上一篇文章介绍了Spring MVC的注解,这篇文章再来做一些练习来巩固。
html文件要放在static当中
1. 加法器
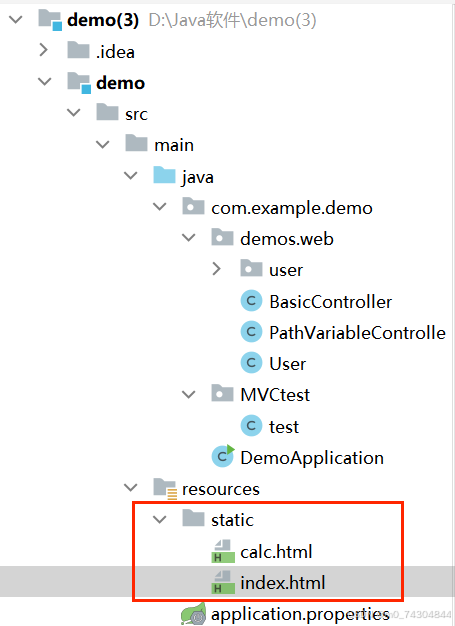
html
calc.html代码:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<form action="calc/sum" method="post">
<h1>计算器</h1>
数字1:<input name="a" type="text"><br>
数字2:<input name="b" type="text"><br>
<input type="submit" value=" 点击相加 ">
</form>
</body>
</html>
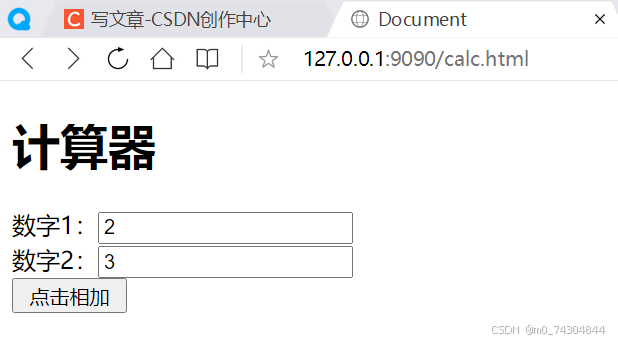
后端代码的形参名要跟前端html代码中的参数名相同
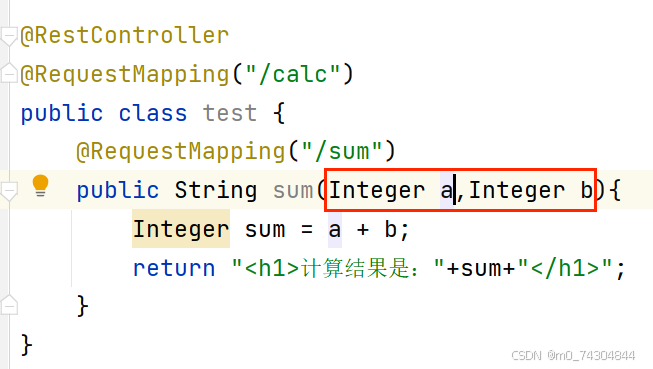
点击相加之后可以正确得到结果
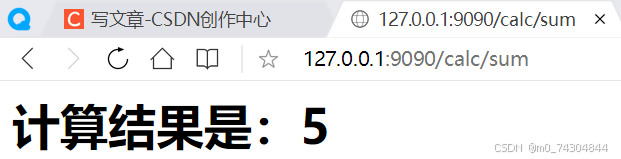
2. 登录
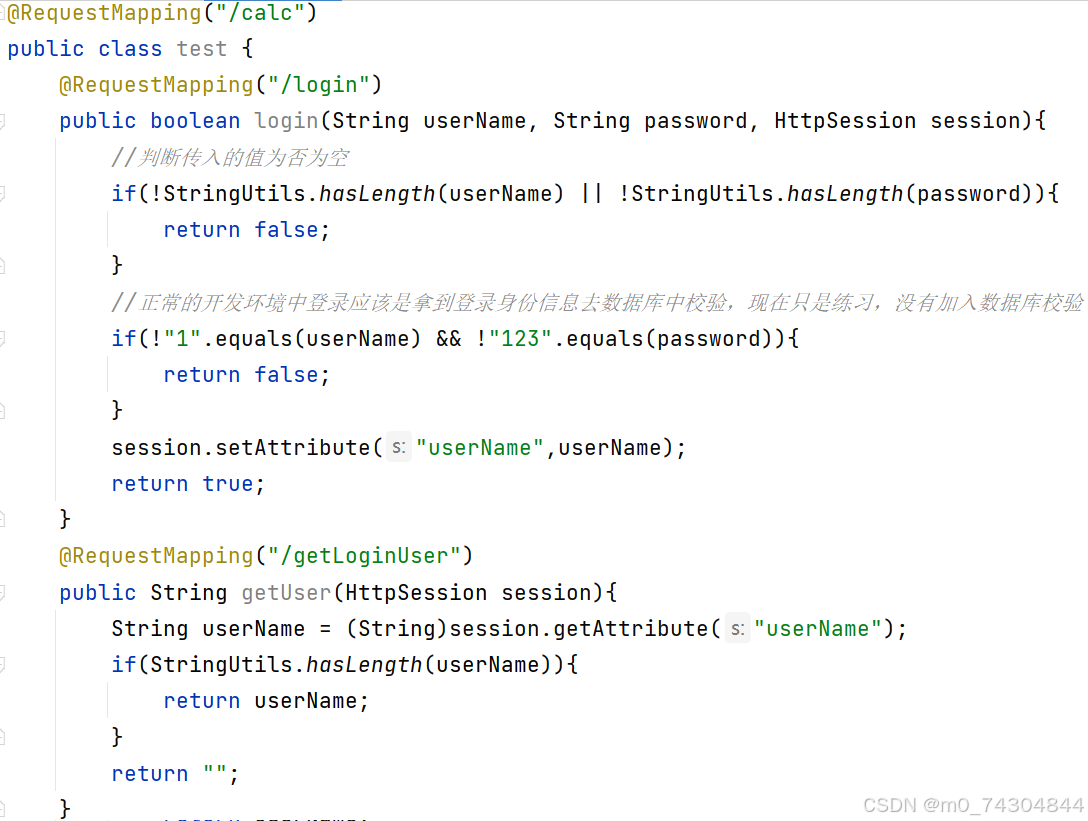
login.html代码:
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>登录页面</title>
</head>
<body>
<h1>用户登录</h1>
用户名:<input name="userName" type="text" id="userName"><br>
密码:<input name="password" type="password" id="password"><br>
<input type="button" value="登录" onclick="login()">
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.4/jquery.min.js"></script>
<script>
function login() {
$.ajax({
type: "post",
url: "/calc/login",
data: {
"userName": $("#userName").val(),
"password": $("#password").val()
},
success: function (result) {
if (result) {
location.href = "/index.html"
} else {
alert("账号或密码有误.");
}
}
});
}
</script>
</body>
</html>
html
这个index.html代码有一些问题,无法正常读取到登录的用户名
由于Java程序猿主要负责后端开发,前端的代码我不太擅长,
哪位大佬如果能看到问题在哪,麻烦指正一下,多谢!!
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>用户登录首页</title>
</head>
userName
<body>
登录人: <span id="userName"></span>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.4/jquery.min.js"></script>
<script>
$.ajax({
type: "get",
url: "/calc/getLoginUser",
success: function (result) {
$("#userName").text(result);
}
});
</script>
</body>
</html>
下面使用了直接访问路由映射的方式,可以正常响应,说明后端代码是没问题的,问题出在前端代码上。
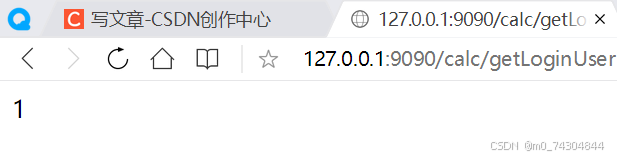
3. 留言板
引入lombok
java
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
先来看Java程序的运行原理
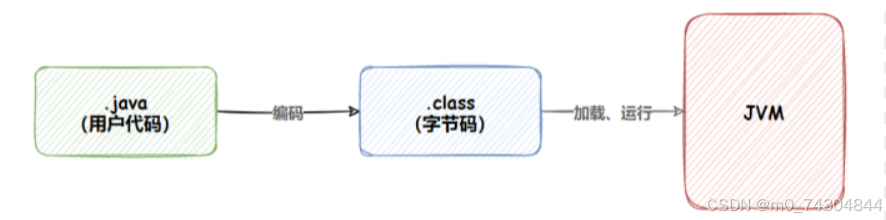
lombok的原理:
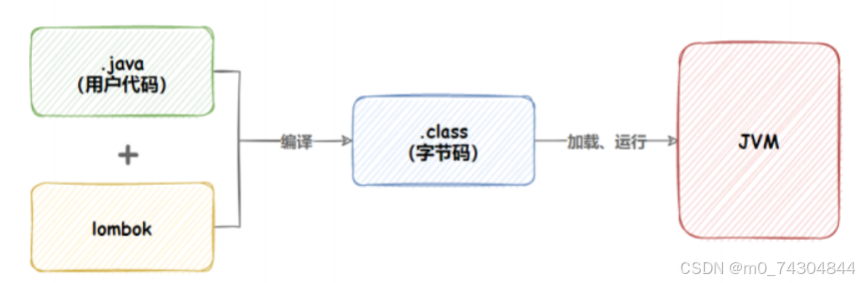
lombok中有一个注解@Data,这个
@Data = @Getter + @Setter + @ToString + @EqualsAndHashCode+@RequiredArgsConstructor + @NoArgsConstructor
|--------------------------|------------------------------------|
| 注解 | 作用 |
| @Getter | 自动添加getter方法 |
| @Setter | 自动添加setter方法 |
| @ToString | 自动添加toString方法 |
| @EqualsAndHashCode | 自动添加equals和hashCode方法 |
| @NoArgsConstructor | 自动添加无参构造方法 |
| @AllArgsConstructor | 自动添加全属性构造方法, 顺序按照属性的定义顺序 |
| @NonNull | 属性不能为null |
| @RequiredArgsConstructor | 自动添加必需属性的构造方法,final+@NonNull的属性为必须 |
举例说明一下:
java
@Data
public class Person {
public String name;
public Integer id;
public Integer password;
}
使用@Data注解后不需要自己再写getter和setter方法和toString方法,全部自动生成
自动包含下面内容
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", id=" + id +
", password=" + password +
'}';
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public Integer getPassword() {
return password;
}
public void setPassword(Integer password) {
this.password = password;
}
下面是留言板的代码
java
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>留言板</title>
<style>
.container {
width: 350px;
height: 300px;
margin: 0 auto;
/* border: 1px black solid; */
text-align: center;
}
.grey {
color: grey;
}
.container .row {
width: 350px;
height: 40px;
display: flex;
justify-content: space-between;
align-items: center;
}
.container .row input {
width: 260px;
height: 30px;
}
#submit {
width: 350px;
height: 40px;
background-color: orange;
color: white;
border: none;
margin: 10px;
border-radius: 5px;
font-size: 20px;
}
</style>
</head>
<body>
<div class="container">
<h1>留言板</h1>
<p class="grey">输入后点击提交, 会将信息显示下方空白处</p>
<div class="row">
<span>谁:</span> <input type="text" name="" id="from">
</div>
<div class="row">
<span>对谁:</span> <input type="text" name="" id="to">
</div>
<div class="row">
<span>说什么:</span> <input type="text" name="" id="say">
</div>
<input type="button" value="提交" id="submit" onclick="submit()">
<!-- <div>A 对 B 说: hello</div> -->
</div>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.4/jquery.min.js"></script>
<script>
function submit(){
//1. 获取留言的内容
var from = $('#from').val();
var to = $('#to').val();
var say = $('#say').val();
if (from== '' || to == '' || say == '') {
return;
}
//2. 构造节点
var divE = "<div>"+from +"对" + to + "说:" + say+"</div>";
//3. 把节点添加到页面上
$(".container").append(divE);
//4. 清空输入框的值
$('#from').val("");
$('#to').val("");
$('#say').val("");
}
</script>
</body>
</html>
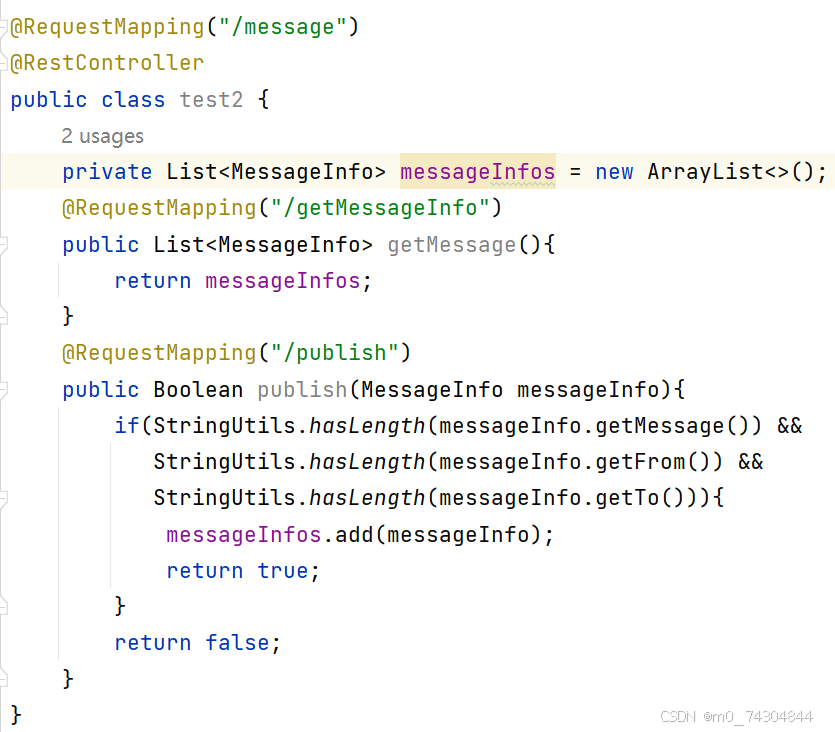
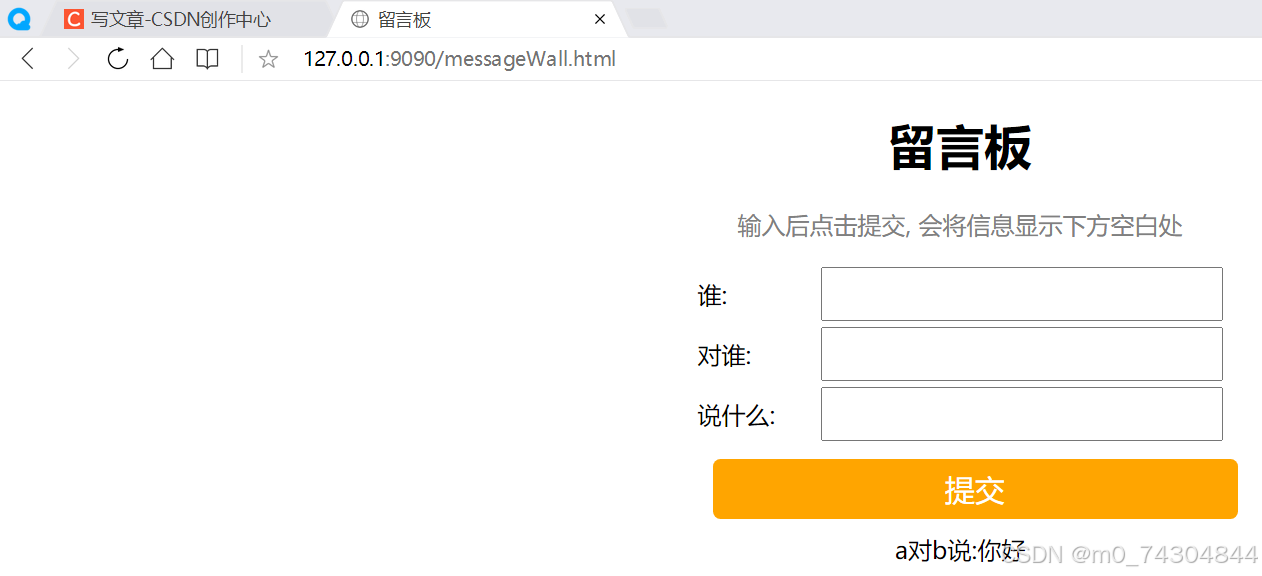