一、引言
首先我们应该回顾动态内存开辟的原理 这部分知识对于内存访问至关重要 然而顺序表的实现都是基于C语言的基础 包括指针 结构体 动态内存开辟 realloc malloc h函数的使用与实现
既然要学习顺序表 我们不仅要知道这个实现是基于C语言知识的基础 我们还要知道什么是表 应该先知道线性表
二、线性表
线性表(linear list)是n个具有相同特性的数据元素的有限序列 。 线性表是一种在实际中广泛使用的数据结构,常见的线性表:顺序表、链表、栈、队列、字符串...
线性表在逻辑上是线性结构,也就说是连续的一条直线。但是在物理结构上并不一定是连续的,线性表在物理上存储时,通常以数组和链式结构的形式存储
三、顺序表
顺序表是用一段物理地址连续 的存储单元依次存储数据元素的线性结构,一般情况下采用数组存储。在数组上完成数据的增删查改。
顺序表一般可以分为:
-
静态顺序表:使用定长数组存储元素。
-
静态顺序表:使用动态开辟的数组存储。
接下来我们通过代码实现顺序表的各种功能 。
1.静态顺序表与动态顺序表 对比
cs
//静态顺序表
typedef int SLDataType;
#define N 1000
typedef struct SeqList
{
SLDataType a[N];
int size;//有效数据个数
}SL;
cs
typedef int SLDataType;
#define INIT_CAPACITY 4
typedef struct SeqList
{
SLDataType* a;
int size;//有效数据个数
int capacity;//空间容量
}SL;
设置成动态的顺序表好处是 可以按需申请空间 相比于静态顺序表 会导致开少了不够用开多了浪费 SLDataType* a ; 这个就可以看作是int*a 由于typedef 定义的是int 4个字节 因为我们创建的是一个表 不能直接指明是什么类型 只能我们自己定义 定义结构体 定义我们所需的 变量
2.顺序表的初始化
cs
void SLInit(SL* ps)
{
assert(ps);
ps->a = (SLDataType*)malloc(sizeof(SLDataType) * INIT_CAPACITY);//开辟内存空间
if (ps->a == NULL)
{
perror("malloc fail");//显示错误原因
return;
}
ps->size = 0;
ps->capacity = INIT_CAPACITY;
}
3.顺序表的销毁
将里面的元素置为空 里面的有效空间个数 和有效的数据个数 置为0
cs
void SLDestroy(SL* ps)
{
assert(ps);
free(ps->a);
ps->a = NULL;
ps->capacity = ps->size = 0;
}
4.顺序表的打印
通过循环遍历的方式 打印顺序表中的元素个数
SLDataType* a ; 指针就是数组
cs
void SLPrint(SL*ps)
{
assert(ps);
for (int i = 0; i < ps->size; i++)
{
printf("%d ", ps->a[i]);
}
}
5.顺序表的检查
主要检查顺序表是否满足扩容的空间
realloc与malloc函数的区别
realloc函数的出现让动态内存管理更加灵活。
有时会我们发现过去申请的空间太小了,有时候我们又会觉得申请的空间过大了,那为了合理的时
候内存,我们一定会对内存的大小做灵活的调整。那 realloc 函数就可以做到对动态开辟内存大小
的调整。
void* realloc (void* ptr, size_t size);
函数原型如下:
ptr 是要调整的内存地址
size 调整之后新大小
返回值为调整之后的内存起始位置。
这个函数调整原内存空间大小的基础上,还会将原来内存中的数据移动到 新 的空间。
realloc在调整内存空间的是存在两种情况:
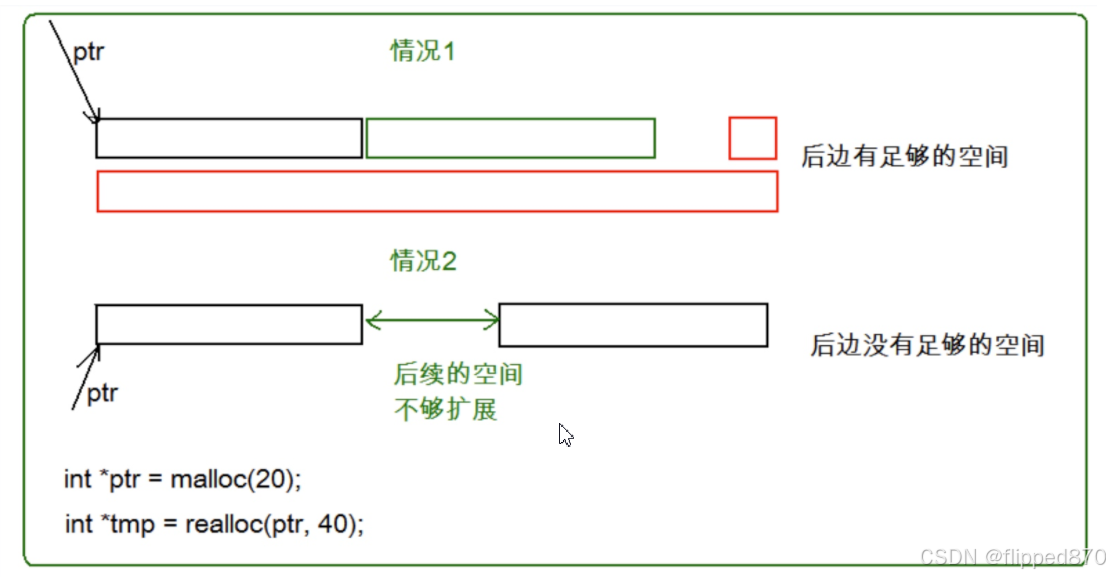
情况1
当是情况1 的时候,要扩展内存就直接原有内存之后直接追加空间,原来空间的数据不发生变化 。
情况2
当是情况2 的时候,原有空间之后没有足够多的空间时,扩展的方法是:在堆空间上另找一个合适大小的连续空间来使用。这样函数返回的是一个新的内存地址。
malloc主要用来直接开辟一块空间
realloc主要用来扩容,就是开辟一块新的空间,将之前的空间释放掉 realloc扩容分为原地扩容 就是 后面的空间还有 异地扩容没有连续的空间 被被人占用了
注意 这种情况下的扩容就是将原来空间里的内容放到新的空间 然后把之间没扩容的空间销毁掉 注意 这里的销毁不是真的销毁 而是把这些内存空间还给操作系统
cs
void SLCheckCapacity(SL* ps)
{
assert(ps);
if (ps->size == ps->capacity)
{
SLDataType* tmp = (SLDataType*)relloc(ps->a, sizeof(SLDataType)*ps->capacity * 2);
if (tmp == NULL)
{
perror("realloc fail");
return;
}
ps->a = tmp;
ps->capacity *= 2;
}
}
6.顺序表的尾插
ps->a[ps->size] = x; ps是结构体指针,访问a之后,a是一个数组,可以通过下标去访问,ps还有一个成员是size,作为下标访问之后,将x的值赋值给这个元素
cs
void SLPushBack(SL* ps, SLDataType x)
{
assert(ps);
//扩容
SLCheckCapacity(ps);
ps->a[ps->size] = x;
ps->size++;
ps->a[ps->size++] = x;
}
7.顺序表的尾删
cs
void SLPopBack(SL* ps)
{
assert(ps);
assert(ps->size > 0);
ps->size--;
}
8.顺序表的头插
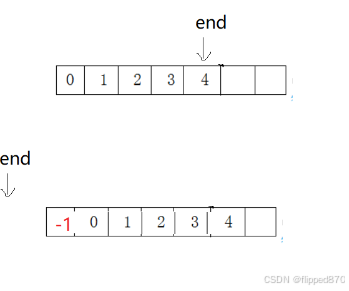
cs
void SLPushFront(SL* ps, SLDataType x)
{
assert(ps);
SLCheckCapacity(ps);
int end = ps->size - 1;
while (end >= 0)
{
ps->a[end + 1] = ps->a[end];
--end;
}
ps->a[0] = x;
ps->size++;
}
9.顺序表的头删
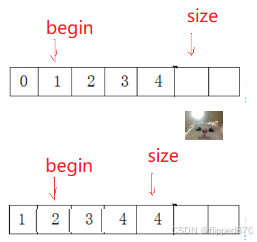
cs
void SLPopFront(SL* ps)
{
assert(ps);
assert(ps->size > 0);
int begin = 1;
while (begin < ps->size)
{
ps->a[begin - 1] = ps->a[begin];
begin++;
}
}
10.顺序表的插入
解释 :顺序表的元素插入和插队是一个意思的。想象一下,有一个人要插队,他要插到第3个位置去,那么他前面的两个人不用动,而他后面的人都得动。具体步骤是:最后面的那个人后退一个位置,倒数第二个人后退到原来最后一个人的位置,这样子后面的每个人依次后退,最后就空出来了一个位置,这个人就插队进去了。顺序表也是这么插入的。有效元素size+1
元素插入有一些要求:
1.元素下标是否越界(有没有插队到奇怪的位置)
2.顺序表存储空间是否满了(有没有位置让shi是你插队)
size - 1是有效数组的下一个位置
cs
void SLInsert(SL* ps, int pos, SLDataType x)
{
assert(ps);
assert(pos>=0&&pos<=ps->size);
SLCheckCapacity(ps);
int end = ps->size - 1;
while (end >= pos)
{
ps->a[end + 1] = ps->a[end];
--end;
}
ps->a[pos] = x;
ps->size++;
}
11.顺序表的删除
删除和插入的操作类型,比如一群人在排队,有一个人有事临时走了,那么这个人的位置就空出来了,后面的人就一个个往前一步,补上这个空位。
元素删除有一些要求:
1.元素下标是否越界(走的人是不是这个排队里面的人)
2.顺序表存储空间是否为空(有没有人可以走)
cs
void SLErase(SL* ps, int pos)
{
assert(ps);
assert(pos >= 0 && pos<ps->size);
int begin = pos + 1;
while(begin<ps->size)
{
ps->a[begin - 1] = ps->a[begin];
begin++;
}
ps->size--;
}
12.顺序表的查找
顺序表又随机存取功能 可以通过直接利用数组下标查找
//
cs
int SLFind(SL* ps, SLDataType x)
{
assert(ps);
for (int i = 0; i < ps->size; i++)
{
if (ps->a[i] == x)
{
return i;
}
}
return -1;
}
四、顺序表的完整版代码
++其中头插 尾插 头删 尾删 这些内容都在插入删除中 都可被替代++
代码的实现是在VS2022编译器下完成的 首先我们要创建3个文件夹 逻辑的测试text .c 顺序表的实现SeqList.h SeqList.c
cs
#define _CRT_SECURE_NO_WARNINGS
#include"SeqList.h"
void SLInit(SL* ps)
{
assert(ps);
ps->a = (SLDataType*)malloc(sizeof(SLDataType) * INIT_CAPACITY);//开辟内存空间
if (ps->a == NULL)
{
perror("malloc fail");//显示错误原因
return;
}
ps->size = 0;
ps->capacity = INIT_CAPACITY;
}
void SLDestroy(SL* ps)
{
assert(ps);
free(ps->a);
ps->a = NULL;
ps->capacity = ps->size = 0;
}
void SLPrint(SL*ps)
{
assert(ps);
for (int i = 0; i < ps->size; i++)
{
printf("%d ", ps->a[i]);
}
}
void SLCheckCapacity(SL* ps)
{
assert(ps);
if (ps->size == ps->capacity)
{
SLDataType* tmp = (SLDataType*)realloc(ps->a, sizeof(SLDataType)*ps->capacity * 2);
//
if (tmp == NULL)
{
perror("realloc fail");
return;
}
ps->a = tmp;
ps->capacity *= 2;
}
}
void SLPushBack(SL* ps, SLDataType x)
{
assert(ps);
//扩容
SLCheckCapacity(ps);
ps->a[ps->size] = x;
ps->size++;
ps->a[ps->size++] = x;
}
void SLPopBack(SL* ps)
{
assert(ps);
assert(ps->size > 0);
ps->size--;
}
void SLPushFront(SL* ps, SLDataType x)
{
assert(ps);
SLCheckCapacity(ps);
int end = ps->size - 1;
while (end >= 0)
{
ps->a[end + 1] = ps->a[end];
--end;
}
ps->a[0] = x;
ps->size++;
}
void SLPopFront(SL* ps)
{
assert(ps);
assert(ps->size > 0);
int begin = 1;
while (begin < ps->size)
{
ps->a[begin - 1] = ps->a[begin];
begin++;
}
}
void SLInsert(SL* ps, int pos, SLDataType x)
{
assert(ps);
assert(pos>=0&&pos<=ps->size);
SLCheckCapacity(ps);
int end = ps->size - 1;
while (end >= pos)
{
ps->a[end + 1] = ps->a[end];
--end;
}
ps->a[pos] = x;
ps->size++;
}
void SLErase(SL* ps, int pos)
{
assert(ps);
assert(pos >= 0 && pos<ps->size);
int begin = pos + 1;
while(begin<ps->size)
{
ps->a[begin - 1] = ps->a[begin];
begin++;
}
}
int SLFind(SL* ps, SLDataType x)
{
assert(ps);
for (int i = 0; i < ps->size; i++)
{
if (ps->a[i] == x)
{
return i;
}
}
return -1;
}
cs
#include<stdio.h>
#include<stdlib.h>
#include<assert.h>
typedef int SLDataType;
#define INIT_CAPACITY 4
#define N 1000
//动态顺序表
typedef struct SeqList
{
SLDataType* a;
int size;//有效数据个数
int capacity;//空间容量
}SL;
//
//typedef struct SeqList
//{
// SLDataType a[N];
// int size;//有效数据个数
//
//}SL;
void SLInit(SL* ps);
void SLDestroy(SL* ps);
void SLPrint(SL* ps);
void SLCheckCapacity(SL* ps);
void SLPushBack(SL* ps, SLDataType x);
void SLPopBack(SL* ps);
void SLPushFront(SL* ps, SLDataType x);
void SLPopFront(SL* ps);
void SLInsert(SL* ps, int pos, SLDataType x);
void SLErase(SL* ps, int pos);
int SLFind(SL* ps, SLDataType x);
时间复杂度 和头删 头插 尾删 尾插
要考虑最坏的情况, 单独插入的话,头插,需要挪动n个元素,实践复杂度为O(n)