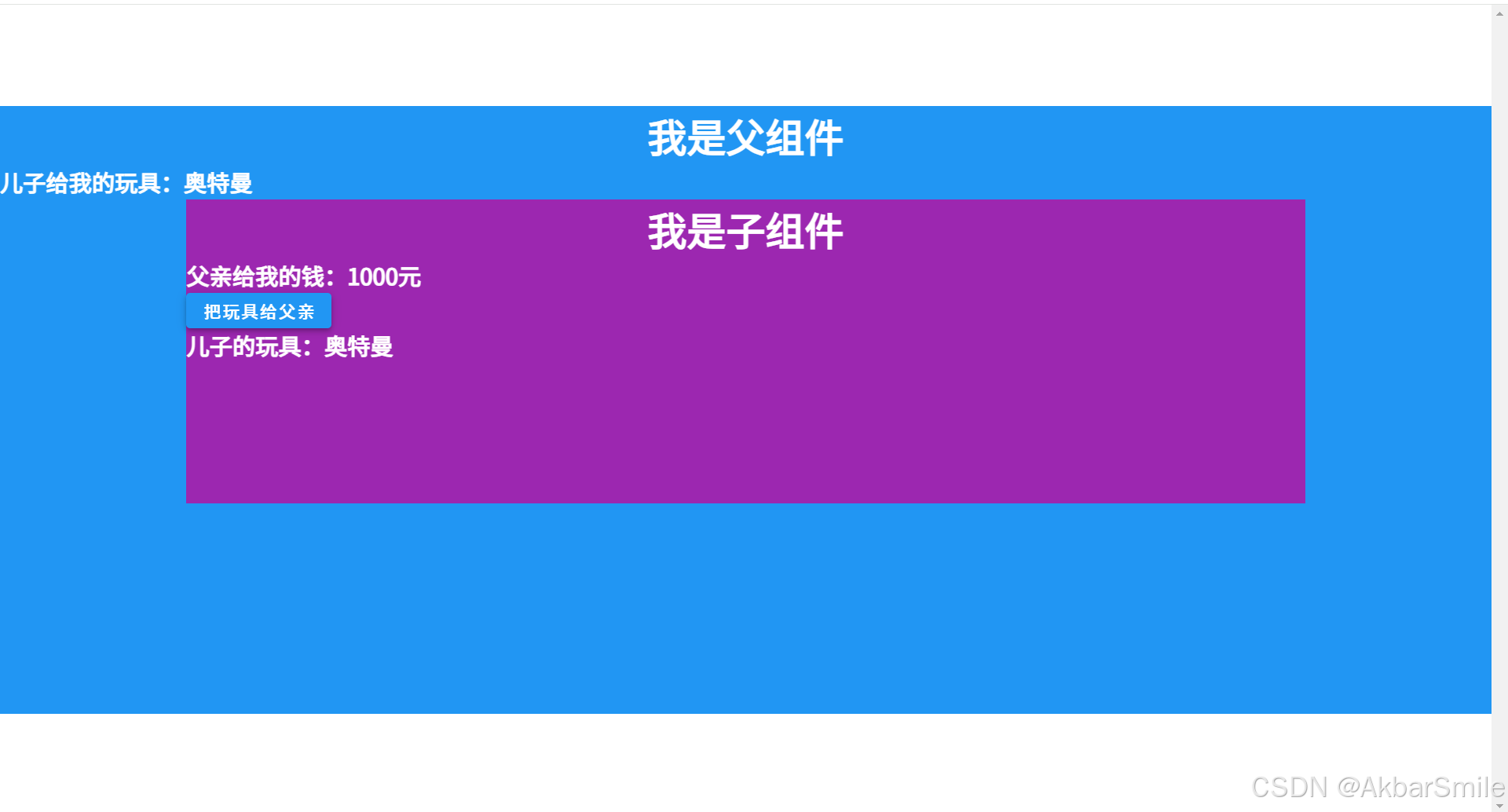