- get()方法读取一个字符,使用getline()来读取一行字符。
1.编写一个程序,读取键盘输入,直到遇到@符号为止,并回显输入(数字除外),同时将大写字符转换为小写字符,将小写字符转换为大写(别忘了cctype函数系列)
cpp
#include <iostream>
#include<cctype>
using namespace std;
int main()
{
cout << "请输入字符('@'停止):";
char input;
cin.get(input); // 从输入读取一个字符
while (input != '@')
{
if (islower(input))
{
input = toupper(input);
}
else if (isupper(input))
{
input = tolower(input);
}
else if (isdigit(input))
{
cin.get(input); // 读取下一个字符,忽略数字
continue;
}
// 输出转换后的字符
cout << input;
// 再次读取一个字符
cin.get(input);
}
cout << endl;
system("pause");
return 0;
}
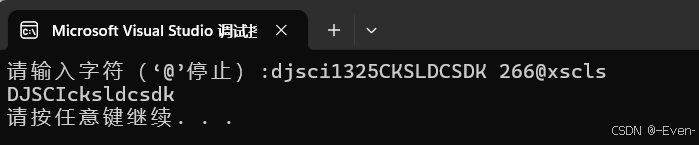
2.编写一个程序,最多将10个donation值读入一个double数组中(如果你愿意,也可以使用模板类array)。程序遇到非数字输入时将结束输入,并报告这些数字的平均值以及数组中有多少个数字大于平均值。
cpp
#include <iostream>
#include <array>
using namespace std;
int main()
{
array<double, 10> donation;
double input;
int count = 0;
double sum = 0, avg = 0;
cout << "请输入10个数字(输入非数字结束):";
for (int i = 0; (i < 10) && (cin >> input); i++)
{
donation[i] = input;
count++;
sum += donation[i];
}
avg = sum / count;
int large = 0;
for (int i = 0; i < count; i++)
{
if (donation[i] > avg)
{
large++;
}
}
cout << "平均值:" << avg << "数组中有" << large << "个数字大于平均值" << endl;
system("pause");
return 0;
}
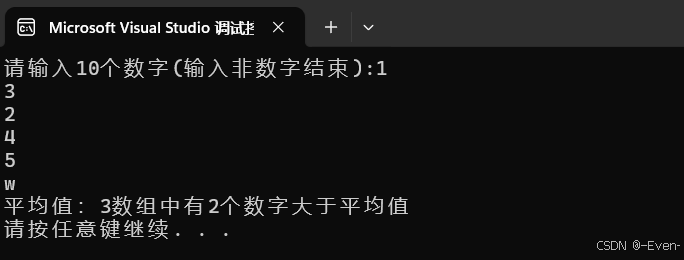
3.编写一个菜单驱动程序的雏形。该程序显示一个提供四个选项的菜单--每个选项用一个字母表标记。如果用户使用有效选项之外的字母进行响应,程序将提示用户输入有效的字母,直到用户这样选择为止。然后,该程序使用一条switch语句,根据用户的选择执行一个简单操作。该程序的运行情况如下:
cpp
Please enter one of the following choices:
c) carnivore p) pianist
t) tree g) game
f
Please enter a c,p,t or g:q
Please enter a c,p,t or g:t
A maple is a tree.
cpp
#include <iostream>
using namespace std;
int main()
{
cout << "Please enter one of the following choices:" << endl;
cout << "c) carnivore p) pianist" << endl;
cout << "t) tree g) game" << endl;
char choices;
cin.get(choices);
while (choices != 'c' && choices != 'p' && choices != 't' && choices != 'g')
{
cin.get();
cout << "Please enter a c,p,t or g:";
cin.get(choices);
}
switch (choices)
{
case 'c':
break;
case 'p':
break;
case 't':
cout << "对了!" << endl;
break;
case 'g':
break;
}
system("pause");
return 0;
}
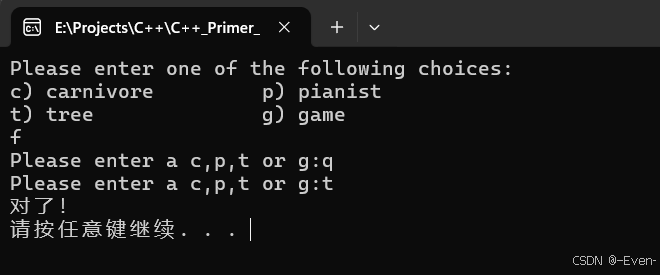
4.加入Benevolent Order of Programmer后,在BOP大会上,人们便可以通过加入者的真实姓名、头衔或秘密BOP姓名来了解他(她)。请编写一个程序,可以使用真实姓名、头衔、秘密姓名或成员偏好来列出成员。编写该程序时,请使用下面的结构:
cpp
//Benevolent Order of Programmers name structure
struct bop{
char fullname[strsize]; //real name
char title[strzie]; //job title
char bopname[strsize]; //secret BOP name
int preference; //0=fullname,1=title,2=bopname
};
该程序创建一个由上述结构组成的小型数组,并将其初始化为适当的值。另外,该程序使用一个循环,让用户在下面的选项中进行选择:
cpp
a.display by name b.diaplay by title
c.display by bopname d.display by preference
q.quit
注意,"display by preference"并不意味显示成员的偏好,而是意味着根据成员的偏好来列出成员。例如,如果偏好号为1,则选择d将显示程序员的头衔。该程序的运行情况如下:
cpp
Benevolent Order of Programmers Report
a. display by name b. display by title
c. display by bopname d. display by preference
q. quit
Enter your choice: a
Wimp Macho
Raki Rhodes
Celia Laiter
Hoppy Hipman
Pat Hand
Next choice: d
Wimp Macho
Junior Programmer
MIPS
Analyst Trainee
LOOPY
Next choice: q
Bye!
代码
cpp
#include <iostream>
using namespace std;
const int structsize = 40;
struct bop {
char fullname[structsize]; //real name
char title[structsize]; //job title
char bopname[structsize]; //secret BOP name
int preference; //0=fullname,1=title,2=bopname
};
bop user[] = {
{"even taylor","engineer","Ping",0},
{"niko tr","juni oop","",1},
{"celina hup","","MIPS",2},
{"sasiuciu","","Loop",2},
};
const int len = sizeof(user) / sizeof(user[0]);
void print_by_name();
void print_by_title();
void print_by_bopname();
void print_by_pref();
int main()
{
cout << "Benevolent Order of Programmers Report" << endl;
cout << "a. display by name b. display by title" << endl;
cout << "c. display by bopname d. display by preference" << endl;
cout << "q. quit" << endl;
cout << "Enter your choice: ";
char choices;
cin.get(choices);
while (choices != 'q')
{
switch (choices)
{
case 'a':
print_by_name();
break;
case 'b':
print_by_title();
break;
case 'c':
print_by_bopname();
break;
case 'd':
print_by_pref();
break;
default:
cout << "请输入a、b、c、d:";
break;
}
cin.get();
cout << "请输入你的下一个选项:";
cin.get(choices);
}
system("pause");
return 0;
}
void print_by_name() {
for (int i = 0; i < len; i++)
{
cout << user[i].fullname << endl;
}
}
void print_by_title() {
for (int i = 0; i < len; i++)
{
cout << user[i].title << endl;
}
}
void print_by_bopname() {
for (int i = 0; i < len; i++)
{
cout << user[i].bopname << endl;
}
}
void print_by_pref() {
for (int i = 0; i < len; i++)
{
if (user[i].preference == 0)
{
cout << user[i].fullname << endl;
}
else if (user[i].preference == 1)
{
cout << user[i].title << endl;
}
else
{
cout << user[i].bopname << endl;
}
}
}
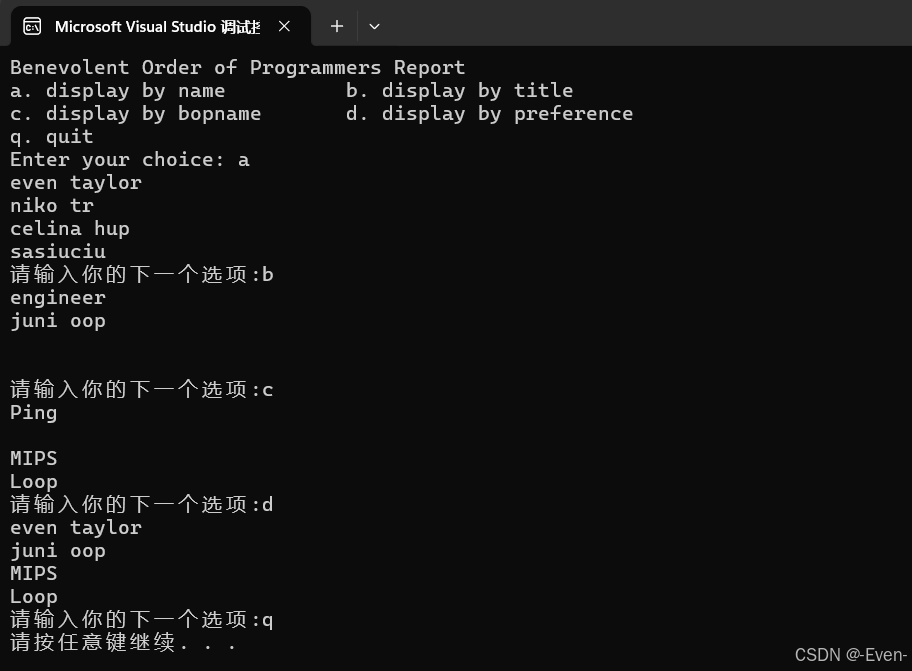
5.在Neutronia王国,货币单位是tvarp,收入所得税的计算方式如下:
5000 tvarps: 不收税
5001-15000 tvarps: 10%
15001-35000 tvarps: 15%
35000 tvarps以上: 20%
例如,收入为38000 tvarps时,所得税为5000×0.00+10000×0.10+2000×0.15+3000×0.20,即 4600tvarps。请编写一个程序,使用循环来要求用户输入收入,并报告所得税,当用户输入负数或非数字时,循环将结束。
cpp
#include <iostream>
using namespace std;
int main()
{
cout << "请输入收入(负数或者非数字结束):";
double income;
double tax;
while (cin >> income)
{
if (income > 35000)
{
tax = 10000 * 0.10 + 20000 * 0.15 + (income - 35000) * 0.20;
}
else if (income >= 15001)
{
tax = (income - 3500) * 0.15 + 10000 * 0.10;
}
else if (income >= 5001)
{
tax = (income - 5000) * 0.10;
}
else if (income >= 0)
{
tax = 0;
}
else
{
cout << "请输入正确数字" << endl;
break;
}
cout << "你的所得税为:" << tax << " tvarps" << endl;
cout << "请输入收入(负数或者非数字结束):";
}
system("pause");
return 0;
}
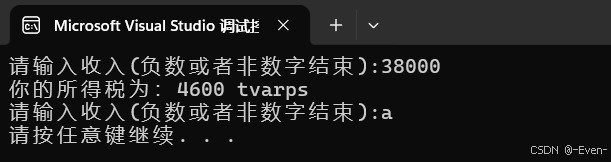
6.编写一个程序,记录捐助给"维护合法权利团体"的资金。该程序要求用户输入捐赠者数目,然后要求用户输入每一个捐献者的姓名和款项。这些信息被存储在一个动态分配的结构数组中.每个数据结构有两个成员:用来存储姓名的字符串数组(或string对象)和用来存储款项的double成员。读取所有的数据后,程序将显示所有捐款超过10000的捐款着姓名及其捐款数额。该列表前应包含一个标题,指出下面的捐款者是重要捐款人(Grand Patrons)。然后,程序将列出其他的捐款者,该列表要以Partons开头。如果某种类别没有捐款者,则程序将打印单词"none"。该程序只显示这两种类别,而不进行排序。
cpp
#include <iostream>
#include <string>
using namespace std;
struct Donation
{
string name; //姓名
double fund; //捐款数额
};
void display(Donation* p, int patrona_number, bool isGrandPatrons);
int main()
{
cout << "How many patrons? ";
int patrona_number;
cin >> patrona_number;
cin.get();
//建立动态数组
Donation* p = new Donation[patrona_number];
cout << "Starting to input patrons' info:" << endl;
int id = 0;
while (id < patrona_number)
{
cout << "Enter the name of patrons:";
getline(cin, p[id].name);
cout << "Enter the fund of " << p[id].name << " :";
cin >> p[id].fund;
cin.get();
id++;
cout << "Continue to input,or press(f)to finished.";
if (cin.get() == 'f')
{
break;
}
}
cout << "******Grand Partons******" << endl;
display(p, patrona_number, true);
cout << "******Partons******" << endl;
display(p, patrona_number, false);
system("pause");
return 0;
}
void display(Donation* p, int patrona_number, bool isGrandPatrons) {
int num = 0;
for (int i = 0; i < patrona_number; i++)
{
if (isGrandPatrons) {
if (p[i].fund > 10000)
{
num++;
cout << p[i].name << endl;
}
}
else
{
if (p[i].fund <= 10000)
{
num++;
cout << p[i].name << endl;
}
}
}
if (num == 0) {
cout << "none" << endl;
}
}
7.编写一个程序,它每次读取一个单词,直到用户只输入q。然后,该程序指出有多少个单词以元音打头,有多少个单词以辅音打头,还有多少个单词不属于这两类。为此,方法之一是,使用isalpha()来区分以字母和其他字母打头的单词,然后对于通过isalpha()测试的单词,使用if或switch语句来确定哪些以元音打头。该程序的运行情况如下:
cpp
Enter words (q to quit):
The 12 awesome oxem ambled
quiety across 15 meters of lawn. q
5 words beginning with vowels
4 words beginning with consonants
2 others
cpp
#include <iostream>
#include <string>
using namespace std;
int main()
{
char words;
cout << "Enter words (q to quit):" << endl;
int other = 0; //记录其他个数
int num = 0;//记录元音个数
int count = 0; //记录非元音个数
while (cin >> words)
{
if (words == 'q')
{
break;
}
else
{
if (isalpha(words))
{
switch (words)
{
case 'a':
case 'e':
case 'i':
case 'o':
case 'u':
num++;
break;
default:
count++;
break;
}
}
else
{
other++;
}
}
}
cout << num << " words beginning with vowels" << endl;
cout << count << " words beginning with consonants" << endl;
cout << other << " others" << endl;
system("pause");
return 0;
}
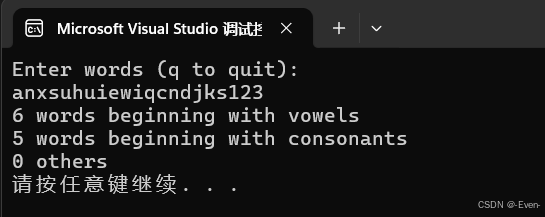
8.编写一个程序,他打开一个文件,逐个字地读取该文件,直到到达文件末尾,然后指出该文件中包含多少个字符。
cpp
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main()
{
ifstream stream;
string file_name;
cout << "Enter the file name: ";
getline(cin, file_name);
// 通过文件流打开文件、如果打开失败则终止
stream.open(file_name);
if (!stream.is_open())
{
cout << "Error to open file." << endl;
exit(1);
}
char read_char;
int count = 0;
// efo()判断是否到达文件末尾
while (!stream.eof())
{
stream >> read_char;
count++;
}
cout << file_name << "文件一共有" << count << "字符" << endl;
//关闭文件流
stream.close();
system("pause");
return 0;
}
9.完成编程练习6,但从文件中读取所需的信息。该文件的第一项应为捐款人数,余下的内容应为对的行。在每一对中,第一行为捐款人姓名,第二行为捐款数额。
cpp
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
struct Donation
{
string name; //姓名
double fund; //捐款数额
};
void display(Donation* p, int patrona_number, bool isGrandPatrons);
int main()
{
ifstream fin;
fin.open("09.txt", ios::in);
if (!fin.is_open()) {
cout << "Error to open file." << endl;
exit(1);
}
int number = 0;
fin >> number; //读取人数
fin.get();
if (number <= 0)
{
exit(1);
}
//动态数组
Donation* p = new Donation[number];
//循环读取捐款人信息
cout << "******读取文件信息******" << endl;
for (int i = 0; i < number; i++)
{
getline(fin, p[i].name);
fin >> p[i].fund;
fin.get();
cout << p[i].name << " -> " << p[i].fund << endl;
}
cout << "******Grand Partons******" << endl;
display(p, number, true);
cout << "******Partons******" << endl;
display(p, number, false);
system("pause");
return 0;
}
void display(Donation* p, int patrona_number, bool isGrandPatrons) {
int num = 0;
for (int i = 0; i < patrona_number; i++)
{
if (isGrandPatrons) {
if (p[i].fund > 10000)
{
num++;
cout << p[i].name << endl;
}
}
else
{
if (p[i].fund <= 10000)
{
num++;
cout << p[i].name << endl;
}
}
}
if (num == 0) {
cout << "none" << endl;
}
}