Android XML 约束布局 参考
TextView居中
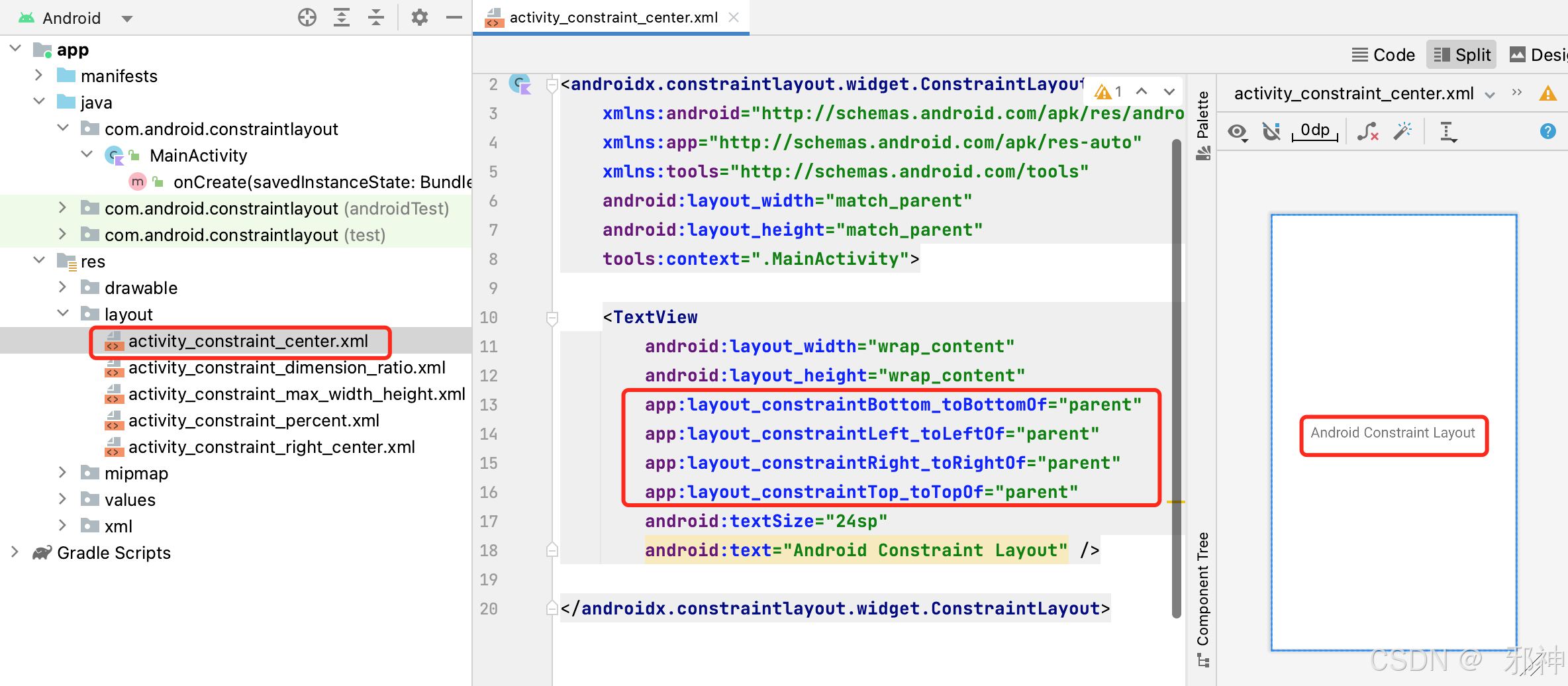
TextView 垂直居中并且靠右
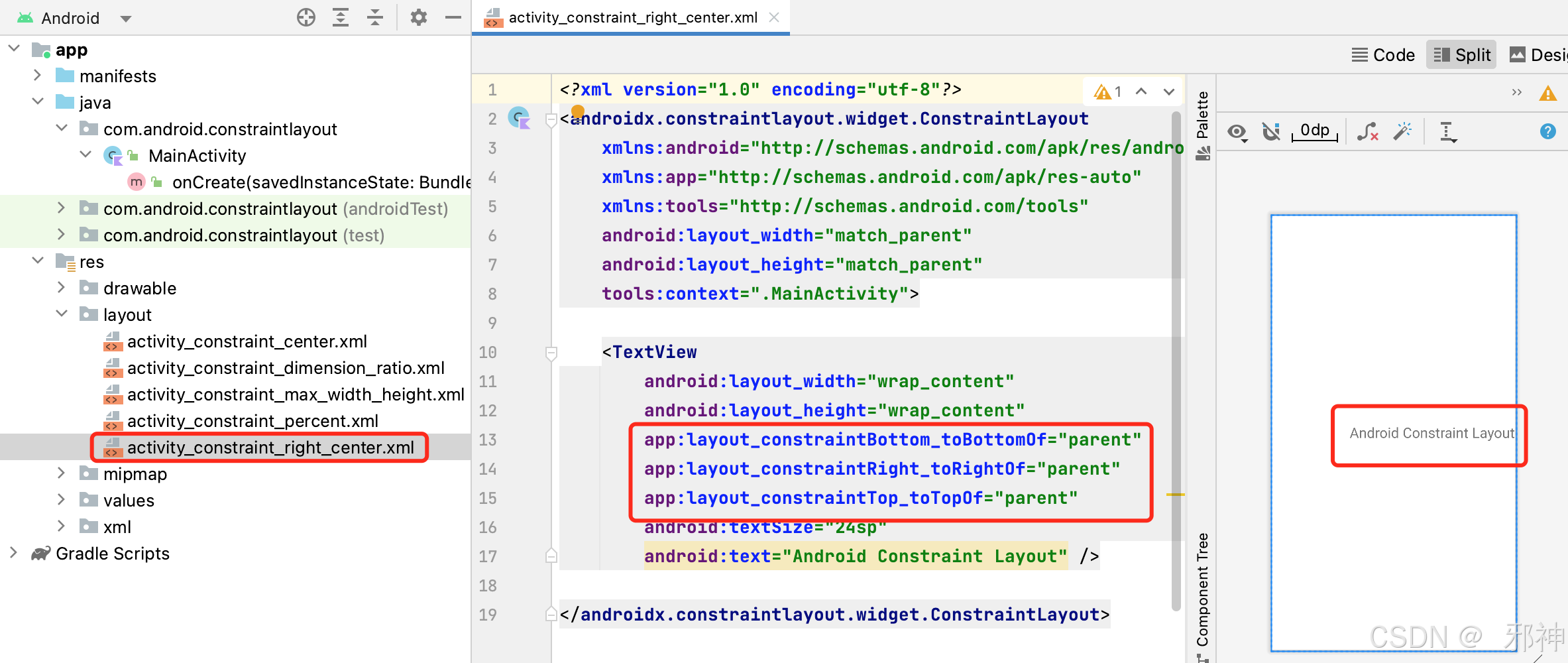
TextView 宽高设置百分比
宽和高的比例
app:layout_constraintDimensionRatio="h,2:1"
表示子视图的宽高比为2:1,其中 h
表示保持宽度不变,高度自动调整。
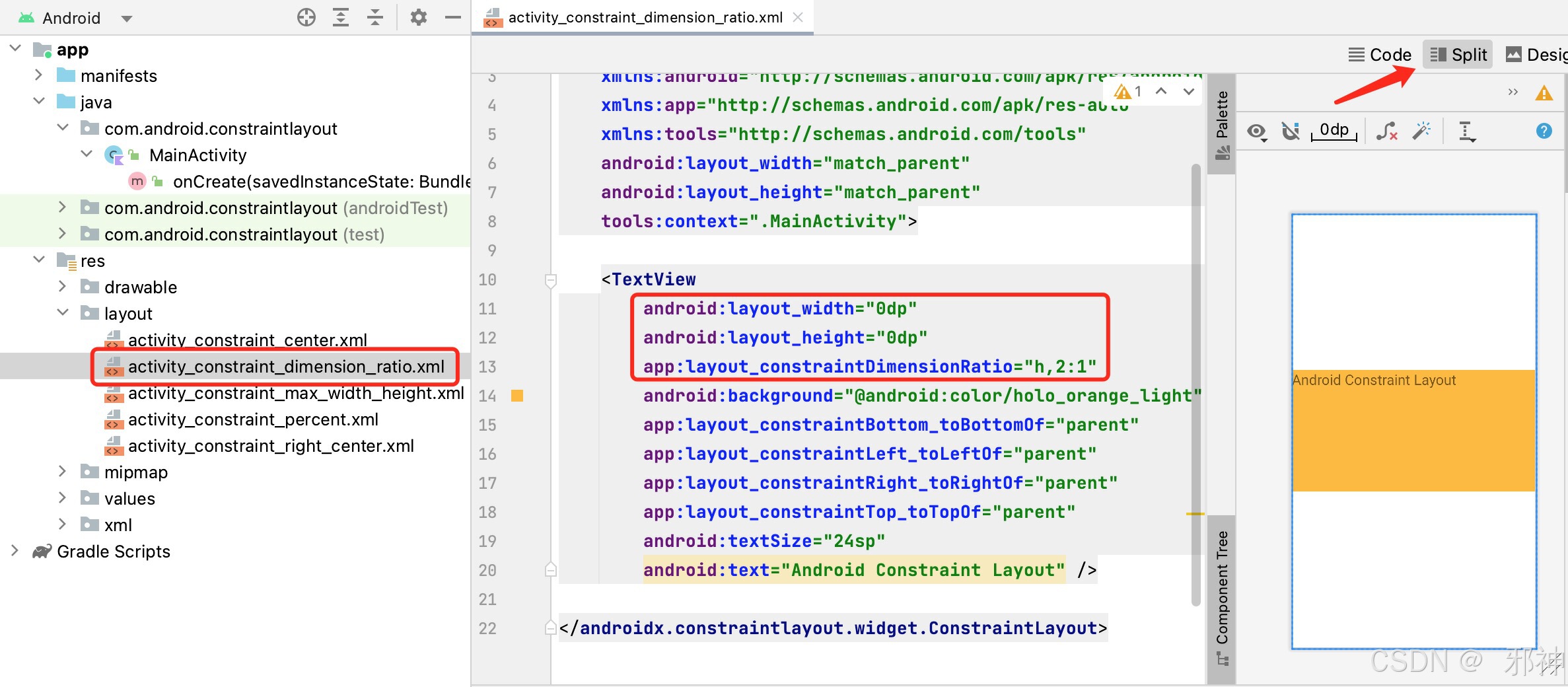
最大宽度
设置最大宽度,超过这个宽度就会发生换行。
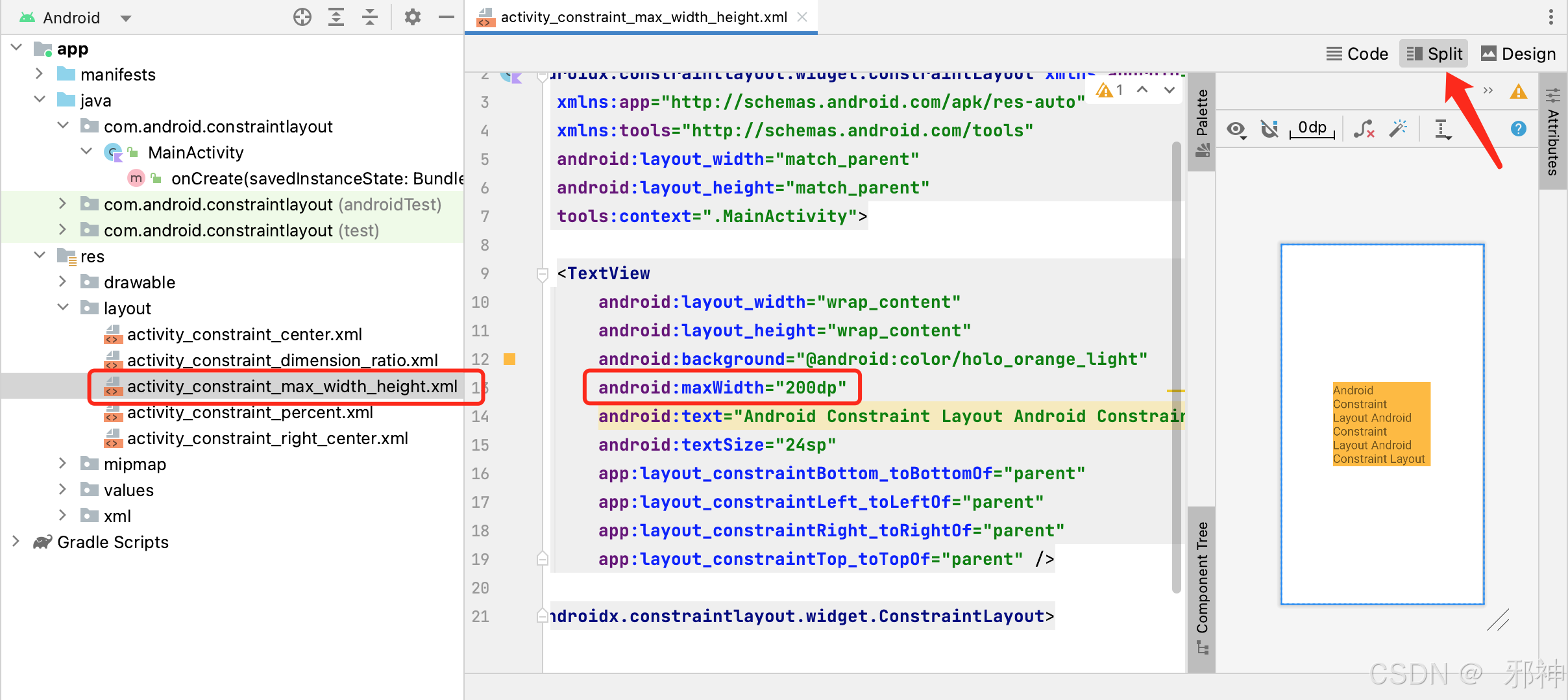
Android Compose 约束布局
导入依赖包
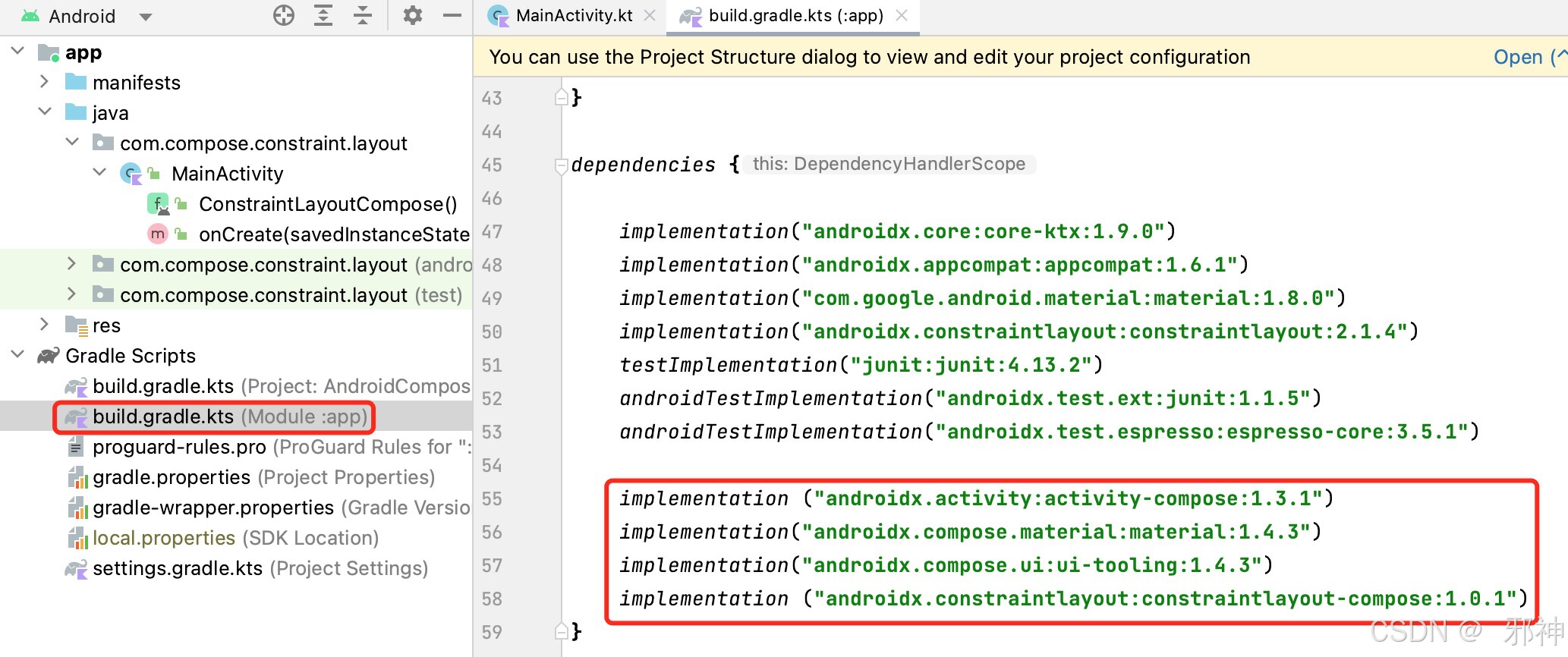
启用Compose
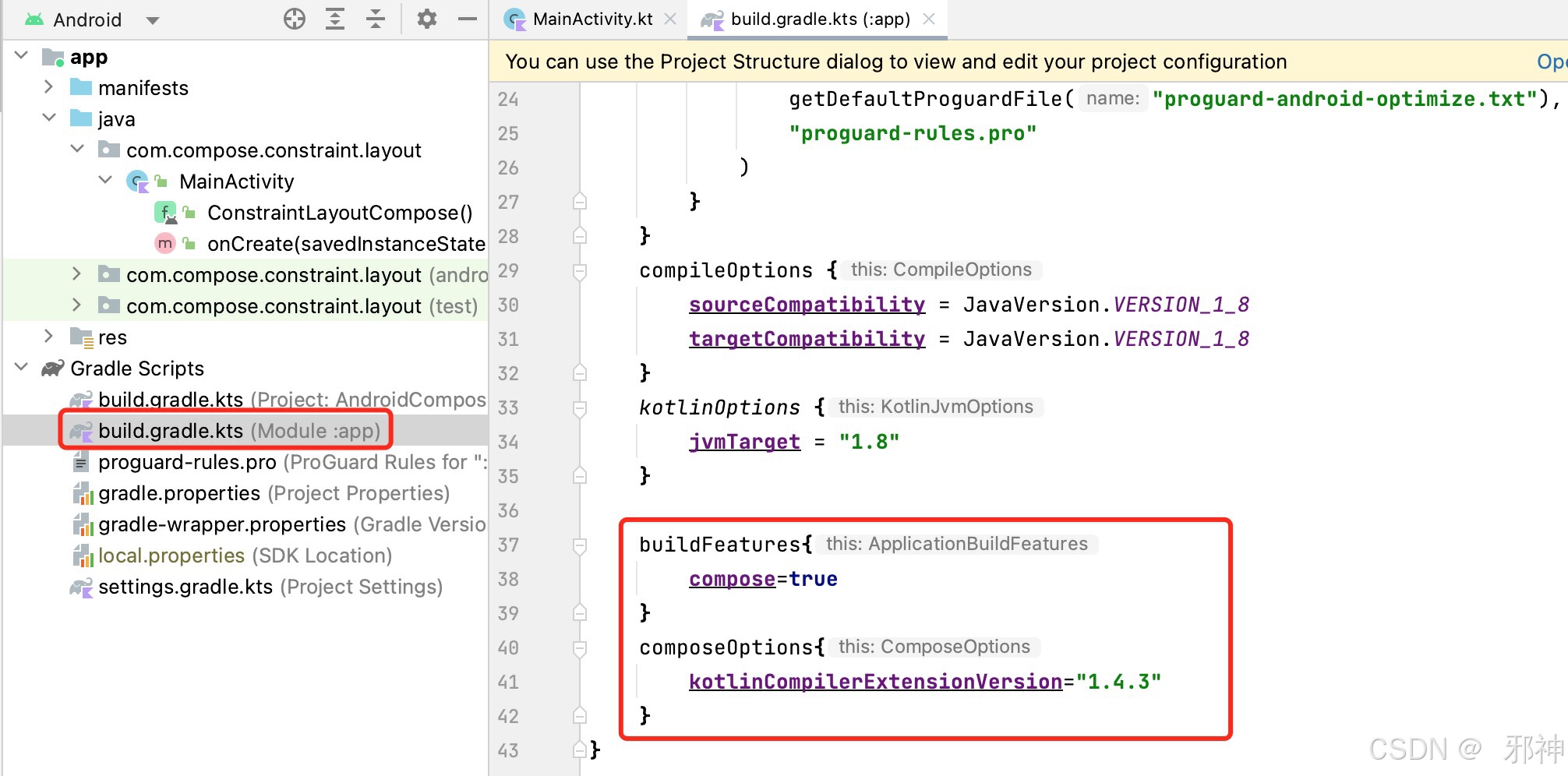
Compose Text居中
Dart
//Compose Text居中
@Preview
@Composable
fun ConstraintLayoutCompose() {
ConstraintLayout(modifier = Modifier.fillMaxSize()) {
val (text) = createRefs()
Text(
text = "Text 居中",
modifier = Modifier
.width(180.dp)// 宽度
.height(200.dp)// 高度
.background(color = Color.Cyan)
.constrainAs(text) {
start.linkTo(parent.start)//位于父视图开始
end.linkTo(parent.end)//位于父视图结束
top.linkTo(parent.top)//位于父视图顶部
bottom.linkTo(parent.bottom)//位于父视图底部
},
textAlign = TextAlign.Center,
)
}
}
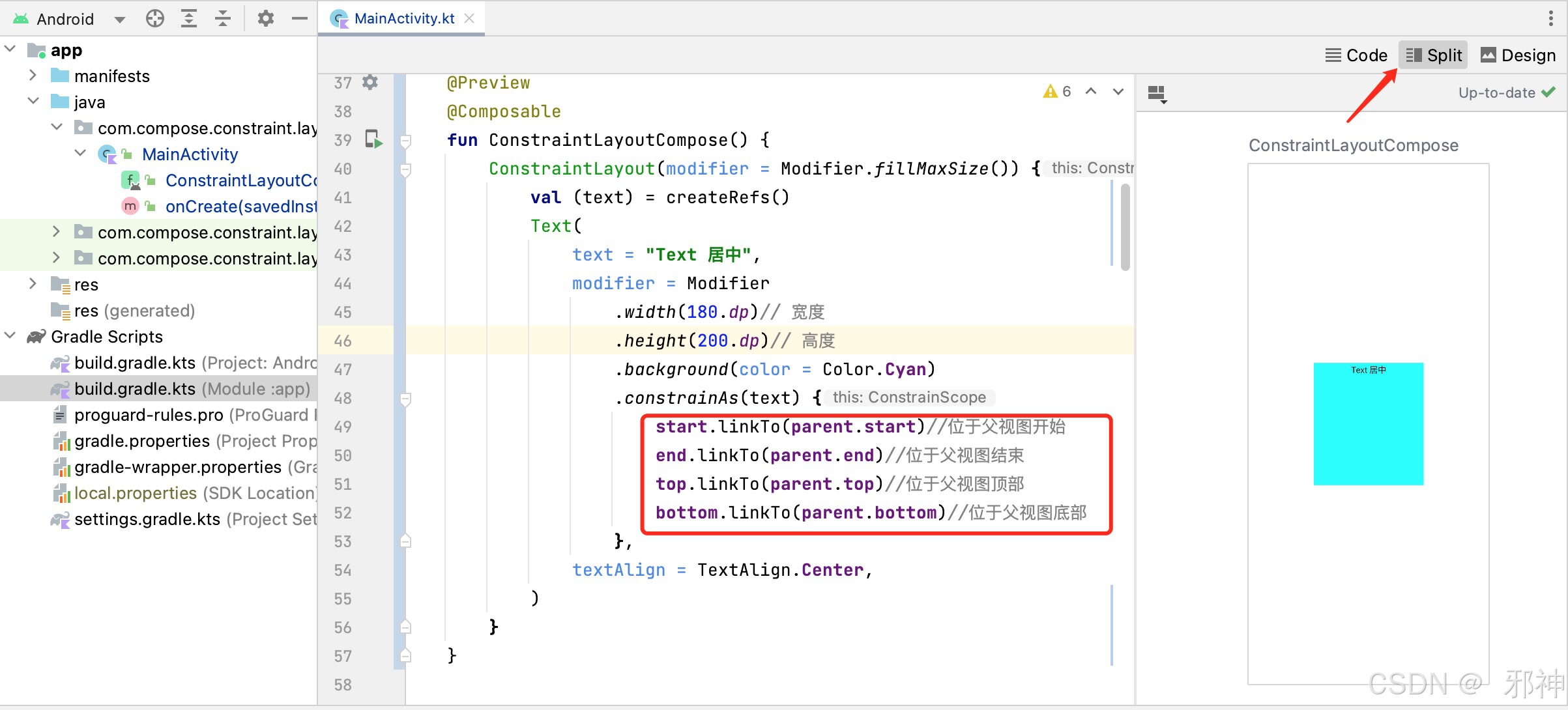
Compose Text 垂直居中并且靠右
Dart
// Compose Text 垂直居中并且靠右
@Preview
@Composable
fun ConstraintLayoutCompose() {
ConstraintLayout(modifier = Modifier.fillMaxSize()) {
val (text) = createRefs()
Text(
text = "Text 居中",
modifier = Modifier
.width(180.dp)// 宽度
.height(200.dp)// 高度
.background(color = Color.Cyan)
.constrainAs(text) {
end.linkTo(parent.end)//位于父视图结束
top.linkTo(parent.top)//位于父视图顶部
bottom.linkTo(parent.bottom)//位于父视图底部
},
textAlign = TextAlign.Center,
)
}
}
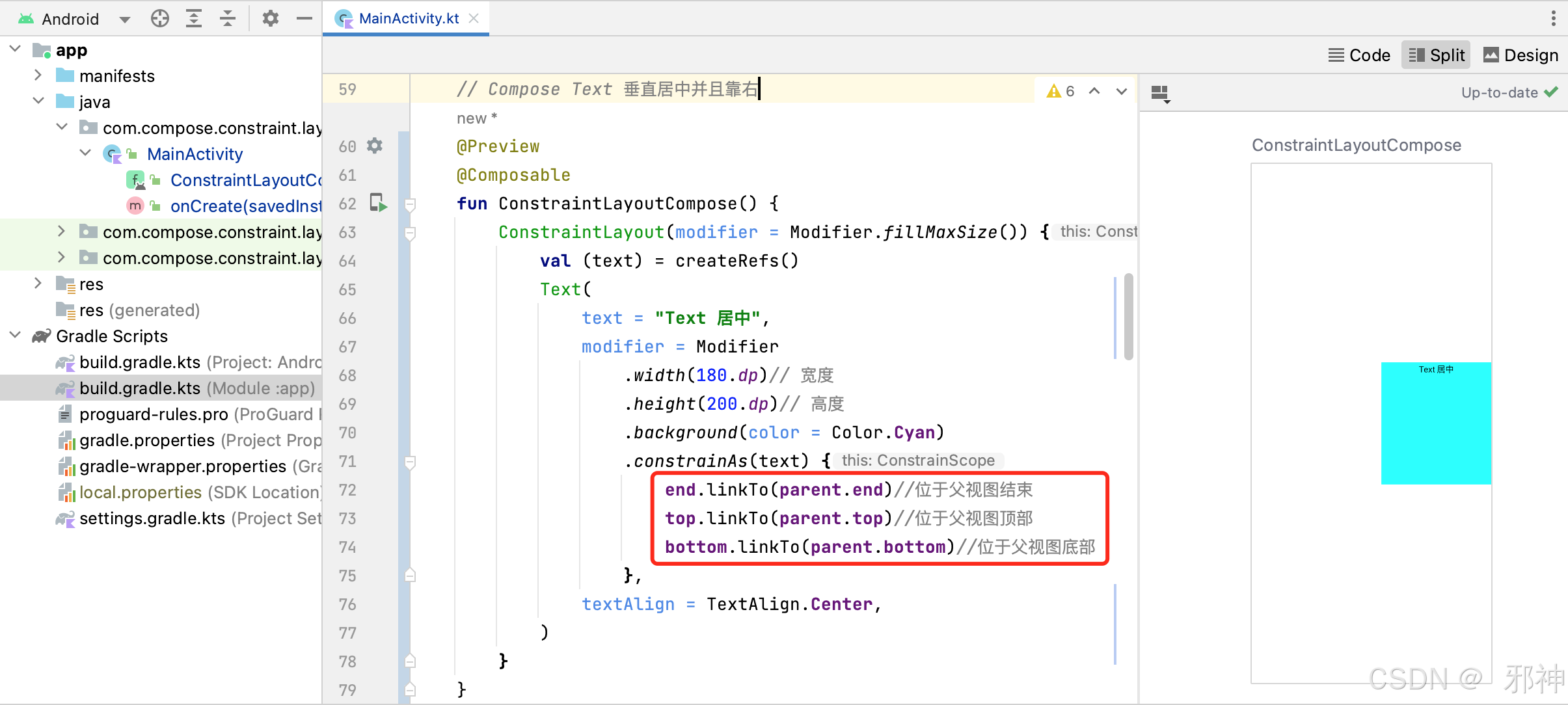
Compose Text 宽高设置百分比
Dart
// Compose Text 宽高设置百分比
@Preview
@Composable
fun ConstraintLayoutCompose() {
ConstraintLayout(modifier = Modifier.fillMaxSize()) {
val (text) = createRefs()
Text(
text = "Text 居中",
modifier = Modifier
.background(color = Color.Cyan)
.constrainAs(text) {
start.linkTo(parent.start) // 位于父视图开始
end.linkTo(parent.end)//位于父视图结束
top.linkTo(parent.top)//位于父视图顶部
bottom.linkTo(parent.bottom)//位于父视图底部
// 设置宽度为父视图宽度的50%
width = Dimension.percent(0.5F)
// 设置高度为父视图宽度的50%
height = Dimension.percent(0.5F)
},
textAlign = TextAlign.Center,
)
}
}
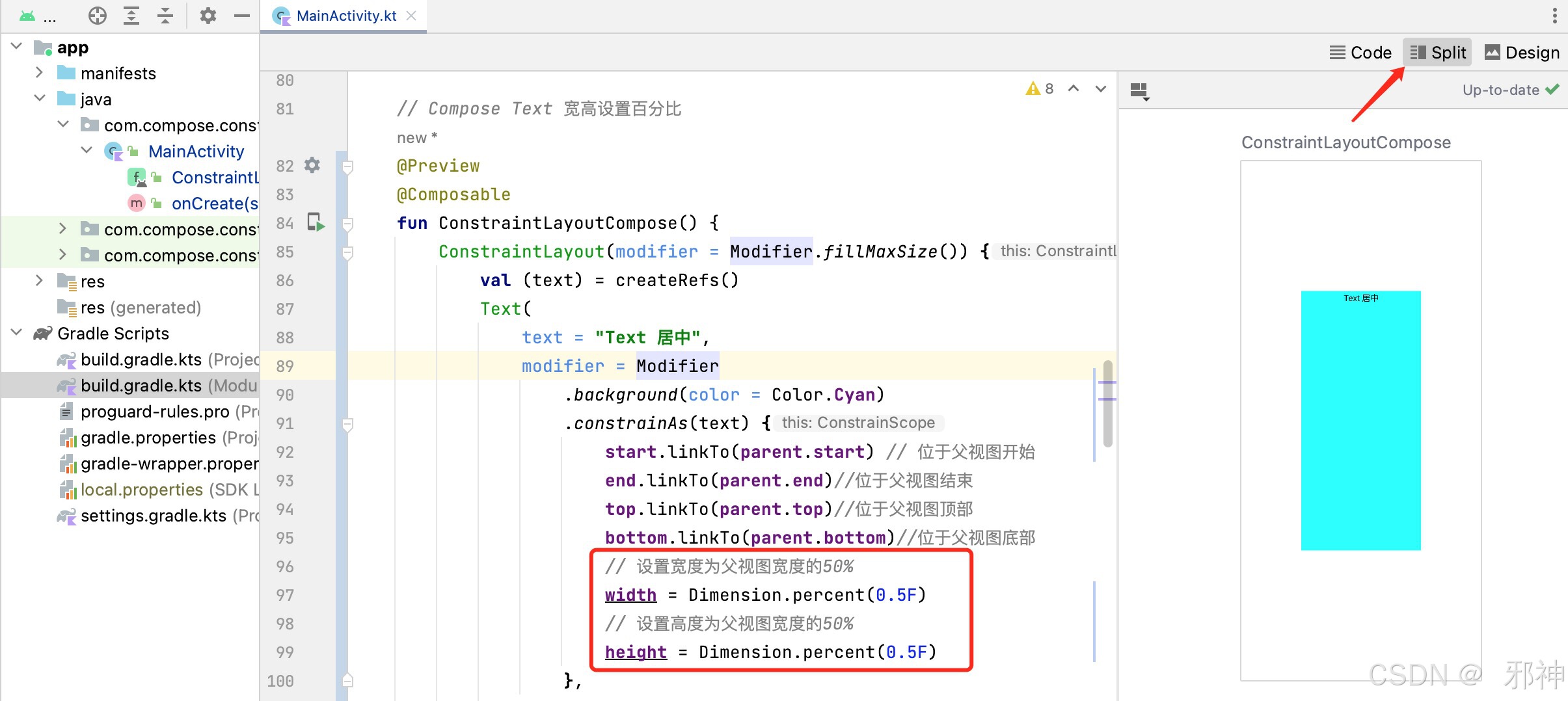
Compose Text 宽和高的比例 2:1
Dart
// Compose Text 宽和高的比例 2:1
@Preview
@Composable
fun ConstraintLayoutCompose() {
ConstraintLayout(modifier = Modifier.fillMaxSize()) {
val (text) = createRefs()
Text(
text = "Text 居中",
textAlign = TextAlign.Center,
modifier = Modifier
.background(color = Color.Cyan)
.constrainAs(text) {
start.linkTo(parent.start) // 位于父视图开始
end.linkTo(parent.end)//位于父视图结束
top.linkTo(parent.top)//位于父视图顶部
bottom.linkTo(parent.bottom)//位于父视图底部
// 设置宽度为父视图宽度的100%
width = Dimension.percent(1.0f)
}
.aspectRatio(2.0f)// 宽高比例 2:1
)
}
}
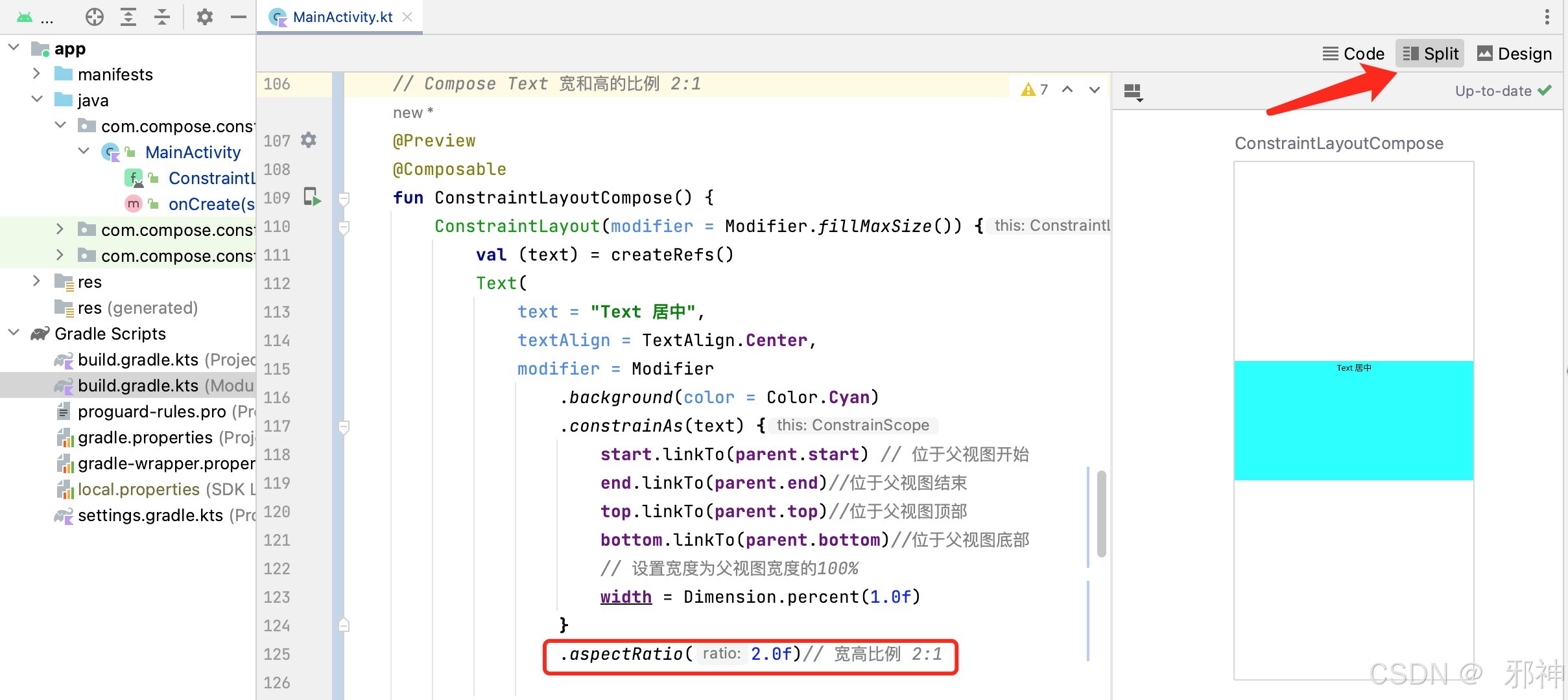
Compose Text 设置最大宽度
Dart
//Compose Text 设置最大宽度
@Preview
@Composable
fun ConstraintLayoutCompose() {
ConstraintLayout(modifier = Modifier.fillMaxSize()) {
val (text) = createRefs()
Text(
text = "Compose Text 设置最大宽度 Compose Text 设置最大宽度 Compose Text 设置最大宽度 ",
textAlign = TextAlign.Start,
modifier = Modifier
.background(color = Color.Cyan)
.widthIn(max = 160.dp) // 设置最大宽度
.constrainAs(text) {
start.linkTo(parent.start) // 位于父视图开始
end.linkTo(parent.end)//位于父视图结束
top.linkTo(parent.top)//位于父视图顶部
bottom.linkTo(parent.bottom)//位于父视图底部
}
)
}
}
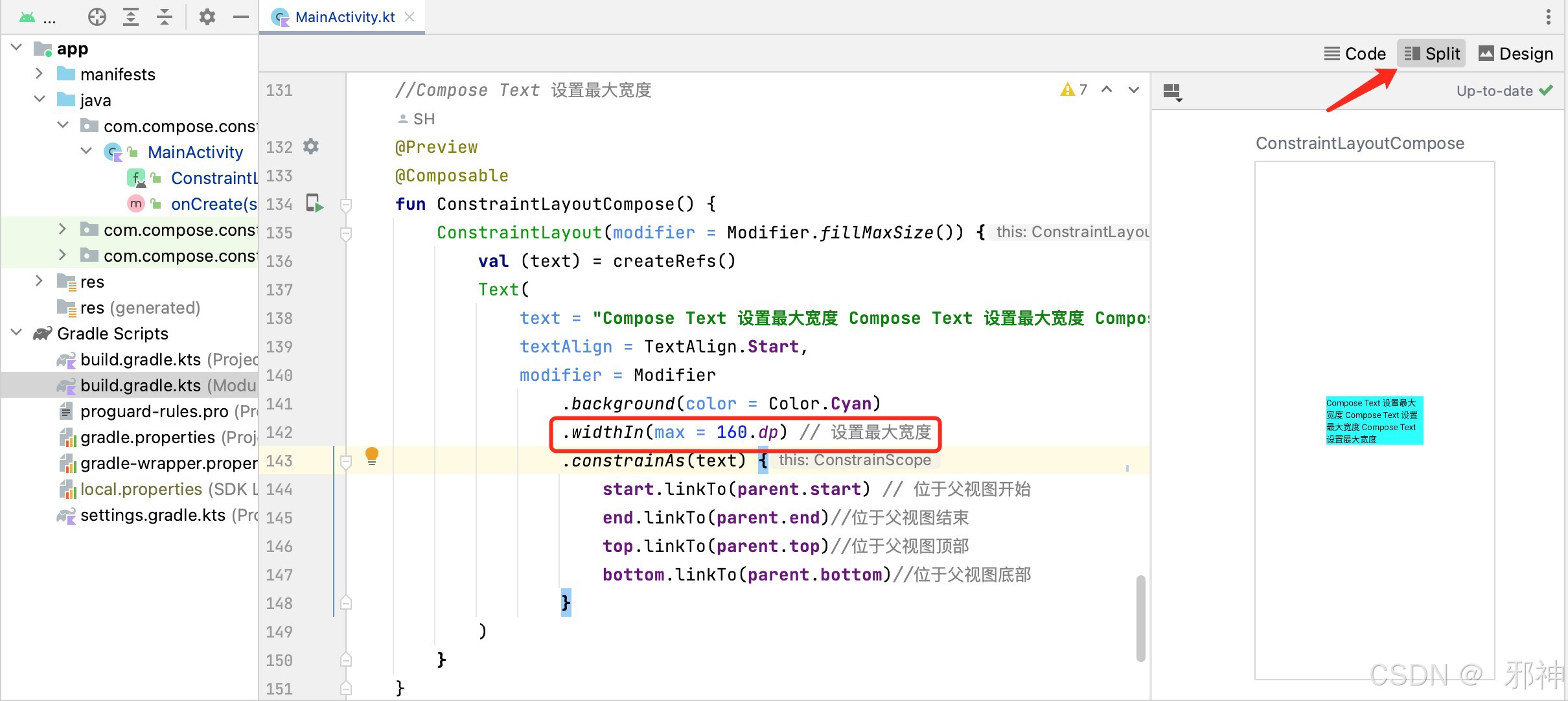
IOS Object-c 约束布局
pod init
如果不存在 Podfile 文件,切换到工程目录,就执行**pod init
**命令
pod install
配置 Masonry
依赖 ,然后执行 pod install
命令
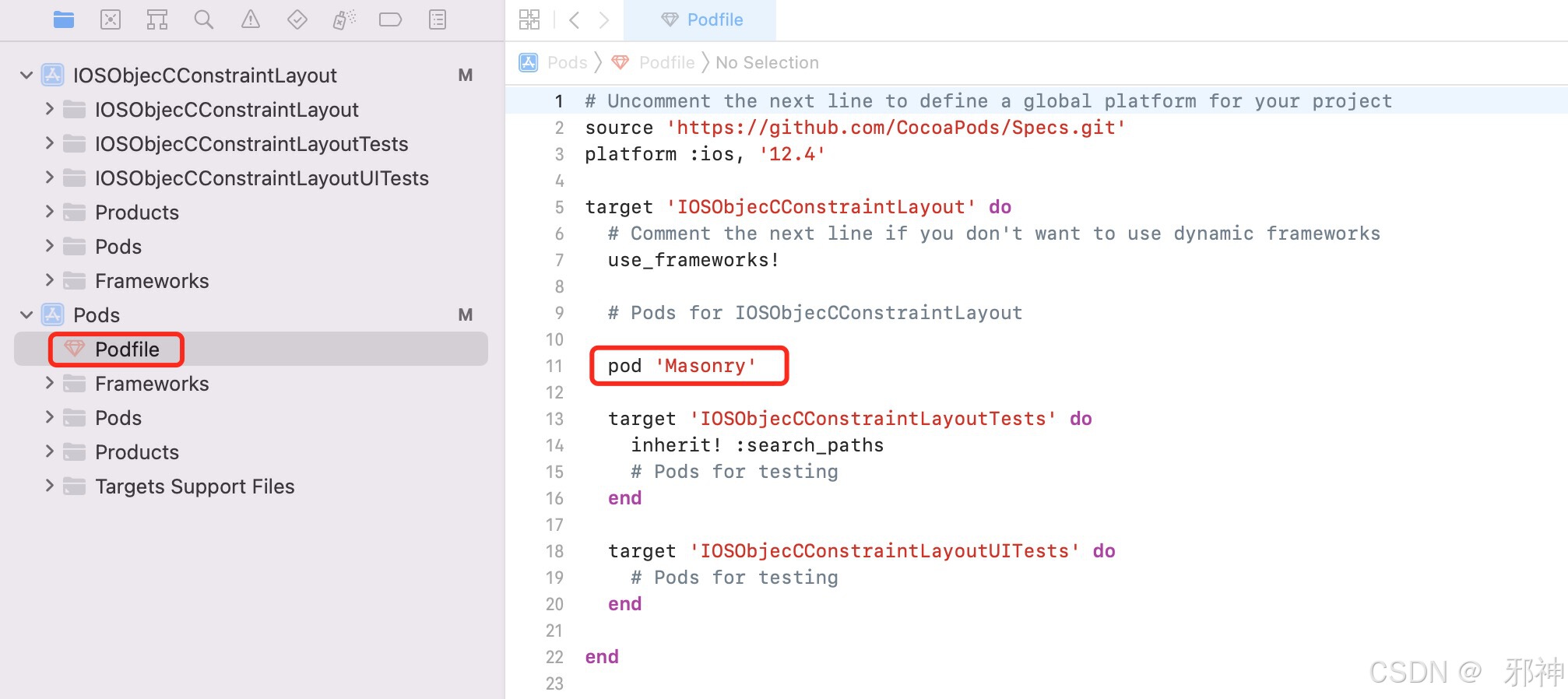
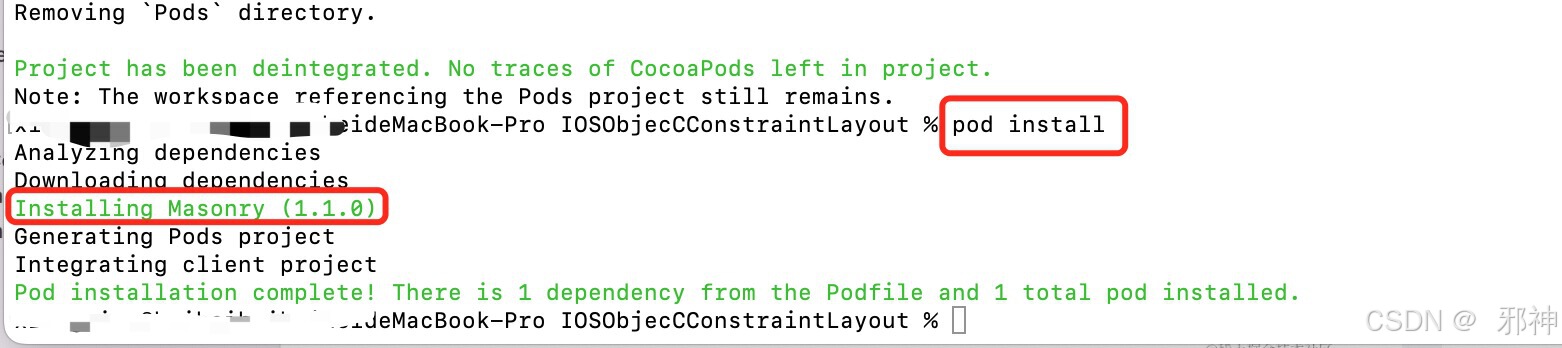
编译是遇到的问题 参考
file not found libarclite_iphonesimulator.a
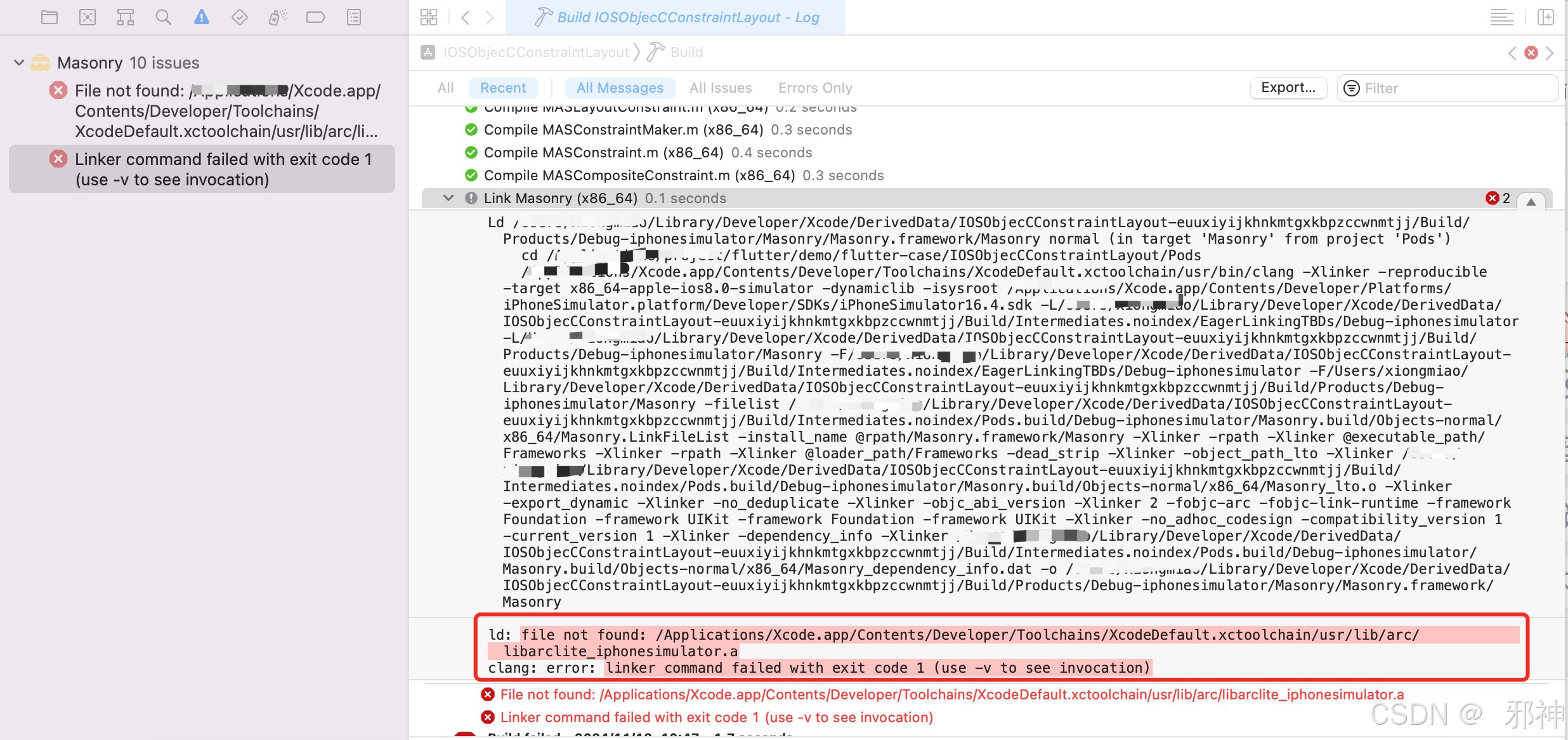
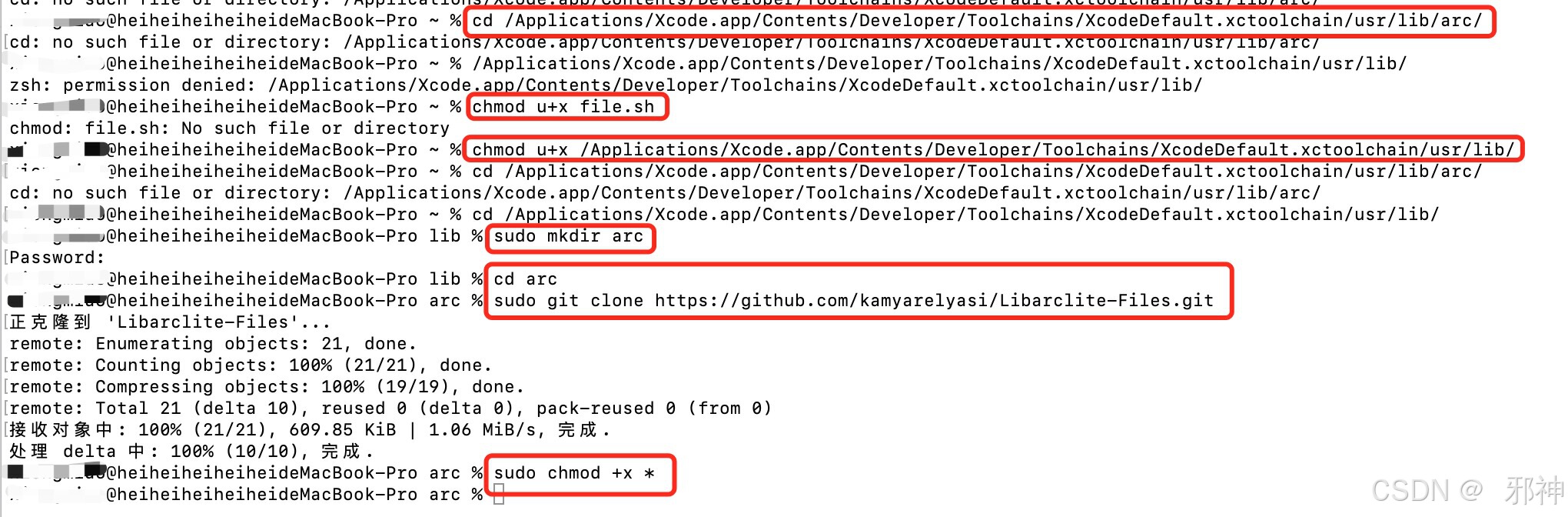
Podfile 中添加脚本 将Cocopods 引入的库的最低版本改为 iOS 11.0,再次执行 pod install 命令
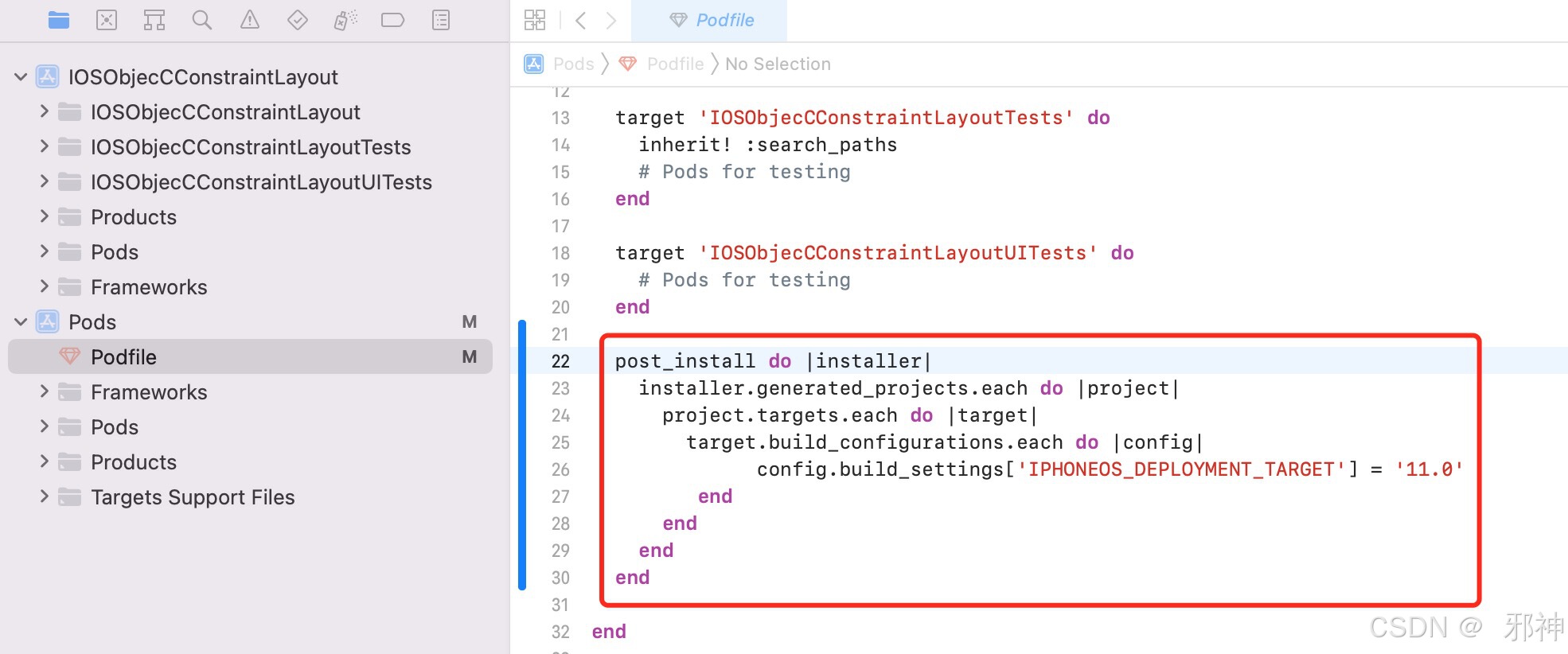
UITextView 居中
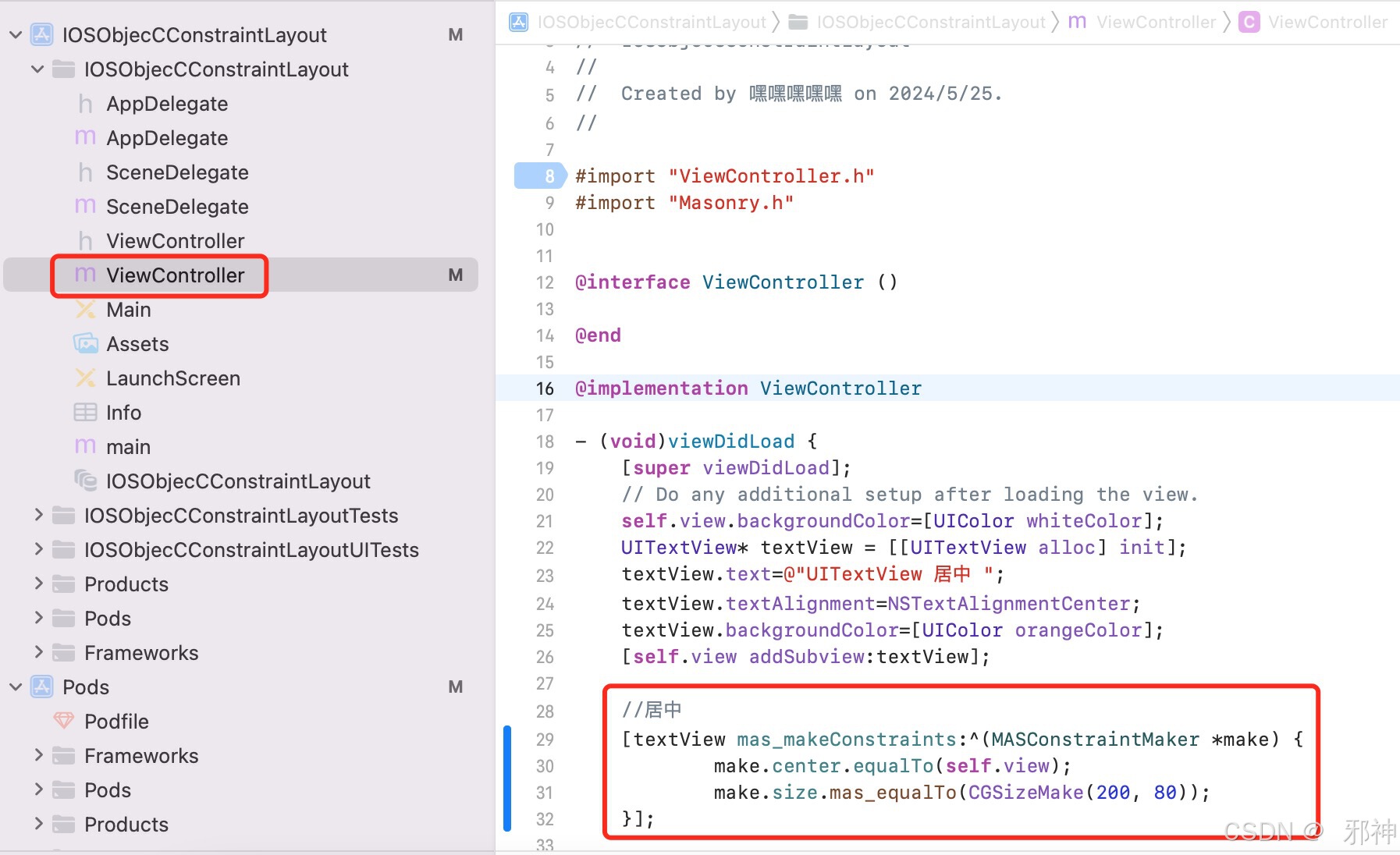
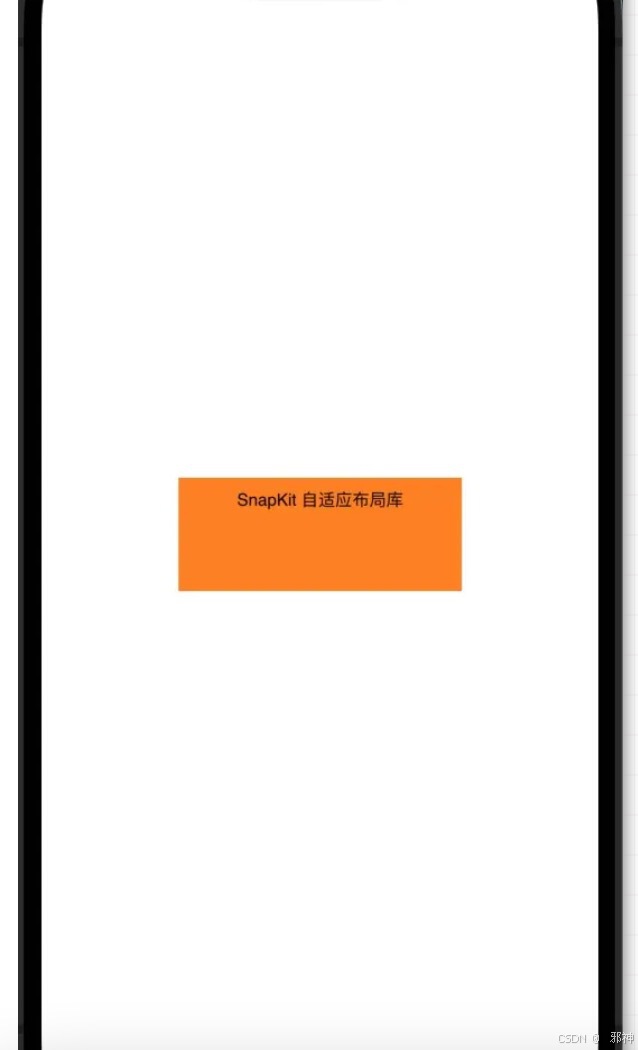
UITextView 垂直居中并且靠右
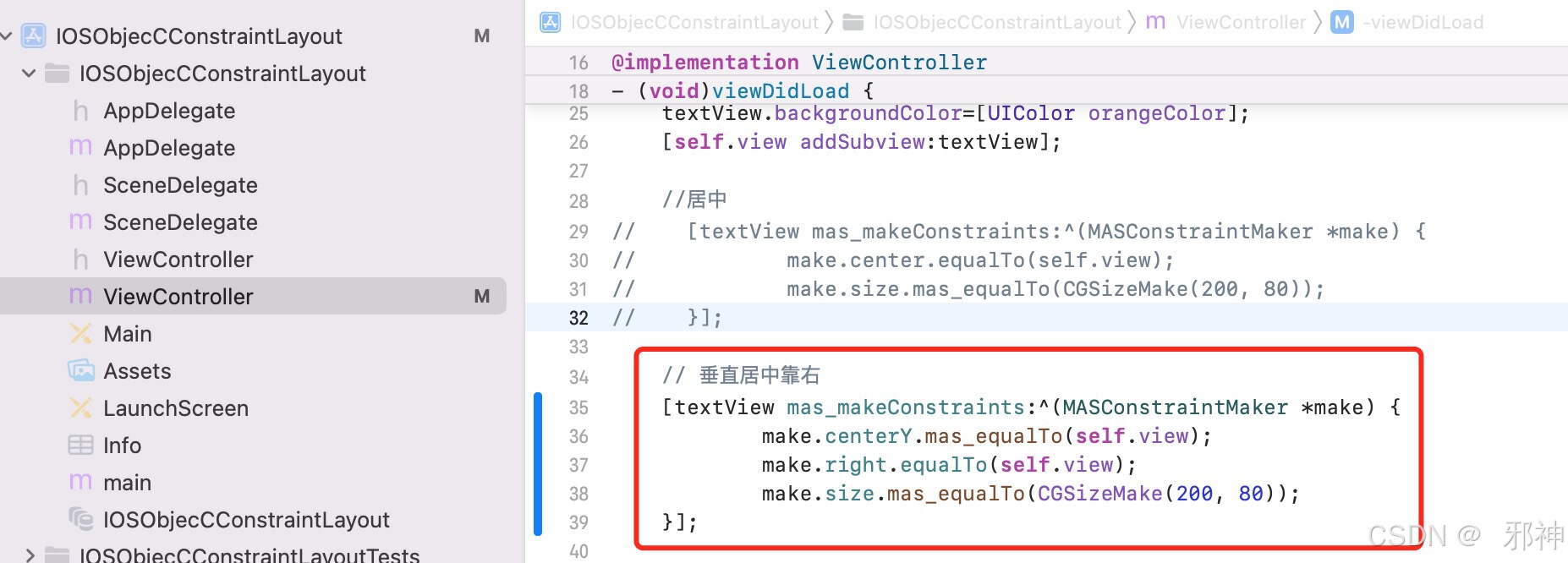
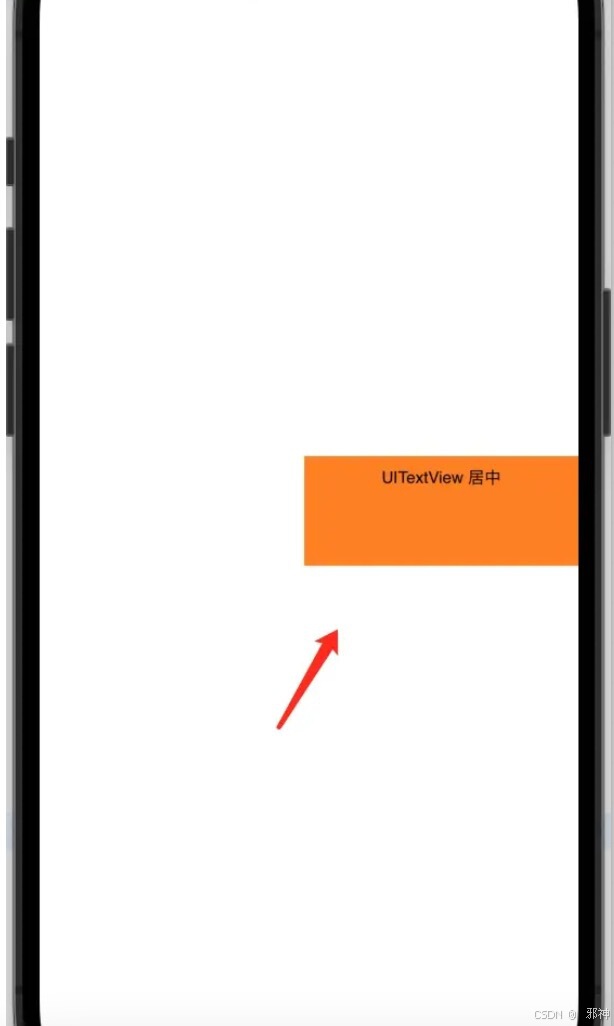
UITextView 宽和高的比例 2:1
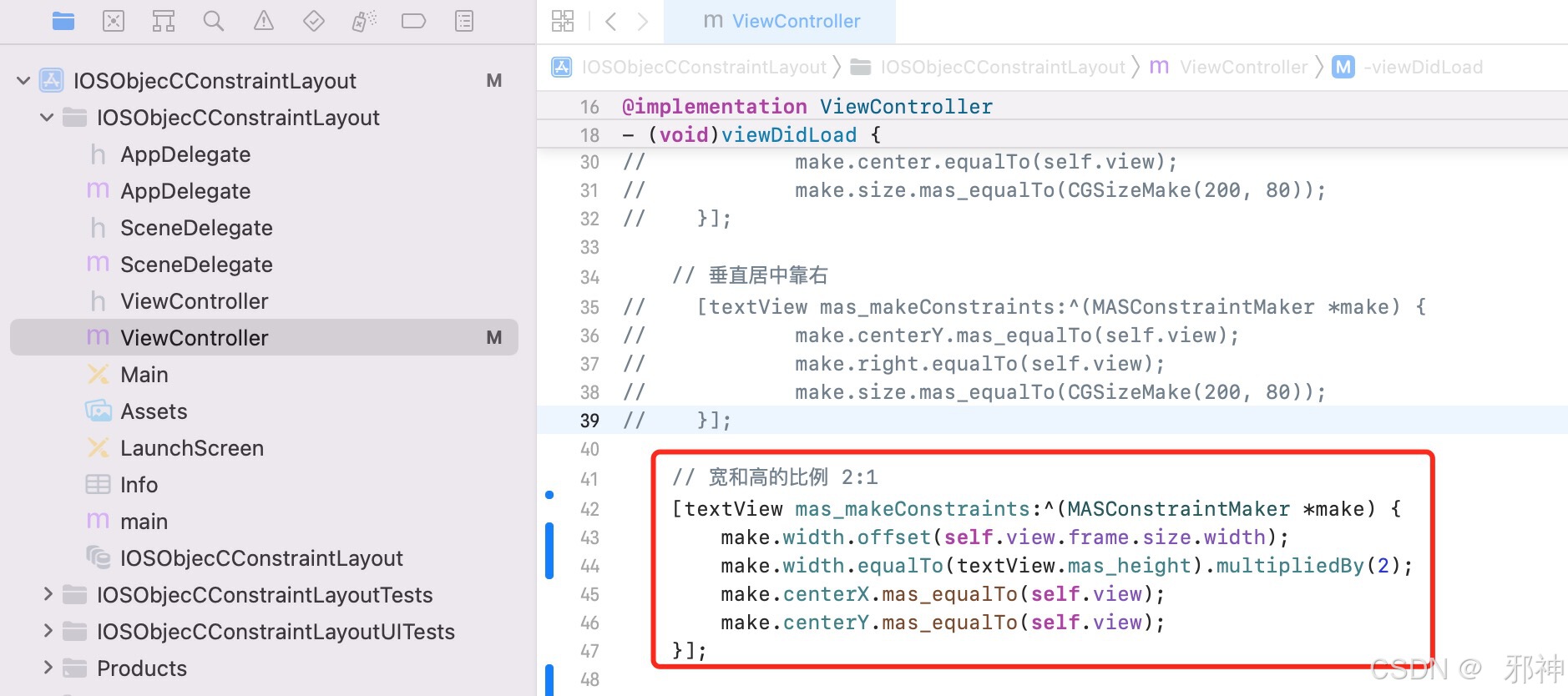
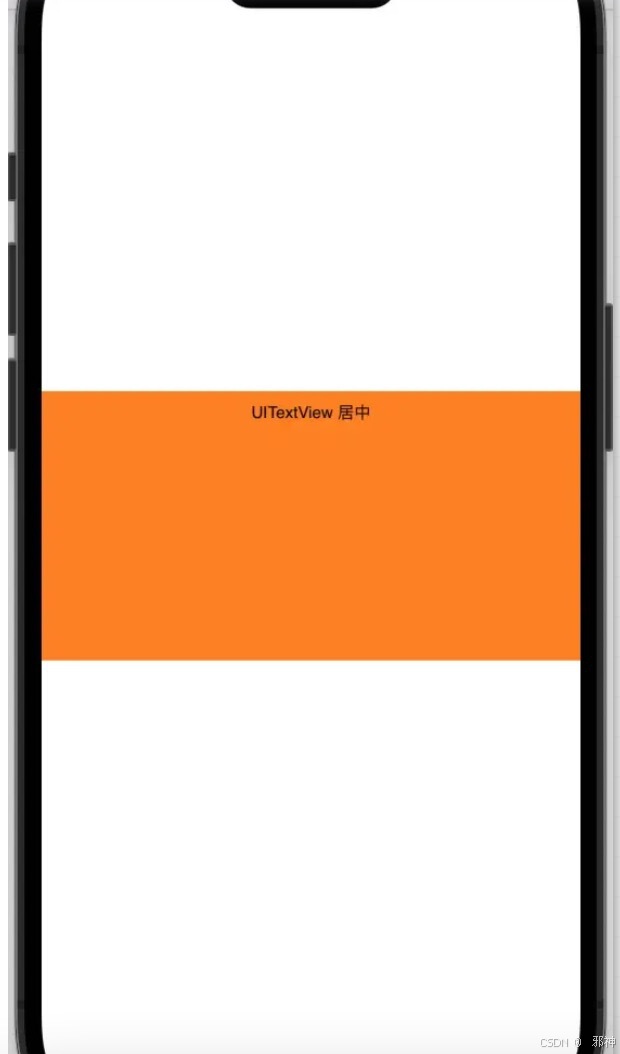
UITextView 最大宽度
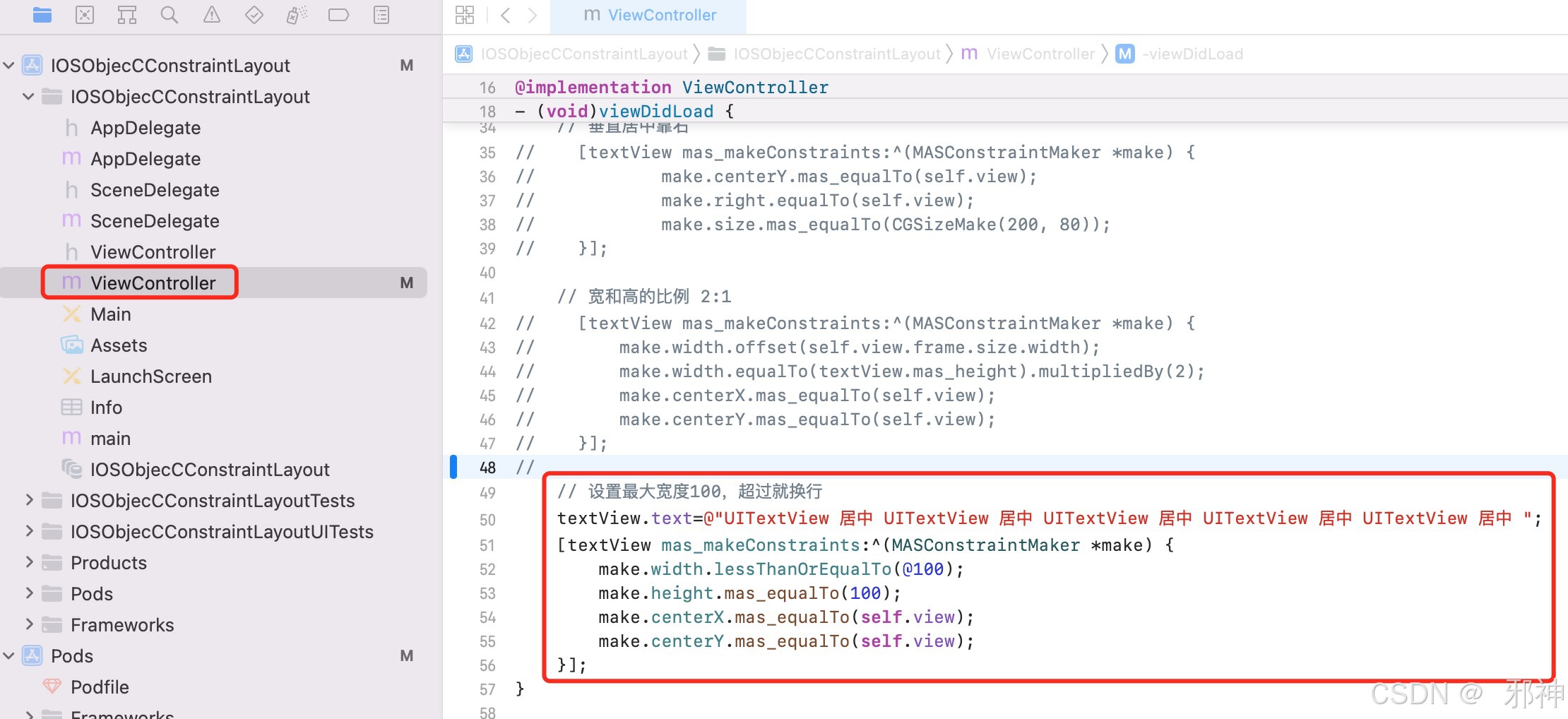
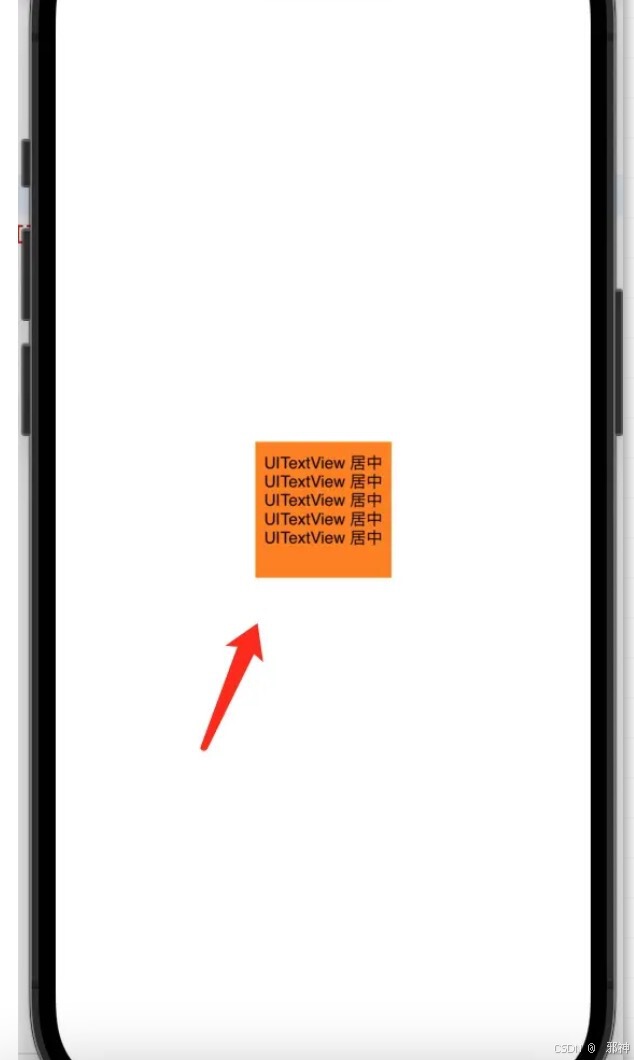
IOS Swifit 约束布局
pod init
如果不存在 Podfile 文件,切换到工程目录,就执行 pod init
命令
pod install
配置 Masonry
依赖 ,然后执行 pod install
命令
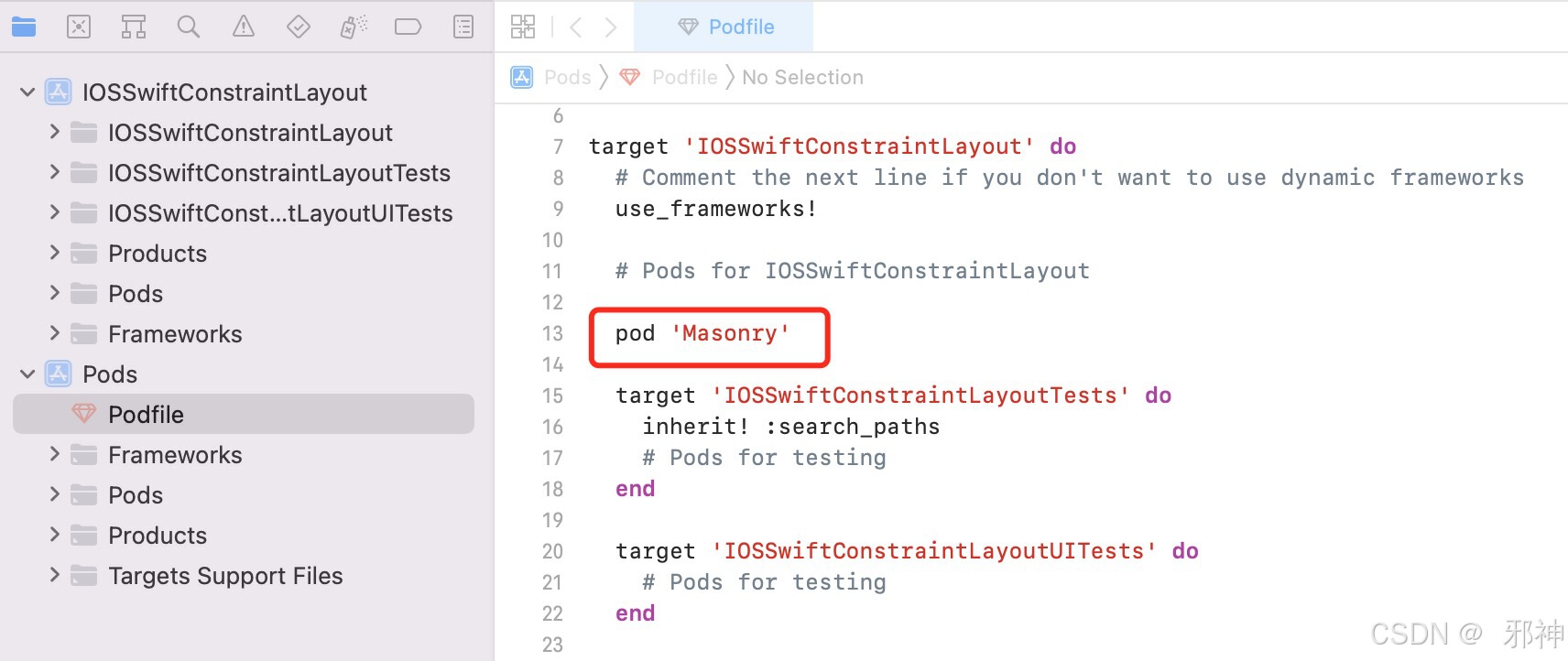
UITextView 居中
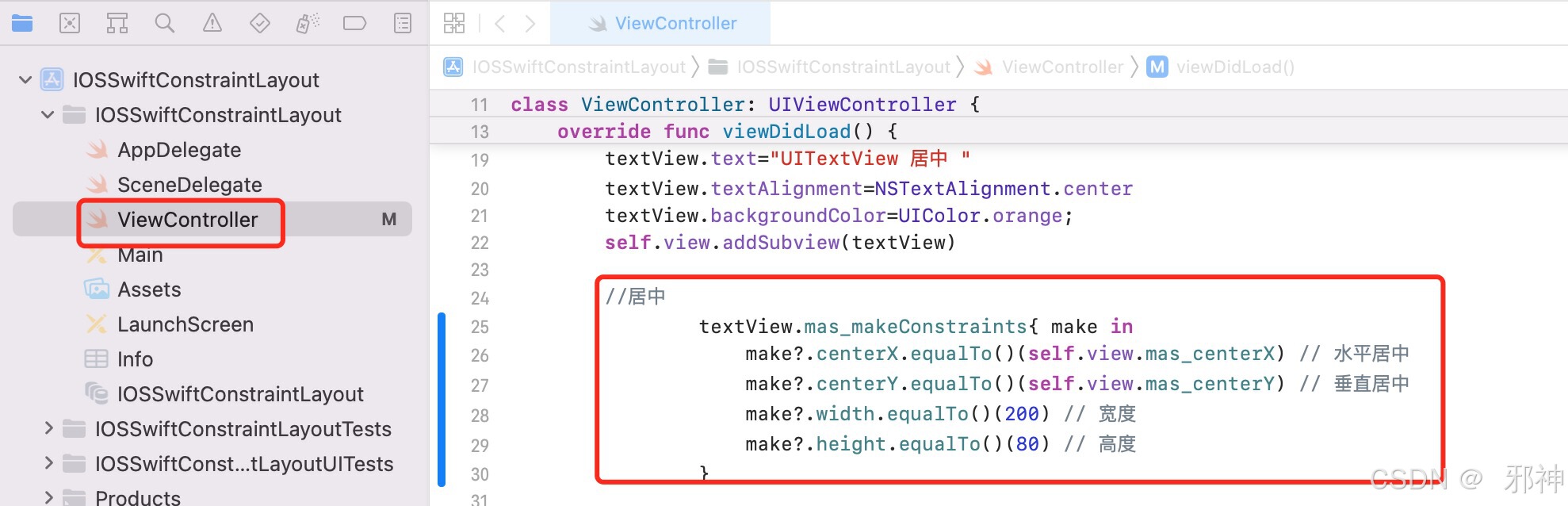
UITextView 垂直居中并且靠右
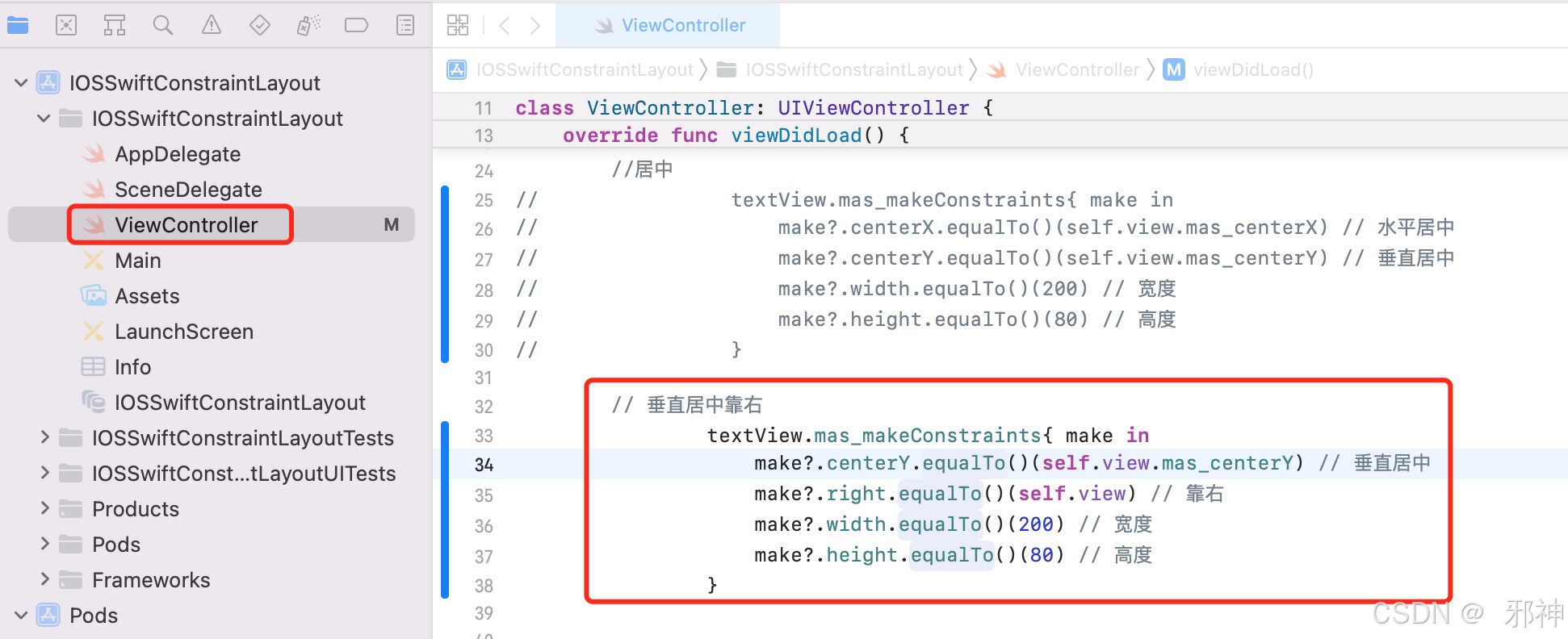
UITextView 宽和高的比例 2:1
UITextView 最大宽度
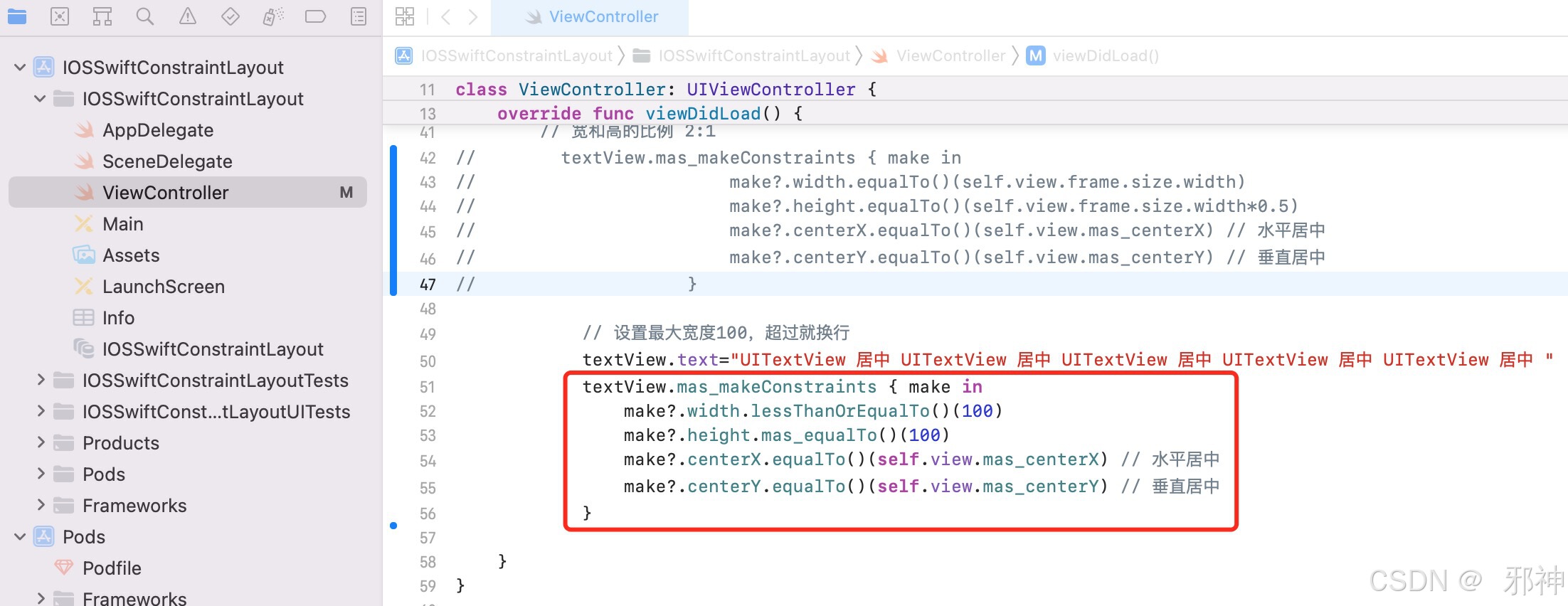
Flutter 约束布局
配置依赖
pubspec.yaml 里面配置 flutter_constraintlayout
依赖
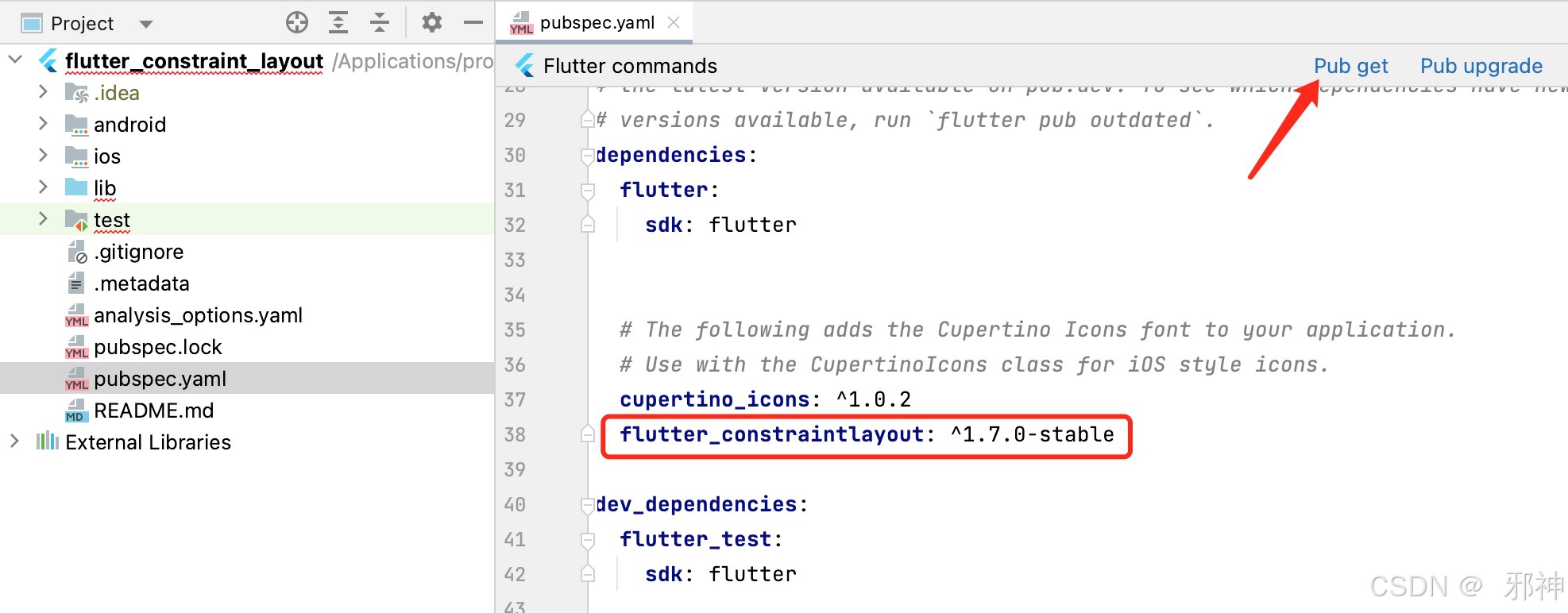
Flutter Text居中
Dart
// flutter text 居中显示
Widget textCenter() {
return ConstraintLayout(
children: [
Container(
color: Colors.orange,
alignment: Alignment.center,
child: const Text('flutter text 居中'),
).applyConstraint(
width: 200.0,
height: 100.0,
centerTo: parent, // Text 居中
)
],
);
}
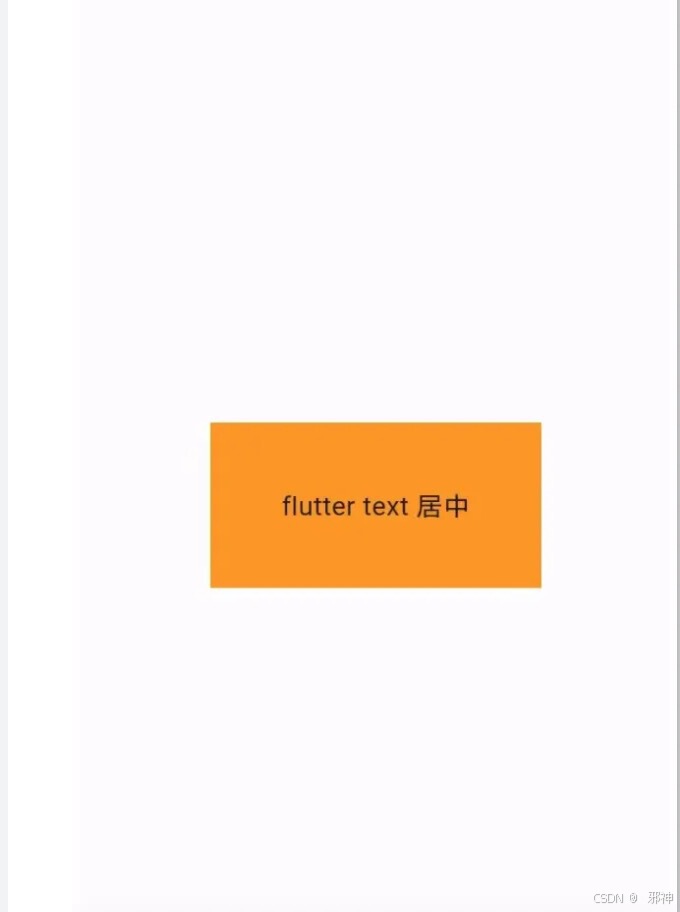
Flutter Text 垂直居中靠右显示
Dart
// flutter text 垂直居中靠右显示
Widget textVerticalCenterToRight() {
return ConstraintLayout(
children: [
Container(
color: Colors.orange,
alignment: Alignment.center,
child: const Text('flutter text 居中'),
).applyConstraint(
width: 200.0, height: 100.0, centerRightTo: parent // 垂直居中 靠右显示
)
],
);
}
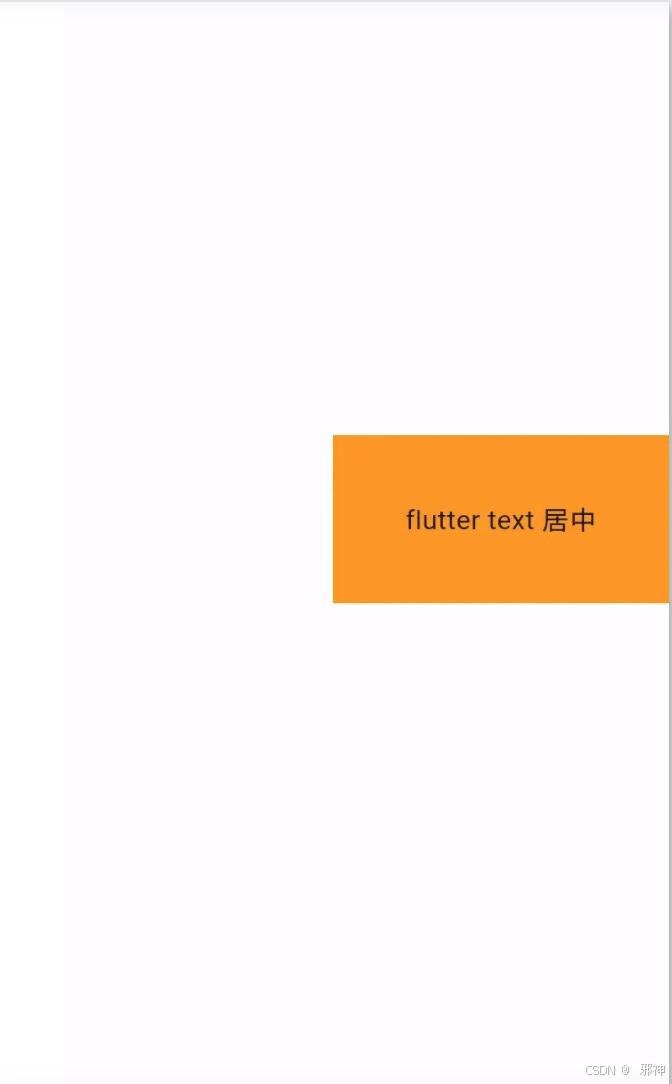
Flutter Text 宽高设置百分比
Dart
// flutter text 宽高设置百分比
Widget textWidthHeightPercent() {
return ConstraintLayout(
children: [
Container(
color: Colors.orange,
alignment: Alignment.center,
child: const Text('flutter text 宽高设置百分比'),
).applyConstraint(
width: matchConstraint,
height: matchConstraint,
heightPercent: 0.5,
widthPercent: 0.5,
centerTo: parent
)
],
);
}
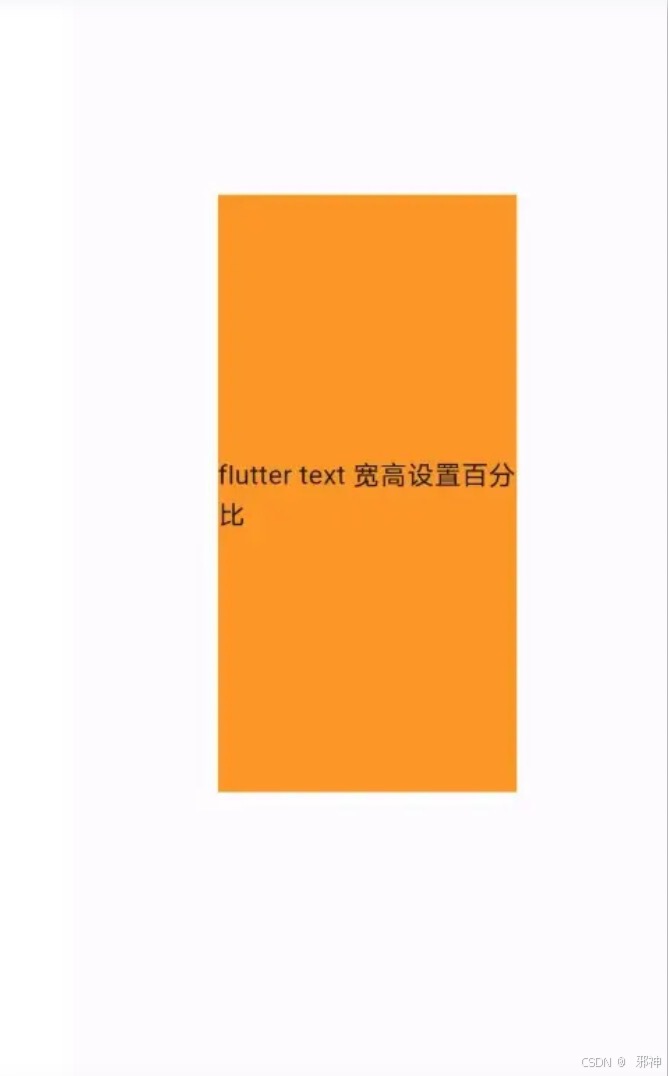
Flutter Text 宽和高的比例 2:1
Dart
// flutter 宽高比例 2:1
Widget textWidthHeightRatio() {
return ConstraintLayout(
children: [
Container(
color: Colors.orange,
alignment: Alignment.center,
child: const Text('flutter 宽高比例 2:1'),
).applyConstraint(
width: 300,
height: matchConstraint,
widthHeightRatio: 2 / 1,
centerTo: parent)
],
);
}
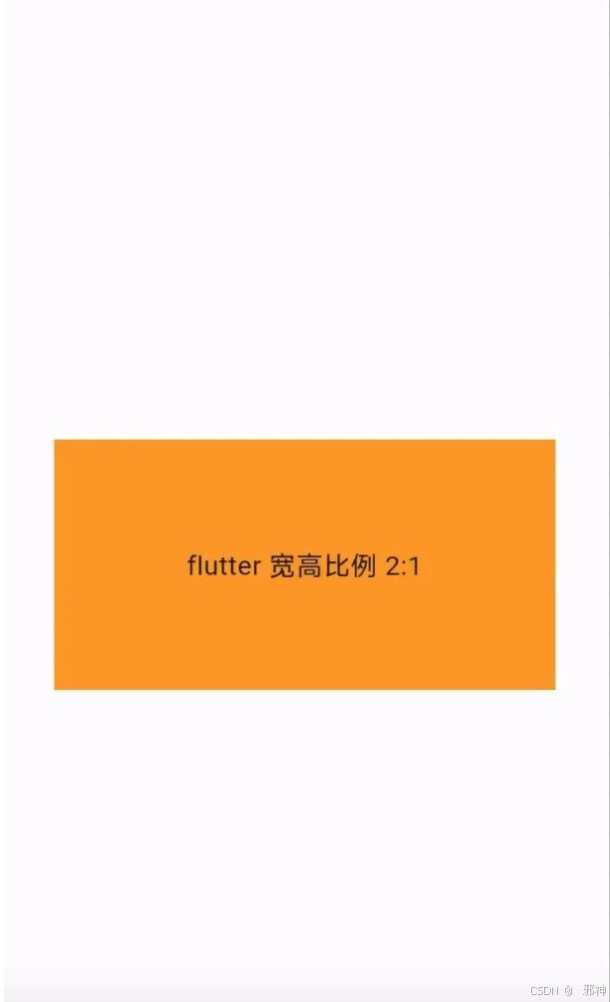
Flutter 设置最大宽度
Dart
// flutter 设置最大宽度
Widget textMaxWidth() {
return ConstraintLayout(
children: [
Container(
color: Colors.orange,
alignment: Alignment.center,
child:
const Text('flutter 最大宽度 flutter 最大宽度 flutter 最大宽度 flutter 最大宽度'),
).applyConstraint(maxWidth: 200, height: 400, centerTo: parent)
],
);
}
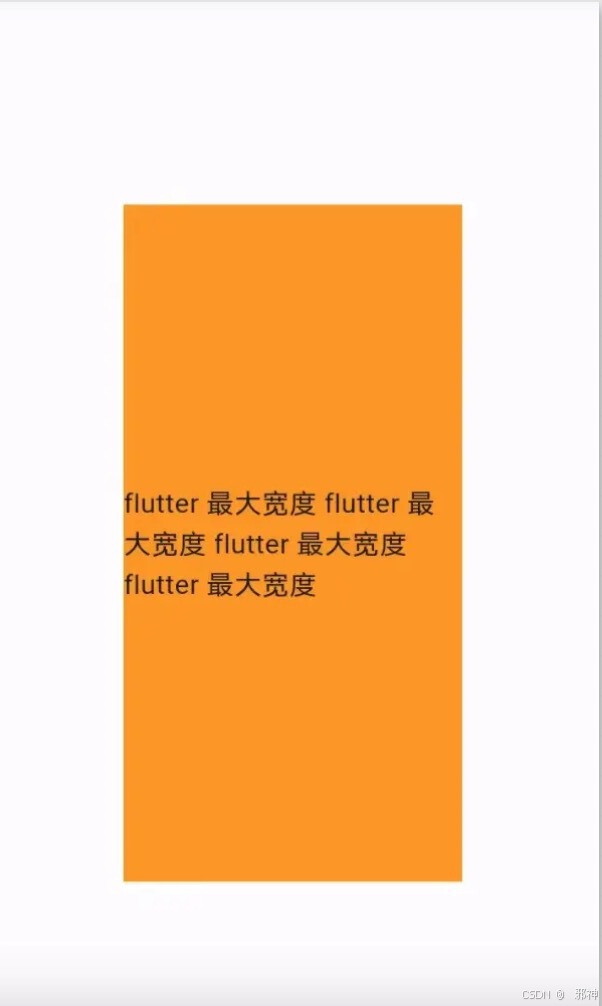
鸿蒙 布局 Flexbox
鸿蒙 Text 居中
Dart
@Entry
@Component
struct Index {
build() {
// 鸿蒙 Text 居中
Flex({ alignItems: ItemAlign.Center, justifyContent: FlexAlign.Center }) {
Text('鸿蒙 Text 居中').width('50%').height(80)
.textAlign(TextAlign.Center)
.backgroundColor(0xF5DEB3)
}.width('100%').height('100%').backgroundColor(0xFFFFFFFF)
}
}
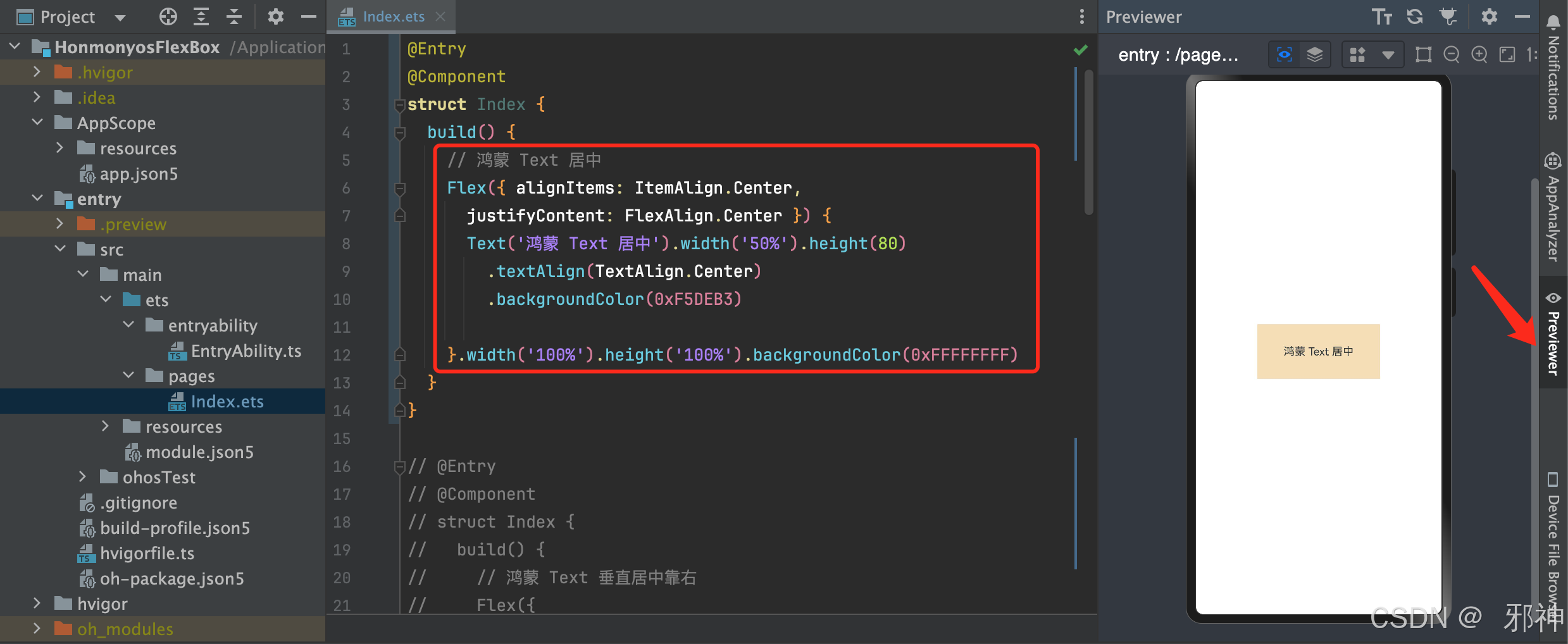
鸿蒙 Text 垂直居中靠右
Dart
@Entry
@Component
struct Index {
build() {
// 鸿蒙 Text 垂直居中靠右
Flex({
alignItems: ItemAlign.Center, //垂直居中
justifyContent: FlexAlign.End //水平靠右
}) {
Text('鸿蒙 Text 居中').width('50%').height(80)
.textAlign(TextAlign.Center)
.backgroundColor(0xF5DEB3)
}.width('100%').height('100%').backgroundColor(0xFFFFFFFF)
}
}
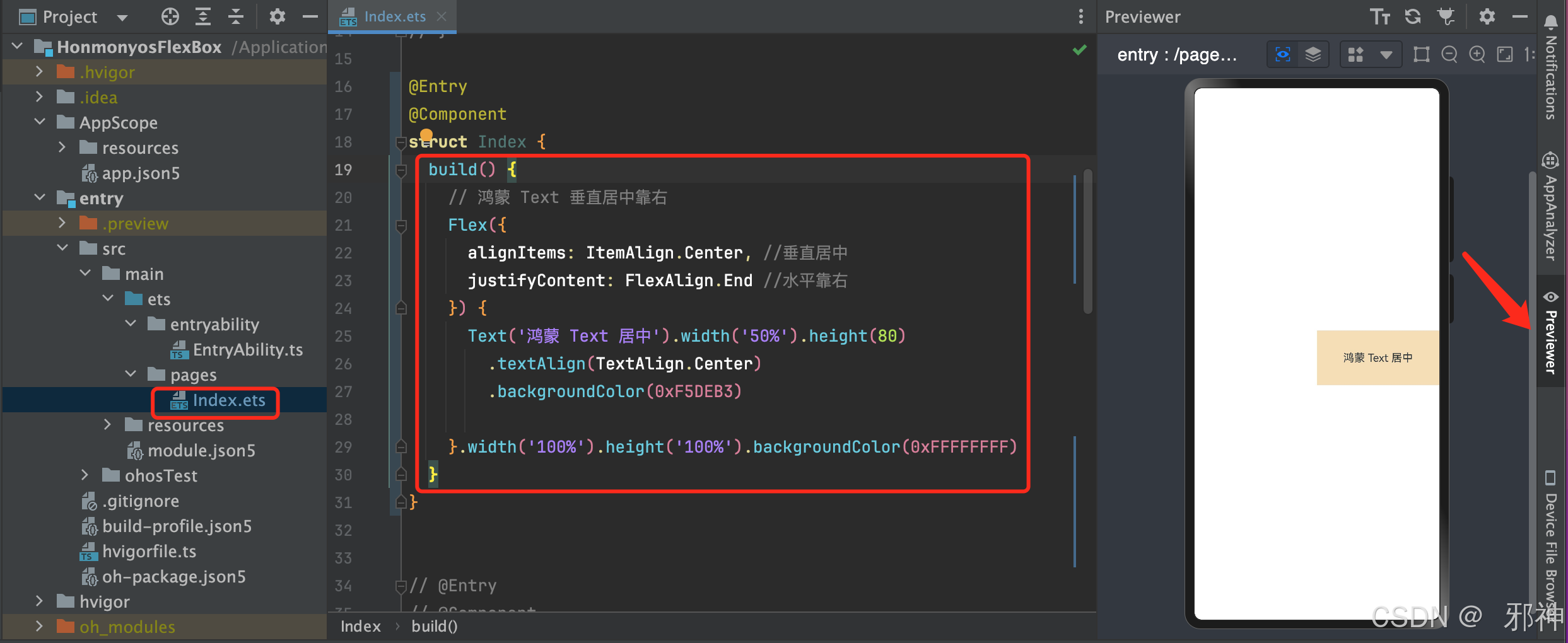
鸿蒙 宽和高的比例 2:1
Dart
@Entry
@Component
struct Index {
build() {
// 鸿蒙 宽和高的比例 2:1
Flex({
alignItems: ItemAlign.Center, //垂直居中
justifyContent: FlexAlign.End //水平靠右
}) {
Text('鸿蒙 宽和高的比例 2:1').width('100%')
.aspectRatio(2) //宽高比例 2:1
.textAlign(TextAlign.Center)
.backgroundColor(0xF5DEB3)
}.width('100%').height('100%').backgroundColor(0xFFFFFFFF)
}
}
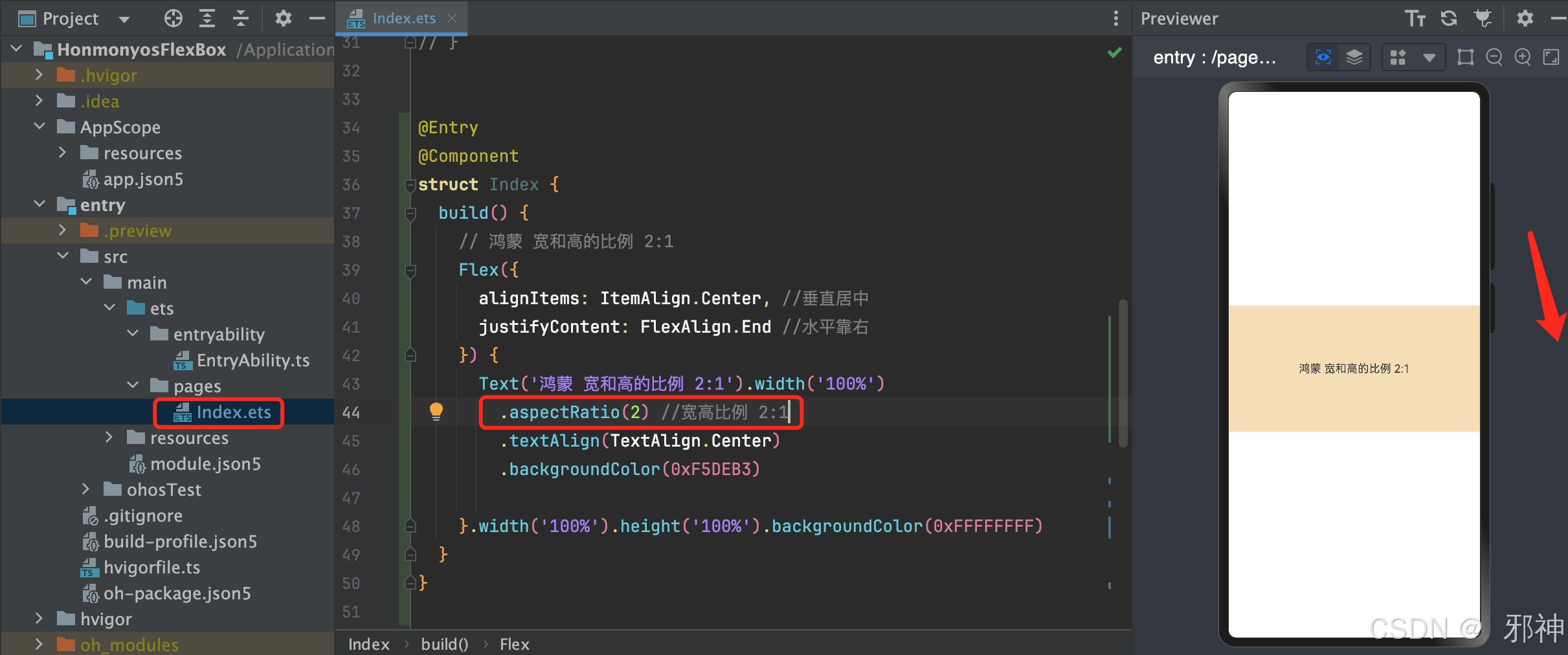
鸿蒙 Text 设置最大宽度
Dart
@Entry
@Component
struct Index {
build() {
// 鸿蒙 最大宽度
Flex({
alignItems: ItemAlign.Center, //垂直居中
justifyContent: FlexAlign.Center //水平居中
}) {
Text('鸿蒙 最大宽度 鸿蒙 最大宽度 鸿蒙 最大宽度 鸿蒙 最大宽度')
.constraintSize({
maxWidth: 160 //最大宽度
})
.textAlign(TextAlign.Center)
.backgroundColor(0xF5DEB3)
}.width('100%').height('100%').backgroundColor(0xFFFFFFFF)
}
}
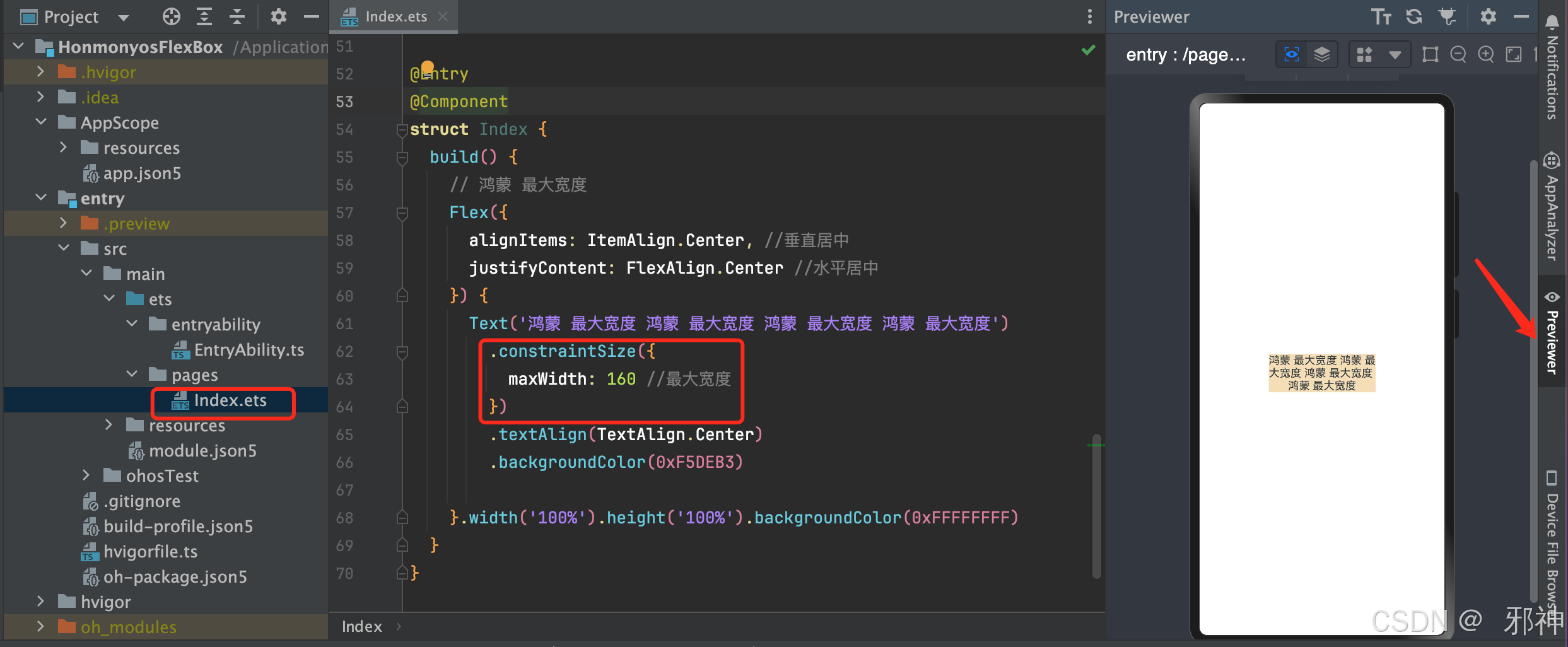
ReactNative Flexbox
ReactNative Text 居中
Dart
import React from 'react';
import {AppRegistry,View,Text } from 'react-native';
import App from './App';
import {name as appName} from './app.json';
const CenteredText = () => (
<View style={{
flex: 1,
justifyContent: 'center',//内容垂直居中
alignItems: 'center' // 内容水平居中
}}>
<Text style={{
backgroundColor: '#6200EE', // 背景颜色
height:60.0, //高度
width: '80%', // 宽度
textAlign: 'center', // 文本水平居中
color: '#ffffff', // 文本颜色
textAlignVertical: 'center',//文本垂直居中
}}>
React Native 居中文本
</Text>
</View>
);
AppRegistry.registerComponent(appName, () => CenteredText);
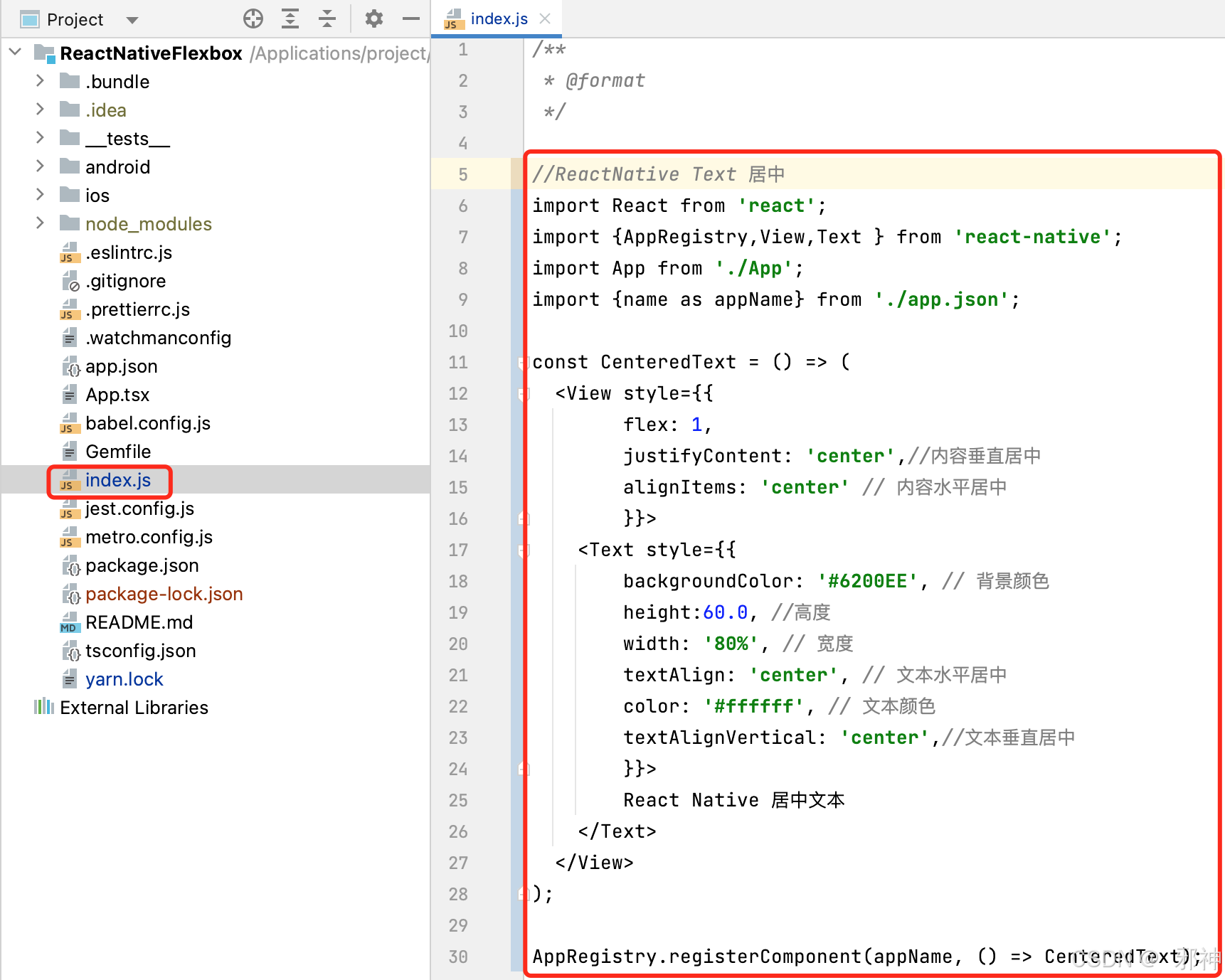
执行 npm install 安装项目所需要的依赖
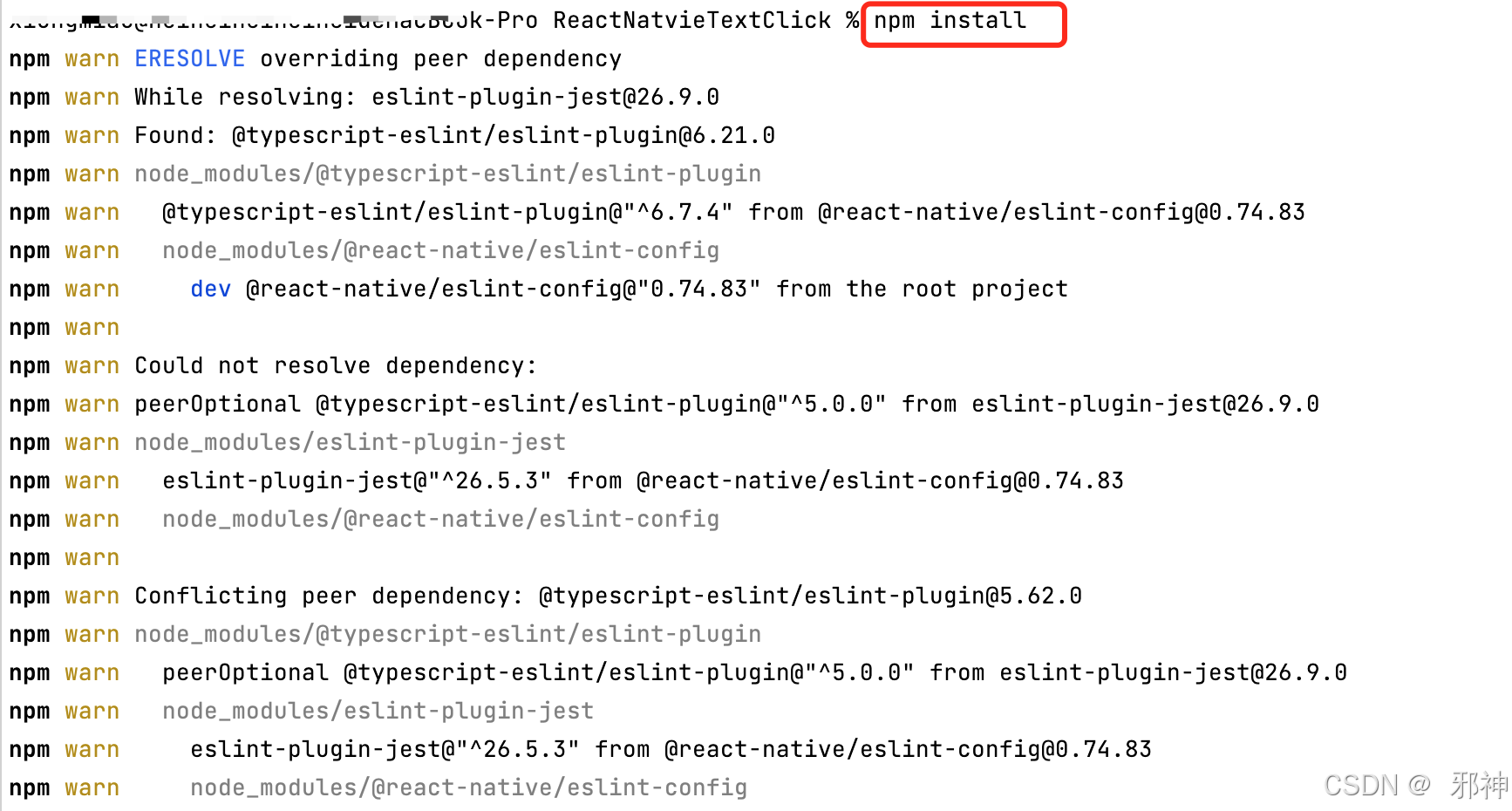
运行到安卓
采用 npx react-native run-android 或npm start 运行
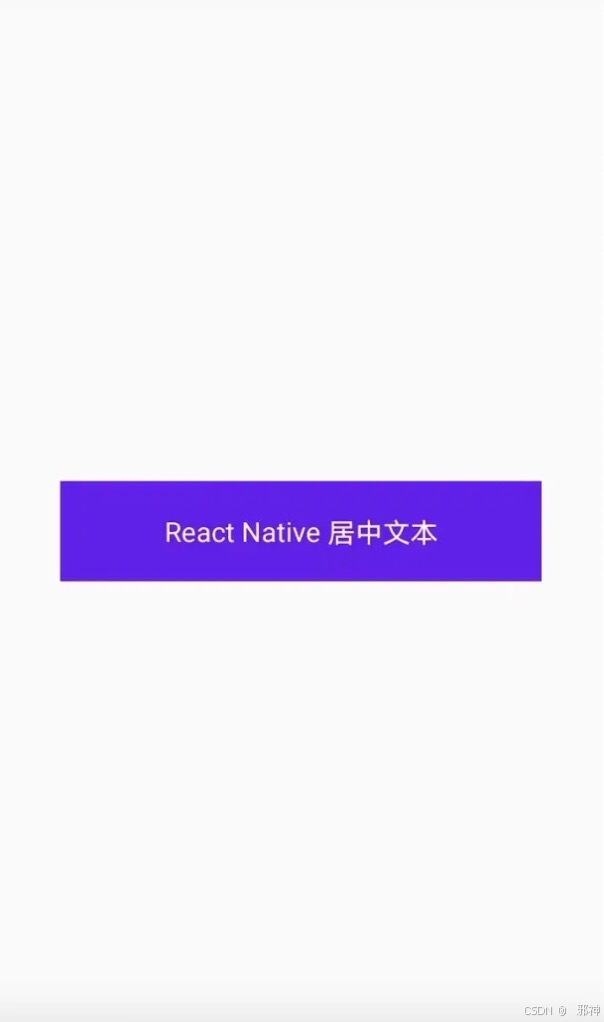
运行到IOS平台
采用 npx react-native run-ios 或npm start 运行
切换到iOS目录从新安装依赖
Dart
// 清除缓存
pod cache clean --all
//移出本地 pod文件依赖
pod deintegrate
//执行安装显示下载信息
pod install --verbose --no-repo-update
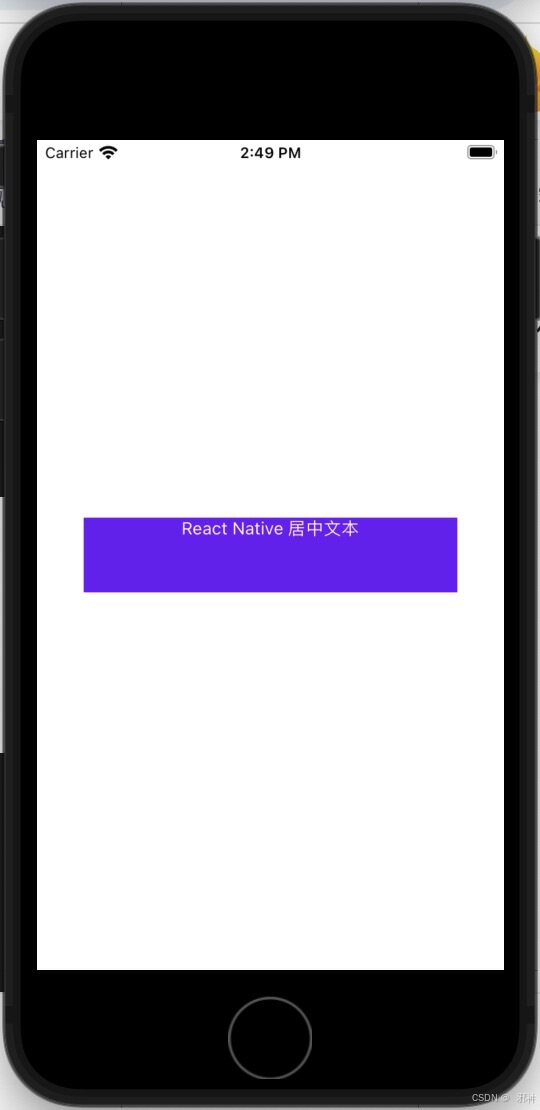
ReactNative Text 垂直居中并且靠右
Dart
import React from 'react';
import {AppRegistry,View,Text } from 'react-native';
import App from './App';
import {name as appName} from './app.json';
const CenteredText = () => (
<View style={{
flex: 1,
justifyContent: 'center',//内容垂直居中
alignItems: 'flex-end' // 内容居右
}}>
<Text style={{
backgroundColor: '#6200EE', // 背景颜色
height:60.0, //高度
width: '80%', // 宽度
textAlign: 'center', // 文本水平居中
color: '#ffffff', // 文本颜色
textAlignVertical: 'center',//文本垂直居中
}}>
React Native 居中文本
</Text>
</View>
);
AppRegistry.registerComponent(appName, () => CenteredText);
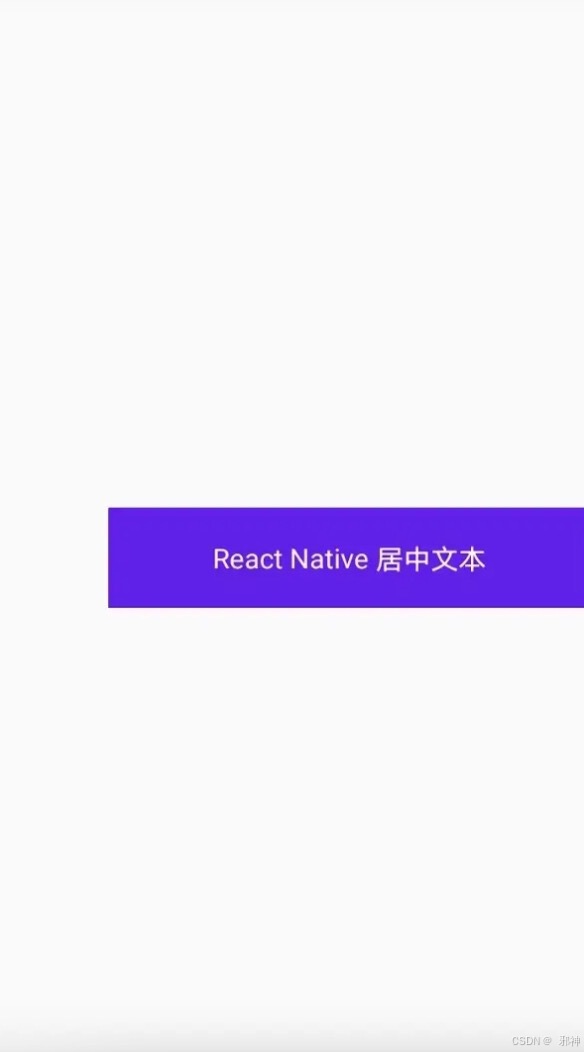
ReactNative Text 宽和高的比例 2:1
Dart
import React from 'react';
import {AppRegistry,View,Text } from 'react-native';
import App from './App';
import {name as appName} from './app.json';
const CenteredText = () => (
<View style={{
flex: 1,
justifyContent: 'center',//内容垂直居中
alignItems: 'center' // 内容水平居中
}}>
<Text style={{
backgroundColor: '#6200EE', // 背景颜色
width: '80%', // 宽度
textAlign: 'center', // 文本水平居中
color: '#ffffff', // 文本颜色
textAlignVertical: 'center',//文本垂直居中
aspectRatio: 2, // 宽高比为2:1
}}>
ReactNative Text 宽和高的比例 2:1
</Text>
</View>
);
AppRegistry.registerComponent(appName, () => CenteredText);
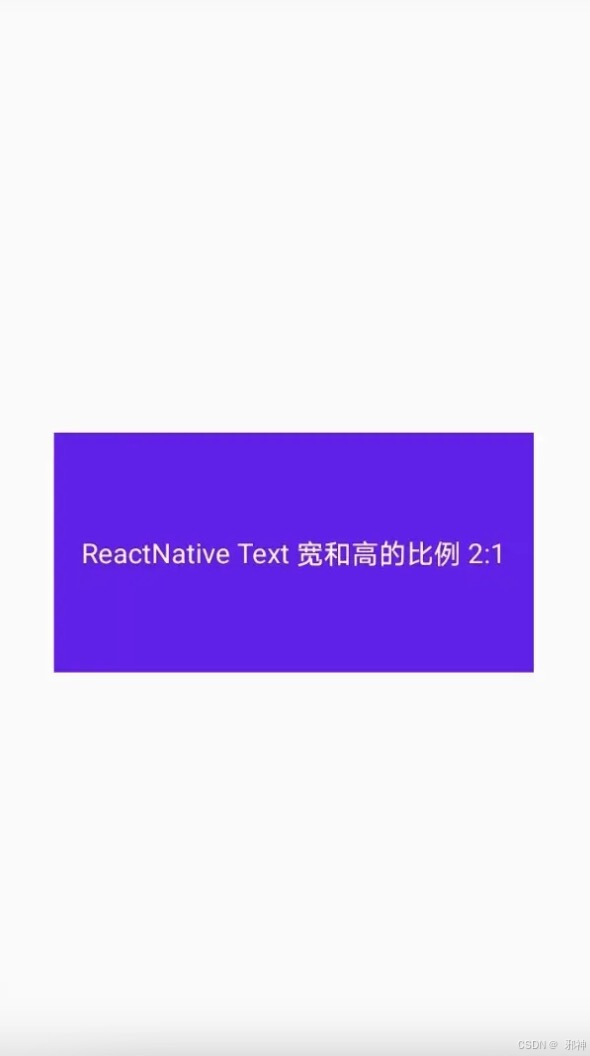
ReactNative Text 最大宽度
Dart
import React from 'react';
import {AppRegistry,View,Text } from 'react-native';
import App from './App';
import {name as appName} from './app.json';
const CenteredText = () => (
<View style={{
flex: 1,
justifyContent: 'center',//内容垂直居中
alignItems: 'center' // 内容水平居中
}}>
<Text style={{
backgroundColor: '#6200EE', // 背景颜色
maxWidth: '50%', // 最大宽度
textAlign: 'center', // 文本水平居中
color: '#ffffff', // 文本颜色
textAlignVertical: 'center',//文本垂直居中
}}>
ReactNative Text 最大宽度 ReactNative Text 最大宽度 ReactNative Text 最大宽度
</Text>
</View>
);
AppRegistry.registerComponent(appName, () => CenteredText);
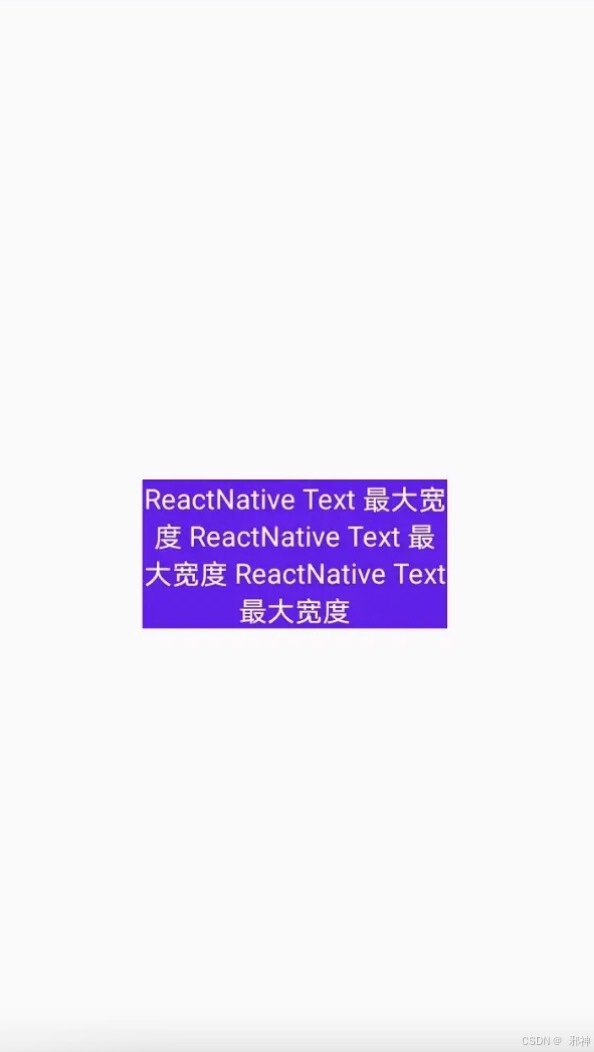
案例
所属分支
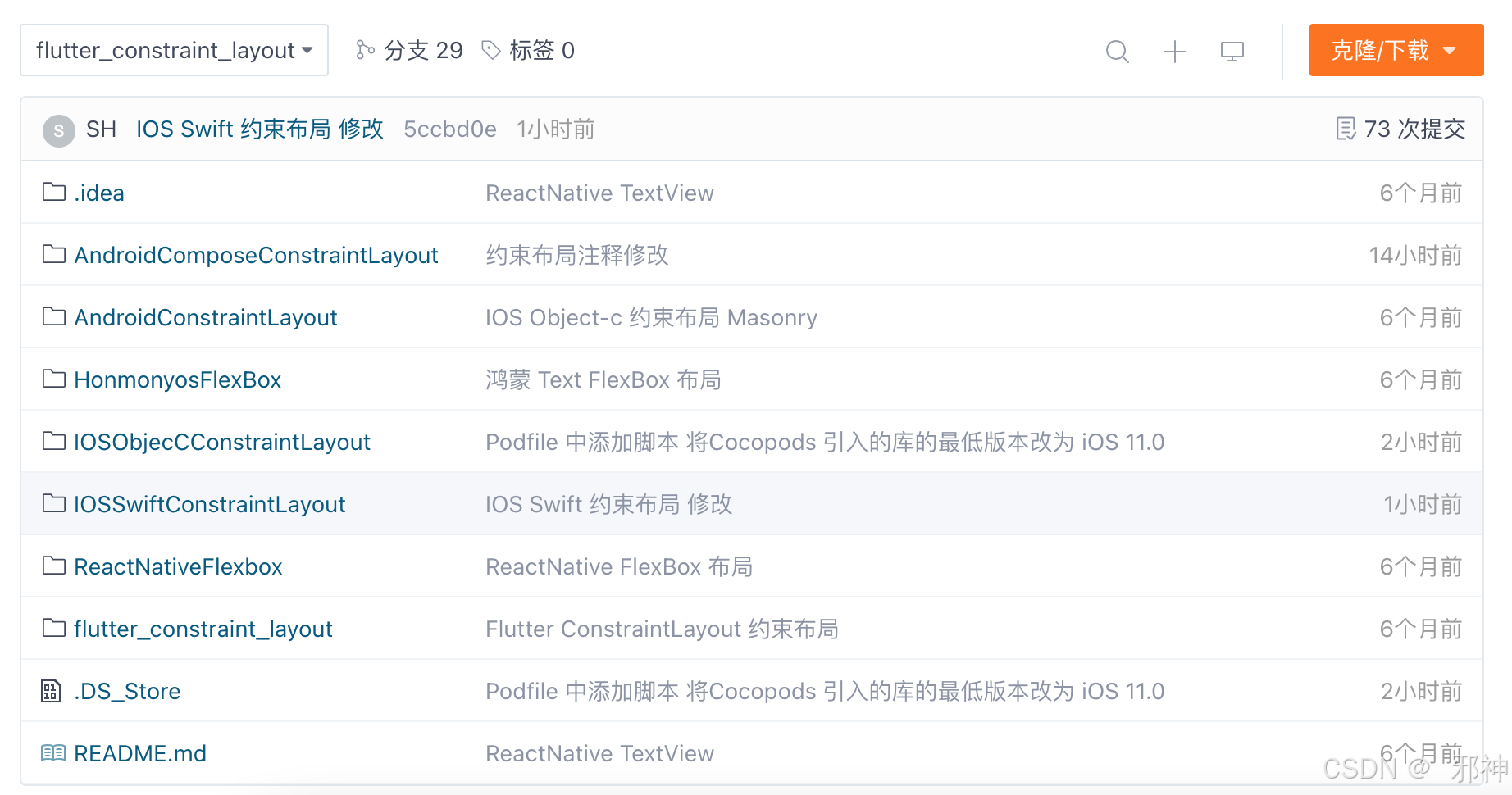