文章目录
- 【例6-2】编写选课程序。利用利用列表框和组合框增加和删除相关课程,并统计学时数
-
-
- [1. 表6-2 属性设置](#1. 表6-2 属性设置)
- [2. 设计窗体及页面](#2. 设计窗体及页面)
- [3. 代码实现](#3. 代码实现)
- [4. 运行效果](#4. 运行效果)
-
【例6-2】编写选课程序。利用利用列表框和组合框增加和删除相关课程,并统计学时数
分析:
(1)组合框comboBox1 的选项在窗体载入事件Form1_Load中用Items.Add方法添加。
(2)加入按钮把listBox1 选中项利用Items,Add方法添加到listBox1的列表中。
(3)删除按钮把listBox1选中项利用Items,Remove删除。
(4)程序中使用类Course来定义课程,包含课程名和学时数域。
以下是一个 Windows Forms 应用程序的界面设计属性设置表。
1. 表6-2 属性设置
控件名称 | 属性名称 | 属性值 | 控件名称 | 属性名称 | 属性值 |
---|---|---|---|---|---|
Form1 | Text | 选课程序 | button2 | Text | 删除 |
label1 | Text | 请选择课程 | button2 | TextAlign | MiddleRight |
comboBox1 | DropDownStyle | DropDownList | button2 | Image | del.jpg |
button1 | Text | 加入 | button2 | ImageAlign | MiddleLeft |
button1 | TextAlign | MiddleRight | listBox1 | Name | listBox1 |
button1 | Image | add.jpg | label2 | Text | 总课时: |
textBox1 | ReadOnly | True |
表格中的 "Forml" 应该是 "Form1" 的笔误。此外,Image
属性的文件类型是 image/jpeg
,但在这个表格中并没有特别指出,只是提到了文件名。
这个表格描述了一个简单的选课程序界面,其中包含一个下拉列表(comboBox1
),两个按钮(button1
和 button2
),一个列表框(listBox1
),两个标签(label1
和 label2
),以及一个文本框(textBox1
)。每个控件都有特定的属性设置,以定义它们在界面上的外观和行为。例如,button1
有一个添加课程的图片(add.jpg
),而 button2
有一个删除课程的图片(del.jpg
)。textBox1
被设置为只读,用于显示总课时。
2. 设计窗体及页面
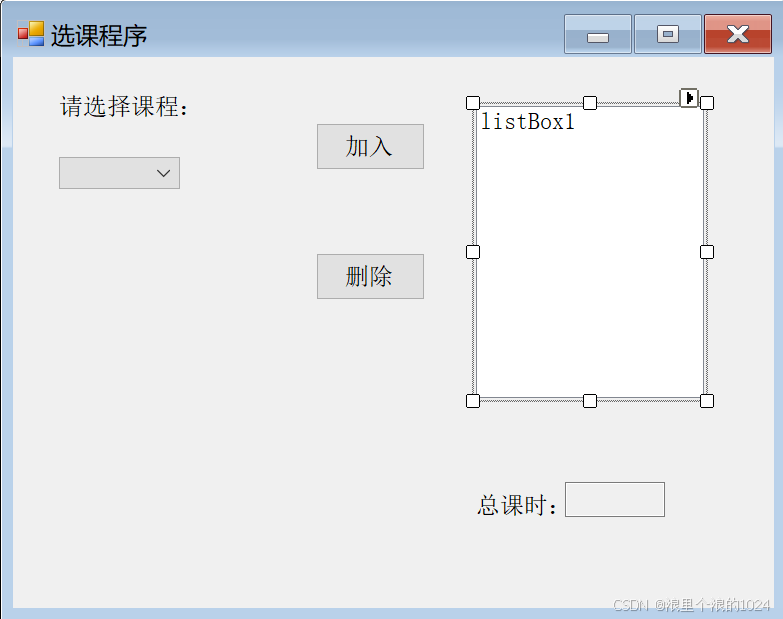
3. 代码实现
csharp
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Pages_127__例6_2_选课程序
{
public partial class Form1 : Form
{
private int totalHours = 0; // 用于存储已选课程的总学分
// 构造函数,初始化窗体
public Form1()
{
InitializeComponent(); // 初始化窗体上的控件
}
// 当窗体加载时,初始化课程数据并添加到ComboBox控件中
private void Form1_Load(object sender, EventArgs e)
{
// 初始化课程数组
Course[] courses = new Course[7] {
new Course("大学英语", 50),
new Course("高等数学", 55),
new Course("数理统计", 35),
new Course("大学物理", 60),
new Course("电子电工", 25),
new Course("计算机应用基础", 65),
new Course("C语言程序设计", 80)
};
// 将课程添加到 ComboBox
foreach (Course course in courses)
{
comboBox1.Items.Add(course); // 将每个课程对象添加到ComboBox的Items集合中
}
}
// Course类定义,用于表示课程信息
public class Course
{
public string Name; // 课程名称
public int Hours; // 课程学分
// 构造函数,用于创建Course对象
public Course(string name, int hours)
{
Name = name;
Hours = hours;
}
// 重写ToString方法,用于返回课程名称和学分的字符串表示
public override string ToString()
{
return Name + " (" + Hours + " 学分)";
}
}
// 当ComboBox的选中项发生变化时,更新TextBox显示的学分
private void comboBox1_SelectedIndexChanged(object sender, EventArgs e)
{
// 获取选中的课程
if (comboBox1.SelectedItem is Course selectedCourse)
{
// 更新 textBox1 的文本为选中课程的学分
textBox1.Text = selectedCourse.Hours.ToString();
}
}
// 当点击添加按钮时,将选中的课程添加到ListBox中,并更新总学分
private void button1_Click(object sender, EventArgs e)
{
if (comboBox1.SelectedItem is Course selectedCourse)
{
if (!listBox1.Items.Contains(selectedCourse))
{
listBox1.Items.Add(selectedCourse); // 将选中的课程添加到ListBox中
totalHours += selectedCourse.Hours; // 更新总学分
textBox1.Text = totalHours.ToString(); // 更新TextBox显示的总学分
}
}
}
// 当点击删除按钮时,从ListBox中移除选中的课程,并更新总学分
private void button2_Click(object sender, EventArgs e)
{
if (listBox1.SelectedItem is Course selectedCourse && listBox1.SelectedIndex != -1)
{
listBox1.Items.Remove(selectedCourse); // 从ListBox中移除选中的课程
totalHours -= selectedCourse.Hours; // 更新总学分
textBox1.Text = totalHours.ToString(); // 更新TextBox显示的总学分
}
}
}
}
4. 运行效果
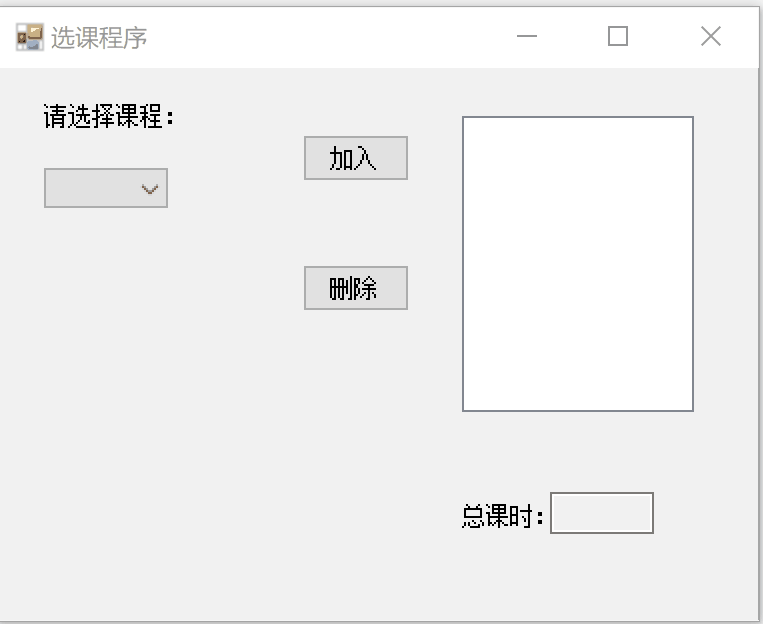