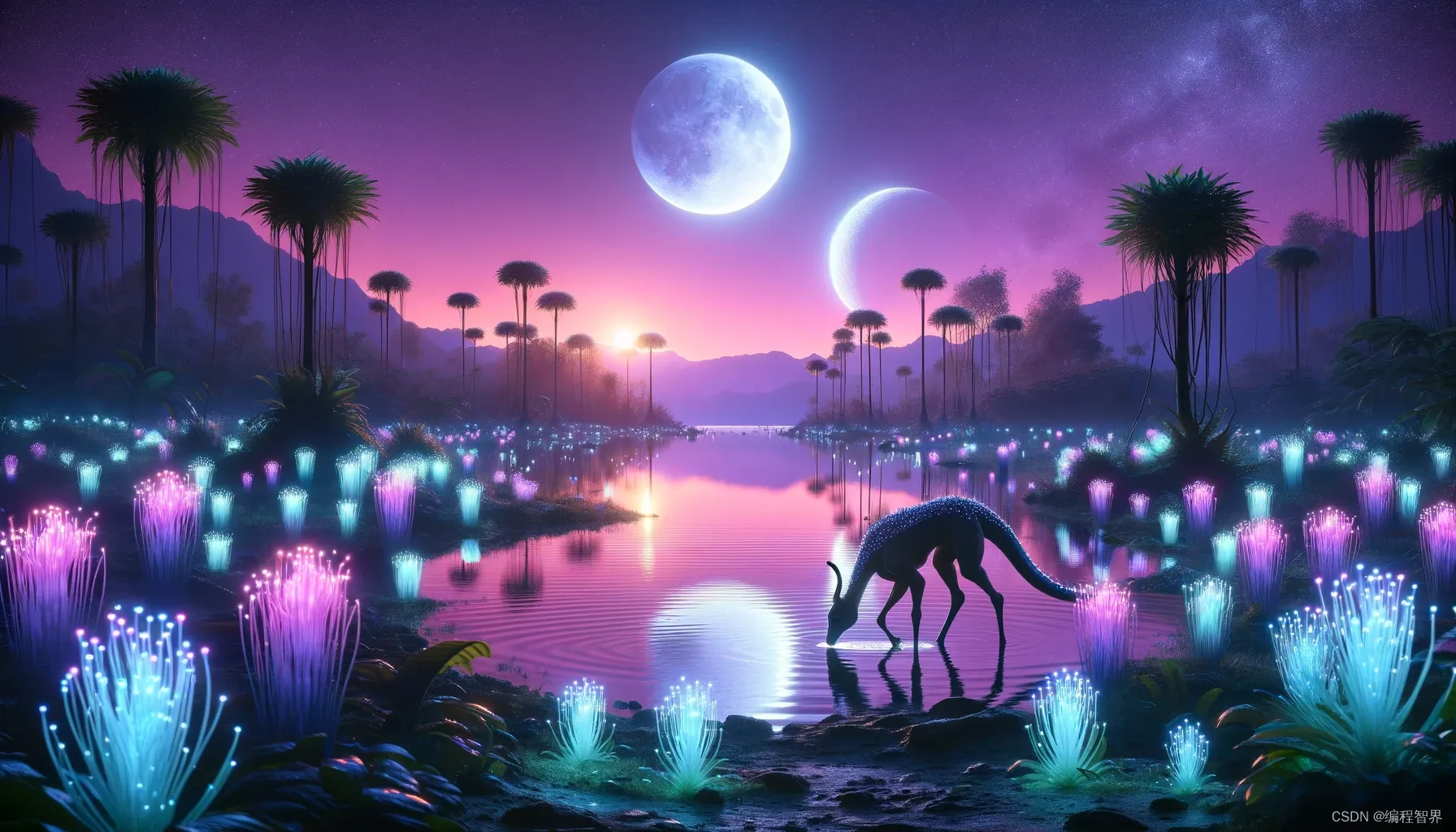
现代C++(特别是C++11及以后的版本)增强了对编译时常量求值的支持,包括constexpr
函数、constinit
和consteval
关键字。这些特性允许在编译时进行更多的计算,有助于优化运行时性能并确保编译时的数据不变性。
1. constexpr
- 编译时常量表达式
constexpr
指示编译器尽可能在编译时求值。
cpp
constexpr int factorial(int n) {
return (n <= 1) ? 1 : (n * factorial(n - 1));
}
constexpr int val = factorial(5); // 编译时计算5的阶乘
void constexprExample() {
static_assert(val == 120, "Factorial of 5 should be 120");
}
2. consteval
- 强制编译时求值
C++20引入的consteval
确保函数必须在编译时求值。
cpp
consteval int square(int n) {
return n * n;
}
constexpr int squaredVal = square(5); // 编译时计算5的平方
void constevalExample() {
static_assert(squaredVal == 25, "Square of 5 should be 25");
// int runtimeVal = square(6); // 错误:consteval函数必须在编译时求值
}
3. constinit
- 确保静态和线程局部变量在编译时初始化
constinit
确保变量在编译时初始化,但与constexpr
不同,它不要求变量在其生命周期内都是常量。
cpp
constinit static int staticVal = factorial(4); // 编译时计算4的阶乘
void constinitExample() {
static_assert(staticVal == 24, "Factorial of 4 should be 24");
// staticVal = 5; // 允许在运行时修改值
}
4. 编译时与运行时的区分
通过使用这些特性,开发者可以明确地指示哪些计算应该在编译时进行,哪些在运行时进行,提高代码的可预测性和效率。
cpp
constexpr int compileTimeCalculation = factorial(5); // 编译时求值
int runtimeCalculation = factorial(5); // 运行时求值
void compileTimeVsRuntime() {
static_assert(compileTimeCalculation == 120, "Compile-time and runtime results should match");
std::cout << "Runtime calculation: " << runtimeCalculation << std::endl;
}