文章目录
检测环境
laravel 的环境配置
安装两个插件
增
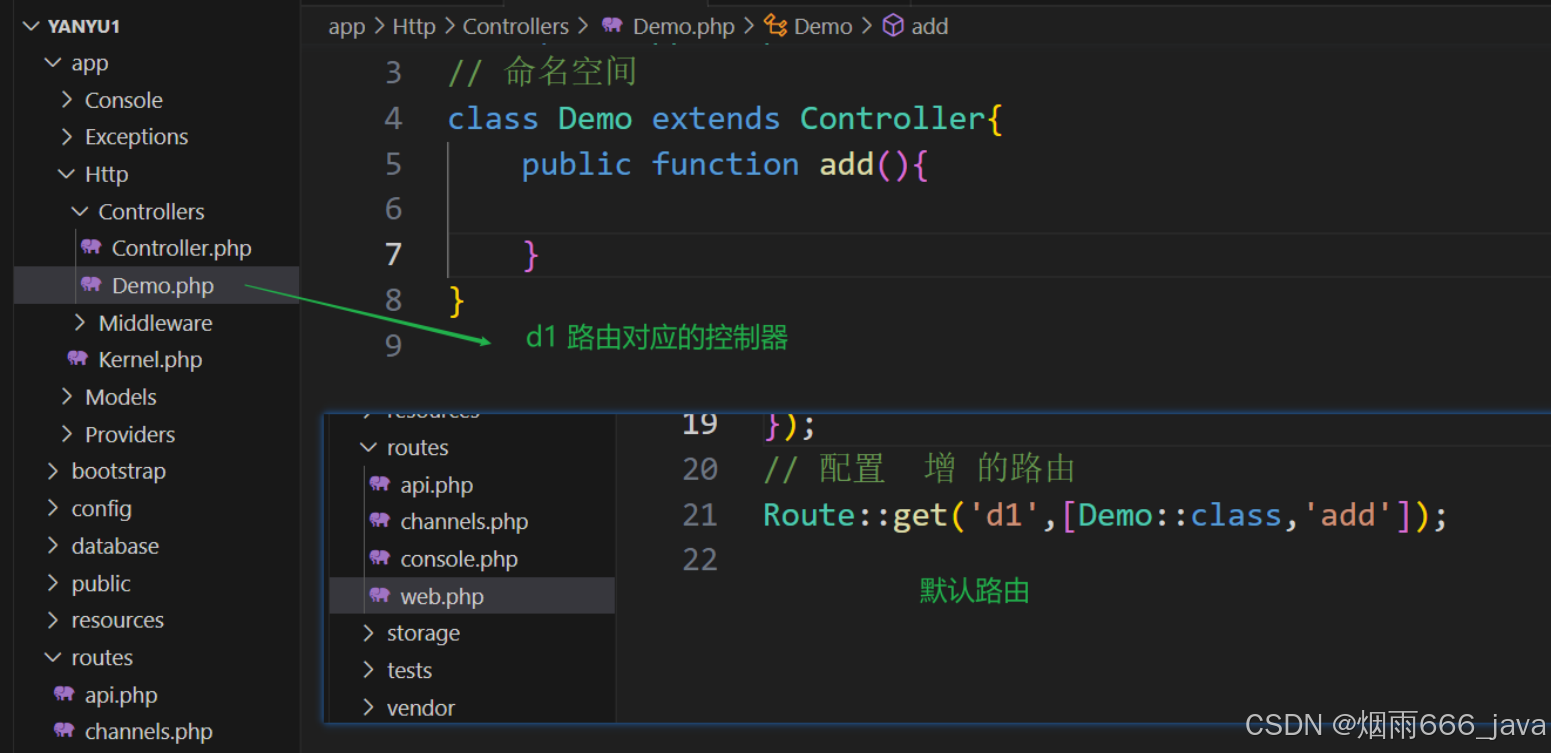
编写SQL插入语句
php
<?php
namespace App\Http\Controllers;
use Illuminate\Support\Facades\DB;
// 命名空间
class Demo extends Controller{
public function add(){
// 插入一行数据
DB::table('user')
->insert(['name'=>'yyy','age'=>11]);
// 批量插入数据
DB::table('user')
->insert([
['name'=>'烟雨3','age'=>22],
['name'=>'烟雨2','age'=>20]
]
);
}
}
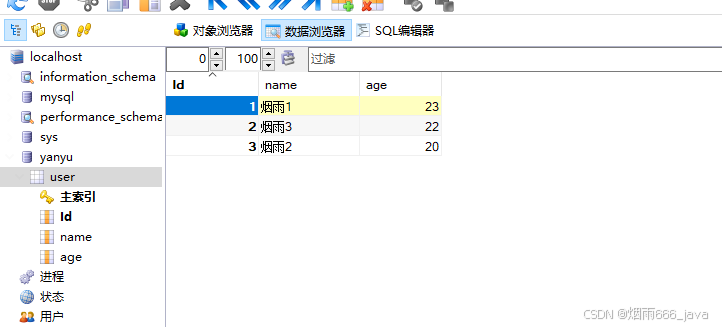
建库建表----建工程 --- 改 .env 环境 ---- 路由 -- 控制器
改
php
public function update(){
DB::table('user')
->update(['age'=>10]);
// 没有 where 限定 ,就会把所有的 age 改成 10
}
php
Route::get('d2',[Demo::class,'update']);
没有where限定,导致所有人的age 都被更新为 10
where 限定
php
public function update(){
// DB::table('user')
// ->update(['age'=>10]);
// 没有 where 限定 ,就会把所有的 age 改成 10
// where 限定 name = yyy 把名字 改为 mmm
DB::table('user')
->where('name','=','yyy')
->update(['name'=> 'mmm']);
}
php
Route::get('d2',[Demo::class,'update']);
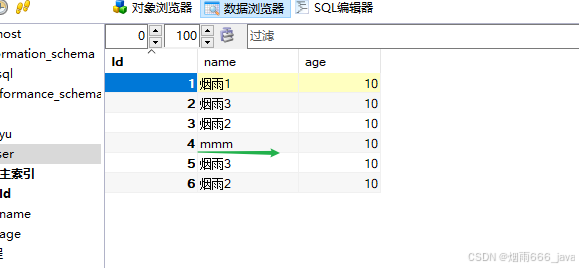
更新 或者 插入
第一个参数存在,则作为 where 限定,去更新更新第二个参数,执行的是更新
第一个参数不存在,则把第一个第二个参数 插入到 表中,执行的是 插入操作
php
public function update(){
// DB::table('user')
// ->update(['age'=>10]);
// 没有 where 限定 ,就会把所有的 age 改成 10
// where 限定 name = yyy 把名字 改为 mmm
// DB::table('user')
// ->where('name','=','yyy')
// ->update(['name'=> 'mmm']);
DB::table('user')
->updateOrInsert(
// 参数1 ['name'=>'mmm']
// 参数2 ['age'=>2]
['name'=>'mmm'],
['age'=>2]
);
}
删
php
public function delete(){
DB::table('user')
->where('age','>',10)
->delete();
}
php
Route::get('d3',[Demo::class,'delete']);
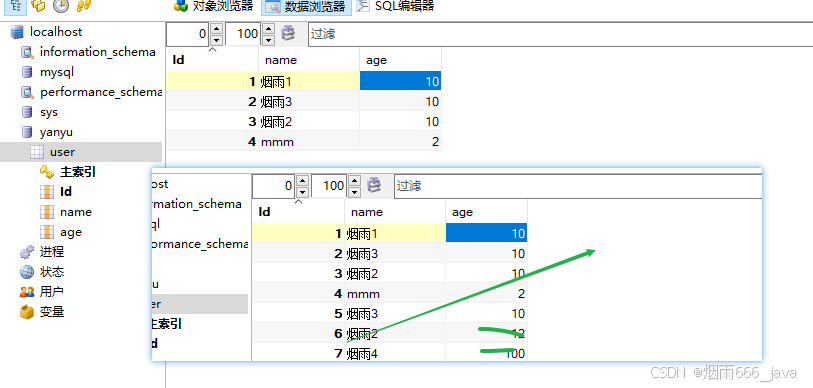
delete 删除前,id 最大 = 7,重新插入数据,id 从 8 开始
php
public function delete(){
// DB::table('user')
// ->where('age','>',10)
// ->delete();
DB::table('user')->truncate();
// 针对整个表操作,无法像 delete 一样,限定范围 删除
// 删除数据,保留表的结构,重置 id ,重新插入数据。id 从 1 开始递增
}
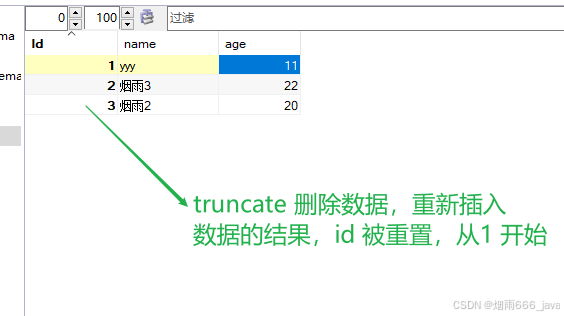
事务控制
php
public function add()
{
DB::transaction(
// transaction( 放置一个匿名函数(把多个数据库操作放在改匿名函数的函数体之内) )
function () {
// 插入一行数据
DB::table('user')
->insert(['name' => 'yyy', 'age' => 11]);
// 批量插入数据
DB::table('user')
->insert(
[
['name' => '烟雨3', 'age' => 22],
['name' => '烟雨2', 'age' => 20]
]
);
}
);
}