一、简介
本文介绍了如何使用OpenGL实现硬阴影效果,并在最后给出了全部的代码。本文基于[OpenGL]渲染Shadow Map,实现硬阴影的流程如下:
- 首先,以光源为视角,渲染场景的深度图,将light space中的深度图存储到深度缓冲
depthTextur
中。 - 然后,以相机为视角,渲染场景。在fragment shader中根据各个片段在light space中的实际深度的与
depthTexture
中对应坐标中的深度值作对比,假如实际深度大于depthTexture中深度值,说明在light space中,该片段对应的三角面片(点)会被场景中的其他三角面片遮挡,因此在阴影中。否则,说明不在阴影中。
按照本文代码实现完成后,理论上可以得到如下结果:
二、使用OpenGL实现硬阴影
0. 环境需要
-
Linux,或者 windos下使用wsl2。
-
安装GLFW和GLAD。请参考[OpenGL] wsl2上安装使用cmake+OpenGL教程。
-
安装glm。glm是个可以只使用头文件的库,因此可以直接下载release的压缩文件,然后解压到
include
目录下。例如,假设下载的release版本的压缩文件为glm-1.0.1-light.zip
。将glm-1.0.1-light.zip
复制include
目录下,然后执行以下命令即可解压glm源代码:shellunzip glm-1.0.1-light.zip
-
需要下载 stb_image.h 作为加载
.png
图像的库。将 stb_image.h 下载后放入include/
目录下。
1. 项目目录
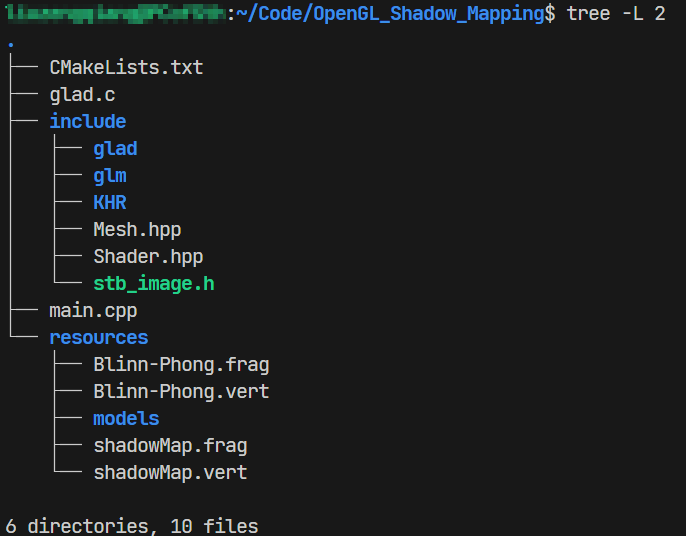
其中:
Mesh.hpp
包含了自定义的 Vertex, Texture, 和 Mesh 类,用于加载 obj 模型、加载图片生成纹理。Shader.hpp
用于创建 shader 程序。shadowMap.vert
和shadowMap.frag
是用于渲染shadow map
的 顶点着色器 和 片段着色器 代码,该shader
以light为视角,渲染得到light space下的深度图,并将其存储到depthTexture
中。BlinnPhong.vert
和BlinnPhong.frag
是用于渲染场景,根据depthTexture实现阴影效果
的 顶点着色器 和 片段着色器 代码。
下面介绍各部分的代码:
2. CMakeLists.txt代码
cpp
cmake_minimum_required(VERSION 3.10)
set(CMAKE_CXX_STANDARD 14)
project(OpenGL_Shadow_Mapping)
include_directories(include)
find_package(glfw3 REQUIRED)
file(GLOB project_file main.cpp glad.c)
add_executable(${PROJECT_NAME} ${project_file})
target_link_libraries(${PROJECT_NAME} glfw)
3. Mesh.hpp 代码
Mesh.hpp 代码与[OpenGL]渲染Shadow Map中的Mesh.hpp基本相同。主要区别是,本文的Mesh在加载模型时,手动在模型下方添加了一个 pedestal,用于显示模型产生的阴影。
另外,本文中的Draw(Shader, GLuint depthTexture)
函数是使用两个纹理对象,一个是默认的模型纹理,另一个是传入的参数depthTexture
,将其作为shadow map
。
Mesh.hpp的主要代码如下:
cpp
extern unsigned int SCR_WIDTH;
extern unsigned int SCR_HEIGHT;
class Mesh
{
public:
// mesh Data
vector<Vertex> vertices; // vertex 数据,一个顶点包括 position, normal 和 texture coord 三个信息
vector<unsigned int> indices; // index 数据,用于拷贝到 EBO 中
Texture texture;
unsigned int VAO;
Mesh(vector<Vertex> vertices_, vector<unsigned int> indices_, Texture texture_)
: vertices(vertices_), indices(indices_), texture(texture_)
{
setupMesh();
}
Mesh(string obj_path, string texture_path = "")
{
// load obj
ifstream obj_file(obj_path, std::ios::in);
if (obj_file.is_open() == false)
{
std::cerr << "Failed to load obj: " << obj_path << "\n";
return;
}
int position_id = 0;
int normal_id = 0;
int texture_coord_id = 0;
string line;
while (getline(obj_file, line))
{
std::istringstream iss(line);
std::string prefix;
iss >> prefix;
if (prefix == "v") // vertex
{
if (vertices.size() <= position_id)
{
vertices.push_back(Vertex());
}
iss >> vertices[position_id].Position.x;
iss >> vertices[position_id].Position.y;
iss >> vertices[position_id].Position.z;
position_id++;
}
else if (prefix == "vn") // normal
{
if (vertices.size() <= normal_id)
{
vertices.push_back(Vertex());
}
iss >> vertices[normal_id].Normal.x;
iss >> vertices[normal_id].Normal.y;
iss >> vertices[normal_id].Normal.z;
normal_id++;
}
else if (prefix == "vt") // texture coordinate
{
if (vertices.size() <= texture_coord_id)
{
vertices.push_back(Vertex());
}
iss >> vertices[texture_coord_id].TexCoords.x;
iss >> vertices[texture_coord_id].TexCoords.y;
texture_coord_id++;
}
else if (prefix == "f") // face
{
for (int i = 0; i < 3; ++i)
{
std::string vertexData;
iss >> vertexData;
unsigned int ver, tex, nor;
sscanf(vertexData.c_str(), "%d/%d/%d", &ver, &tex, &nor);
indices.push_back(ver - 1);
}
}
}
obj_file.close();
// 在模型下面加上一个 pedestal
int temp_index = vertices.size();
vertices.push_back({{-2, -0.8, -2}, {0, 1, 0}, {-1, -1}});
vertices.push_back({{-2, -0.8, 2}, {0, 1, 0}, {-1, -1}});
vertices.push_back({{2, -0.8, 2}, {0, 1, 0}, {-1, -1}});
vertices.push_back({{2, -0.8, -2}, {0, 1, 0}, {-1, -1}});
indices.push_back(temp_index + 0);
indices.push_back(temp_index + 1);
indices.push_back(temp_index + 2);
indices.push_back(temp_index + 0);
indices.push_back(temp_index + 2);
indices.push_back(temp_index + 3);
// load texture
GLuint textureID;
glGenTextures(1, &textureID); // 生成纹理 ID
glBindTexture(GL_TEXTURE_2D, textureID); // 绑定纹理,说明接下来对纹理的操作都应用于对象 textureID 上
// 设置纹理参数
// 设置纹理在 S 方向(水平方向)的包裹方式为 GL_REPEAT
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_REPEAT);
// 设置纹理在 T 方向(垂直方向)的包裹方式为 GL_REPEAT
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_REPEAT);
// 设置纹理的缩小过滤方式,当纹理变小时,使用 GL_LINEAR (线性过滤)方式
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR);
// 设置纹理的放大过滤方式,当纹理变大时,使用 GL_LINEAR (线性过滤)方式
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR);
// 加载纹理图像
int width, height, nrChannels;
stbi_set_flip_vertically_on_load(true);
unsigned char *data = stbi_load(texture_path.c_str(), &width, &height, &nrChannels, 0);
if (data)
{
GLenum format;
if (nrChannels == 1)
format = GL_RED;
else if (nrChannels == 3)
format = GL_RGB;
else if (nrChannels == 4)
format = GL_RGBA;
// 生成纹理
glTexImage2D(GL_TEXTURE_2D, 0, format, width, height, 0, format, GL_UNSIGNED_BYTE, data);
glGenerateMipmap(GL_TEXTURE_2D); // 生成 Mipmaps
}
else
{
std::cerr << "Failed to load texture: " << texture_path << "\n";
}
stbi_image_free(data); // 释放图像内存
glBindTexture(GL_TEXTURE_2D, 0); // 解绑纹理
texture.Id = textureID;
texture.path = texture_path;
setupMesh();
}
// render the mesh
void Draw(Shader &shader)
{
// draw mesh
...
}
void DrawWithShadowMap(Shader &shader, GLuint shadowMap)
{
// draw mesh
glActiveTexture(GL_TEXTURE0); // 激活 纹理单元0
glBindTexture(GL_TEXTURE_2D, texture.Id); // 绑定纹理,将纹理texture.id 绑定到 纹理单元0 上
glUniform1i(glGetUniformLocation(shader.ID, "texture1"), 0); // 将 blinnPhongShader 中的 texture1 绑定到 纹理单元0
glActiveTexture(GL_TEXTURE1); // 激活 纹理单元1
glBindTexture(GL_TEXTURE_2D, shadowMap); // 绑定纹理,将深度纹理 shadowMap 绑定到 纹理单元1 上
glUniform1i(glGetUniformLocation(shader.ID, "shadowMap"), 1); // 将 blinnPhongShader 中的 shadowMap 绑定到 纹理单元1
glBindVertexArray(VAO);
glDrawElements(GL_TRIANGLES, static_cast<unsigned int>(indices.size()), GL_UNSIGNED_INT, 0);
glBindTexture(GL_TEXTURE_2D, 0);
glBindVertexArray(0);
}
// 用于打印 depthTexture 数据
void printDepthTexture(GLuint textureId)
{
glBindTexture(GL_TEXTURE_2D, textureId); // 绑定纹理,将纹理texture.id 绑定到 纹理单元0 上
int width;
int height;
GLint format;
glGetTexLevelParameteriv(GL_TEXTURE_2D, 0, GL_TEXTURE_WIDTH, &width);
glGetTexLevelParameteriv(GL_TEXTURE_2D, 0, GL_TEXTURE_HEIGHT, &height);
glGetTexLevelParameteriv(GL_TEXTURE_2D, 0, GL_TEXTURE_INTERNAL_FORMAT, &format);
std::cout << "Texture information :\n";
std::cout << "width:" << width << ", height:" << height << ", format:" << format << "\n";
// return ;
// 创建一个缓冲区来存储纹理数据
std::vector<GLfloat> textureData(width * height, 0);
// 读取纹理数据
glGetTexImage(GL_TEXTURE_2D, 0, GL_DEPTH_COMPONENT, GL_FLOAT, textureData.data());
std::cout << *max_element(textureData.begin(), textureData.end()) << "\n";
std::cout << *min_element(textureData.begin(), textureData.end()) << "\n";
glBindTexture(GL_TEXTURE_2D, 0);
}
void DrawToTexture(Shader &shader, GLuint &depthTexture)
{
// 1. 设置 帧缓存
// 2. 设置 纹理 (renderedTexture,由于存储渲染结果)
// 3. 设置 深度缓存
// 4. 开始渲染
// 1. 设置 帧缓存
// framebuffer
GLuint FramebufferName = 0;
glGenFramebuffers(1, &FramebufferName);
glBindFramebuffer(GL_FRAMEBUFFER, FramebufferName);
// 2. 设置 纹理 (depthTexture,由于存储渲染结果)
// texture
// GLuint depthTexture;
if (glIsTexture(depthTexture) == false)
{
glGenTextures(1, &depthTexture);
}
// "Bind" the newly created texture : all future texture functions will modify this texture
// 将 depthTexture 绑定到 GL_TEXTURE_2D 上,接下来所有对 TEXTURE_2D 的操作都会应用于 depthTexture 上
glBindTexture(GL_TEXTURE_2D, depthTexture);
// Give an empty image to OpenGL ( the last "0" )
// glTexImage2d() 用于创建并初始化二维纹理数据的函数, 参数含义如下:
// 1. 目标纹理类型, GL_TEXTURE_2D 为 2D 类型纹理
// 2. 详细级别(mipmap级别),基础图像级别通常设置为0
// 3. internal format: 存储格式,GL_DEPTH_COMPONENT16 表示为 16位的深度缓存
// 4,5. 纹理宽,高,设为800, 600(与窗口同宽、高)
// 6. 边框宽度,设为0
// 7. 传入数据的纹理格式,此处选择 GL_DEPTH_COMPONENT (由于我们使用 null
// 指针处地数据初始化纹理,不管此处选择什么对结果都无影响)
// 8. format 数据类型,每个颜色通道内的数据类型,设为 GL_FLOAT,数值范围在 [0.0,1.0]
// 9. 指向纹理图像数据(初始数据)的指针,设为0(null),使用空置初始化纹理
glTexImage2D(GL_TEXTURE_2D, 0, GL_DEPTH_COMPONENT16, 800, 600, 0, GL_DEPTH_COMPONENT, GL_FLOAT, nullptr);
// Poor filtering
// 设置 GL_TEXTURE_2D 纹理的过滤方式
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_NEAREST);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_NEAREST);
// 设置 GL_TEXTURE_2D 纹理的边缘处理方式
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP_TO_EDGE);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP_TO_EDGE);
// 3. 设置 深度缓存
// The depth buffer
// 为上面的 framebuffer 申请一个 depth buffer (用于正确绘制)
// 手动申请的 framebuffer 不会自动带有 depth buffer or template buffer or color buffer,必须手动设置
// 此处收到设置一个 depth buffer
// 由于正确地渲染结果(主要根据渲染场景的深度信息确定哪些部分需要渲染,哪些部分可以丢弃,跟正常渲染流程一样)
GLuint depthrenderbuffer;
glGenRenderbuffers(1, &depthrenderbuffer);
// 绑定渲染缓冲对象,指定后续的 操作(设置) 目标为 depthrederbuffer
glBindRenderbuffer(GL_RENDERBUFFER, depthrenderbuffer);
// 指定渲染缓冲的内部格式为深度格式,意味着这个缓冲区将用于存储深度信息
glRenderbufferStorage(GL_RENDERBUFFER, GL_DEPTH_COMPONENT, 800, 600);
// 将渲染缓冲对象附加到当前绑定的帧缓冲对象
glFramebufferRenderbuffer(GL_FRAMEBUFFER, GL_DEPTH_ATTACHMENT, GL_RENDERBUFFER, depthrenderbuffer);
// Set "renderedTexture" as our colour attachement #0
// 设置 renderedTexture 附加到 帧缓冲对象上, 并设置 深度缓冲槽位 为 GL_DEPTH_ATTACHMENT
glFramebufferTexture(GL_FRAMEBUFFER, GL_DEPTH_ATTACHMENT, depthTexture, 0);
// Set the list of draw buffers.
// 设置不渲染任何 color , 因为我们关心的只是 depth
glDrawBuffer(GL_NONE);
// Always check that our framebuffer is ok
if (glCheckFramebufferStatus(GL_FRAMEBUFFER) != GL_FRAMEBUFFER_COMPLETE)
{
std::cout << "Error";
return;
}
// Render to our framebuffer
// 绑定 FramebufferName,接下来的渲染将写入到 FramebufferName 帧缓存中
glBindFramebuffer(GL_FRAMEBUFFER, FramebufferName);
// 申请生成 depth buffer 后尽量(必须)手动 clear 一下
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
// 4. 开始渲染
// 开始渲染,将渲染结果存储到 renderedTexture
// draw mesh
glBindVertexArray(VAO);
glDrawElements(GL_TRIANGLES, static_cast<unsigned int>(indices.size()), GL_UNSIGNED_INT, 0);
glBindTexture(GL_TEXTURE_2D, 0);
glBindVertexArray(0);
// 解绑 FramebufferName,接下来的渲染将写入默认的帧缓冲(屏幕) 中
glBindFramebuffer(GL_FRAMEBUFFER, 0);
/****************/
// printDepthTexture(depthTexture);
}
...
};
4. shadowMap shader 代码
由于我们只需要使用场景渲染shader得到场景的深度缓冲,因此只需要在 shadow map vertex shader 中处理顶点的坐标即可,无需使用纹理、光照模型等与深度信息无关的数据。
shadow map shader的顶点着色器代码如下:
shadowMap.vert
:
cpp
#version 330 core
layout(location = 0) in vec3 aPos;
layout(location = 1) in vec3 aNor;
layout(location = 2) in vec2 aTexCoord;
uniform mat4 lightMVP;
void main() {
// 裁剪空间坐标系 (clip space) 中 点的位置
gl_Position = lightMVP * vec4(aPos, 1.0f);
}
片段着色器无需处理颜色、纹理、光照等信息,因此shadow map shader的片段着色器可以空着,如下:
shadowMap.frag
:
cpp
#version 330 core
void main() {
// do nothing
}
5. Blinn-Phong shader 代码
渲染场景的 Blinn-Phong shader使用Blinn-Phong模型渲染场景,并且根据输入的 shadowMap 处理产生阴影效果。
Blinn-Phong shader的顶点着色器和片段着色器代码:
Blinn-Phong.vert
:
cpp
#version 330 core
layout(location = 0) in vec3 aPos;
layout(location = 1) in vec3 aNor;
layout(location = 2) in vec2 aTexCoord;
uniform mat4 model;
uniform mat4 view;
uniform mat4 projection;
uniform mat4 lightMVP;
out vec3 vertexPos;
out vec3 vertexNor;
out vec2 textureCoord;
out vec4 vertexPosLightSpace;
void main() {
textureCoord = aTexCoord;
// 裁剪空间坐标系 (clip space) 中 点的位置
gl_Position = projection * view * model * vec4(aPos, 1.0f);
// 世界坐标系 (world space) 中 点的位置
vertexPos = (model * vec4(aPos, 1.0f)).xyz;
// 世界坐标系 (world space) 中 点的法向
vertexNor = mat3(transpose(inverse(model))) * aNor;
vertexPosLightSpace = lightMVP * vec4(aPos, 1.0f);
}
Blinn-Phong.frag
:
cpp
#version 330 core
out vec4 FragColor;
in vec3 vertexPos;
in vec3 vertexNor;
in vec2 textureCoord;
in vec4 vertexPosLightSpace;
// vertexPosLightSpace
uniform vec3 cameraPos;
uniform vec3 lightPos;
uniform vec3 k;
uniform sampler2D texture1;
uniform sampler2D shadowMap;
// 计算阴影系数 shadow
// 如果该片段在阴影中 返回 1.0
// 如果该片段不在阴影中 返回 0.0
float ShadowCalculation(vec4 fragPosLightSpace, vec3 normalDir, vec3 lightDir) {
// 执行透视除法
vec3 projCoords = fragPosLightSpace.xyz / fragPosLightSpace.w;
if (projCoords.z > 1.0) {
// 所有在 视锥远平面 之外的都视作不被遮挡
return 0.0;
}
// 变换到[0,1]的范围
projCoords = projCoords * 0.5 + 0.5;
// 取得最近点的深度
float closestDepth = texture(shadowMap, projCoords.xy).r;
// 取得当前片段在光源视角下的实际深度
float currentDepth = projCoords.z;
// 检查当前片段是否在阴影中
float bias = max(0.05 * (1.0 - dot(normalDir, lightDir)), 0.005); // 使用 bais 处理阴影失真的问题
float shadow = currentDepth - bias > closestDepth ? 1.0 : 0.0;
return shadow;
}
void main() {
vec3 lightColor = vec3(1.0f, 1.0f, 1.0f);
// Ambient
// Ia = ka * La
float ambientStrenth = k[0];
vec3 ambient = ambientStrenth * lightColor;
// Diffuse
// Id = kd * max(0, normal dot light) * Ld
float diffuseStrenth = k[1];
vec3 normalDir = normalize(vertexNor);
vec3 lightDir = normalize(lightPos - vertexPos);
vec3 diffuse =
diffuseStrenth * max(dot(normalDir, lightDir), 0.0) * lightColor;
// Specular (Phong)
// Is = ks * (view dot reflect)^s * Ls
// float specularStrenth = k[2];
// vec3 viewDir = normalize(cameraPos - vertexPos);
// vec3 reflectDir = reflect(-lightDir, normalDir);
// vec3 specular = specularStrenth *
// pow(max(dot(viewDir, reflectDir), 0.0f), 2) * lightColor;
// Specular (Blinn-Phong)
// Is = ks * (normal dot halfway)^s Ls
float specularStrenth = k[2];
vec3 viewDir = normalize(cameraPos - vertexPos);
vec3 halfwayDir = normalize(lightDir + viewDir);
vec3 specular = specularStrenth *
pow(max(dot(normalDir, halfwayDir), 0.0f), 2) * lightColor;
// Obejct color
vec3 objectColor = vec3(0.8, 0.8, 0.8);
if (textureCoord.x >= 0 && textureCoord.y >= 0) {
objectColor = texture(texture1, textureCoord).xyz;
}
// shadow
float shadow = ShadowCalculation(vertexPosLightSpace, normalDir, lightDir);
// Color = Ambient + Diffuse + Specular -->
// Color = Ambient + (1-shadow) * (Diffuse + Specular), 阴影只会影响 diffuse 和 specular 项
// I = Ia + Id + Is --> I = Ia + (1-shodaw)*(Id + Is)
FragColor = vec4(
(ambient + (1.0 - shadow) * (diffuse + specular)) * objectColor, 1.0f);
}
6. main.cpp 代码
6.1). 代码整体流程
- 初始化glfw,glad,窗口
- 编译 shader 程序
- 加载obj模型、纹理图片
- 设置光源和相机位置,Blinn-phong模型参数
- 开始渲染
5.1 使用 shadowShader, 渲染场景,将场景的深度缓冲存储到 depthTexture 中
5.2 使用 blinnPhongShader, 渲染场景,并且使用 depthTexture 实现阴影效果 - 释放资源
6.2). main.cpp代码
cpp
#include <glad/glad.h>
#include <GLFW/glfw3.h>
#include "Shader.hpp"
#include "Mesh.hpp"
#include "glm/ext.hpp"
#include "glm/mat4x4.hpp"
#include <random>
#include <iostream>
// 用于处理窗口大小改变的回调函数
void framebuffer_size_callback(GLFWwindow *window, int width, int height);
// 用于处理用户输入的函数
void processInput(GLFWwindow *window);
// 指定窗口默认width和height像素大小
unsigned int SCR_WIDTH = 800;
unsigned int SCR_HEIGHT = 600;
/************************************/
int main()
{
/****** 1.初始化glfw, glad, 窗口 *******/
// glfw 初始化 + 配置 glfw 参数
glfwInit();
glfwWindowHint(GLFW_CONTEXT_VERSION_MAJOR, 3);
glfwWindowHint(GLFW_CONTEXT_VERSION_MINOR, 3);
glfwWindowHint(GLFW_OPENGL_PROFILE, GLFW_OPENGL_CORE_PROFILE);
// 在创建窗口之前
glfwWindowHint(GLFW_SAMPLES, 4); // 设置多重采样级别为4
// glfw 生成窗口
GLFWwindow *window = glfwCreateWindow(SCR_WIDTH, SCR_HEIGHT, "LearnOpenGL", NULL, NULL);
if (window == NULL)
{
// 检查是否成功生成窗口,如果没有成功打印出错信息并且退出
std::cout << "Failed to create GLFW window" << std::endl;
glfwTerminate();
return -1;
}
// 设置窗口window的上下文
glfwMakeContextCurrent(window);
// 配置window变化时的回调函数
glfwSetFramebufferSizeCallback(window, framebuffer_size_callback);
// 使用 glad 加载 OpenGL 中的各种函数
if (!gladLoadGLLoader((GLADloadproc)glfwGetProcAddress))
{
std::cout << "Failed to initialize GLAD" << std::endl;
return -1;
}
// 启用 深度测试
glEnable(GL_DEPTH_TEST);
// 启用 多重采样抗锯齿
glEnable(GL_MULTISAMPLE);
// glPolygonMode(GL_FRONT_AND_BACK, GL_LINE); // 使用线框模式,绘制时只绘制 三角形 的轮廓
glPolygonMode(GL_FRONT_AND_BACK, GL_FILL); // 使用填充模式,绘制时对 三角形 内部进行填充
/************************************/
/****** 2.编译 shader 程序 ******/
// 渲染shadow map 的 shader
Shader shadowMapShader("../resources/shadowMap.vert", "../resources/shadowMap.frag");
// 渲染场景的shader
Shader blinnPhongShader("../resources/Blinn-Phong.vert", "../resources/Blinn-Phong.frag");
// 渲染depth的 shader
// Shader showDepthShader("../resources/showDepth.vert", "../resources/showDepth.frag");
/************************************/
/****** 3.加载obj模型、纹理图片、Phong模型参数 ******/
// 3.1 scene mesh
Mesh ourModel("../resources/models/spot/spot.obj", "../resources/models/spot/spot.png"); // dairy cow
Texture depthTexture;
/************************************/
/****** 4.设置光源和相机位置,Phong(Blinn-phong)模型参数 ******/
// I = Ia + Id + Is
// Ia = ka * La
// Id = kd * (normal dot light) * Ld
// Is = ks * (reflect dot view)^s * Ls
// 模型参数 ka, kd, ks
float k[] = {0.1f, 0.7f, 0.2f}; // ka, kd, ks
// 光源位置
glm::vec3 light_pos = glm::vec3(-2.0f, 2.0f, 0.0f);
// 相机位置
glm::vec3 camera_pos = glm::vec3(0.0f, 1.0f, 1.5f);
/************************************/
/****** 5.开始渲染 ******/
float rotate = 90.0f;
while (!glfwWindowShouldClose(window))
{
rotate += 0.5f;
// input
// -----
processInput(window);
// render
// ------
glClearColor(0.2f, 0.3f, 0.3f, 1.0f);
// 清除颜色缓冲区 并且 清除深度缓冲区
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
// 5.1 使用 shadowShader, 渲染场景,将场景的深度缓冲存储到 depthTexture 中
shadowMapShader.use();
// 设置 light_MVP 矩阵, 假设以 light 为视角,渲染 light 视角下的场景深度图
// light model 矩阵
glm::mat4 model = glm::mat4(1.0f);
model = glm::translate(model, glm::vec3(0.0f, 0.0f, 0.0f));
model = glm::rotate(model, glm::radians(0.0f), glm::vec3(1.0f, 0.0f, 0.0f));
model = glm::rotate(model, glm::radians(rotate), glm::vec3(0.0f, 1.0f, 0.0f));
model = glm::rotate(model, glm::radians(0.0f), glm::vec3(0.0f, 0.0f, 1.0f));
model = glm::scale(model, glm::vec3(0.5f, 0.5f, 0.5f));
// light view 矩阵
glm::mat4 view = glm::mat4(1.0f);
view = glm::lookAt(light_pos, glm::vec3(0.0f, 0.0f, 0.0f), glm::vec3(0.0f, 1.0f, 0.0f));
// light projection 矩阵
glm::mat4 projection = glm::mat4(1.0f);
// 假设 light 为平行光,因此使用 正交投影 ortho
projection = glm::ortho(-2.0, 2.0, -2.0, 2.0, 0.1, 5.0);
glm::mat4 lightMVP = projection * view * model;
shadowMapShader.setMat4("lightMVP", lightMVP);
ourModel.DrawToTexture(shadowMapShader, depthTexture.Id);
///
// 5.2 使用 blinnPhongShader, 渲染场景,并且使用 depthTexture 实现阴影效果
blinnPhongShader.use();
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
// 设置 camera_MVP 矩阵, 假设以 camera 为视角,渲染 camera 视角下的场景深度图
// camera model 矩阵
model = glm::mat4(1.0f);
model = glm::translate(model, glm::vec3(0.0f, 0.0f, 0.0f));
model = glm::rotate(model, glm::radians(0.0f), glm::vec3(1.0f, 0.0f, 0.0f));
model = glm::rotate(model, glm::radians(90.0f), glm::vec3(0.0f, 1.0f, 0.0f));
model = glm::rotate(model, glm::radians(0.0f), glm::vec3(0.0f, 0.0f, 1.0f));
model = glm::scale(model, glm::vec3(0.5f, 0.5f, 0.5f));
// camera view 矩阵
view = glm::lookAt(camera_pos, glm::vec3(0.0f, 0.0f, 0.0f), glm::vec3(0.0f, 1.0f, 0.0f));
// camera projection 矩阵
projection = glm::perspective(glm::radians(60.0f), (float)SCR_WIDTH / (float)SCR_HEIGHT, 0.1f, 100.0f);
blinnPhongShader.setMat4("model", model);
blinnPhongShader.setMat4("view", view);
blinnPhongShader.setMat4("projection", projection);
blinnPhongShader.setVec3("k", k[0], k[1], k[2]);
blinnPhongShader.setVec3("cameraPos", camera_pos);
blinnPhongShader.setVec3("lightPos", light_pos);
blinnPhongShader.setMat4("lightMVP", lightMVP);
// 使用 depthTexture 作为 shadow map texture
ourModel.DrawWithShadowMap(blinnPhongShader, depthTexture.Id);
///
glfwSwapBuffers(window); // 在gfw中启用双缓冲,确保绘制的平滑和无缝切换
glfwPollEvents(); // 用于处理所有挂起的事件,例如键盘输入、鼠标移动、窗口大小变化等事件
}
/************************************/
/****** 6.释放资源 ******/
// glfw 释放 glfw使用的所有资源
glfwTerminate();
/************************************/
return 0;
}
// 用于处理用户输入的函数
void processInput(GLFWwindow *window)
{
// 当按下 Esc 按键时调用 glfwSetWindowShouldClose() 函数,关闭窗口
if (glfwGetKey(window, GLFW_KEY_ESCAPE) == GLFW_PRESS)
{
glfwSetWindowShouldClose(window, true);
}
}
// 在使用 OpenGL 和 GLFW 库时,处理窗口大小改变的回调函数
// 当窗口大小发生变化时,确保 OpenGL 渲染的内容能够适应新的窗口大小,避免图像被拉伸、压缩或出现其他比例失真的问题
void framebuffer_size_callback(GLFWwindow *window, int width, int height)
{
SCR_WIDTH = width;
SCR_HEIGHT = height;
glViewport(0, 0, width, height);
}
7. 编译运行及结果
编译运行:
cpp
cd ./build
cmake ..
make
./OpenGL_Shadow_Mapping
渲染结果:
三、全部代码及模型文件
全部代码以及模型文件可以在[OpenGL]使用OpenGL实现硬阴影效果中下载。