**问题:**设计字符串类, 支持下面的操作
MyString s1;// 默认构造函数
MyString s2("hello");// 含参构造函数
MyString s3(s1); // 传参构造函数
MyString s4(5, 'c');// 自定义构造函数
=// 运算符重载
== != > < // 运算符重载
代码:
cpp
#include <iostream>
#include <cstring>
using namespace std;
class Mystring{
// <<
friend ostream& operator<<(ostream &out,Mystring &s);
// >>
friend istream& operator>>(istream &in,Mystring &s);
private:
char *m_space; // 存储数据
size_t m_len; // 字符串长度
public:
// 默认构造函数
Mystring(){
m_space = new char;
strcpy(m_space,"");
m_len = 0;
}
// 构造函数
Mystring(const char *indata){
m_len = strlen(indata);
m_space = new char[m_len + 1];
strcpy(m_space,indata);
}
// 拷贝构造函数(深拷贝)
Mystring(const Mystring &s){
m_len = s.m_len;
m_space = new char[m_len + 1];
strcpy(m_space,s.m_space);
}
// 析构函数
~Mystring(){
if(m_space != nullptr){
delete [] m_space;
m_space = nullptr;
}
}
// 重载赋值运算符 =
Mystring& operator=(const Mystring &s){
if(m_space != nullptr){
delete [] m_space;
m_space = nullptr;
}
m_len = s.m_len;
m_space = new char[m_len + 1];
strcpy(m_space,s.m_space);
return *this;
}
};
// <<
ostream& operator<<(ostream &out,Mystring &s){
out<<s.m_space;
return out;
}
istream& operator>>(istream &in,Mystring &s){
// 对之前的内存进行管理(避免内存泄漏)
if(s.m_space != nullptr){
delete [] s.m_space;
s.m_space = nullptr;
}
// 输入新的字符串
char buf[1024];
in>>buf;
s.m_len = strlen(buf);
s.m_space = new char[s.m_len + 1];
strcpy(s.m_space,buf);
return in;
}
int main(){
Mystring s1;
Mystring s2("Hello");
cout<<"Please input data:"<<endl;
cin>>s2;
s1 = s2;
cout<<"s2: "<<s2<<endl;
cout<<"s1: "<<s1<<endl;
return 0;
}
输出:
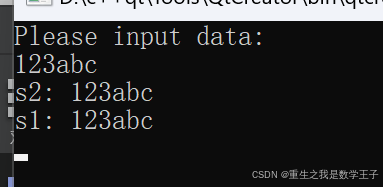