在 C# 中,ICollection 和 IList 是两个常见的集合接口,它们都继承自 IEnumerable,但有不同的功能和用途。理解它们之间的区别非常重要,尤其是在处理集合类时。
1. ICollection 接口
ICollection 是 .NET 集合类的基础接口之一。它继承自 IEnumerable,所以它提供了 foreach 循环支持(即支持迭代)。ICollection 定义了处理集合中的元素的基本方法和属性,如添加、移除元素,获取集合的大小等。
ICollection 接口主要成员:
- Count: 获取集合中元素的数量。
- IsReadOnly: 判断集合是否是只读的。
- Add(T item): 向集合中添加元素。
- Clear(): 清空集合中的所有元素。
- Contains(T item): 判断某个元素是否在集合中。
- Remove(T item):从集合中删除指定的元素。
- CopyTo(T[] array, int arrayIndex): 将集合的元素复制到数组中。
ICollection 不会强制实现元素的索引访问,而是强调集合的基本操作,如添加、移除、查找和复制。
2. IList 接口
IList 继承自 ICollection,因此它具有 ICollection 中定义的所有功能(如添加、删除元素等),但还提供了额外的功能,特别是支持按索引访问元素。
IList 接口主要成员:
- this[int index]: 允许按索引访问元素。
- Insert(int index, T item): 在指定索引处插入元素。
- RemoveAt(int index): 移除指定索引位置的元素。
- IndexOf(T item): 返回指定元素的索引。
IList 主要用于表示一个有序的集合,并提供了对集合元素的按位置访问和修改功能。因此,IList 适用于需要按位置操作的场景,如数组和列表。
3. 区别总结
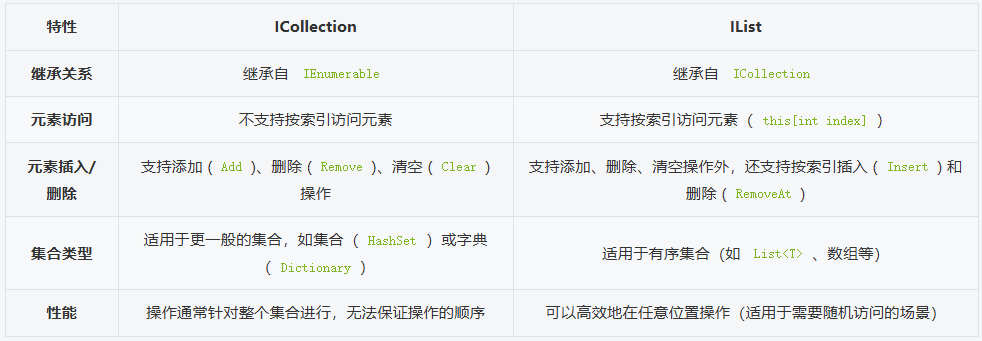
4. 代码示例
示例 1:使用 ICollection
csharp
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
ICollection<int> collection = new List<int> { 1, 2, 3, 4 };
// 打印集合中的元素
foreach (var item in collection)
{
Console.WriteLine(item);
}
// 使用 ICollection 方法
collection.Add(5);
collection.Remove(3);
Console.WriteLine("Count after modification: " + collection.Count);
// 复制到数组
int[] array = new int[collection.Count];
collection.CopyTo(array, 0);
Console.WriteLine("Array after copy:");
foreach (var item in array)
{
Console.WriteLine(item);
}
}
}
输出:
csharp
1
2
3
4
Count after modification: 4
Array after copy:
1
2
4
5
在这个示例中,ICollection 提供了常见的集合操作,如 Add、Remove 和 CopyTo,但它并不允许通过索引来访问集合中的元素。
示例 2:使用 IList
csharp
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
IList<int> list = new List<int> { 1, 2, 3, 4 };
// 使用索引访问和修改元素
Console.WriteLine("Element at index 2: " + list[2]);
list[2] = 10; // 修改元素
Console.WriteLine("Modified element at index 2: " + list[2]);
// 插入和删除元素
list.Insert(1, 20); // 在索引 1 处插入 20
list.RemoveAt(3); // 删除索引 3 处的元素
Console.WriteLine("List after insertion and removal:");
foreach (var item in list)
{
Console.WriteLine(item);
}
}
}
输出:
csharp
Element at index 2: 3
Modified element at index 2: 10
List after insertion and removal:
1
20
10
4
在这个示例中,IList 允许我们使用索引访问元素,并提供了方法(如 Insert 和 RemoveAt)来修改集合中的元素。
5. 使用场景
- ICollection: 用于需要执行基本集合操作(如添加、删除、查找)的场景。适合不需要索引访问的集合,如哈希集合、字典等。
- IList: 用于需要按位置操作元素的场景,如 List、数组等。适合顺序访问和修改元素的位置。
总的来说,ICollection 是一个更基础的接口,而 IList 在此基础上增加了更多操作,适合有序集合和需要按索引操作的场景。如果你只需要进行基本的集合操作,使用 ICollection 会更加简单直接;如果你需要按索引访问和操作元素,IList 是更合适的选择。