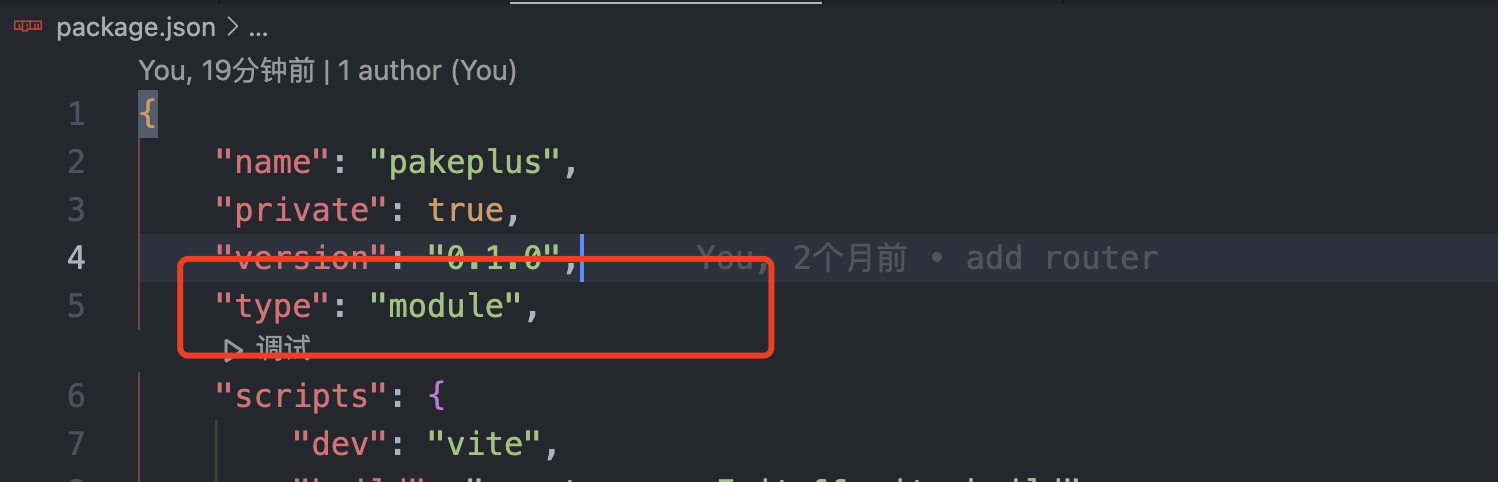
"type": "module"
是 Node.js 中 package.json
文件的一个字段,用于指示该项目的模块系统类型。它决定了项目中的 .js
文件应被视为 ECMAScript 模块 (ESM) 还是 CommonJS 模块 (CJS)。
含义和作用:
"type": "module"
:项目中的.js
文件将默认被视为 ECMAScript 模块 (ESM/ES6)。- 默认行为(未定义
"type"
) :项目中的.js
文件将默认被视为 CommonJS 模块 (CJS)。
区别:
-
CommonJS (
"type"
未定义或等于"type": "commonjs"
):- 使用
require()
和module.exports
。 - 文件扩展名
.cjs
通常用于明确表示 CommonJS 文件。
- 使用
-
ECMAScript 模块 (
"type": "module"
):- 使用
import
和export
语法。 - 文件扩展名
.mjs
通常用于明确表示 ECMAScript 模块文件。 - 不支持
require()
,只能使用import
语法。
- 使用
什么时候使用 "type": "module"
?
如果你正在编写现代 JavaScript 应用程序,并希望使用原生的 ESM 语法 (import
和 export
),可以在 package.json
中添加 "type": "module"
。这使得你的 .js
文件默认被解析为 ECMAScript 模块。
示例:
package.json
:
javascript
{
"name": "my-project",
"version": "1.0.0",
"type": "module"
}
文件中:
你可以使用 import
:
javascript
import path from 'path';
import fs from 'fs';
注意 : 如果你的项目中有需要同时使用 ESM 和 CJS 的文件,可以使用 .mjs
(ESM)和 .cjs
(CJS)扩展名来明确区分模块类型,而不用依赖 package.json
中的 "type"
字段。
所以我写了一个js脚本,使用require的时候报错了:
node ./cli/creatIcon.js
file:///Users/song/Project/my/PakePlus/cli/creatIcon.js:1
const path = require('path')
^
ReferenceError: require is not defined in ES module scope, you can use import instead
This file is being treated as an ES module because it has a '.js' file extension and '/Users/song/Project/my/PakePlus/package.json' contains "type": "module". To treat it as a CommonJS script, rename it to use the '.cjs' file extension.
这是因为你在项目的 package.json
中使用了 "type": "module"
,使得 Node.js 默认将 .js
文件视为 ES 模块(ESM)。在 ESM 模块中不能使用 require()
,需要使用 import
。你有两种解决方案:
解决方案 1:将文件扩展名改为 .cjs
将 creatIcon.js
文件重命名为 creatIcon.cjs
,这样 Node.js 会将其视为 CommonJS 模块,你可以继续使用 require()
。
解决方案 2:修改代码以使用 import
将 require()
替换为 import
语法,示例如下:
这两种方法都可以解决 require is not defined
的错误。
-
修改
creatIcon.js
:javascriptimport path from 'path'; import fs from 'fs'; import png2icons from 'png2icons'; import sharp from 'sharp'; // 你的其他代码逻辑
-
确保
package.json
中有"type": "module"
: 如果已经存在,则无需更改。
注意事项
import
语法只能在支持 ESM 的环境中使用(Node.js 版本必须是 12+)。- 如果使用
import
语法,文件加载等异步操作需要await
,并且在模块作用域中需要使用async
函数或top-level await
。