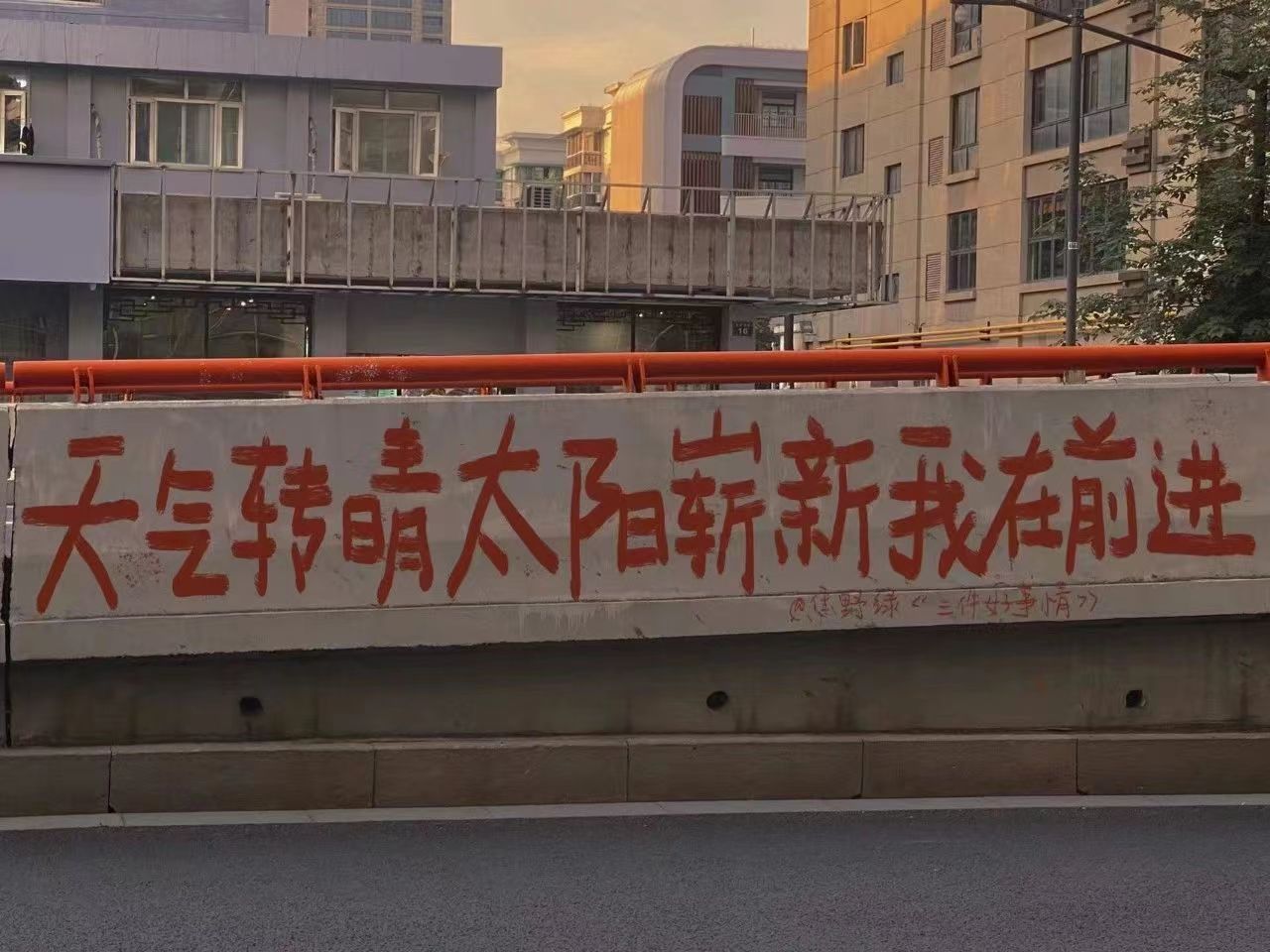
博主主页: 码农派大星.
数据结构专栏 :Java数据结构
数据库专栏: MySQL数据库
JavaEE专栏: JavaEE
软件测试专栏 :软件测试
关注博主带你了解更多知识

目录
[1. MyBatis-Plus介绍](#1. MyBatis-Plus介绍)
[2. 快速上⼿](#2. 快速上⼿)
[2.1 添加MyBatis-Plus和MySQL依赖,配置数据库连接信息](#2.1 添加MyBatis-Plus和MySQL依赖,配置数据库连接信息)
[2.2 编码](#2.2 编码)
[2.3 常⻅注解](#2.3 常⻅注解)
[2.4 打印⽇志](#2.4 打印⽇志)
[3. MyBatis-Plus复杂操作](#3. MyBatis-Plus复杂操作)
[3.1 QueryWrapper](#3.1 QueryWrapper)
[3.2 UpdateWrapper](#3.2 UpdateWrapper)
1. MyBatis-Plus介绍
MyBatis-Plus是⼀个 MyBatis 的增强⼯具, 在 MyBatis 的基础上只做增强不做改变, 为简化开发. 提⾼效率⽽⽣
⽀持数据库:
PostgreSQL, MySQL, MariaDB, Oracle, SQL Server, OceanBase, H2, DB2... 任何能使⽤MyBatis进⾏增删改查,并且⽀持标准SQL的数据库应该都在MyBatis-Plus的⽀持范围
2. 快速上⼿
2.1 添加MyBatis-Plus和MySQL依赖,配置数据库连接信息
Spring Boot
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-spring-boot3-starter</artifactId>
<version>3.5.5</version>
</dependency>
MySQL
<dependency>
<groupId>com.mysql</groupId>
<artifactId>mysql-connector-j</artifactId>
<scope>runtime</scope>
</dependency>
配置连接数据库
application.yml⽂件
# 数据库连接配置
spring:
datasource:
url: jdbc:mysql://127.0.0.1:3306/mybatis_test?
characterEncoding=utf8&useSSL=false
username: root
password: root
driver-class-name: com.mysql.cj.jdbc.Drive
2.2 编码
MybatisPlus提供了⼀个基础的 BaseMapper 接⼝,已经实现了单表的CRUD,我们⾃定义的 Mapper只需要继承这个BaseMapper,就⽆需⾃⼰实现单表CRUD
创建实体类UserInfo
实 体类的属性名与表中的字段名⼀⼀对应

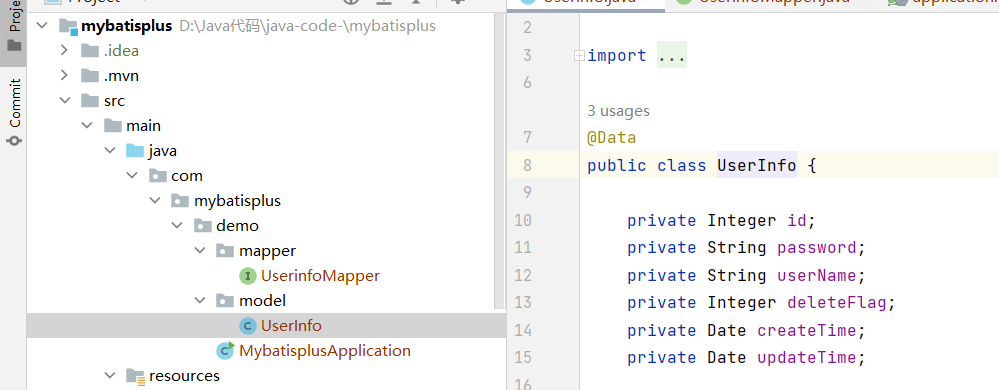
编写Mapper接⼝类
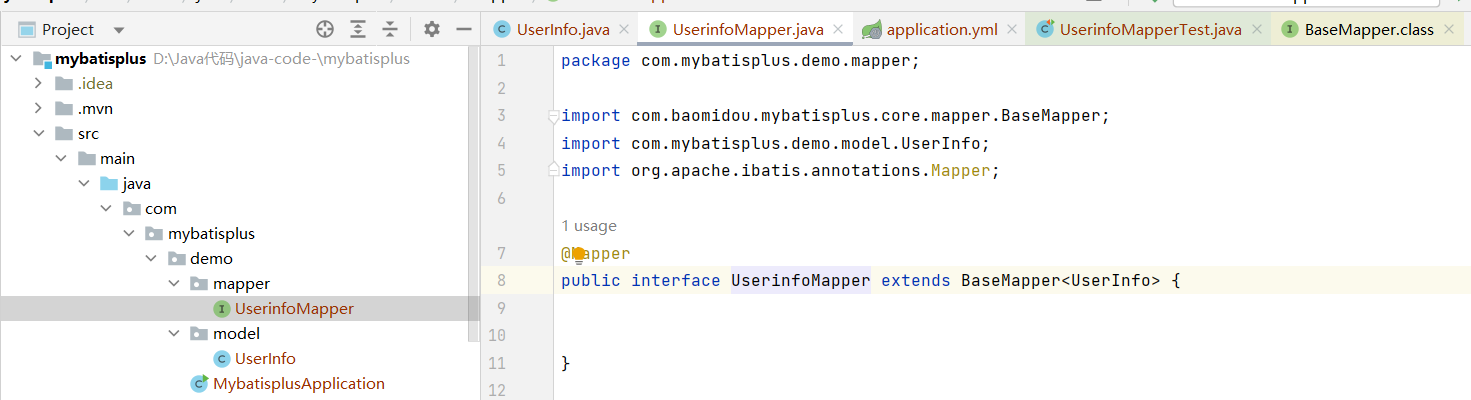
2.3 常⻅注解
MyBatis是如何知道,我们要操作的是哪张表,表⾥有哪些字段呢?
@Mapper
public interface UserinfoMapper extends BaseMapper<UserInfo> {
}
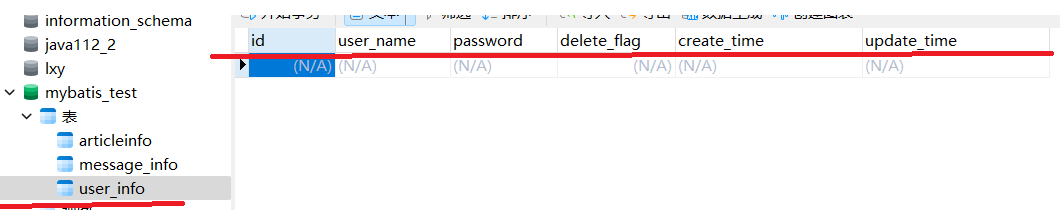
UserInfoMapper 在继承 BaseMapper 时,指定了⼀个泛型,这个UserInfo就是与数据库表相对应的实
MyBatis-Plus会根据这个实体类来推断表的信息.
默认情况下:
-
表名:实体类的驼峰表⽰法转换成蛇形表⽰法(下划线分割),作为表名.⽐如UserInfo->user_info
-
字段:根据实体类的属性名转换为蛇形表⽰法作为字段名.⽐如deleteFlag->delete_flag
-
主键:默认为id
那如果实体类和数据库不是按照上述规则定义的呢?MyBatis-Plus也给我们提供了⼀下注解,让我们标 识表的信息
@TableName

从⽇志可以看到,默认查找的表名为userinfo.
我们可以通过 @TableName 来标识实体类对应的表
修改实体类名Userinfo为user_info,重新执⾏测试⽅法
@Data
@TableName("user_info")
public class Userinfo {
private Integer id;
private String username;
private String password;
private Integer age;
private Integer gender;
private String phone;
private Integer deleteFlag;
private Date createTime;
private Date updateTime;
}
@TableField

我们可以通过 @TableField 来标识对应的字段名
修改属性名deleteflag为delete_flag,重新执⾏测试⽅法
@Data
@TableName("user_info")
public class Userinfo {
private Integer id;
private String username;
private String password;
private Integer age;
private Integer gender;
private String phone;
@TableField("delete_flag")
private Integer deleteflag;
private Date createTime;
private Date updateTime;
}
@TableId
我们可以通过 @TableId 来指定对应的主键
修改属性名userId为id
@Data
@TableName("user_info")
public class Userinfo {
@TableId("id")
private Integer userId;
private String username;
private String password;
private Integer age;
private Integer gender;
private String phone;
再次运⾏程序,程序运⾏结果正常
2.4 打印⽇志
Mybatis-Plus配置⽇志如下:
mybatis-plus:
configuration: # 配置打印 MyBatis⽇志
log-impl: org.apache.ibatis.logging.stdout.StdOutImpl
3. MyBatis-Plus复杂操作
条件构造器
QueryWrapper:⽤于构造查询条件,在AbstractWrapper的基础上拓展了⼀个select⽅法,允许指 定查询字段
UpdateWrapper:⽤于构造更新条件,可以在更新数据时指定条件
3.1 QueryWrapper
QueryWrapper并不只⽤于查询语句,⽆论是修改,删除,查询,都可以使⽤QueryWrapper来构建查询条件.
如何完成如下sql语句查询呢
SELECT id,username,password,age FROM user_info WHERE age = 18 AND username
"%min%"
测试如下:
@Autowired
private UserinfoMapper userinfoMapper;
@Test
void SelectByWrapper(){
QueryWrapper<UserInfo> queryWrapper = new QueryWrapper<>();
queryWrapper.select("username","password","gender")
.eq("age",18)
.like("username","min");
userinfoMapper.selectList(queryWrapper).forEach(System.out::println);
}
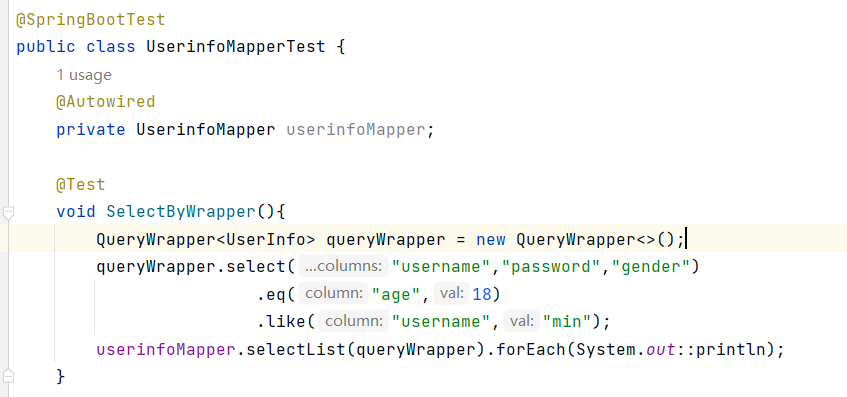

lt :"less than" 的缩写,表⽰⼩于.
le :"lessthanorequalto"的缩写,表⽰⼩于等于
ge :"greaterthanorequalto"的缩写,表⽰⼤于等于.
gt :"greaterthan"的缩写,表⽰⼤于.
eq :"equals"的缩写,表⽰等于.
ne :"notequals"的缩写,表⽰不等于
3.2 UpdateWrapper
对于更新,我们也可以直接使⽤UpdateWrapper,在不创建实体对象的情况下,直接设置更新字段和条 件
完成下列sql修改
UPDATE user_info SET delete_flag=0, age=5 WHERE id IN (2,3)
@Test
void updateByWrapper() {
UpdateWrapper<UserInfo> updateWrapper = new UpdateWrapper<>();
updateWrapper.set("delete_flag",0).set("age",5).in("id", List.of(2,3));
userinfoMapper.update(updateWrapper);
}
