添加依赖
在 build.gradle
文件中添加 OkHttp 依赖:
dependencies {
implementation("com.squareup.okhttp3:okhttp:4.10.0")
}
使用OkHttp发起GET请求
同步请求
java
public class MainActivity extends AppCompatActivity {
// Used to load the 'okhttptest' library on application startup.
static {
System.loadLibrary("okhttptest");
}
private ActivityMainBinding binding;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
binding = ActivityMainBinding.inflate(getLayoutInflater());
setContentView(binding.getRoot());
// Example of a call to a native method
TextView tv = binding.sampleText;
tv.setText(stringFromJNI());
new Thread(new Runnable() {
@Override
public void run() {
// 创建 OkHttpClient
OkHttpClient client = new OkHttpClient();
// 创建 Request 对象
Request request = new Request.Builder()
.url("https://www.httpbin.org/get") // URL
.build();
// 同步请求
try (Response response = client.newCall(request).execute()){
if(response.isSuccessful()){
String responseBody = response.body().string(); // 读取响应体
Log.d("Response:",responseBody);
// 在主线程中显示 Toast
runOnUiThread(new Runnable() {
@Override
public void run() {
// 显示 Toast
Toast.makeText(MainActivity.this, responseBody, Toast.LENGTH_SHORT).show();
}
});
}else {
Log.e("Request failed", String.valueOf(response.code()));
}
} catch (IOException e) {
throw new RuntimeException(e);
}
}
}).start();
}
public native String stringFromJNI();
}
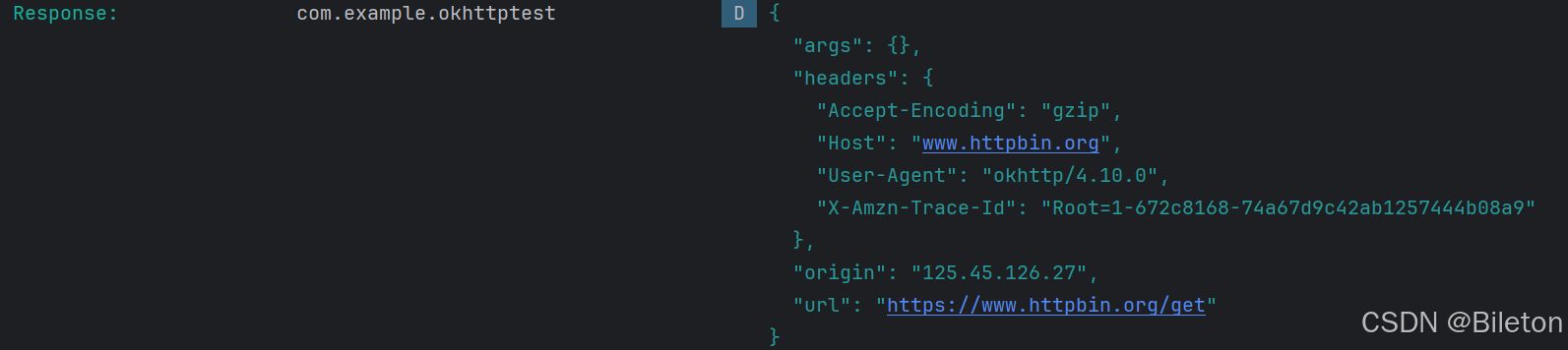
异步请求
java
public class MainActivity extends AppCompatActivity {
// Used to load the 'okhttptest' library on application startup.
static {
System.loadLibrary("okhttptest");
}
private ActivityMainBinding binding;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
binding = ActivityMainBinding.inflate(getLayoutInflater());
setContentView(binding.getRoot());
// Example of a call to a native method
TextView tv = binding.sampleText;
tv.setText(stringFromJNI());
new Thread(new Runnable() {
@Override
public void run() {
// 创建 OkHttpClient
OkHttpClient client = new OkHttpClient();
// 创建 Request 对象
Request request = new Request.Builder()
.url("https://www.httpbin.org/get") // URL
.build();
// 同步请求
client.newCall(request).enqueue(new Callback() {
@Override
public void onFailure(@NonNull Call call, @NonNull IOException e) {
Log.e("Request failed: ",e.getMessage());
}
@Override
public void onResponse(@NonNull Call call, @NonNull Response response) throws IOException {
String ResponseBody = response.body().string();
if (response.isSuccessful()){
Log.e("Response: " ,ResponseBody);
runOnUiThread(new Runnable() {
@Override
public void run() {
Toast.makeText(MainActivity.this,ResponseBody,Toast.LENGTH_SHORT).show();
}
});
}else {
Log.e("Request failed:",String.valueOf(response.code()));
}
}
});
}
}).start();
}
public native String stringFromJNI();
}
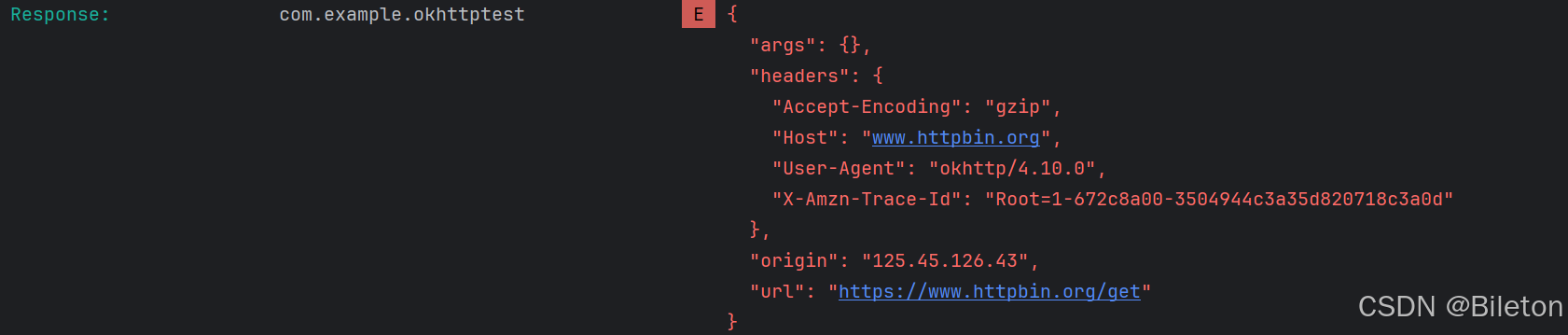
POST请求
允许通过HTTP访问网络资源,Android 默认禁止应用使用非加密(HTTP)通信,以保护用户数据的安全。默认情况下,Android 9(API 级别 28)及更高版本需要通过 HTTPS 进行网络通信。
通过修改AndroidManifest.xml文件来临时允许明文HTTP流量。
xml
<application
android:usesCleartextTraffic="true"
...
</application>
发送请求
java
public class MainActivity extends AppCompatActivity {
// Used to load the 'okhttptest' library on application startup.
static {
System.loadLibrary("okhttptest");
}
private ActivityMainBinding binding;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
binding = ActivityMainBinding.inflate(getLayoutInflater());
setContentView(binding.getRoot());
// Example of a call to a native method
TextView tv = binding.sampleText;
tv.setText(stringFromJNI());
new Thread(new Runnable() {
@Override
public void run() {
// 创建 OkHttpClient
OkHttpClient client = new OkHttpClient();
RequestBody formBody = new FormBody.Builder()
.add("name","Bileton")
.add("age","21")
.build();
// 创建 Request 对象
Request request = new Request.Builder()
.url("http://www.httpbin.org/post") // URL
.post(formBody)
.build();
// 同步请求
client.newCall(request).enqueue(new Callback() {
@Override
public void onFailure(@NonNull Call call, @NonNull IOException e) {
Log.e("Request failed: ",e.getMessage());
}
@Override
public void onResponse(@NonNull Call call, @NonNull Response response) throws IOException {
String ResponseBody = response.body().string();
if (response.isSuccessful()){
Log.e("Response: " ,ResponseBody);
runOnUiThread(new Runnable() {
@Override
public void run() {
Toast.makeText(MainActivity.this,ResponseBody,Toast.LENGTH_SHORT).show();
}
});
}else {
Log.e("Request failed:",String.valueOf(response.code()));
}
}
});
}
}).start();
}
public native String stringFromJNI();
}
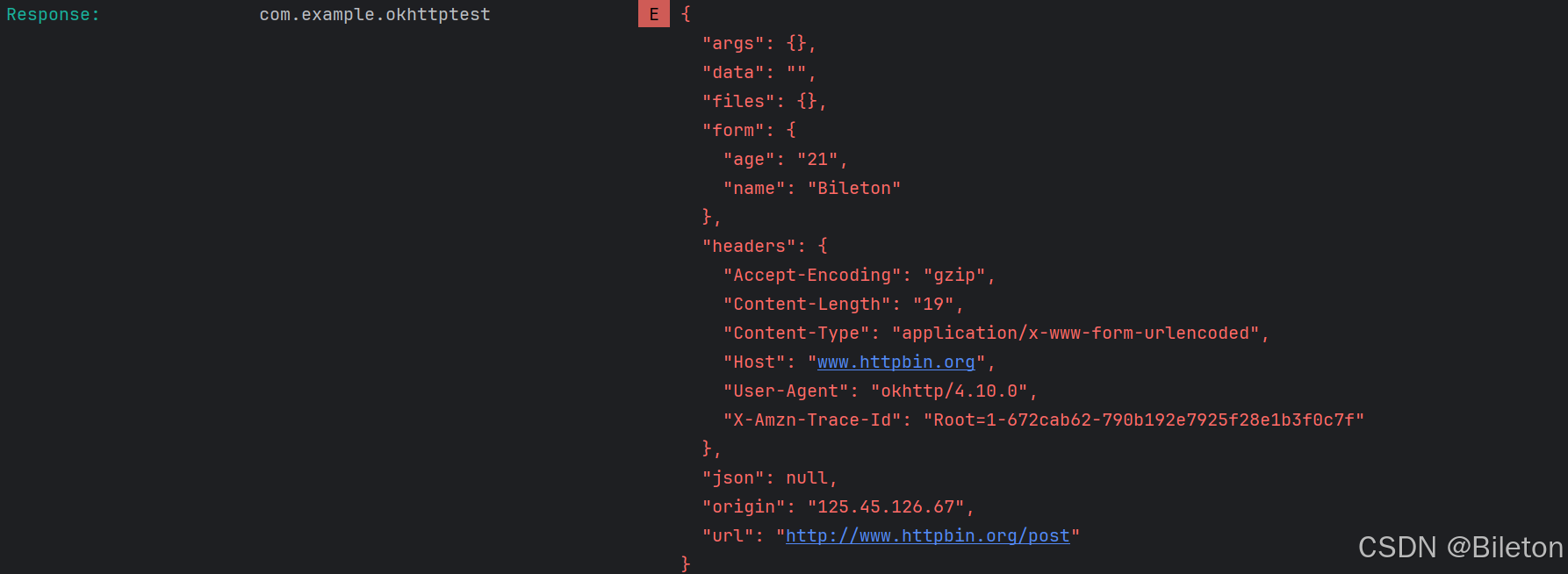
拦截器
OkHttp 的拦截器是一个非常强大的工具,可以在请求和响应过程中拦截和修改 HTTP 请求和响应。拦截器可以用于日志记录、修改请求/响应、添加通用头部信息、缓存等功能。
OkHttp提供了两种类型的拦截器:
-
应用拦截器(Application Interceptor)
应用拦截器可以在请求被发送之前或响应到达客户端之后进行拦截。应用拦截器不会直接影响网络层操作。
javaOkHttpClient client = new OkHttpClient.Builder() .addInterceptor(new Interceptor() { @Override public Response intercept(Chain chain) throws IOException { Request originalRequest = chain.request(); // 添加头部信息 Request modifiedRequest = originalRequest.newBuilder() .header("Authorization", "Bearer your_token") .build(); // 继续链条中的下一个拦截器 return chain.proceed(modifiedRequest); } }) .build();
-
网络拦截器(Network Interceptor)
网络拦截器更接近网络层,能访问和修改在网络上传输的数据。它们可以用来实现诸如缓存和压缩的功能。
javaOkHttpClient client = new OkHttpClient.Builder() .addNetworkInterceptor(new Interceptor() { @Override public Response intercept(Chain chain) throws IOException { Request request = chain.request(); // 打印请求信息 long startTime = System.nanoTime(); System.out.println(String.format("Sending request %s on %s%n%s", request.url(), chain.connection(), request.headers())); Response response = chain.proceed(request); // 打印响应信息 long endTime = System.nanoTime(); System.out.println(String.format("Received response for %s in %.1fms%n%s", response.request().url(), (endTime - startTime) / 1e6d, response.headers())); return response; } }) .build();
在请求中使用拦截器
java
OkHttpClient client = new OkHttpClient.Builder()
.addInterceptor(new CustomInterceptor()) // 添加应用拦截器
.addNetworkInterceptor(new CustomNetworkInterceptor()) // 添加网络拦截器
.build();
Request request = new Request.Builder()
.url("https://www.example.com")
.build();
client.newCall(request).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
e.printStackTrace();
}
@Override
public void onResponse(Call call, Response response) throws IOException {
if (response.isSuccessful()) {
System.out.println(response.body().string());
}
}
});