B3663 [语言月赛202209] Luogu Academic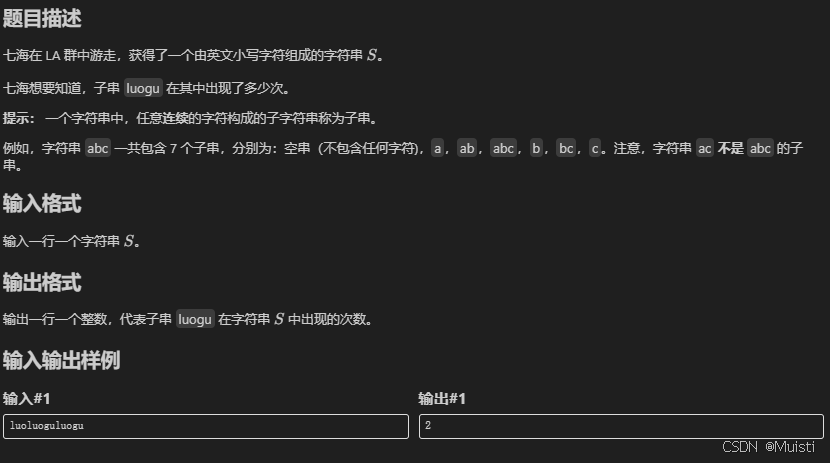
cpp
#include <iostream>
using namespace std;
# include <string.h>
#include <ctype.h>
#include <algorithm>
int main(){
string s;
cin>>s;
int cnt=0;
string s1= "luogu";
size_t index =s.find(s1);//返回第一个s1的位置,指的是L这个字符所在位置,而不是u字符
while(index != string::npos){
cnt++;
index = s.find(s1,index+5);
// index = s.find(s1,index+1);
}
cout<<cnt;
}
代码逻辑解释
这段代码使用了 `string` 的 `find` 函数来查找子串 `"luogu"` 在字符串 `s` 中的出现次数。具体逻辑如下:
- **初始化目标子串和计数器**:
```cpp
string target = "luogu";
int count = 0;
```
-
目标子串为 `"luogu"`。
-
计数器 `count` 初始化为 `0`,用于记录子串出现的次数。
- **使用 `find` 函数查找子串的第一次出现**:
```cpp
size_t pos = s.find(target);
```
-
`s.find(target)` 在字符串 `s` 中查找子串 `target`(即 `"luogu"`)的第一次出现位置,并返回它的起始索引位置(`size_t` 类型)。
-
如果 `find` 没有找到子串,会返回 `string::npos`(一个特殊值,表示"未找到")。
- **循环查找剩余的子串**:
```cpp
while (pos != string::npos) {
count++;
pos = s.find(target, pos + 1);
}
```
-
只要 `pos != string::npos`,说明子串还存在于字符串 `s` 中,进入循环。
-
每次找到 `target`,计数器 `count` 加 `1`。
-
然后,`s.find(target, pos + 1)` 会从当前匹配位置的下一个字符开始继续搜索。`pos + 1` 是为了确保不计数重叠部分的子串,并且从找到的子串之后开始新的搜索。
- **输出结果**:
```cpp
cout << count << endl;
```
- 输出子串 `"luogu"` 在字符串 `s` 中的出现次数。
`find` 函数的使用说明
- **用法**:
```cpp
size_t pos = s.find(substring);
size_t pos = s.find(substring, startIndex);
```
-
`s.find(substring)`:在字符串 `s` 中查找 `substring` 的第一次出现,返回它的起始位置。如果找不到,返回 `string::npos`。
-
`s.find(substring, startIndex)`:从 `startIndex` 开始,在字符串 `s` 中查找 `substring` 的第一次出现。
-
**特性**:
-
`find` 函数不会返回重叠的子串的索引。每次找到目标子串后,下一次搜索会从当前匹配位置的下一个字符开始。
-
`string::npos` 是一个常量值,表示"找不到目标子串"。
代码运行示例
示例 1:
-
输入字符串:`"luluoguluogu"`
-
输出:`1`
-
只有一次非重叠的子串 `"luogu"`。
示例 2:
-
输入字符串:`"luoguluoguluogu"`
-
输出:`3`
-
子串 `"luogu"` 在 `s` 中出现了三次。
总结
-
`find` 函数用于搜索子串在字符串中的位置。
-
使用 `while (pos != string::npos)` 循环可以遍历所有出现的子串。
-
每次使用 `pos + 1` 作为起点确保从当前匹配位置的下一个字符继续搜索。
是的,`find` 函数返回的是子串在字符串中首次出现的 **从0开始的下标位置**。如果子串在字符串中存在,`find` 会返回它的起始位置索引,否则返回 `string::npos` 表示未找到。
示例
```cpp
#include <iostream>
#include <string>
using namespace std;
int main() {
string s = "hello world";
size_t pos = s.find("world");
if (pos != string::npos) {
cout << "Found at index: " << pos << endl;
} else {
cout << "Not found" << endl;
}
return 0;
}
```
-
在这个例子中,`s.find("world")` 会返回 `6`,因为子串 `"world"` 从索引 `6` 开始。
-
如果找不到子串,`find` 会返回 `string::npos`。
结论
-
**`find` 的返回值是一个从0开始的索引**,表示子串在字符串中的起始位置。
-
这种索引机制与大多数编程语言的字符串处理方式一致,即索引从 `0` 开始计数。