演示效果:
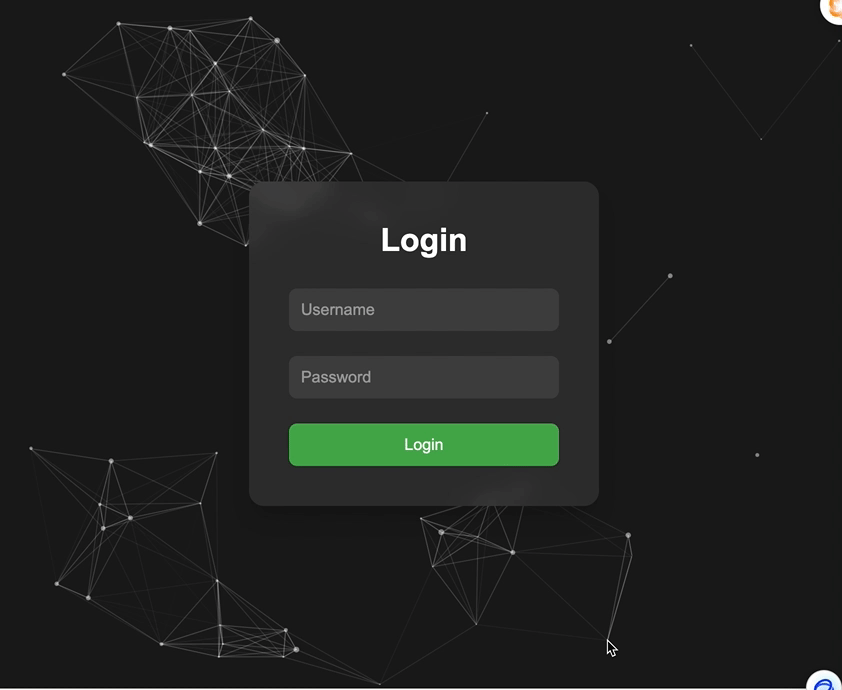
代码如下:
html
<!DOCTYPE html>
<html>
<head>
<style>
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
display: flex;
justify-content: center;
align-items: center;
min-height: 100vh;
background: #1a1a1a;
font-family: Arial, sans-serif;
}
#particles-js {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
z-index: 1;
}
.login-container {
position: relative;
z-index: 2;
background: rgba(255, 255, 255, 0.1);
padding: 40px;
border-radius: 15px;
backdrop-filter: blur(10px);
box-shadow: 0 15px 25px rgba(0, 0, 0, 0.2);
width: 350px;
transition: all 0.3s ease;
}
.login-container:hover {
transform: translateY(-5px);
box-shadow: 0 20px 30px rgba(0, 0, 0, 0.3);
}
.login-container h2 {
color: #fff;
text-align: center;
margin-bottom: 30px;
font-size: 2em;
font-weight: 600;
}
.input-group {
margin-bottom: 25px;
position: relative;
}
.input-group input {
width: 100%;
padding: 12px;
border: none;
background: rgba(255, 255, 255, 0.1);
border-radius: 8px;
color: #fff;
outline: none;
font-size: 16px;
transition: all 0.3s ease;
}
.input-group input:focus {
background: rgba(255, 255, 255, 0.15);
box-shadow: 0 0 10px rgba(255, 255, 255, 0.1);
}
.input-group input::placeholder {
color: rgba(255, 255, 255, 0.5);
}
button {
width: 100%;
padding: 12px;
border: none;
background: #4CAF50;
color: #fff;
border-radius: 8px;
cursor: pointer;
font-size: 16px;
font-weight: 500;
transition: all 0.3s ease;
position: relative;
overflow: hidden;
}
button:hover {
background: #45a049;
transform: translateY(-2px);
box-shadow: 0 5px 15px rgba(76, 175, 80, 0.3);
}
button:active {
transform: translateY(0);
}
/* Add ripple effect */
button .ripple {
position: absolute;
background: rgba(255, 255, 255, 0.3);
border-radius: 50%;
transform: scale(0);
animation: ripple 0.6s linear;
}
@keyframes ripple {
to {
transform: scale(4);
opacity: 0;
}
}
</style>
</head>
<body>
<div id="particles-js"></div>
<div class="login-container">
<h2>Login</h2>
<div class="input-group">
<input type="text" placeholder="Username" autocomplete="off">
</div>
<div class="input-group">
<input type="password" placeholder="Password">
</div>
<button id="loginBtn">Login</button>
</div>
<!-- 加载 particles.js -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/particles.js/2.0.0/particles.min.js"></script>
<script>
// Particles.js 配置
particlesJS("particles-js", {
"particles": {
"number": {
"value": 80,
"density": {
"enable": true,
"value_area": 800
}
},
"color": {
"value": "#ffffff"
},
"shape": {
"type": "circle"
},
"opacity": {
"value": 0.5,
"random": false,
"anim": {
"enable": false
}
},
"size": {
"value": 3,
"random": true,
"anim": {
"enable": false
}
},
"line_linked": {
"enable": true,
"distance": 150,
"color": "#ffffff",
"opacity": 0.4,
"width": 1
},
"move": {
"enable": true,
"speed": 2,
"direction": "none",
"random": false,
"straight": false,
"out_mode": "bounce",
"bounce": false,
"attract": {
"enable": true,
"rotateX": 600,
"rotateY": 1200
}
}
},
"interactivity": {
"detect_on": "canvas",
"events": {
"onhover": {
"enable": true,
"mode": "grab"
},
"onclick": {
"enable": true,
"mode": "push"
},
"resize": true
},
"modes": {
"grab": {
"distance": 140,
"line_linked": {
"opacity": 1
}
},
"push": {
"particles_nb": 4
}
}
},
"retina_detect": true
});
// 添加按钮点击波纹效果
document.getElementById('loginBtn').addEventListener('click', function(e) {
let ripple = document.createElement('span');
ripple.classList.add('ripple');
this.appendChild(ripple);
let x = e.clientX - e.target.offsetLeft;
let y = e.clientY - e.target.offsetTop;
ripple.style.left = x + 'px';
ripple.style.top = y + 'px';
setTimeout(() => {
ripple.remove();
}, 600);
});
// 添加输入框聚焦效果
document.querySelectorAll('.input-group input').forEach(input => {
input.addEventListener('focus', function() {
this.parentElement.classList.add('focused');
});
input.addEventListener('blur', function() {
if (!this.value) {
this.parentElement.classList.remove('focused');
}
});
});
</script>
</body>
</html>